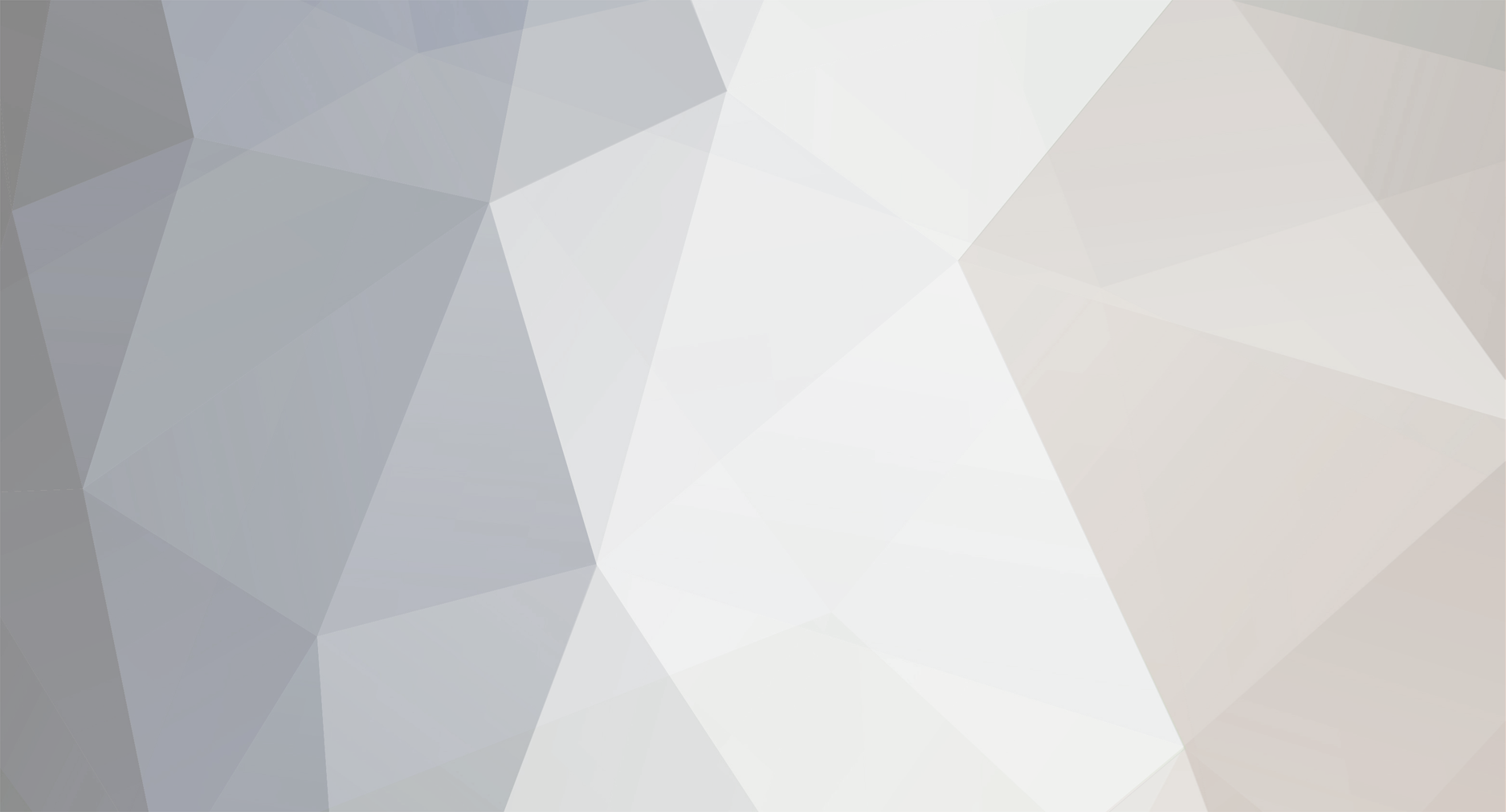
Daniel0
Staff Alumni-
Posts
11,885 -
Joined
-
Last visited
Everything posted by Daniel0
-
<?php $string = "This is a test of the truncate function"; function truncate($string, $max_length) { if(strlen($string) > $max_length-3) { $string = substr($string, 0, $max_length-3).'...'; } return $string; } echo truncate($string, 15); // This is a te... function truncate2($string, $max_words) { $str = null; if(str_word_count($string) > $max_words) { preg_match('/^\s*(?:\S+\s*){1,'. (int) $max_words .'}/', $string, $matches); $str = trim($matches[0]).'...'; } else { $str = $string; } return $str; } echo truncate2($string, 4); // This is a test... ?>
-
Using a table-less design would make it more search engine friendly.
