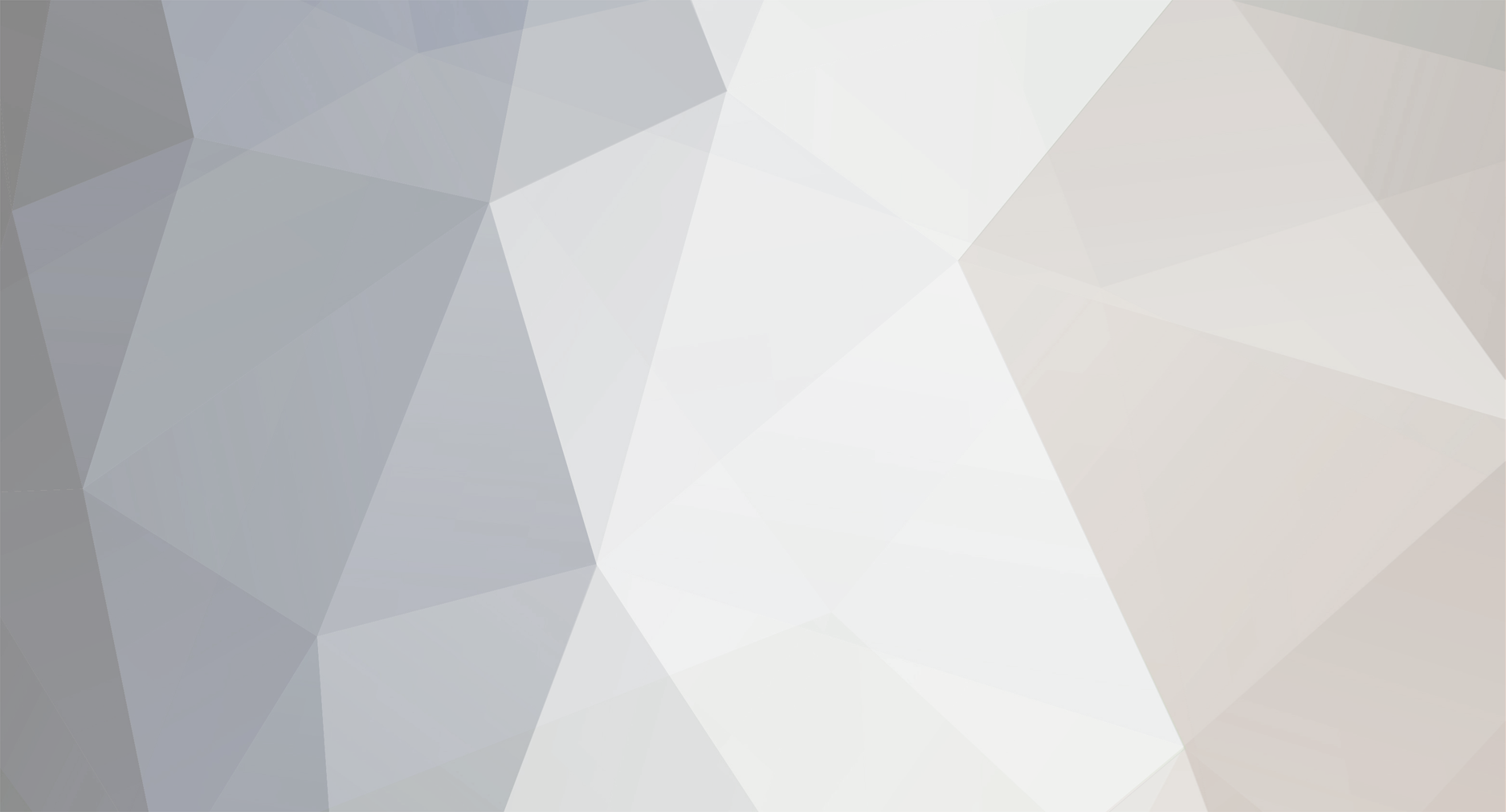
Daniel0
Staff Alumni-
Posts
11,885 -
Joined
-
Last visited
Everything posted by Daniel0
-
Uhm... well, you see, for the most part, in aggregation the containing object shouldn't be concerned with the instantiation of the contained object. Otherwise you create a dependency issue. Consider the following definitions: abstract class Animal { private $name; public function __construct($name) { $this->name = $name; } public function getName() { return $this->name; } } class Monkey extends Animal {} class Elephant extends Animal {} Now imagine this: class Zoo { private $animals = array(); public function __construct() { $this->animals[] = new Monkey('Joe'); $this->animals[] = new Elephant('Jack'); } } $zoo = new Zoo(); Now consider the following instead: class Zoo { private $animals = array(); public function addAnimal(Animal $animal) { $this->animals[] = $animal; return $this; } } $zoo = new Zoo(); $zoo->addAnimal(new Monkey('Joe')) ->addAnimal(new Elephant('Jack')); What's the difference? Both result in Zoo having the same animals, you could say that Zoo has the same state in both instances. In the first example the zoo is dependent on Monkey and Elephant. In the second example the zoo has has the individual animals passed to it. This decouples the elements is thus better designed. The latter example is called aggregation. There is another thing called composition. Essentially, the difference between composition and aggregation is that with composition, when the owning object ceases to exist, so do the contained objects. In aggregation that's not the case, the contained objects will persist even if the containing object doesn't. Now, in terms of your zoo, the latter would be more true to real life seeing as it uses composition whereas the former uses composition. If a zoo closes in real life, then it doesn't automatically mean that all the animals in the zoo will die. In OOP you can say that you are modeling the world using objects that represent real life entities. Of course you cannot decouple all classes from all classes. That wouldn't be a natural system. Some things are logically connected to each other. The idea is, however, to avoid establishing unnecessary dependencies in your application because that could result in maintainability and portability issues.
-
