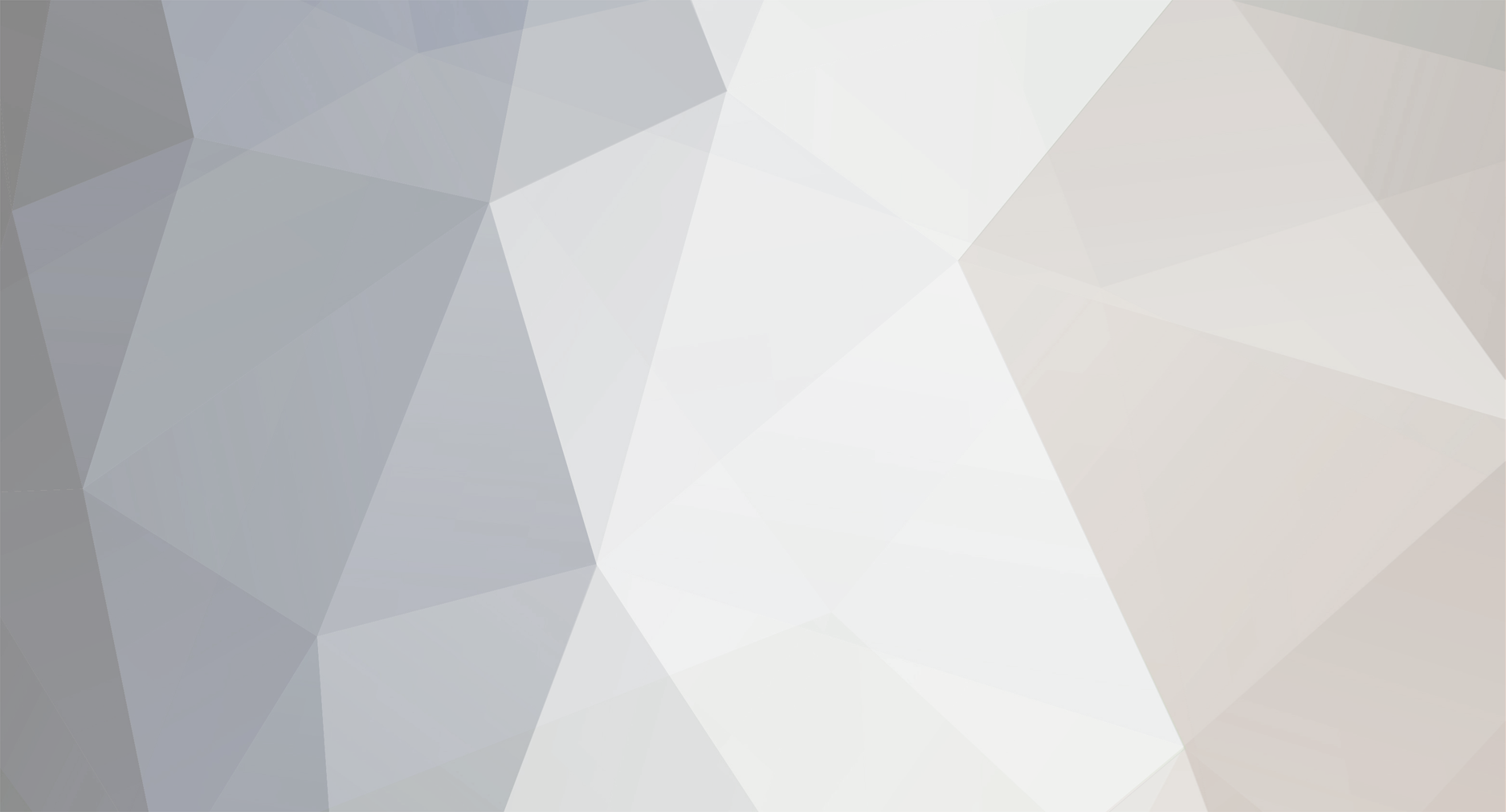
php_joe
-
Posts
175 -
Joined
-
Last visited
Never
Posts posted by php_joe
-
-
You probably have to set your headers to HTML, like this:
[code]<?php
$to = 'nobody@example.com';
$subject = 'the subject';
$message = 'hello';
$headers = 'MIME-Version: 1.0' . "\r\n";
$headers .= 'Content-type: text/html; charset=iso-8859-1' . "\r\n";
$headers .= 'From: Your Name <your_address@example.com>' . "\r\n";
mail($to, $subject, $message, $headers);
?>[/code] -
Hi,
I'm playing around with a new proxy.
