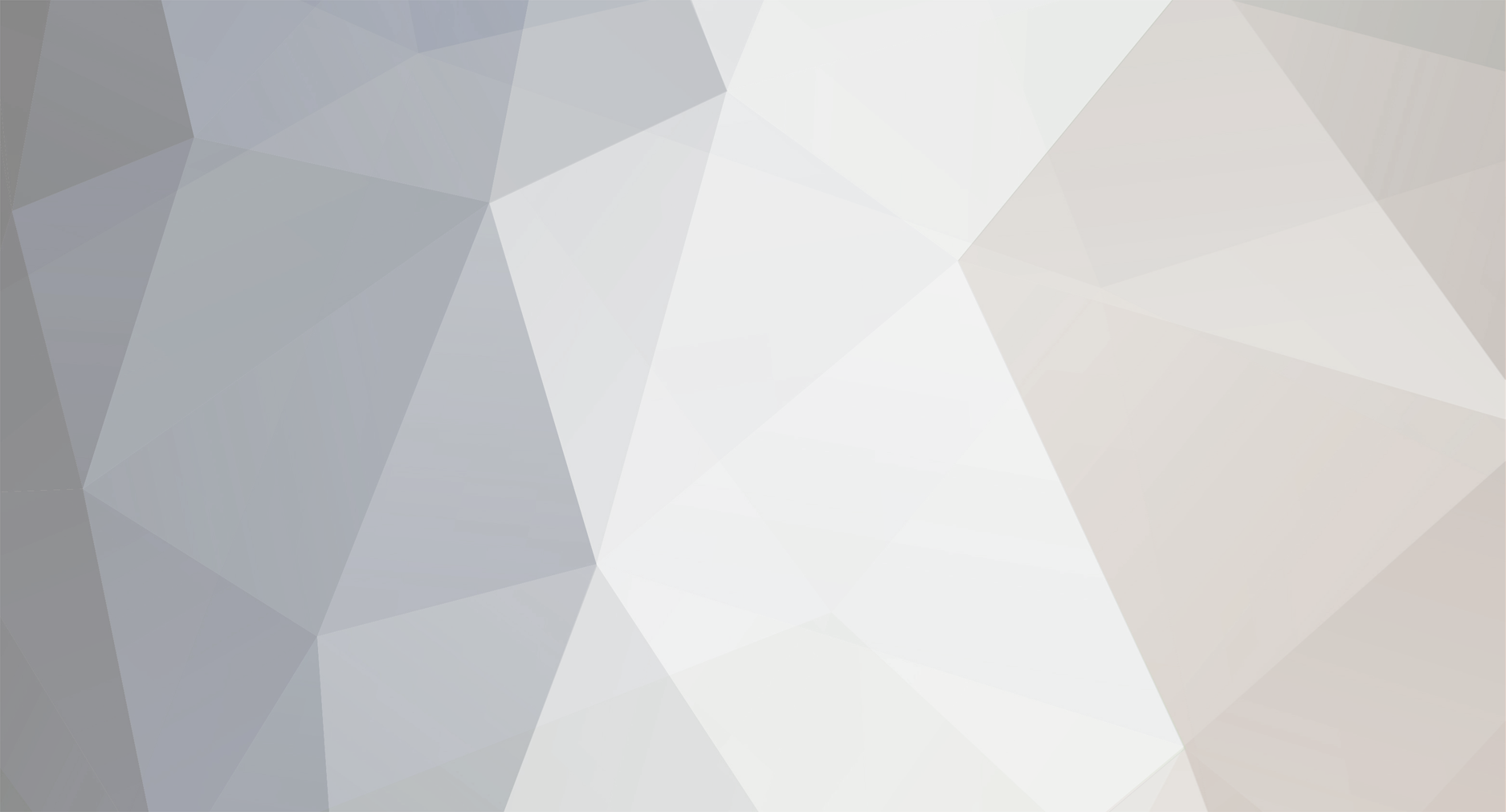
akitchin
Staff Alumni-
Posts
2,515 -
Joined
-
Last visited
Never
Everything posted by akitchin
-
note that when using str_pad(), you don't even need to check the string's length. no padding will be performed if the input string is equal to or greater than the pad length.
-
are you saying the face was accidentally photoshopped?