Well, that's definitely a no-go. This means you are creating inter-object dependencies which sort of counters OOP's decoupling concepts.
-
Nope, I'm being 100% honest. Either of those could be the best depending on what you're trying to do. In your latest example it appears that two in example two is a factory. Even then, without two's role being clear it's not possible to determine the best practice, though it would indicate that in this particular case example two is. Trust me when I say that best practice cannot be determined without a context.
-
Yes, but as mentioned, by doing that you're breaking forward compatibility. Now imagine that after doing this, IE will support XHTML. You are then actively hindering the adoption of the very technology you yourself is promoting. Of course your site alone won't make a difference, but the aforementioned is why UA sniffing is generally regarded as bad practice. Even then, why jump through hoops like that when you could just make it universally correct by coding in HTML 4.01 Strict?
-
Well, your code snippets don't make any sense, really. In neither of the examples do the class one accomplish anything and are as such redundant. Again, I'm afraid you'll have to come up with a concrete example before we can begin to talk about best practice. Without knowing what purpose the methods and classes serve it's impossible to tell which course of action would be superior.
-
You could do that, but history shows that UA sniffing breaks forward compatibility: http://webaim.org/blog/user-agent-string-history/ You cannot rely on content negotiation either. As explained earlier, Internet Explorer claims to support */* (i.e. literally anything) in its Accept header. That's obviously untrue.
-
Your second example is still invalid. To declare a class property you must use the keyword var in PHP4 or one of the keywords public, private or protected (depending on the wanted visibility) in PHP5. I'd follow Maq's recommendation of reading the part of the manual concerning OOP. In regards to best practice we cannot really tell which would be better. You'll have to come up with a concrete example to get a definite answer.
-
The second example is invalid. You cannot execute code within the body of a class definition. It has to be within a method. Neither are good though; they're both using the old PHP4 syntax for constructors.
