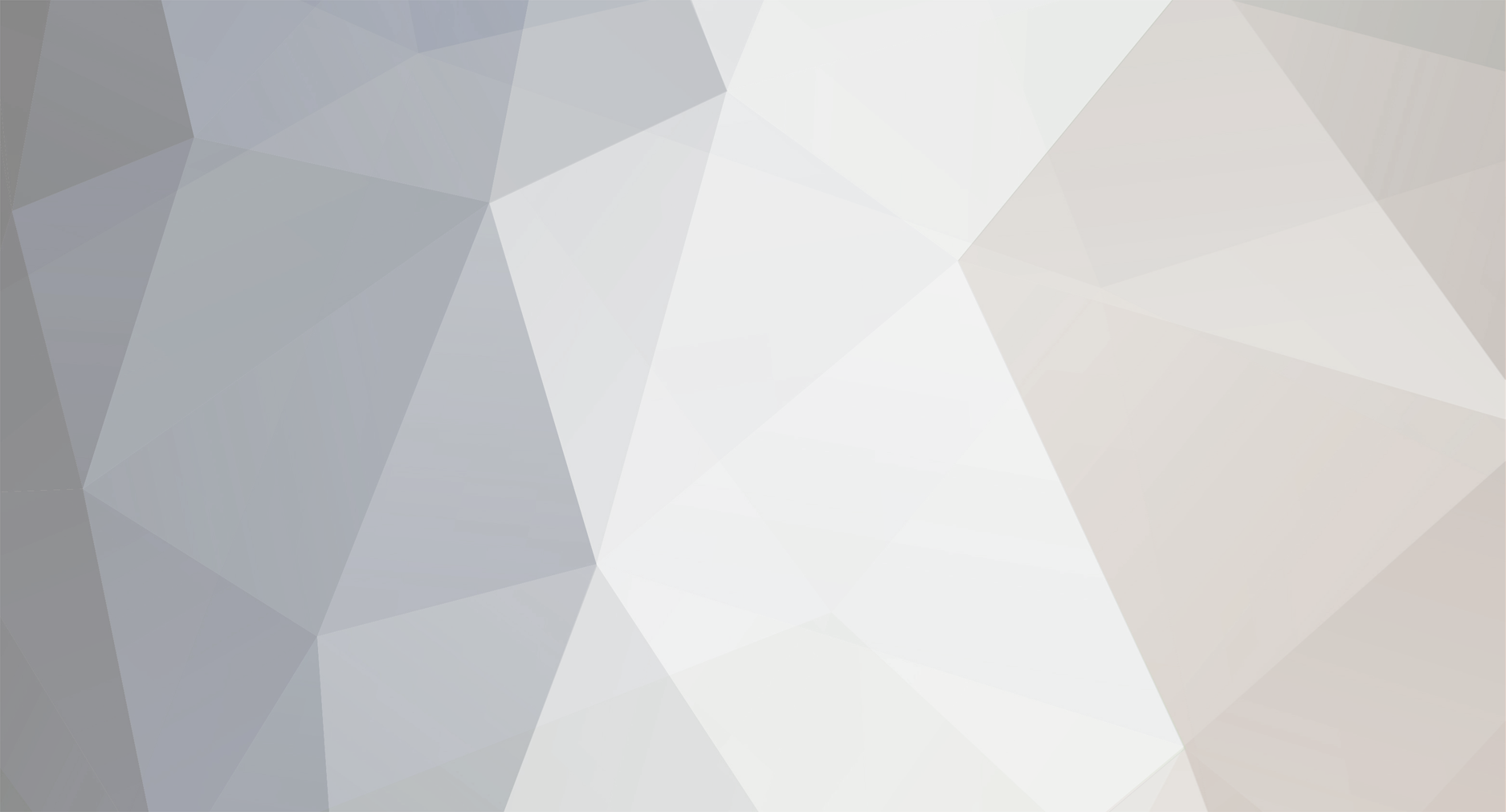
akitchin
Staff Alumni-
Posts
2,515 -
Joined
-
Last visited
Never
Everything posted by akitchin
-
have you tried turning error reporting on to see what the problem is when you get a blank screen? try putting this at the top of the file: error_reporting(E_ALL); ini_set('display_errors', 'On'); what you should be looking for is a warning (not a notice). EDIT: there's a chance it could be your mysql_real_escape_string() that's causing the warning if you haven't initiated a connection to the database yet.
