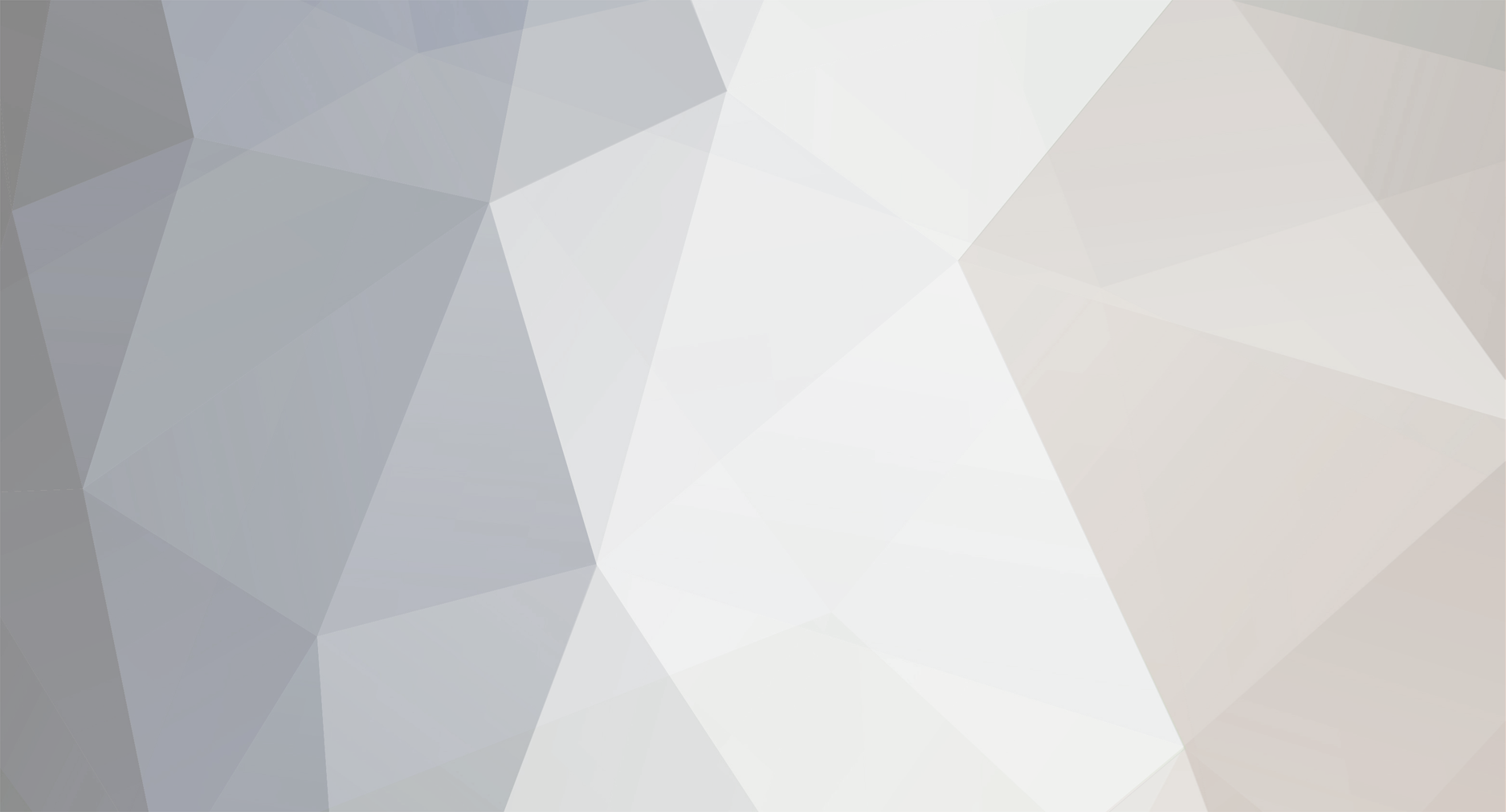
Daniel0
Staff Alumni-
Posts
11,885 -
Joined
-
Last visited
Everything posted by Daniel0
-
Well, that depends. If you're getting it from a database you could do something like this: $id = isset($_GET['id']) ? (int) $_GET['id'] : 0; // or you could do: $id = (int) filter_input(INPUT_GET, 'id', FILTER_UNSAFE_RAW) $result = mysql_query('SELECT * FROM users WHERE id = ' . $id . ' LIMIT 1'); if (mysql_num_rows($result)) { $user = mysql_fetch_assoc($result); // do stuff } else { echo 'User does not exist.'; }
-
if (!isset($_GET['id'])) { echo 'hi'; }
-
Well, say you have something like this in a form: <label for="pickupMonth">Month:</label> <select name="pickup[month]" id="pickupMonth"> <option value="1">January</option> <option value="2">February</option> <!-- etc. --> <option value="12">December</option> </select> <label for="pickupDay">Day:</label> <select name="pickup[day]" id="pickupDay"> <option value="1">1</option> <option value="2">2</option> <!-- etc. --> <option value="31">31</option> </select> <label for="pickupYear">Year:</label> <input type="text" name="pickup[year]" id="pickupYear"> Then you can do like this: if (!isset($_POST['pickup']['month']) || !isset($_POST['pickup']['day']) || !isset($_POST['pickup']['year'])) { echo 'please complete all fields'; } else if (!checkdate((int) $_POST['pickup']['month'], (int) $_POST['pickup']['day'], (int) $_POST['pickup']['year'])) { echo 'please enter a valid date'; } else { $mysqlDate = sprintf('%04d-%02d-%02d', $_POST['pickup']['year'], $_POST['pickup']['month'], $_POST['pickup']['day']); } To validate the date and make it fit a MySQL DATE field, which is stored in ISO 8901 format (that is YYYY-MM-DD).
