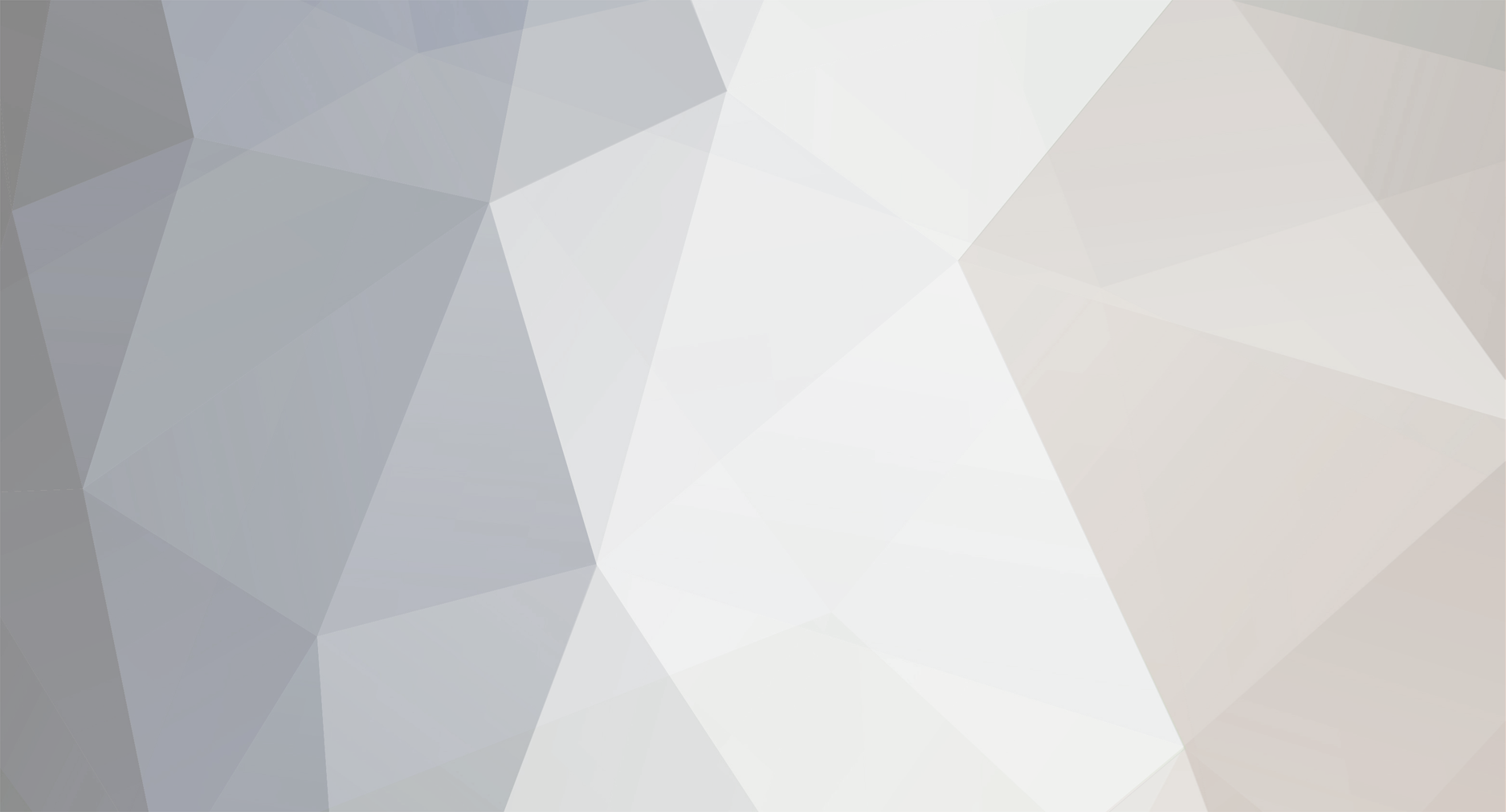
php_joe
-
Posts
175 -
Joined
-
Last visited
Never
Posts posted by php_joe
-
-
sorry, I forgot to put that in.
