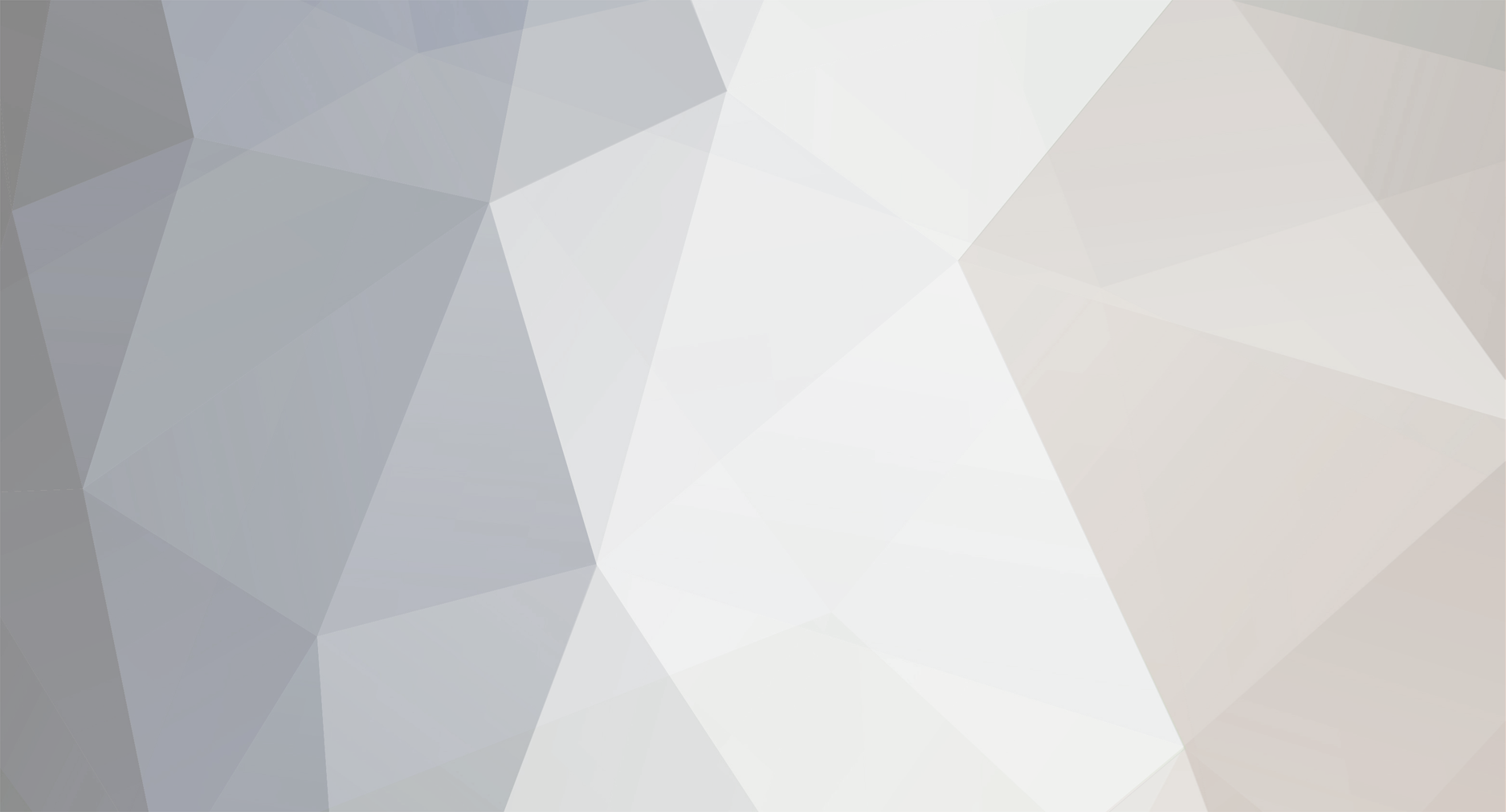
Daniel0
Staff Alumni-
Posts
11,885 -
Joined
-
Last visited
Everything posted by Daniel0
-
Can i somhow limit the function by definition?
Daniel0 replied to sangoku's topic in PHP Coding Help
PHP doesn't support scalar type hinting. Currently there are plans for implementing scalar type hints in a future version of PHP, but exactly how it's going to work hasn't been decided yet. -
I think underlined would be best. Underlining links is a convention. Everybody will understand what it means.
-
What is the best free "PHP Article organizer/manager"?
Daniel0 replied to ask9's topic in Miscellaneous
What's an "article manager"? -
Finding Nth last number in a Singly Linked List in Java
Daniel0 replied to proggR's topic in Other Programming Languages
If you have to need to select the nth element, why not choose a data structure that has O(1) access time like an array or hash table instead of a linked list which takes O(n)? If you just want to be able to move backwards, then use a doubly linked list. Just remember that finding the 998th last element in for instance a 1000 element list is the same as finding the 2nd element. For the general problem, it makes no difference if you go forwards or backwards. Worst case will always be that you have to go through all the elements. Maybe if you tell what you need the data for, we'll be able to help you better. -
http://www.google.com/search?q=wysiwyg+editor+javascript
-
Plus, you might also need the username in a non-HTML context. For instance if you send an email reminder.