I noticed with other proxies that their ip address get's blocked because so many people use it.
I know that you can get the viewer's IP address using [b]$_SERVER['REMOTE_ADDR'][/b]
Is there a way to change what is return using the remote address superglobal?
Thanks,
Joe -
Thank you very much!
-
I want to break up a word into it's individual letters so that I can stick things between them, reverse them, whatever.
I knocked up this bit of code:
[code]$word = "Mary had a little lamb";
$wordcount = strlen($word);
$i = "0";
while($i < $wordcount){
$key = "$i";
echo "$word[$key]";
$i = ($i + 1);
}[/code]
which works, but I'm sure that there's a better way to do it. Is there a way to explode the characters in the text or something?
Thanks,
Joe -
[quote author=fert link=topic=98964.msg389509#msg389509 date=1151651557]
use change the file extension to .php and then you can use php and html
[/quote]
I think he means without changing the extension (for example: so it runs on a website that is not php enabled, such as geocities).
I've heard that you can do this with JavaScript. They always say to use this:
[code]<script language="javascript" src="http://whatever/php_script.php."> </script>[/code]
But that's never worked for me. I've also seen this suggested:
[code]<img src="php_script.php" />[/code]
But I only get a red X when I tried it.
Feel free to try them if you like.
I did find a JavaScript snippit that did return the php output, but only the output from the php file and nothing from the original html webpage (no code... nothing). Not only that, but when I tried to refresh the page (after deleting the JavaScript) it wouldn't change. The php output always showed up even with no code in the html file and the php file renamed!
I'm also interested in a way to display a php output on an html page (aside from using an iframe) on a server such as geocities that doesn't support php.
Joe -
Thanks wildteen88! It worked.
And thanks for the suggestion Buyocat. It's always a good idea to make it as simple as possible. -
Ok,
I have a file with a table on it:
[code]<table>
<tr><td>Pet</td><td>Owner</td><td>Location</td></tr>
<tr><td>Cat</td><td>John</td><td>City</td></tr>
<tr><td>Dog</td><td>Amy</td><td>City</td></tr>
<tr><td>Dog</td><td>Wayne</td><td>Country</td></tr>
<tr><td>Cat</td><td>Sarah</td><td>City</td></tr>
<tr><td>Cat</td><td>Jack</td><td>Country</td></tr>
</table>[/code]
I want to only show the rows with Cats. So far I have:
[code]$content = file_get_contents($url);
$content[1] = str_replace("<table>", "", $content[1]);
$content[1] = str_replace("<tr><td>", "", $content[1]);
$content[1] = str_replace("</td></tr>", "", $content[1]);
$content[1] = str_replace("</table>", "", $content[1]);
$content[1] = str_replace("</td><td>", "|", $content[1]);[/code]
If I echo $content[1] I will get:
[b]Pet|Owner|Location
Cat|John|City
Dog|Amy|City
Dog|Wayne|Country
Cat|Sarah|City
Cat|Jack|Country[/b]
I obviously want to explode the data at each "|" but how do I loop through each line? I have been trying to figure out [b]foreach[/b] because that seems to be what I need, but I can't seem to figure it out. :(
Thanks in advance!
Joe -
Thanks a lot [b]SemiApocalyptic[/b] and [b]kenrbnsn[/b]!
You guys are the greatest!
I hope that I will be good enough to contribute as well as benifit.
Joe
I finished the code so that it does what I wanted it to do:
[code]<html>
<body>
<form method="post">
<textarea col="50" rows="10" name="str"></textarea>
<input type="submit" value="Submit">
</form>
<?
$str = str_replace(",", "", $str);
$str = str_replace(".", "", $str);
$str = str_replace(";", "", $str);
$str = str_replace(";", "", $str);
$str = str_replace("'", "", $str);
$str = str_replace('"', '', $str);
$str = str_replace("?", "", $str);
$str = str_replace("!", "", $str);
$array = explode(" ",$str);
$list = array_unique($array);
$print = implode(" ",$list);
echo "$print";
?>
</body>
</html>[/code] -
Thanks [b]SemiApocalyptic[/b].