-
[SOLVED] Invalid argument supplied for foreach()
akitchin replied to sawade's topic in PHP Coding Help
this is a question concerning PEAR - i don't know specifically what format PEAR expects your headers to be in (beyond requiring that it's an array), but i would assume that they are expecting it in the format: 'header_name' => 'header_value' in that case, your headers should be: $headers['From'] = 'CONTACT_US'; // note that this is invalid - if you want to use the constant CONTACT_US, drop the quotes $headers['MIME-Version'] = '1.0'; $headers['Content-Type'] = 'multipart/mixed; boundary=\"{$mime_boundary}\"'; // note that $mime_boundary won't be replace here, as you're using single quotes to delimit the string do you have documentation on the format PEAR requires? -
first, do you have an entry for each player in the rfa table, just with a value of either "yes" or "no"? or is there simply no entry for the player if they have no RFA? if the former, you will need to change the condition to rfa.rfa <> 'Yes', since even when they have no RFA, they will still have a value for it. as for the PGID issue, it might be helpful to stipulate which table all the data is coming from: SELECT players.* FROM players ... see if that helps. if it doesn't, try print_r() on $row to see what data is coming out of the query - that might help you find the correct key. i would suspect it's tripping up because there is a PGID in both tables.
-
[SOLVED] override input from combo box with text field
akitchin replied to lucy's topic in PHP Coding Help
simply place the text input below the select box, and then when you're processing the form, check if the text input is empty first: <select name="selected_data"> <!-- blah --> </select> <input type="text" name="override_selected_data" /> then, when processing the form: if (!empty($_POST['override_selected_data'])) { // use the value entered into $_POST['override_selected_data'] } else { // use the value selected in $_POST['selected_data'] } -
[SOLVED] override input from combo box with text field
akitchin replied to lucy's topic in PHP Coding Help
it would probably be easiest to simply put a text input below the select list. then, when you're processing the form, if the text box is NOT empty, use its value instead of the selected item. this would work on the assumption that the only reason anyone would enter anything into the text input is if they don't want to select one. -
in order to remove the dash, you can use str_replace() to replace the dash with an empty string. as for ignace's code, in case there are multiple dashes and you only want the very last portion, use: $var2 = end($parts); rather than specifying $parts[1] explicitly.
-
what will work better than a UNION here is a LEFT OUTER JOIN. you can pop table 2 onto the end of table 1's information and use RFA in the WHERE clause to determine if there is an entry for them there or not: SELECT t1.Names AS names, t2.RFA AS RFA FROM table1 t1 LEFT OUTER JOIN table2 t2 ON t1.ID=t2.ID WHERE t1.Contract_Left=1 AND t2.RFA IS NULL ORDER BY t1.Names the LEFT OUTER JOIN appends the matching row of t2 to the right-hand side of t1, and in the case that there is no matching row, it appends a series of NULL values. this helps to identify any item in t1 without a matching entry in t2.
-
you can fix the notice by checking if the item is set before checking if it is numeric. the reason it is giving an undefined index is because the index doesn't exist in the GET array (obviously the var wasn't passed with the URL): $currentpage = (isset($_GET['currentpage']) && is_numeric($_GET['page'])) ? (int)$_GET['currentpage'] : 1; the question and colon are part of the ternary form for if() statements - it's in the manual. briefly: (condition) ? statement if true : statement if false; note that although the notices do help point out spots where your code could (and should) be better written and places where potential problems may occur, they will not halt your script. you should try to set the error reporting at a lower level when you move this script to production. EDIT: beaten to the punch on the ternary front.
-
one option is to append the value into the array with a specific key, sort the array while preserving keys, and then move the array pointer to the element now located AFTER the one you just tossed in.
-
somewhat related: http://www.smh.com.au/technology/technology-news/microsoft-apologises-for-changing-race-in-photo-20090827-f04p.html
-
it's for future reference. the session_start() movement was a better-practice thing, the error code helps you debug future scripts (remove before putting these scripts into production, of course).
-
if it fails to work in IE8 even when using the POST-concatenated $rate variable, it's not IE8's session handling that is to blame. there's no real reason for IE8 to fail vs. any other browser if it's passing back the same form info that other browsers are, because beyond that, it's the server's duty. are you absolutely certain that only the browser has changed between attempts? did you maybe change the data submitted via the form?
-
as far as i know, it wouldn't really matter - PHP still has to extract the variables from the array returned by mysql_fetch_assoc() or mysql_fetch_row(). the only difference is the assignment of an intermediate variable. you could always run a benchmark. one question i've always had is why bother extracting the variables in the first place? why not just use them directly from the array returned by the fetching function?
-
the issue is with an SQL statement, and therefore the only way IE could be affecting it differently than other browsers (since it's a server-side statement) is through its handling of sessions. check your privacy settings on IE8; could be that its default is to reject all cookies, thereby killing sessions. as a simple verification, try echoing your SESSION vars when viewing with IE8. i vaguely recall old version of IE requiring the header "Cache-control: private" along with sessions, but i could be wrong. google IE8 and session handling and you may get some answers if the privacy settings aren't the issue. EDIT: premature posting - see the post below. i didn't notice the SESSION vars are being assigned directly from POST vars in the same section.
