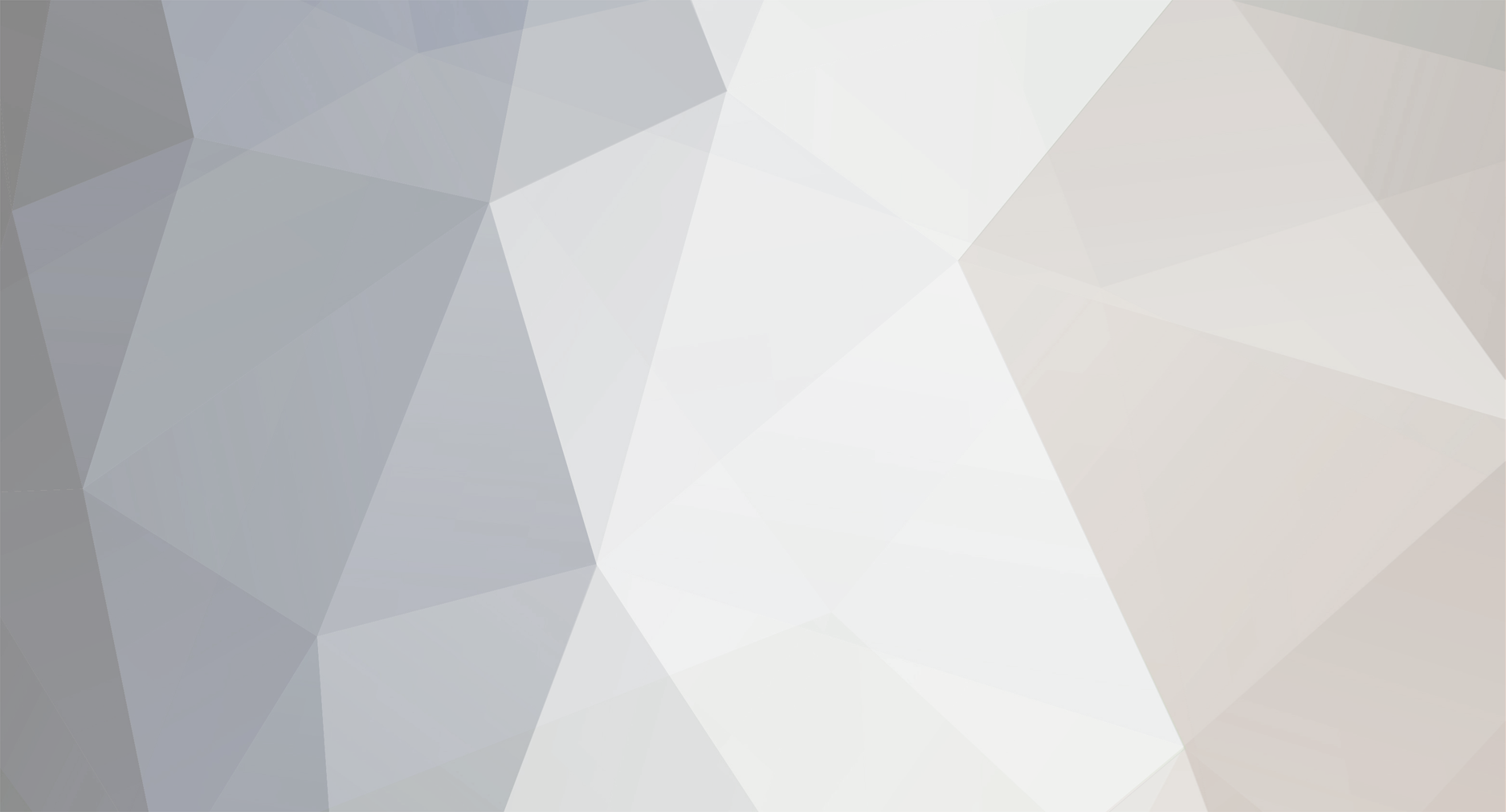
akitchin
Staff Alumni-
Posts
2,515 -
Joined
-
Last visited
Never
Everything posted by akitchin
-
i see a few issues. first, you never close the parenthesis on that first subquery. second, you have a several JOIN conditions that simply end with a table and no field: ON (t1.`employee_name` = lp0.) LEFT OUTER JOIN `employees` AS lp1 ON (t1.`gevity_number` = lp1.) lp0.what? lp1.what?
-
you tell us... what are you seeing in your browser's location bar when you are redirected? how are you trying to access username on index.php? we need more than two lines to diagnose your problem.