I am trying to figure out implode() right now.
I should have been more precise in my OP. I want to take a block of text (entered via a form) and list each word that was entered, but with only one example of each word (no duplicates).
I thought that the code that I had did that (but in an Array) but I was wrong. It just listed each word in the text. :(
Anyone have any suggestions for me?
Thanks,
Joe
[!--quoteo(post=383758:date=Jun 14 2006, 09:27 PM:name=kenrbnsn)--][div class=\'quotetop\']QUOTE(kenrbnsn @ Jun 14 2006, 09:27 PM) [snapback]383758[/snapback][/div][div class=\'quotemain\'][!--quotec--]
Your function is doing way too much work. I took the liberty of rewriting it.
Ken
[/quote]
Thanks Ken!
You did quite a bit of good work.
Joe [img src=\"style_emoticons/[#EMO_DIR#]/smile.gif\" style=\"vertical-align:middle\" emoid=\":smile:\" border=\"0\" alt=\"smile.gif\" /] -
Hi.
I have a script that will list each word entered into a textbox. What I am having trouble figuring out is how to remove the array keys. What is the best way to do this? is there a way to convert an array into a string and simply echo it?
Thanks,
Joe
-
[!--quoteo(post=361828:date=Apr 5 2006, 11:54 AM:name=Jonquil)--][div class=\'quotetop\']QUOTE(Jonquil @ Apr 5 2006, 11:54 AM) [snapback]361828[/snapback][/div][div class=\'quotemain\'][!--quotec--]
I need help to get this program to connect to the database.
The database is set up but the script won't save data into the database.
Willing to pay reasonable amount for ongoing help.
Jonquil
[/quote]
If you want anyone to help you, you could [b]at least[/b] tell us what script you are using... -
[!--quoteo(post=381625:date=Jun 9 2006, 06:34 AM:name=me1000)--][div class=\'quotetop\']QUOTE(me1000 @ Jun 9 2006, 06:34 AM) [snapback]381625[/snapback][/div][div class=\'quotemain\'][!--quotec--]
ok i moved where it defines the function, IDK why it didnt work, i havent moved any of that particular code around at all!
andyway it works now.
thank you for all your help!!! :)
[/quote]
Hi,
I am working on something simular and I would like to have the image broken up into several small pieces (say 9 equal sized pieces, like a tic-tac-toe board) and renamed something like image1-1.jpg, image1-2.jpg, etc...
Is there a simple way of doing this, or do I need to use imagecopy 9 times?
Thanks!
Joe -
[!--quoteo(post=376628:date=May 24 2006, 07:13 PM:name=runnerjp)--][div class=\'quotetop\']QUOTE(runnerjp @ May 24 2006, 07:13 PM) [snapback]376628[/snapback][/div][div class=\'quotemain\'][!--quotec--]
yes it has php... right iget you upload the notpad file with code onto webhost i know how to do that but its part where you have to make it appear on you webpage... wat html do u put onto your webpage for this to appear :S[/quote]
Sounds like you're trying to do a swan dive before you learn how to swim.
Start with this page:
[a href=\"http://www.phpfreaks.com/phpmanual/page/introduction.html\" target=\"_blank\"]http://www.phpfreaks.com/phpmanual/page/introduction.html[/a]
and then browse the tutorial.
Also check out:
[a href=\"http://www.php.net\" target=\"_blank\"]http://www.php.net[/a]
They also have a tutorial and you can search for functions.
Since you're new, it might be easiest to just install a freeware script
You can find some at:
[a href=\"http://www.hotscripts.com\" target=\"_blank\"]http://www.hotscripts.com[/a] -
Is there a PHP function that will keep track of the bandwidth usage in a folder (including sub-folders, if possible)?
Thanks!
Joe
%^% -
[!--quoteo(post=375531:date=May 21 2006, 12:37 AM:name=yonta)--][div class=\'quotetop\']QUOTE(yonta @ May 21 2006, 12:37 AM) [snapback]375531[/snapback][/div][div class=\'quotemain\'][!--quotec--]
You could use the [a href=\"http://pt.php.net/manual/en/function.pathinfo.php\" target=\"_blank\"]pathinfo [/a]function.[/quote]
Thanks!
Now for what is probably a stupid question...