-
You have this: while($getmsg3=mysql_fetch_array($getmsg2)) { $message=' '; $message=Smiley($message); //Smiley faces print "<font color='teal'><b>$getmsg3[name]:</b></font> $getmsg3[message]<br>"; } It should be: while($getmsg3=mysql_fetch_array($getmsg2)) { $getmsg3['message'] = Smiley($getmsg3['message']); print "<font color='teal'><b>$getmsg3[name]:</b></font> $getmsg3[message]<br>"; }
-
You will have to do $getmsg3['message'] = Smiley($getmsg3['message']);
-
Knock off your personal attacks. Both in PMs and here. You've been told the solution numerous times. I refuse to help you anymore.
-
It makes perfect sense. In the latter you are making the red text a link. In the former you are trying to make the link red, but seeing as the link already has its own style it's not affected by the rule you're trying to apply. If you cannot (or will not) understand it, then there is nothing I can do about it. You can show me all the screenshots in the world, but that doesn't mean you are right. You cannot say X is Y, therefore Z is Y when X and Z aren't the same things.
-
Seriously... Can you tell me the difference between these two things? <span style="color:red"><a href="#">foo</a></span> <a href="#"><span style="color:red">foo</span></a> Maybe you should stop acting smug when you don't know what you're doing.
-
Right, that's what I'm telling you. It will not result in a red link. You have to style the link yourself, hence the reason why you should learn CSS. I don't build bridges without knowing physics either.
-
[SOLVED] PHP Chat - Scroll down upon refresh
Daniel0 replied to twilitegxa's topic in PHP Coding Help
You could also periodically load new messages using AJAX and generate the DOM elements. That would prevent refreshing. -
Plain <font color="red">foo <a href="#">bar</a></font> will never result in a red link. Things aren't horseshit because you don't know how to use them. On the contrary, one could attach certain adjectives to you because you are using the things incorrectly, but I shall refrain from doing so.
-
Yeah, there is a reason. As I said, you should learn CSS. You need to apply rules to a selector called .se a otherwise the a will take precedence.
-
[SOLVED] PHP Chat - Scroll down upon refresh
Daniel0 replied to twilitegxa's topic in PHP Coding Help
Try something like this in your frame: <!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01//EN" "http://www.w3.org/TR/html4/strict.dtd"> <html> <head> <title>Test</title> </head> <body> <div> foo<br>foo<br>foo<br>foo<br>foo<br>foo<br>foo<br>foo<br>foo<br> foo<br>foo<br>foo<br>foo<br>foo<br>foo<br>foo<br>foo<br>foo<br> foo<br>foo<br>foo<br>foo<br>foo<br>foo<br>foo<br>foo<br>foo<br> foo<br>foo<br>foo<br>foo<br>foo<br>foo<br>foo<br>foo<br>foo<br> foo<br>foo<br>foo<br>foo<br>foo<br>foo<br>foo<br>foo<br>foo<br> foo<br>foo<br>foo<br>foo<br>foo<br>foo<br>foo<br>foo<br>foo<br> foo<br>foo<br>foo<br>foo<br>foo<br>foo<br>foo<br>foo<br>foo<br> foo<br>foo<br>foo<br>foo<br>foo<br>foo<br>foo<br>foo<br>foo<br> foo<br>foo<br>foo<br>foo<br>foo<br>foo<br>foo<br>foo<br>foo<br> foo<br>foo<br>foo<br>foo<br>foo<br>foo<br>foo<br>foo<br>foo<br> foo<br>foo<br>foo<br>foo<br>foo<br>foo<br>foo<br>foo<br>foo<br> foo<br>foo<br>foo<br>foo<br>foo<br>foo<br>foo<br>foo<br>foo<br> foo<br>foo<br>foo<br>foo<br>foo<br>foo<br>foo<br>foo<br>foo<br> foo<br>foo<br>foo<br>foo<br>foo<br>foo<br>foo<br>foo<br>foo<br> foo<br>foo<br>foo<br>foo<br>foo<br>foo<br>foo<br>foo<br>foo<br> foo<br>foo<br>foo<br>foo<br>foo<br>foo<br>foo<br>foo<br>foo<br> foo<br>foo<br>foo<br>foo<br>foo<br>foo<br>foo<br>foo<br>foo<br> foo<br>foo<br>foo<br>foo<br>foo<br>foo<br>foo<br>foo<br>foo </div> <script type="text/javascript"> window.onload = function() { window.scrollTo(0, document.body.scrollHeight); } </script> </body> </html> -
Oh alright So class="supercoolawesomelink" is better? Yeah, or "closed", or "important" or whatever.