$cookies2 = $_COOKIE['password']; $cookies = $_COOKIE['id'];
I made the user ID lowercase to avoid problems in the future with people registering under the same name using upper & lower case letter. it doesn't really matter to the problem at hand.
-
Ok, I'll try that.
But why does it work when I use the link then?
-
Hi,
I'm having a problem with my cookies.
Here are my login & logout functions:
function login($id, $password){ setcookie("password", "$password"); setcookie("id", "$id"); header("Location: ?"); } function logout($id, $password){ setcookie("password", "$password", time() - 1); setcookie("id", "$id", time() - 1); header("Location: ?"); }
Then the script that calls the functions:
if($action == "login"){ $lowercase_id = strtolower($login_id); login($lowercase_id, $login_password); } if($action == "logout"){ logout($id, $password); }
Then I have a code that checks isset() and displays the appropriate info:
if(isset($cookies)){ echo "<div>You are logged in.<br /><a href=\"?action=logout\">Log Out</a></div>"; }else{ echo "You are logged out.<br /><a href=\"?action=login\">Log In</a>"; //require "./not_logged_in.php"; }
The problem is: if I use this link:
echo "You are logged out.<br /><a href=\"?action=login\">Log In</a>";
it works fine, but if I require the logged out page:
require "./not_logged_in.php";
it will run the login() function, but the isset() will fail.
not_logged_in.php looks like this:
<form method="post"> <input type="hidden" name="action" value="login" /> Name:<input type="text" name="login_name" /> Password:<input type="password" name="login_password" /> <input type="submit" name="submit" value="Log In" /> </form>
if the code runs the login() function and isset() works when I use the link, why does it run the login() function and isset() fails when I use the form to post the info to the page?
I'm not even checking the information posted against anything yet, shouldn't the fact that $action == "login" be enough... ???
-
It's because you have if(!isset inside the if(isset brackets.
Try this:
if(isset($sub)){ echo "Complete"; }else{ echo "Failed"; }
-
If you're storing your info as lines in a text file instead of a mysql database, you could do what you said and add a field to the front of each line, getting the info for field with time().
Then, before you write to the file, use sort() or arsort() to sort the info.
Joe
-
The function worked great, thank you very much!
I was able to cut out the second function by adding
to the first one. I also added >>> <s> to the array.
-
Heres a code snippet for bbcode parser with regex to get you started quickly - CLICKY
Thank you very much for this function. I hope I can get it to work!
-
I've been messing with this all day. I think that there must be some kind of conflict with the rest of the code, because it's just not behaving right.
-
Thanks guys, using multiple variable names works.
I have not tried the preg_replace or array yet, but I will.
Joe
-
Actually, I'm trying to change [b] into <b>
But, for some reason, when I tried
$clean_message = str_replace('[b]', '<b>', $message);
by itself it works, but when I put
$clean_message = str_replace('[b]', '<b>', $message); $clean_message = str_replace('[/b]', '</b>', $message);
In, only the second one works.
-
I'm editing a bbs program I have and I'm having problems changing the BBCode into HTML.
I tried:
$message = str_replace('[b]', '<b>', $message);
which didn't work.
I tried:
$message = str_replace('[', '<', $message);
which also didn't work.
Why can't I change '['?
-
OK, I figured it out:
<? if(!$sort) $sort = '0'; $line = file("./test.txt"); foreach($line as $key => $row){ $col = explode("|", $row); $array2 = "$col[$sort]"; $result = array_merge($array2, $col); $new_row[$key] = implode("|", $result); } if(is_numeric($array2)){ sort($new_row, SORT_NUMERIC); }else{ sort($new_row); } foreach($new_row as $key => $value){ echo "<div>$value</div>"; } ?>
Still.... if anyone knows a better way to sort a table from a text file, let me know
-
There's no query, what you see is what I have: a text file and a php file.
