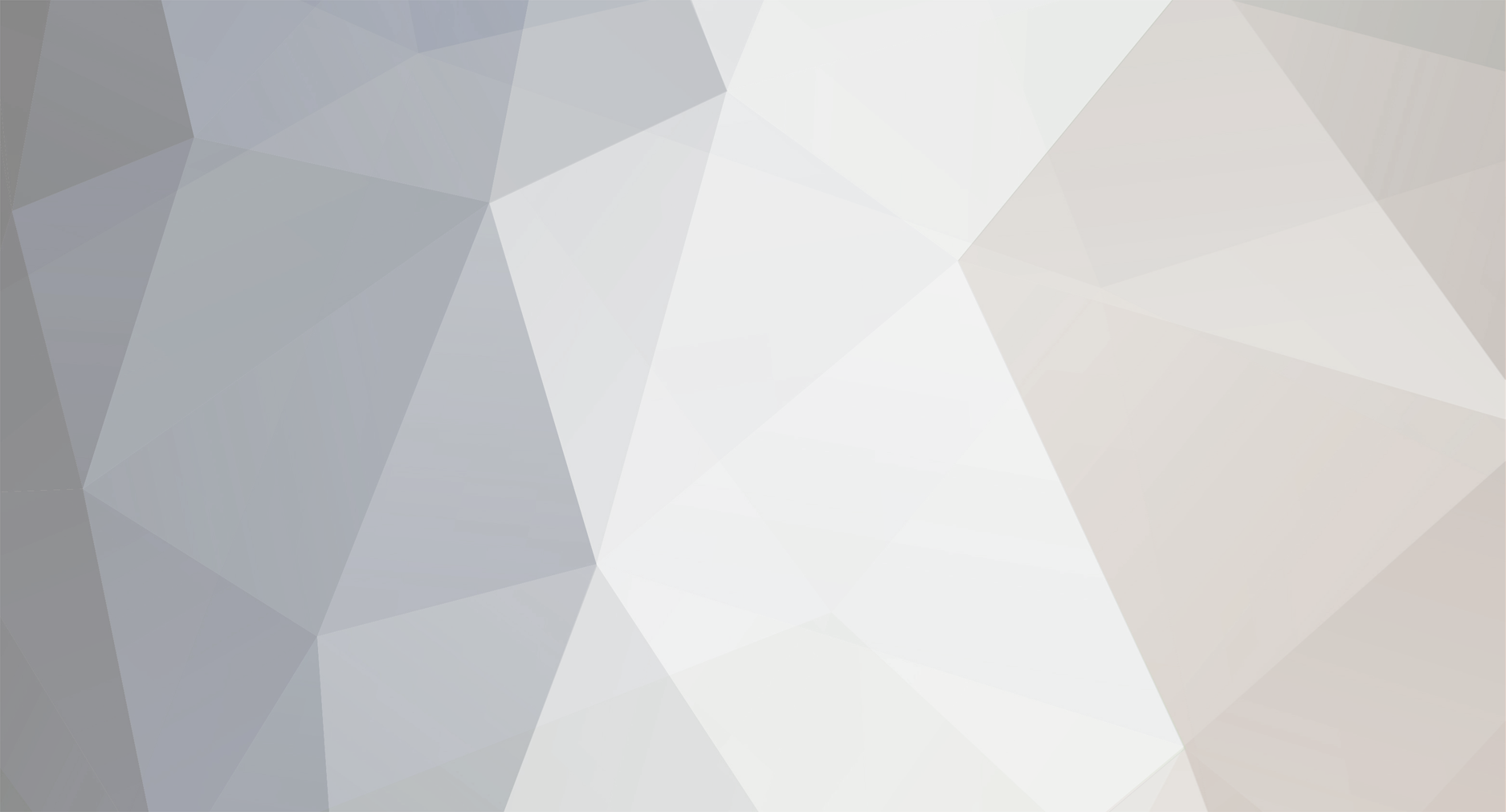
Daniel0
Staff Alumni-
Posts
11,885 -
Joined
-
Last visited
Everything posted by Daniel0
-
You know, there is no need to write a PHP parser yourself; there is already one in... PHP. Consider the following file (test.php): <?php function foo() { echo 'hello world'; } echo 2 + 4; function hello () { // aslkjdlakjds return 'abc' + 1;} echo 'more junk here'; ?> Then you can extract all the functions in that file like this: <?php $tokens = token_get_all(file_get_contents('test.php')); $started = false; $open = 0; $functions = array(); $tmp = ''; foreach ($tokens as $token) { if (!$started && $token[0] === T_FUNCTION) { $started = true; $tmp .= $token[1]; } else if ($started) { if (is_array($token)) { $tmp .= $token[1]; } else { $tmp .= $token; if ($token === '{') { ++$open; } else if ($token === '}') { if (--$open === 0) { $started = false; $functions[] = $tmp; $tmp = ''; } } } } } var_dump($functions); The output of running that would be: array(2) { [0]=> string(42) "function foo() { echo 'hello world'; }" [1]=> string(79) "function hello () { // aslkjdlakjds return 'abc' + 1;}" }
-
Also have a look at http://php.net/foreach
-
Oh really? Who would have known that?! Maybe you should read the topic before you reply so you don't repeat other people. PFMaBiSmAd said that in the second reply to this topic.