-
that's because you're just assigning the value of $value to the variable $key. if you want to use $key as the variable name, you need to use variable variable notation: $$key = $value; however, the extract() function will do this for you.
-
... why would you use MySQL for this instead of PHP? the data's already in PHP. my suggestion would be to select a UNIX_TIMESTAMP()d version of the dates to compare, and then use PHP's min function.
-
are you getting an error? if so, what is it? is that the exact string you're passing to MySQL? if so, you should remove the semicolon.
-
how about using array_diff? this will take a lot of the work out of it for you. there are a number of variations of it as well, depending on your key and value setups - head over to the php manual and see which one works best for you. EDIT: should point out array_diff_assoc is probably better-suited to the task here.
-
[SOLVED] Proper syntax to dynamically generate lines of code
akitchin replied to imimin's topic in PHP Coding Help
yea, but i don't think that's the root cause of his issues at the moment (although that will pre-emptively fix the issue when he does sort out the current one). good catch. -
alright, there are still some problems with your code: 1. you need session_start() at the top of EVERY PAGE. that INCLUDES checklogin.php and login.php. 2. if you're submitting the form and getting t_nick via $_POST, you don't need it via $_GET. remove that line from the top of checklogin.php. 3. session_is_registered() is deprecated, and you're not even passing it a string. you're trying to pass it a constant that doesn't exist. in index.php you can simply use: if (!isset($_SESSION['t_nick'])) 4. in index.php, 't_nick' will be in the $_SESSION variable, not $_GET. use: $t_nick = $_SESSION['t_nick']; i'll be honest and say i think you're out of your element here - you may want to follow along some basic tutorials regarding variable types, assignment, and handling.
-
my mistake, sorry!
-
returning value from php function, can't get it to work
akitchin replied to stevieontario's topic in PHP Coding Help
it is, but only partially, for your first issue (which was getsourceid() not working). see above for how to make it work. i would suggest adding a third parameter to the function that allows you to keep the connection alive after closing. since you usually won't care about getting the results from an INSERT query anyway, you could even just add a case to the $sets switch() that does this: function sqlquery($myquery,$sets=0) { global $db_hostname,$db_name,$db_username,$db_password; $db_connection = mysql_connect($db_hostname, $db_username, $db_password) or die("Could not connect: " . mysql_error()); mysql_select_db($db_name, $db_connection); $results = mysql_query($myquery, $db_connection) or die(mysql_error()); switch ($sets) { case 1: $sqlres = mysql_num_rows($results); break; case 2: $sqlres = mysql_fetch_array($results); break; case 3: $sqlres = $db_connection; break; default: $sqlres = $results; break; } if ($sets != 3) mysql_close($db_connection); return $sqlres; } whenever you send a statement to sqlquery() that you don't want closing the connection afterward, send it with 3 as the second parameter: $query = 'INSERT INTO info stuff'; $conn = sqlquery($query); $lastid = mysql_insert_id($conn); this is a bit of band-aid solution, as it's been spliced into your function instead of redesigning the function itself, but it should work. did you write sqlquery() yourself? -
... did you not just paste back to him EXACTLY what he put in his first post?