-
[SOLVED] Proper syntax to dynamically generate lines of code
akitchin replied to imimin's topic in PHP Coding Help
first, move these lines to the top: /* Enable displaying of errors*/ error_reporting(E_ALL); ini_set('display_errors', 'On'); second, it could be that your script is terminating because of a lack of GET['cat'] and your output is being hidden somehow. -
[SOLVED] IF/While loop and 2 Sql INSERT statements
akitchin replied to CodeMama's topic in PHP Coding Help
if you've just inserted the name and address, doesn't it stand to reason that the name and address now exist in the database? or are you just collecting all the information in one form and are wondering how to check if the name and address from that form already exist? if the latter, you may want to reconsider your design - someone might, for example, put in "Faye's Restaurant" and an inspection for her restaurant. someone else may then come in and enter an inspection for "Faye's restaurant," causing a duplicate entry because the string doesn't match exactly. it gets even more muddled with address, since everyone has a different way of typing the same address. -
[SOLVED] Proper syntax to dynamically generate lines of code
akitchin replied to imimin's topic in PHP Coding Help
i suspect with all the different versions of this snippet that have floated around, you're still making either the $cat=all or the string concatenation error. what snippet are you currently using and getting a blank page for? -
[SOLVED] Proper syntax to dynamically generate lines of code
akitchin replied to imimin's topic in PHP Coding Help
the issue actually originally was that the line that is left uncommented is a syntax error ($cat=all) - i should have picked that out the first time. comment that line out and uncomment the line above it: <?php if(!isset($_GET['cat'])){ die("Category isn't specified"); } /* Enable displaying of errors */ error_reporting(E_ALL); ini_set('display_errors', 'On'); $cat = mysql_real_escape_string($_GET['cat']); //$cat=all <-- THIS IS A SYNTAX ERROR $get_items = "SELECT * FROM poj_products WHERE cat='$cat'"; $get_items = mysql_query($get_items); if($get_items){ $item_row = mysql_fetch_assoc($get_items); echo 'fadeimages[0]=["http://www.patternsofjoy.com/images/thumbs/'.$item_row['img'].', "", ""]'; }else{ die("Unable to connect to database.".mysql_error()); } ?> you can get rid of extract() altogether and it will work just fine (i've done that in this snippet). -
the reason is that any two cities with the same distance will overwrite each other. instead we can use the city as the index and overwrite it, which actually saves you the trouble of using array_unique: $sqlqueryaccountra = "select * from zipcodes where st = '$thestate' order by city"; $resultaccountra = mysql_query($sqlqueryaccountra); $cities = array(); while ($row = @mysql_fetch_array ($resultaccountra)) { $theirlat = $row['lat']; $theirlon = $row['lon']; $cityname = $row['city']; $theuserarea = distance($mylat, $mylon, $theirlat, $theirlon, "m"); if ($theuserarea < $myradius) { if (isset($cities["$cityname"]) && $theuserarea < $cities["$cityname"]) { $cities["$cityname"] = $theuserarea; } else { $cities += array($cityname => $theuserarea); } } } if (!empty($cities)) { foreach($cities AS $city => $theuserarea) { echo $city.' - '.$theuserarea.'<br />'; } } else { echo 'No cities found! You are all alone!'; } this will overwrite the distance stored if it is less than one that was previously stored for the same city, and will add it to the list of cities if it hasn't previously been added. however, i would also give kickstart's suggestion a try and see how that works out.
-
first - please use code tags in your posts. it makes things much easier to read through. second, the issue is with your variable spelling. you're assigning and using "usearea" in the calculation and the if() statement below it, then any reference i'm using is "userarea." reconcile the two and take another stab.
-
[SOLVED] Proper syntax to dynamically generate lines of code
akitchin replied to imimin's topic in PHP Coding Help
if you're going to concatenate a variable into the string, you shouldn't be using single quotes around it. this: echo 'fadeimages[0]=["http://www.patternsofjoy.com/images/thumbs/'.'$item_row['img']"'.', "", ""]'; should be: echo 'fadeimages[0]=["http://www.patternsofjoy.com/images/thumbs/'.$item_row['img'].'", "", ""]'; notice that all it's doing is breaking out of the string, appending the variable, and returning to finish the string. PS: why are you using extract() if you're just going to use the array anyway? -
you'll need to brush up on some manual literature: mysql_query mysql_query() returns a resource identifier, which in essence is just a handle or pointer to the actual resultset. in order to retrieve that resultset, you'll need to run one of the fetching functions on it. take your pick: mysql_fetch_array mysql_result mysql_fetch_assoc mysql_fetch_object
-
to do what you're wanting it to do, you'll want to plug the results into an array rather than echoing them in the while() loop, and then running array_unique against the results: $sqlqueryaccountra = "select * from zipcodes where st = '$thestate' order by city"; $resultaccountra = mysql_query($sqlqueryaccountra); $cities = array(); while ($row = @mysql_fetch_array ($resultaccountra)) { $theirlat = $row['lat']; $theirlon = $row['lon']; $cityname = $row['city']; $theusearea = distance($mylat, $mylon, $theirlat, $theirlon, "m"); if ($theusearea < $myradius) { $cities += array($theuserarea => $cityname); } } $unique_cities = array_unique($cities); if (empty($unique_cities)) { foreach($unique_cities AS $distance => $city) { echo $city.' - '.$distance.'<br />'; } } else { echo 'No cities found! You are all alone!'; }
-
have you tried style="width: 100%;"? not sure if this would work, but it's worth a shot.