-
If it's as "good" as SMF's, good luck trying to integrate it with anything. These kind of things are typically written so you will have to build your site around them and not so you can use them as a library.
-
Uh... yeah. It's built into every browser. Otherwise text that isn't explicitly styled by the web developer would be displayed all next to each other. I think what you should do is to learn about CSS. Amtran, class="red" is pointless. Classes should convey semantics, not presentation. What if you decide that all the links that have class="red" should later be blue?
-
[SOLVED] PHP Chat - Scroll down upon refresh
Daniel0 replied to twilitegxa's topic in PHP Coding Help
<div style="height:200px;overflow:auto" id="theDiv"> foo<br />foo<br />foo<br />foo<br />foo<br />foo<br />foo<br />foo<br />foo<br /> foo<br />foo<br />foo<br />foo<br />foo<br />foo<br />foo<br />foo<br />foo<br /> foo<br />foo<br />foo<br />foo<br />foo<br />foo<br />foo<br />foo<br />foo<br /> foo<br />foo<br />foo<br />foo<br />foo<br />foo<br />foo<br />foo<br />foo<br /> foo<br />foo<br />foo<br />foo<br />foo<br />foo<br />foo<br />foo<br />foo<br /> foo<br />foo<br />foo<br />foo<br />foo<br />foo<br />foo<br />foo<br />foo<br /> foo<br />foo<br />foo<br />foo<br />foo<br />foo<br />foo<br />foo<br />foo<br /> foo<br />foo<br />foo<br />foo<br />foo<br />foo<br />foo<br />foo<br />foo<br /> foo<br />foo<br />foo<br />foo<br />foo<br />foo<br />foo<br />foo<br />foo<br /> foo<br />foo<br />foo<br />foo<br />foo<br />foo<br />foo<br />foo<br />foo<br /> foo<br />foo<br />foo<br />foo<br />foo<br />foo<br />foo<br />foo<br />foo<br /> foo<br />foo<br />foo<br />foo<br />foo<br />foo<br />foo<br />foo<br />foo<br /> foo<br />foo<br />foo<br />foo<br />foo<br />foo<br />foo<br />foo<br />foo<br /> foo<br />foo<br />foo<br />foo<br />foo<br />foo<br />foo<br />foo<br />foo<br /> foo<br />foo<br />foo<br />foo<br />foo<br />foo<br />foo<br />foo<br />foo<br /> foo<br />foo<br />foo<br />foo<br />foo<br />foo<br />foo<br />foo<br />foo<br /> foo<br />foo<br />foo<br />foo<br />foo<br />foo<br />foo<br />foo<br />foo<br /> foo<br />foo<br />foo<br />foo<br />foo<br />foo<br />foo<br />foo<br />foo </div> <script type="text/javascript"> object = document.getElementById('theDiv'); object.scrollTop = object.scrollHeight; </script> Doesn't result in a noticeable lag between page load and scroll. -
Because it's a CSS issue, not a PHP issue. Just because you used PHP to output it doesn't mean it's where the problem is. You missed this part:
-
[SOLVED] I need to search an array for a string
Daniel0 replied to refiking's topic in PHP Coding Help
Use in_array. -
The global keyword and the $GLOBALS superglobal variable are both one of the very bad ideas implemented in PHP. Just saying. Learn how to pass things by argument and how to return values from a function.
-
You should have a strict DOCTYPE regardless. As for your problem, instead of using inline styles, define a class and then style it and the links within it. If you just try this: <span style="color:red">foo <a href="http://phpfreaks.com">test</a></span> you'll see that it will not result in a red link either. That's just not how things work.