-
COM is for Windows so it won't work on other platforms.
-
How would redirecting the user take care of normalizing the string?
-
Doing it on-the-fly takes no time. I just tried on a random MP3 file and it took 0.04 seconds completing. If you have a lot of users and are worried about spending a lot of resources, pre-generating them and storing them on the disk would be a good idea. You could implement a trimming function using PHP, but it would be no more efficient than using ffmpeg. Actually, it's unlikely it would be more efficient. Thanks for the idea paddy. Will try it out. That won't work unless you're willing to let anyone download the entire file. What's preventing them from disabling Javascript or just checking out your HTML code?
-
Well, you could do if (strncmp($url, 'http://', 7) != 0) { $url = 'http://' . $url; } You're wrong about the www stuff. The domain name www.example.com doesn't need to point to the same place as example.com.
-
Assuming that you've got ffmpeg installed, that command will take the first 30 seconds of original.mp3 and put them in trimmed.mp3.
-
Need help with preg_match_all matching numbers with decimals
Daniel0 replied to alien73's topic in PHP Coding Help
[abc]? will work just fine See: daniel@daniel-laptop:~$ cat test.php <?php $pattern = '/^[abc]?$/'; var_dump(preg_match($pattern, 'a')); var_dump(preg_match($pattern, '')); var_dump(preg_match($pattern, 'hello')); ?> daniel@daniel-laptop:~$ php test.php int(1) int(1) int(0) Quantifiers just operate on the previous subpattern and a character class qualifies as a subpattern. -
Which version of PHP are you using? Anonymous functions were added in PHP 5.3.0. If you're using an earlier version you'll have to upgrade.
-
If you've got ffmpeg on your server, you can use it to do it. Something like this should do the trick: ffmpeg -t 30 -i original.mp3 -acodec copy trimmed.mp3
-
putting variables inside predefined variables. Possible?
Daniel0 replied to jtorral's topic in PHP Coding Help
Okay... How did you try it, and how does it "not work"? Help me help you. It's not like I can see what's going on on your computer, so vague statements like "it does not work" are entirely useless. -
<?php $array = array( "testInputElement" => array( "type" => "InputElement", "errorMessage" => "Please input a whole number between 30 and 90", "elementOptions" => array(), "validation" => function() {return false;} ), "testInputElement2" => array( "type" => "InputElement", "errorMessage" => "Error Message for form field 2 goes here", "elementOptions" => array(), "validation" => function() {return false;} ) ); var_dump($array); Gives no parse errors for me. Maybe you would like to enlighten us with what error you're getting?
-
Need help with preg_match_all matching numbers with decimals
Daniel0 replied to alien73's topic in PHP Coding Help
It will still match | (vertical bar) as a valid sign. Also, you don't need parentheses around that character class. -
putting variables inside predefined variables. Possible?
Daniel0 replied to jtorral's topic in PHP Coding Help
Do like $_COOKIE[$cn] without the quotes around the variable name. -
The syntax error you're getting has nothing to do with the anonymous functions. Try matching up the opening parentheses with the closing parentheses. You have one more closing parenthesis than opening ones.
-
Need help with preg_match_all matching numbers with decimals
Daniel0 replied to alien73's topic in PHP Coding Help
correct! Heh... are you sure? <?php $sym_price = 'My name is Daniel| 5.00 hello world '; if (preg_match_all("/[-|+]\s\d+\.\d{2}/", $sym_price, $matches)) { echo 'It matches'; } -
Need help with preg_match_all matching numbers with decimals
Daniel0 replied to alien73's topic in PHP Coding Help
Not tested, but how about this? /^[+-]?\d+\.\d+$/ Or if you only want the non-fractional part (is there a word for that) to be a multiple of 10, then perhaps like this: /^[+-]?10*\.\d+$/ -
What exactly are you trying to do? Any reason why you can't use reflection to get information about classes?
-
No, not necessarily. Only if you use the default settings. You may change the lifetime of the session cookie using the session.cookie_lifetime php.ini directive. You can set this (and other settings) using session_set_cookie_params. If you want it in a database (or somewhere else) instead, you can also use session_set_save_handler to accomplish that. Persisting logins are needed in virtually all applications, and that's why there is built-in support for it in PHP. That way everybody don't have to reinvent it all the time.
-
Have a look at the operator precedence table. Type casting takes higher precedence than division.
-
I can not see any HTML tag before DOCTYPE declaration. Then you need to look closer. [attachment deleted by admin]
-
Any particular reason why you can't just use PHP's built-in session mechanism?
-
Only youtube.com can set cookies for youtube.com. That's sort of the point...
-
It might be because you're both declaring an XML namespace and setting the DOCTYPE to an HTML one and that's what's confusing it.