-
merging a couple of gigs of avi's, huh? PORN MASHUPS FTW.
-
this isn't possible, because submitting the form sends a new request to the server and therefore forces a refresh. what exactly are you trying to achieve?
-
that's simply a matter of assigning the array_pop() expression to a variable instead of echoing it: <?php $numberset = range(1,99); shuffle($numberset); $numbers = array(); for ($i = 1; $i <= 5; $i++) { $numbers[] = array_pop($numberset); } echo '<pre>'.print_r($numbers, TRUE).'</pre>'; ?>
-
that's a very intensive way of doing it when you can use MySQL's built-in functions. try something like this: SELECT COUNT(*) AS total, payment AS payment_amount FROM table GROUP BY payment ORDER BY payment the total amount of money paid for each amount is simply the total multiplied by the payment amount. you could even put it into the query: SELECT COUNT(*) AS total_times, SUM(payment) AS total_paid, payment AS payment_amount FROM table GROUP BY payment ORDER BY payment
-
that sucks. by christmas that thing will be about as outdated as a flock of seagulls haircut.
-
if you're wanting to run a batch update on all of the current bets, you would be able to do this by selecting each BettingOfferID from the betting offers table, and run an UPDATE within each loop. if you're going to do this, you might as well grab the result_left and result_right values at the same time and avoid the subqueries within that massive UPDATE, and perform the calculation in PHP: $get_all_bets = 'SELECT BettingOfferID, result_left, result_right FROM Betting_Offer'; $resource = mysql_query($get_all_bets) or trigger_error('MySQL is complaining: '.mysql_error(), E_WARNING); while ($row = mysql_fetch_assoc($resource)) { $discriminant = $row['result_left'] - $row['result_right']; $update_these_bets = "UPDATE Placed_Bets SET profit = CASE WHEN $discriminant > 0 AND (Selected_Tip = '1' OR Selected_Tip = '1X') THEN Odds_of_Selected_Tip * Stake - Stake WHEN $discriminant = 0 AND (Selected_Tip = 'X' OR Selected_Tip = '1X' OR Selected_Tip = 'X2') THEN Odds_of_Selected_Tip * Stake - Stake WHEN $discriminant < 0 AND (Selected_Tip = '2' OR Selected_Tip = 'X2') THEN Odds_of_Selected_Tip * Stake - Stake ELSE -1 * Stake END WHERE BettingOfferID = '{$row['BettingOfferID']}'"; $result = mysql_query($update_these_bets); echo ($result == FALSE) ? 'Bet number '.$row['BettingOfferID'].' not updated properly.' : 'Bet number '.$row['BettingOfferID'].' updated successfully.'; } this will probably take a bit of time, but since it's a batch run, it will take care of all bets currently in your system. if you intend to run this query every single time you input the results for a certain match, you're better off limiting yourself to the UPDATE query previously offered using only the BettingOfferID for that match. otherwise if you just plan to batch UPDATE every now and again, i would add a flag field into the Betting_Offers table that tells you whether the calculation has taken place for that bet already; this will avoid re-calculating the profit for bets that have already undergone the update and will avoid wasting resources.
-
you can use "AND" and "OR" to separate them, but you must include the field in every clause: WHERE this_variable = '$this_variable' OR that_variable = '$that_variable'
-
you can add as many variables as you want to that UPDATE query by adding the other fields separated by commas. is there something in particular you're trying to add to the query?
-
first off, you've got an error in your query. you need to add an operator to that WHERE clause: SELECT `Answer` FROM `questions` WHERE `Qid` = '$qid') i also removed some unnecessary stuff (didn't need to select Qid, and you don't need the table notation when selecting from only one table). second, you're on the right track for checking the answer against the one submitted. within that if() statement (which batosi has corrected for you), you will simply need to check if the two match: if (num_rows thing) { $row = mysql_fetch_assoc($offset_result); if ($_POST['answer'] == $row['Answer']) { // answer is correct } else { // answer is incorrect } } keep in mind you're at the mercy of the machine here - if they aren't identical in spelling, case, and whitespace, the computer won't technically consider them matched.
-
to be honest, i haven't used CASE statements in an UPDATE query before. it could be that you need to enclose the entire statement in parentheses?
-
are you aware there's an entire internet of information out there? google is popular for a reason, you know.