The table on the text file is row separated by \n and column separated by |
-
Hi, I'm trying to write a snippit that will sort rows in a table based on a column's value.
My test table is this:
0|1|2|3|4 1|2|3|4|0 2|3|4|0|1 3|4|0|1|2 4|0|1|2|3
And the code I have so far is:
<? if(!$sort) $sort = '0'; $line = file("./test.txt"); foreach($line as $key => $row){ $col = explode("|", $row); $array2 = "$col[$sort]"; $result = array_merge($array2, $col); $new_row[$key] = implode("|", $result); } sort($new_row); foreach($new_row as $key => $value){ echo "<div>$value</div>"; } ?>
Which works... kind of.
The problem is that the sort() function is sorting the output as text, not numbers, so that when the values go over 9 (i.e. 10) then instead of being sorted as 1, 2, 3, 10, 11 it sorts it as 1, 10, 11, 2, 3.
What can I change to make it sort the values as a number?
Or is there a better way to sort a table using a column's value (other than column 0)?
-
Thank you very much!
-
If the words are text superimposed over an image you could just use an html tag, like <center>, to center it.
Otherwise, I think you'll have to take the width of the text area, divide it in half, and subtract half of the width of the text to find the best starting point.
Or, you could just align the text left.
-
The PHP manual says:
Request variables: $_REQUESTAn associative array consisting of the contents of $_GET, $_POST, and $_COOKIE.
This is a 'superglobal', or automatic global, variable. This simply means that it is available in all scopes throughout a script. You don't need to do a global $_REQUEST; to access it within functions or methods.
If the register_globals directive is set, then these variables will also be made available in the global scope of the script; i.e., separate from the $_REQUEST array. For related information, see the security chapter titled Using Register Globals. These individual globals are not superglobals.
Which is interesting, but not very helpful for me. I could really use a simple example please.
Thank you.
-
You could still use sed.
<?php exec("sed -i -e '" . $linenumber . "d' $textdocument"); ?>
And I'm sticking to it.
Yes, that's what I was looking for.
Thank you very much!
-
Correct me if I'm wrong, but that searches for a line based on the text in the line, right?
I want to single out a line by the line number and replace the text with the new text (the line # is the info I have, not the existing text).
This is what I've trimmed it down to so far:
<? $line = file($textdocument); $line[$line_number] = "$new_text\n"; $newcontent = implode("", $line); $handle = fopen($worldlist, "w"); fwrite($handle, $newcontent); fclose($handle); ?>
And it seems to work now.
-
you can open a csv file like this:
<? $file = "./database.csv"; $row = file($file); // get the rows foreach($rows as $key => $row_content){ // loop through the rows $cell = explode(',', $value); // break the row up into cells // place code for matching data to a particular cell, such as eregi() } ?>
-
I'm using a Linux system, but I don't know 'sed'.
I just want to be able to modify a line on a text document without changing. nothing really fancy.
Something like this:
Cat Dog Bunny
and change it to:
Cat Horse Bunny
-
can you save the database as a csv (coma separated value) file?
You can still open, modify, and save it using Excel, but this way php can read it as a text file.
-
I want to write a snipit that will modify one line in a text file without changing any of the other lines.
I was thinking something like this:
<? $newline = "new junk"; $linenumber = "3"; $file = "./textdocument.txt"; $line = file($file); foreach($line as $key => $value){ if($key == $linenumber) $value = $newline; } $newcontent = implode("\n", $line); $handle = fopen($file, "w"); fwrite($handle, $newcontent); fclose($handle); ?>
Have I made a hash of this?
Is there a better way of doing it?
Maybe without exploding, looping, imploding, and rewriting all the lines?
Thanks in advance!
Joe
-
you could use preg_replace() or str_replace() to remove characters that you don't want.
Cookies set with a link, but not with a form
in PHP Coding Help
Posted
well, I don't know what's up, but suddenly it's working fine. I didn't change anything... ??? weird!