-
If you want to know how to get started, how about looking at how WordPress MU does it?
-
http://www.phpfreaks.com/tutorial/php-security
-
We need to ban Firebug. Clearly it's a terrorist tool!!!1one!1
-
There are also PHP UNIX man pages available for the manual. $ pear channel-discover doc.php.net $ pear install doc.php.net/pman $ pman substr If you use vim, you can then add autocmd FileType php setlocal keywordprg=pman to ~/.vimrc and use the K command when hovering over a function name to look it up.
-
Go look up PHP's object model. That's exactly how it works already without passing by reference. A little example: <?php class Test { public $foo; } function doSomething(Test $obj) { $obj->foo = 'hello'; } $foo = new Test(); $foo->foo = 'hi'; doSomething($foo); var_dump($foo->foo); Output: string(5) "hello"
-
Why are you creating a reference in the first place?
-
You didn't violate any rules. I don't think you offended anyone either.
-
Your code will never return an error if there are no records in the database. It will give you an error if the query fails to execute.
-
It loops over the rows and uses the A and B colums for the matrix indices and C for the value. Matrices are usually implemented using 2D arrays.
-
The first rule of implementing crypto is don't implement crypto. The fact that you are asking this question shows that you shouldn't implement crypto. No offense.
-
Untested: $matrix = array(); foreach ($rows as $row) { if (is_array($matrix[$row['A']])) $matrix[$row['A']] = array(); $matrix[$row['A']][$row['B']] = $row['C']; }
-
On the server or on the client? You can't do this on the client. The reasons why you can't should be fairly obvious.
-
Uh... you're not banned. The ban list is empty actually. Also, please don't bump old topics.
-
That should be pretty easy, just google 'sanitizing inputs php' Again, google that or visit http://regexlib.com/ or http://www.regular-expressions.info/examples.html And possibly this: http://php.net/filter
-
Email piping: Add something like daniel: "|/path/to/some/script.php" to /etc/aliases, run newaliases and then I can read emails to "daniel" from STDIN in that script. It hasn't really got anything to do with PHP, but more with how you setup a mail server. PHP shell: You mean like this. You just run your script by typing php script.php instead of going to http://localhost/script.php. That part of the manual contains the differences between the SAPIs. Using C with PHP: What's that supposed to mean?
-
Of course not. How you would sanitize a value depends on what you're going to use it for. For instance, mysql_real_escape_string is useless for HTML and htmlentities is useless for MySQL databases, and neither are meant for sanitizing data for functions like exec.
-
The regular count function should work equally well for versions prior to 5.3.0.
-
For what it's worth, this will extract functions, classes and interfaces from a file. Took me ten minutes to write. I bet it took longer time writing those regular expressions. Sample file (test.php): <?php function foo() { echo 'hello world'; } echo 2 + 4; function hello () { // aslkjdlakjds return 'abc' + 1;} class Hello { function thisMethodWontGetIncludedInFunctions() { echo 'foo'; } } abstract class Foo {} final class Bar {} interface Baz {} echo 'more junk here'; ?> Parser: <?php class FileParser { private $_path; private $_parsed = false; private $_classes = array(); private $_functions = array(); private $_interfaces = array(); public function __construct($path) { $this->_path = $path; } private function _parse() { if ($this->_parsed) return; $parsing = null; $tmp = ''; $open = 0; foreach (token_get_all(file_get_contents($this->_path)) as $token) { if ($parsing === null && is_array($token)) { switch ($token[0]) { case T_FUNCTION: $parsing = T_FUNCTION; break; case T_CLASS: case T_ABSTRACT: case T_FINAL: $parsing = T_CLASS; break; case T_INTERFACE: $parsing = T_INTERFACE; break; } if ($parsing !== null) $tmp .= $token[1]; } else { if (is_array($token)) { $tmp .= $token[1]; } else { $tmp .= $token; switch ($token) { case '{': ++$open; break; case '}': if (--$open === 0) { switch ($parsing) { case T_FUNCTION: $this->_functions[] = $tmp; break; case T_CLASS: $this->_classes[] = $tmp; break; case T_INTERFACE: $this->_interfaces[] = $tmp; break; } $parsing = null; $tmp = ''; } break; } } } } $this->_parsed = true; } public function getClasses() { $this->_parse(); return $this->_classes; } public function getFunctions() { $this->_parse(); return $this->_functions; } public function getInterfaces() { $this->_parse(); return $this->_interfaces; } } $parser = new FileParser('test.php'); var_dump( $parser->getFunctions(), $parser->getClasses(), $parser->getInterfaces() ); Output: array(2) { [0]=> string(42) "function foo() { echo 'hello world'; }" [1]=> string(81) "+;function hello () { // aslkjdlakjds return 'abc' + 1;}" } array(3) { [0]=> string(95) "class Hello { function thisMethodWontGetIncludedInFunctions() { echo 'foo'; } }" [1]=> string(21) "abstract class Foo {}" [2]=> string(18) "final class Bar {}" } array(1) { [0]=> string(16) "interface Baz {}" }
-
Well, it was not meant to be a complete solution for you. You were supposed to extend it in the same manner to capture classes. Take a look at how it works. When a function declaration starts it ignores the tokens and just adds them to the string until the function declaration ends. You can do the same with classes. When a class declaration starts, ignore everything until it ends.