-
mysql_tablename()
-
Use double quotes and put curly brackets around the variables. That is needed when you are using arrays. Variables will only be parsed when enclosed in double quotes. Edit: You should also use single or double quotes around the array keys. Else you are using constants which is not what you intended. Edit 2: Without curly brackets is called simple syntax and with is called complex syntax (see http://php.net/string)
-
You think you could perhaps look a little longer before posting instead of posting 11 in a row in less than an hour? It can't be that hard to open notepad or something and write it in, then paste it in the post box when you think you're done. In apply.php it says:
-
Try <p>Total Tutorials <?php echo $count;?></p> <?php $result = mysql_query("SELECT SUM(tutorialviews + tutorialviews) FROM tutorials"); $row = mysql_fetch_row($result); ?> <p>Total Views <?php echo $row[0];?></p> instead.
-
You could use heredoc: <?php $string <<<EOF lots of stuff here bla bla bla bla bla bla asdasda sdasd EOF; ?> or you could use output buffering: <?php ob_start(); ?> lots of stuff here bla bla bla bla bla bla asdasda sdasd <?php $string = ob_get_contents(); ob_end_clean(); ?>
-
It echoes it because you step out of PHP. You need to return it.
-
[FireFox] AJAX fetch works when FireBug enabled, not when disabled?! Ideas?
Daniel0 replied to zq29's topic in Miscellaneous
Weird. Which error do you get when Firebug is off? -
[SOLVED] Radio Group inside a while statement wont check
Daniel0 replied to Asheeown's topic in PHP Coding Help
Well, we haven't seen your code, so we can't tell. -
It's because you defined it as $encrypt_pw without a "p".
-
[SOLVED] Radio Group inside a while statement wont check
Daniel0 replied to Asheeown's topic in PHP Coding Help
Did you clear your cache after changing the code? Ctrl+F5. If you refresh normally then the browser will select whatever was selected before. -
SSL encrypts the connection. It means Secure Sockets Layer.
-
Or change mysql_query("UPDATE user_info SET email = '$_POST[settings_email]', location = '$_POST[settings_location]', avatar_url = '$_POST[settings_avatar]', url = $_POST[settings_webpage]' WHERE username = '$_SESSION[uSERNAME]'"); to mysql_query("UPDATE user_info SET email = '{$_POST['settings_email']}', location = '{$_POST['settings_location']}', avatar_url = '{$_POST['settings_avatar']}', url = '{$_POST['settings_webpage']}' WHERE username = '{$_SESSION['USERNAME']}'"); Do you understand those changes and why it'll work while yours won't? Do you understand why e.g. <?php $array = array( array( 'type' => 1, 'name' => 'hi', ), array( 'type' => 2, 'name' => 'hello', ), ); echo "$array[0]['name']"; ?> will output Array['name'] and not hi?
-
Change return ( $college['type'] = 'college' ); to return $college['type'] == 'college' ? $college : false;
-
number_format()
-
This is a pretty stupid poll IMO. Which would you rather be doing? Driving a car or eating an apple?
-
fileperms() will do that.
