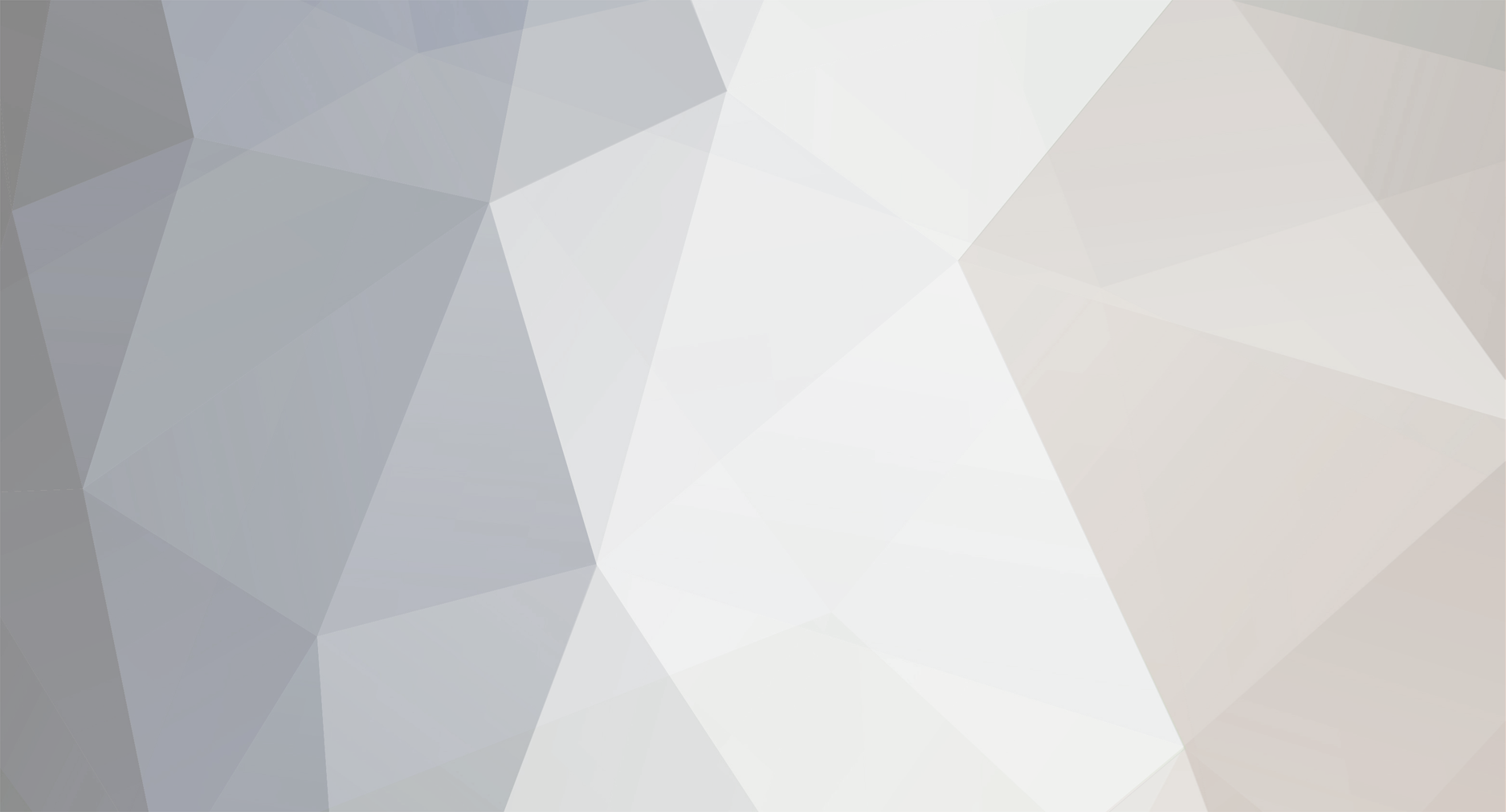
php_joe
-
Posts
175 -
Joined
-
Last visited
Never
Posts posted by php_joe
-
-
Id'e be carefull though... the example I gave would simply run until your server does. It would create an infinant recursion.
There's a way to prevent that though, right? so that the code stops parsing when the page is closed?
-
Seems an odd request given your example, but recursion may be what your after.
Yeah,
you can call a function inside of itself? I hadn't even thought to try that!
Thanks!
-
is there a command that will abort a function and rewind it back to the beginning?
Something along the lines of this:
<? if($handle = fopen($url)){ fwrite($handle, $info); fclose($handle); }else{ // here is where I want it to return to the beginning of the function } ?>
-
-
the conditions are all in the (). if you require a condition then it looks like this: if($var){blah, blah, blah}. if you require a condition but want to avoid it when another condition applies use if($var1 && !$var2){blah, blah, blah}
-
or I think you could use shuffle($creepinfo); and then use $creepinfo[0] for the enemy's information.
-
"Visitors" use PHP via the webserver to access your pages; they don't do it directly. So whichever user the webserver runs under is who is accessing your filesystem and permissions+ownership will apply to that user.
I've seen websites that have a folder full of images. If you try to view the image directly then it redirects you or says "forbidden" but it still shows up on the webpages.
I tried changing the folder's permissions, but whenever I deny access to the folder all I get is a red X where the image on the webpage should be.
I know that I can put all the remote files in a secret folder. I'm just worried about some hacker guessing the member account's folder's name, or using a search program to find it.
-
it doesn't matter if they're on one page or not. if you have a lot then it might reduce your bandwidth.
-
Tell if this right
you give an example that when i put a value ( any value ) to the $var, thats mean isset() will see that there is a vaule and display echo msg ?