-
returning value from php function, can't get it to work
akitchin replied to stevieontario's topic in PHP Coding Help
ah, then that's your issue. i suspect you're running the INSERT using sqlquery(), which closes the database connection after the query. when mysql_insert_id() is called, it tries to use the last opened connection (if is still open), or it tries to establish a new connection as if mysql_connect() were called without any parameters. it won't find any currently open ones, so it tries to establish a connection and, as the error says, fails to do so. since the getsourceid() is a workaround in the first place, it might be worth re-thinking your strategy regarding queries. perhaps add a parameter to sqlquery() that allows you to stipulate that the connection be left open after the query is run? -
check several variables at once...but only return one
akitchin replied to severndigital's topic in PHP Coding Help
this is a little tough to do without knowing more about the variables. are you able to put them into an array, rather than number them sequentially? if you can't do that, do you have some way of knowing how many variables there are? -
returning value from php function, can't get it to work
akitchin replied to stevieontario's topic in PHP Coding Help
is the ID in info an auto_increment INT primary key? -
this is called a JOIN, and is a very valuable construct to learn. here you'll want a LEFT OUTER JOIN to append the first name and last name to the right side of the table you're querying: SELECT tblpm.*, tblusers.First AS first_name, tblusers.Last AS last_name FROM tblpm LEFT OUTER JOIN tblusers ON tblpm.sender_id=tblusers.usrID WHERE receiver_id ='$usrID' ORDER BY id DESC i'll be honest and say i'm not sure that tblpm.* will work, but if it doesn't, you'll just have to list the fields explicitly. PS: google a few tutorials on JOINs and you'll most likely be able to come up with a more suitable query than this. wikipedia has a pretty decent entry on them.
-
okay, let's see the coding for checking the username, and then the code for index.php.
-
returning value from php function, can't get it to work
akitchin replied to stevieontario's topic in PHP Coding Help
ah, my mistake - sorry, should have read the first post more closely. you're not sending a second parameter to sqlquery(), which will default to $sets = 0, and therefore will just return the resource. this means getsourceid() will also only return the resource, not the actual id. therefore to get the last id using this current setup, you'd need to go: $resource = getsourceid(); // use the function kickstart provided $lastid = $resource['id']; however it's worth noting that mysql_insert_id() will automatically fetch the last ID that was inserted (when you INSERTed into INFO), so you can easily do this: // define and run the INSERT query for INFO $lastid = mysql_insert_id(); // use $lastid for the PERFORMANCE INSERTs saves you some server resources and a pointless function. -
[SOLVED] Proper syntax to dynamically generate lines of code
akitchin replied to imimin's topic in PHP Coding Help
mysql_real_escape_string() won't work if a connection to the database hasn't yet been established. -
you only need session_start(), you don't need: $_SESSION["t_nick"] = $t_nick; in fact, you don't WANT that on every page, since that will just overwrite the variable everytime.
-
then either your query is incorrect, or 'Title' isn't actually a column you're selecting in the query, because MySQL is finding rows somehow.
-
returning value from php function, can't get it to work
akitchin replied to stevieontario's topic in PHP Coding Help
i see no mention of $lastid in any of your code (nor do i see mention of the PERFORMANCE table). you may want to clarify the problem.. -
[SOLVED] good image size limit to upload?
akitchin replied to dadamssg's topic in Application Design
i personally like to use 1.76933MB myself. -
first, i see no mention of $_GET['username'], so i'm not sure how this section is related to your initial question. otherwise, the reason SESSION variables are not being passed is (i suspect) you are missing session_start(); at the very top of the script. that needs to be on EVERY page in order to pass session variables along.
-
if what? the reason it's giving you an error is because there's no condition to go with that if. if you mean you want to display "morning" when there are no matching rows, then you need to use else to compliment the first if(): if (mysql_num_rows($res1) > 0) { while($row = mysql_fetch_assoc($res1)) { echo "".$row['Title']."<br>"; } } else { echo 'morning'; }
-
here you'll actually want implode(). i should also point out that you don't need to set the $errorb flag - simply checking if the $error array exists is sufficient, since it won't be defined unless one of the fields is missing: if (empty($_POST['copyright'])) { $error[] = "copyright"; } if (isset($error) && !empty($error)) { $body .= "<h3 style='color: red;'>You must fill in the following fields: ".implode(', ', $error).".</h3>"; } else { //etc } ?>
-
[SOLVED] Proper syntax to dynamically generate lines of code
akitchin replied to imimin's topic in PHP Coding Help
and still got the same thing. Should I change GET['cat'] to something else? no, but i'd suggest changing the die() statement to echo, so you can see if it's there that the script is halting (or just comment that section out entirely). if you're accessing it directly, $_GET['cat'] won't be defined..