-
You're committing to two logical fallacies: 1) Appeal to novelty (argumentum ad novitatem): Newer doesn't imply better. By that logic I can create any new standard and claim it as a replacement for XHTML, and as such it will automatically be better. It's a logical fallacy. 2) Appeal to common practice/tradition (argumentum ad antiquitatem): Common practice doesn't imply better. Just because a lot of people do X instead of Y doesn't make X better than Y. This would mean that it's better to use horse wagons instead of cars because that's what people always used to do. As a matter of fact, your argumentation is rather peculiar. You claim that XHTML is better because it's newer, but at the same time you claim that XHTML is better because that's what most people use. This essentially turns out to be a logical contradiction. HTML dates farther back than XHTML which would mean that HTML has had a larger user base than XHTML at some point, thus your two claims contradict each other. Further, by showing the "Validated W3C XHTML 1.0" boilerplate you aren't showing anything other than that you are ignorant and do not know what you are doing. I stopped counting the times I've said this, and it's amazing that it's not clear yet: XHTML served as text/html will NOT be parsed as XHTML but rather as HTML, which essentially means it's not XHTML after all. Finally, I really do not get you on the dirty/shiny part. It's completely nonsensical. HTML is not XHTML. Saying that HTML is bad because it doesn't follow XHTML's syntax doesn't make sense. That's like saying Lisp sucks because it doesn't use Haskell's syntax. Complete nonsense. I don't know. I sent them an email and I'll reply here with their answer. Even then that argument doesn't hold up though. How often haven't you heard about corrupt legislators for instance? Do you honestly believe that everybody in your parliament are 100% law-abiding all the time? It could also be that their web devs aren't the same people who are in the spec working groups. In that case it could be that their web devs are oblivious to the fact that what they're doing is wrong according to the specs. I do agree that it's somewhat paradoxical that they aren't compliant with their own recommendations though.
-
Sorry to break it for you, but W3Schools isn't affiliated with W3C. W3Schools is created by an independent consultancy firm called Refsnes Data based in Norway. I guess they're called W3Schools because they try to teach you the technologies set forth by W3C.
-
Indeed, I don't despise XHTML. I just don't think that it currently is a viable option for web development unless you have very particular needs such as having to use e.g. MathML. I also do not see how you can discard all the facts presented in this thread and just say "but I prefer XHTML".
-
Why not learn both? Computer science is a gigantic toolbox. The more you know (the more tools that are in your toolbox) the more things you can do and the more your skills will be worth. Part of what makes you a good developer is knowing when to use what tool over another. In this instance XHTML really is the inferior choice, at least until Microsoft decides to give IE XHTML support and/or IE's market share becomes too insignificant to bother with. I'm afraid there is no catch-all good-for-everything technology you can just use. Otherwise we might as well just use binary punch cards for everything related to computing. Sorry, I didn't actually notice your remark about my website. I just added the "on PHP Freaks we use" thing as a disclaimer before anyone mentioned it. Anyway, the HTML markup is the default Wordpress theme. I've been thinking about creating a custom one for a while, but I've always postponed it for more important stuff such as paid freelance web development and consulting.
-
That's nonsense. The HTML parsing rules are perfectly clear. That's not more difficult to parse. Not all elements allow omission of the end tag. A tag like <p> is an example of a tag where that's optional. The DTD clearly specifies which tags that are allowed to be within a paragraph tag, so if it meets a tag that's not allowed inside <p> then it'll just implicitly close it and start the other one. It's perfectly simple. Again, no problem. Algorithmically it's not really difficult to do a case-insensitive match. Still not a problem. It's very precisely defined which characters you are allowed to use if you omit the quotes. You are allowed to use characters the letters a-z and A-Z, digits 0-9, hyphens, periods, underscores and colons. It's not difficult to parse that at all. So what? How is that a problem? The specs allow for empty attribute values and it's not at all difficult to parse that. Maybe you should have a read over the specs before making claims like that. In fact, in my opinion the HTML 4.01, XHTML 1.0 and CSS 2.1 specifications are required reading for anyone who is serious about web development. As a matter of fact (and this is probably fourth time I've said it now), your so-called "rigid" XHTML is parsed according to the so-called "loose" and "ambiguous" rules of HTML seeing as you're unlikely to block off all the IE users by serving it as application/xhtml+xml.