The opendir() function will only open a folder on my website, right? If I have two websites (hosted on different servers) can I list the contents in a folder located on one website on a page located on the other?
Joe -
I modified the code provided by [b]shocker-z[/b] in [a href=\"http://www.phpfreaks.com/forums/index.php?showtopic=89695&st=0&p=375454entry375454\" target=\"_blank\"]directory listing problem[/a] for listing the contents of a directory so that it wouldn't show the file extensions.
[code]$path = "./";
$dir = opendir($path);
echo "<ul>\n";
while($file = readdir($dir))
{
if ($file != "." && $file != ".." && $file != ".php")
{
list ($name, $ext) = explode (".", $file);
echo "<li><a href = \"" .$path.$file. "\" target = \" _blank\"> ".$name." </a></li> \n";
}
}
echo "</ul>\n";
closedir($dir);[/code]
Anyone know how to make it show only folders, or to specific file types?
Joe -
[!--quoteo(post=360205:date=Mar 31 2006, 07:54 AM:name=sheepz)--][div class=\'quotetop\']QUOTE(sheepz @ Mar 31 2006, 07:54 AM) [snapback]360205[/snapback][/div][div class=\'quotemain\'][!--quotec--]
you are awsome, it works exactly the way i want it to! thx a bunch!
[/quote]
Is there a way to remove the file extension when it's displayed?
Maybe using [!--coloro:#3333FF--][span style=\"color:#3333FF\"][!--/coloro--]basename()[!--colorc--][/span][!--/colorc--]?
Thanks!
Joe
-
Now that's what I call a "snippit"!
[!--quoteo(post=375310:date=May 20 2006, 03:09 AM:name=Barand)--][div class=\'quotetop\']QUOTE(Barand @ May 20 2006, 03:09 AM) [snapback]375310[/snapback][/div][div class=\'quotemain\'][!--quotec--]
OK, minimalist version
[code]$lines = file('myfile.txt');
vprintf ('%s live in the %s and eat %s', explode(';', trim($lines[array_rand($lines)])));[/code]
[/quote]
-
[!--quoteo(post=374174:date=May 16 2006, 09:34 AM:name=willdikuloz)--][div class=\'quotetop\']QUOTE(willdikuloz @ May 16 2006, 09:34 AM) [snapback]374174[/snapback][/div][div class=\'quotemain\'][!--quotec--]
Is there a script/snippet to show X amount of banners in random order?
[/quote]
I found this at [a href=\"http://www.hawkee.com\" target=\"_blank\"]http://www.hawkee.com[/a]:
[code]<?
$random_text = array("Random Text 1",
"Random Text 2",
"Random Text 3",
"Random Text 4",
"Random Text 5");
srand(time());
$sizeof = count($random_text);
$random = (rand()%$sizeof);
print("$random_text[$random]");
?>[/code] -
Wow!
Thank you very much for the incredably fast and detailed answer!
I figured out how to make it random too by making the following changes:
[blockquote]$lines = file('myfile.txt');
[!--coloro:#FF0000--][span style=\"color:#FF0000\"][!--/coloro--]$count = count($lines)[!--colorc--][/span][!--/colorc--];
$wanted = [!--coloro:#FF0000--][span style=\"color:#FF0000\"][!--/coloro--](rand()%$count)[!--colorc--][/span][!--/colorc--];
$line = trim($lines[$wanted]);
list ($species, $habitat, $food) = explode (";", $line);
echo "$species lives in the $habitat and eats $food";[/blockquote]
Thanks again for your help!
Joe
-
Hi,
I want to write a script that will pull a random line of text from an external file (such as a txt file) and then break it into peices and insert it into the webpage.
For example:
If the file contained this;
fish;water;plankton
scorpians;desert;insects
eagles;trees;fish
Then it would grab one line at random (say, line #2) and assign the sections to $species, $habitat, and $food respectivly so that I could end up with an output like this:
[b]scorpians[/b] live in the [b]desert[/b] and eat [b]insects[/b].
I don't expect anyone to write this for me (I can wish, but I don't expect), but if you could tell me what commands I need to learn and/or where to learn about them, that would help tremendously!
Thanks!
Joe
PHP forum?
in PHP Coding Help
Posted
[url=http://tfbb.jcink.com]http://tfbb.jcink.com[/url]