Yes
-
Are these files ever executed? Is the path accessible to the user, i.e., they can type it into the address bar?
Some are, some are not. What I'm mainly worried about is someone injecting a file into a folder and using it to create new files or modify & damage existing files.
chmod dir to 555 or 755?
It might help also to restrict your world writeable files to a specific directory.
I'll try 555, thanks!
Do you know how to resitrict access to the folder so that files on my site can access it but visitors can not access it directly?
-
I have a webpage that some hacker managed to load a file onto.
My website's help support suggested that I should not use chmod 0777 since it is not secure.
However, I need to allow my site's visitors to open files & write information to them.
My question:
Is there a setting that will allow site members to create, open, write to, and delete files using my pages but will not allow them to create their own pages outside of my control?
-
you could also make 2 arrays and then use array_merge.
-
1. make a PHP page like this:
<? $info = "$text\n"; $handle = fopen("./record.txt", "a+"); fwrite($handle, $info); fclose($handle); ?>
I'm calling it record_text.php.
Now you can use javascript's onsubmit function to refresh a frame or iframe every time a user submits a text in the chat room.
-
it looks like it's hotmail's spam filter.
Try sending it using a From address that's in your address book.
-
Hey guys, I'm a beginner and trying to figure out some things in php.
Let's say I have a script count.php and i want it to add all the numbers generated from multiple remote files (number.php)
so count.php is at domain1.com
The number.php would be at domain2.com
and i want count.php to get the number generated from number.php into a variable inside count.php
How would I code count.php to fetch the number from the remote file (number.php)?
Sorry if my word is a little confusing. but i will really appreciate anyone who try to help
merci d'avance.
So, assuming that domain1.com requres a variable input and will display just the number (without any other stuff) then you could do this:
<? $number = file_get_contents("http://www.domain1.com?some_variable=value"); if(!is_numeric($number)) unset($number); ?>
-
Like this:
<?php if (isset($var)) { echo "This var is set so I will print."; } ?>
run the document like this: test.php?var=1 and test.php?var= and you can see the difference.
-
<? $score = '43012'; $number = preg_split('//', $score, -1, PREG_SPLIT_NO_EMPTY); $count = count($number); // <<<< if you need to know how many char in the number (no purpose here) foreach($number as $value){ echo "<img src=\"./{$value}.jpg\">"; } ?>
-
have you tried saving the document as UTF-8 or big unidian?
-
Try this:
<? $lines = file("./link_list.txt"); shuffle($lines); $i = '0'; while($i < 10){ echo "<a href=\"$lines[$i]\">$lines[$i]</a>"; $i++; } ?>
-
why can't you just use str_replace()?
-
Something like this?
<? $table1 = explode(",", "./table1.txt"); $table2 = explode(",", "./table2.txt"); echo "<tr><td>{$table1[0]} = {$table2[0]}</td></tr>"; ?>
-
I am trying to write a function that will strip tags and reduce multiple line breaks & tabs to just one. There's probably a better way of doing this, but this is what I've come up with on my own.
This strips the tags, breaks each line into an array, separates by tabs, then removes white space.
function strip_whitespace($code){ $code = strip_tags($code); $lines = explode("\n", $code); foreach($lines as $lkey => $line){ $pieces = explode("\t", $line); foreach($pieces as $pkey => $piece){ $newpiece[$pkey] = trim($piece); } // end pieces $newline[$lkey] = implode("\t", $newpiece); $newline[$lkey] = trim($newline[$lkey]); } // end lines $output = implode("\n", $newline); return($output); }
However, I still end up with double tabs & double line breaks, so I tried this:
function strip_whitespace($code){ $code = strip_tags($code); $lines = explode("\n", $code); foreach($lines as $lkey => $line){ $pieces = explode("\t", $line); foreach($pieces as $pkey => $piece){ $newpiece[$pkey] = trim($piece); if(!$newpiece[$pkey]) unset($newpiece[$pkey]); // This reverses the array } // end pieces $newline[$lkey] = implode("\t", $newpiece); $newline[$lkey] = trim($newline[$lkey]); if(!$newline[$lkey]) unset($newline[$lkey]); // but this one works fine } // end lines $output = implode("\n", $newline); return($output); }
Why does the $newpiece array order reverse when I unset() the empty values?
-
Nevermind, I got it.
The correct method is:
<? $b = 12 * sin(deg2rad(65)) / sin(deg2rad(50)); echo "$b"; ?>
-
Hi, I'm having some problems with a math function not giving me the answer that I expect.
Wikipedia says that you can find a side of a triangle if you know an angle and 2 sides using the law of cosine:
http://en.wikipedia.org/wiki/Law_of_cosines
so I used this code:
<? $a = '5'; $b = '5'; $c = sqrt(pow($a, 2) + pow($b, 2) - (2 * $a * $b * cos(135))); echo "$c"; ?>
And it gave me the correct (I think) answer: 9.9902148003463
But when I try to find a side using 2 angles and a side using the law of sine it doesn't work.
The equation that I have is "b = (12 Sin 65)/(sin 50)" from http://home.alltel.net/okrebs/page93.html so I used this code:
<? $b = 12 * sin(65) / sin(50); echo "$b"; ?>
Which, according the the website that I got it from, should have printed "14.2", but I got "-37.815911143213" instead.
What am I doing wrong?
can you make a function repeat?
in PHP Coding Help
Posted
Nothing specific at the moment, but I've occationally run into a situation that I'd want to repeat, maybe with a slightly altered set of circumstances.
If you had a situation where the function was in an endless loop (like, you'd set the timeout at 0) would it continue to loop if the visitor closed the webpage?