-
ah yes, forgive my reply - i hadn't thought through the question fully. you can achieve this using subqueries if you want to condense the query to one statement, however, this will be a rather inefficient process: SELECT name FROM applications WHERE applicant_id IN (SELECT applicant_id FROM answers WHERE question_id=2 AND answer='YES') AND applicant_id IN (SELECT applicant_id FROM answers WHERE question_id=3 AND answer='No') ORDER BY name hopefully someone with more SQL prowess can stumble on this thread and offer a better solution.
-
presuming that you have authentication in place that verifies the user's username and password, on the protected pages, you may simply add an item to the WHERE clause that checks the user's info: SELECT stuff FROM users WHERE username='$whatever' AND password='$whatever2' AND column='X' if the user doesn't have the appropriate value in `column`, the query won't SELECT anything (even if the username and password exist and are correct) and the authentication will fail. it's hard to offer any other solutions or fixes without seeing the relevant code you're currently working with.
-
no - you still need to construct the message list within the while() loop. what roopurt meant was that you return this string AFTER constructing it: function Guestbook(){ $guestbook = ''; $query = "SELECT name, comment FROM guestbook"; $result = mysql_query($query); while($row = mysql_fetch_row($result)){ $name = $row[0]; $comment = $row[1]; $guestbook .= "<b>Name</b> : {$name} <br>" . "<b>Message</b> : {$comment} <br><br>"; } return $guestbook; }
-
[SOLVED] How to use this function FillList ($sql, $selected=0)
akitchin replied to arjay_comsci's topic in Applications
1. use code tags. 2. post a question with any code posted. otherwise, my guess on how to use it is: FillList ($sql, $selected); but that's about as useful as your question. -
this is most certainly not best practice. in fact, it's terrible practice. you should never have potentially dynamic columns - what happens if you add a question? while you can easily insert a new entry into the `answers` table, you must add a new column and disrupt the structure of the `applications` column. your better bet is to SELECT from the `answers` table and, if you want the name of the applicant, JOIN the `applications` table on the condition you are using above: SELECT an.*, ap.name AS applicant_name FROM answers AS an LEFT JOIN applications AS ap ON an.applicant_id=ap.applicant_id WHERE (an.question_id=1 AND an.answer='YES') AND (an.question_id=2 AND an.answer='No') ORDER BY ap.name ASC you may add as many criteria as you like to specify question answers.
-
How to make a string from multiple checkbox values?
akitchin replied to giba's topic in Javascript Help
show us what you tried - we may be able to spot where you went wrong. -
from the rules and guidelines: the thread was quite clearly advertising lockerz, including referral links.
-
i have to be honest and say that i think those functions are overkill. there must be a simple discrepancy here, and my suspicion is that it's in the radio button's element code that holds the answer. i would guess it comes back empty because the answer has double-quotes in it and that they're forcing the value attribute to end early. for example: $answer = 'this is "the" answer.'; <input type="radio" name="q1289" value="<?php echo $answer; ?>" /> this will output: <input type="radio" name="q1289" value="this is "the" answer." /> obviously that will cause the value attribute to be cut short to "this is". in the case of your "yes" example, there aren't any characters between the opening double-quote for the value attribute, and the first double-quote in the answer. if you're certain all the escaping backslashes are eliminated from the database, you should only need to do two things: 1. use addslashes() on POST data BEFORE inserting into the database if magic_quotes are OFF. otherwise escaping backslashes are already inserted by PHP. optionally, you may choose to use mysql_real_escape_string(), but be certain that you've used stripslashes() if magic quotes is on (otherwise mysql_real_escape_string() will escape the backslashes added by magic_quotes, as well as the characters magic_quotes was trying to escape). for example, with magic_quotes on, the variable: "yes" would become \"yes\" if you then use mysql_real_escape_string() on that, you will end up with: \\\"yes\\\" because that function will escape the backslashes as well as the quotes. this is why we use stripslashes() if magic_quotes is on and we're using mysql_real_escape_string() to escape the data. 2. now that you've inserted the information into the database correctly, it should only contain quotes. when you retrieve it to place it into the value attribute of a radio button, use addslashes() before echoing so that it escapes any quotes that would otherwise interfere with the value's delimiter. doing only these two things should allow you to compare $_POST answers directly to the answers retrieved from the database. i fear using all the functions that you are using above will not help you learn about how escaping is done in PHP.
-
[SOLVED] PHP or HTML - Display 3 images per row
akitchin replied to Gamerz's topic in PHP Coding Help
are you telling me the manual entry for strrchr, which explains what parameters it takes, what it does, and what it returns, along with user comments and example code, didn't have "anything good?"