-
DOM means Document Object Model. It's a hierarchical tree composed of all the elements of an (X)HTML document (plus a lot of other stuff). Each of the objects in the the DOM tree are called nodes. Each node has a series of attributes. When you are accessing an HTML element using Javascript then it's DOM you're using. Here are some links for you: http://www.w3.org/DOM/DOMTR http://www.w3.org/TR/2000/WD-DOM-Level-1-20000929/ http://www.w3.org/TR/DOM-Level-2-Core/ http://www.w3.org/TR/DOM-Level-3-Core/
-
Yes you can, but you are currently not doing it. You are not using XHTML, but invalid HTML. It will never be XHTML unless you give it the correct mime-type. All UAs are rendering your documents as HTML, a subset of SGML and thus according to its rules. I know this forum is wrongly served as XHTML 1.0 Transitional with text/html, but that's SMF's fault and you'll have to get them to change it. I also know that the main site here is served as XHTML 1.0 Strict with text/html, but that will be fixed in the next release and changed to HTML 4.01 Strict. It's also not just a matter of syntax. There are differences in both DOM and CSS depending on whether the document is XHTML or HTML. Some tags are implicit in HTML, for instance the <tbody> tag. If you omit a <tbody> tag in a <table> then it will still exist DOM. That's not the case in XHTML. It will not be there. HTML is also case-insensitive whereas XHTML isn't. This goes in DOM as well. In XHTML someDocument.onClick is valid in HTML, but invalid in XHTML. You'll also find that document.write() doesn't work in XHTML for instance. You also said yourself that while temporarily having switched to the correct mime type that your Javascript broke. This further emphasizes the fact that it's not just a matter of changing the mime type when switching between XHTML and HTML. There are other, not always obvious, things that need to be taken into consideration, and in some instances those subtle differences may break things. Another reason why one might choose HTML over XHTML could be size considerations. HTML can be smaller because it for some attribute values do not require quotes, some end tags are not required and you will not be using the self closing tag. For a single request this difference is of course negligible, but if it's intended for an environment with extremely limited bandwidth or you are receiving a massive volume of hits then it can make a difference. Take for instance Google. On all their pages they get several billions of hits each months and using HTML can make a notable difference considering it was estimated in 2006 that YouTube alone spent 1 million dollars each month on bandwidth source. Three years have passed since and the bandwidth will undoubtedly have increased.
-
No, you're forgetting something. HTML is forgiving, XHTML (XML) is not. You ARE using HTML because you serve it as text/html. The DTD is not what determines whether a document is XHTML or HTML. It's the mime type. The DTD is merely a set of grammar constraints. Without a DTD, an XML document cannot be said to be valid, but only well-formed. Well-formed-ness is determined the syntax ("are all elements closed?", "are the elements nested properly?" etc.). Validity is determined by DTD. The DTD specifies which elements that are allowed, which elements that are allowed within other elements, which attributes an element may have and possibly what values an attribute may have. Now, seeing as you serve your document as text/html this means it will be parsed according to the rules of HTML, and HTML is not XML despite the fact that it looks fairly similar to it. Because HTML is forgiving it means that it can work without being correct. You are not using XHTML. You are per definition only using XHTML if your document has the mime-type application/xhtml+xml. Saying that your document is XHTML otherwise is pure rubbish.
-
That something works doesn't mean it's used the correct way. People might be able to understand me if I use incorrect grammar when speaking or writing, and as such you could say that it "works". However, just because people understood what I said doesn't make my grammar correct. "Works" and "is correct" are two entirely different concepts.
-
Except you're using it the wrong way, which sort of invalidates your views.
-
The reason why it didn't work for you was that you were trying to make a constraint where sent_to AND sent_by equals id in profile. That'll only be true if sent_to=sent_by, which it never should, thus it fails.
-
Because people think XHTML is cooler and because they do not know what they're doing.
-
Sorry, MTA = Mail Transfer Agent. It's the mail server. Could be e.g. sendmail, postfix or qmail. Yes.
-
Try to make two separate constraints, i.e. ALTER TABLE interest ADD FOREIGN KEY(sent_by) REFERENCES profile(id) ON DELETE CASCADE ON UPDATE CASCADE; ALTER TABLE interest ADD FOREIGN KEY(sent_to) REFERENCES profile(id) ON DELETE CASCADE ON UPDATE CASCADE;
-
The receiving MTA.
-
You can only do that if you have direct access to the server.
-
Decrypt a swf encrypted with Amayeta SWF Encrypt?
Daniel0 replied to Welling's topic in Application Design
You have already decrypted it. There is no such thing as a deobfuscator though. It's not really possible to know what the original variables, functions, etc. were called.