-
<?php mkdir('test'); mkdir('test'); ?> Warning: mkdir() [function.mkdir]: File exists in /var/www/test.php on line 3
-
Unexpected character in input error, please help :-\
Daniel0 replied to NightBeam's topic in PHP Coding Help
Why? It isn't really as hard as somebody makes it seem like. Going through http://php.net/oop5 should enable you to understand the code I posted before. I found this link in one of the comments on the OOP5 section in the manual - it's an okay read too. -
Unexpected character in input error, please help :-\
Daniel0 replied to NightBeam's topic in PHP Coding Help
You should look into making a database class. It'll make it all much easier... Example (not tested, might not work - just fix it if it doesn't): <?php abstract class Database { protected $link; protected $num_queries; protected $queries = array(); protected $last_result; abstract public function __construct($host, $username, $password, $database, $persistent=false); abstract public function query($sql); abstract public function fetch_row($result=null); abstract public function get_num_rows($result=null); abstract public function escape_string($string); public function insert($table, $data=array(), $escape_data=true) { $fields = array(); $values = array(); foreach($data as $field => $value) { $fields[] = $field; $values[] = $escape_data ? "'".$this->escape_string($value)."'" : "'{$value}'"; } return $this->query("INSERT INTO {$table} (".join(',',$fields).") VALUES (".join(',',$values).")"); } } class Database_MySQL extends Database { public function __construct($host, $username, $password, $database, $persistent=false) { $function = $persistent ? 'mysql_pconnect' : 'mysql_connect'; $this->link = @$function($host, $username, $password); if(!is_resource($this->link)) { throw new Exception("Failed connecting to database: ".mysql_error()); } if(!mysql_select_db($database, $this->link)) { throw new Exception("Failed selecting database '{$database}': ".mysql_error()); } } public function query($sql) { $this->num_queries++; $this->queries[] = $sql; $this->last_result = mysql_query($sql, $this->link); if(!is_resource($this->last_result)) { throw new Exception("Failed executing query: ".mysql_error()); } return $last_result; } public function fetch_row($result=null) { return mysql_fetch_assoc(is_resource($result) ? $result : $this->last_result); } public function get_num_rows($result=null) { return mysql_num_rows(is_resource($result) ? $result : $this->last_result); } public function escape_string($string) { return mysql_real_escape_string($string, $this->link); } } $db = new Database_MySQL('localhost', 'root', '', 'something'); $db->insert('users',array( 'username' => 'Somebody', 'password' => md5('qwerty'), 'email' => '[email protected]', )); ?> -
Unexpected character in input error, please help :-\
Daniel0 replied to NightBeam's topic in PHP Coding Help
Try if (isset($_POST['edit'])) { if ($result[$permissionfield] > 1) { $worked = mysql_query('UPDATE '.$arrivaltable.' SET ' ."{$Field2}='".mysql_real_escape_string($_POST[$Field2])."'" ."{$Field3}='".mysql_real_escape_string($_POST[$Field3])."'" ."{$Field4}='".mysql_real_escape_string($_POST[$Field4])."'" ."{$Field5}='".mysql_real_escape_string($_POST[$Field5])."'" ."{$Field6}='".mysql_real_escape_string($_POST[$Field6])."'" ."{$Field7}='".mysql_real_escape_string($_POST[$Field7])."'" ."{$Field8}='".mysql_real_escape_string($_POST[$Field8])."'" ."{$Field9}='".mysql_real_escape_string($_POST[$Field9])."'" ."{$Field10}='".mysql_real_escape_string($_POST[$Field10])."'" ."{$Field11}='".mysql_real_escape_string($_POST[$Field11])."'" ."{$Field12}='".mysql_real_escape_string($_POST[$Field12])."'" ."{$Field13}='".mysql_real_escape_string($_POST[$Field13])."'" ."{$Field14}='".mysql_real_escape_string($_POST[$Field14])."'" ."WHERE '".mysql_real_escape_string($_POST[$Field1])."'"); echo ($worked ? '<p>The edit was successful.</p>' : '<p>The edit was not processed correctly.</p>'); } else { /* I'd edit this if I were you... , I couldn't think of anything else. */ die('You do not have the authority to edit information. Please consult an employee who does.'); } -
Unexpected character in input error, please help :-\
Daniel0 replied to NightBeam's topic in PHP Coding Help
Hmm... ."{$Field2}='{$_POST[$Field2]}'", Note: You should escape the values... ."{$Field2}='".mysql_real_escape_string($_POST[$Field2])."'", -
Unexpected character in input error, please help :-\
Daniel0 replied to NightBeam's topic in PHP Coding Help
Try .$Field2.'`= `'\''.$_POST[$Field2].'\\', `' instead (line 73). Same for similar lines. -
Unexpected character in input error, please help :-\
Daniel0 replied to NightBeam's topic in PHP Coding Help
I know I could just count, but I'm lazy... which line is line 73?