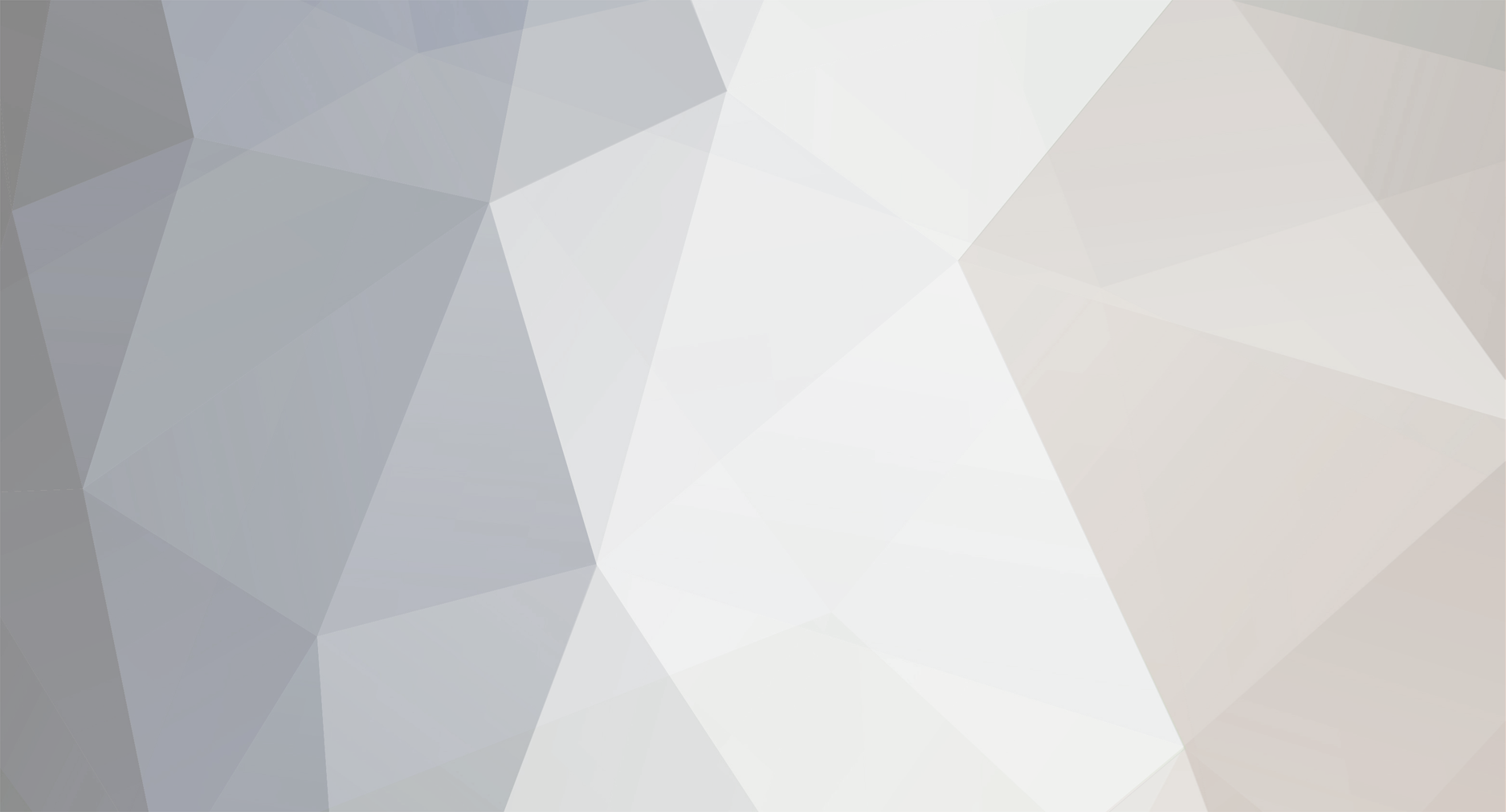
Daniel0
Staff Alumni-
Posts
11,885 -
Joined
-
Last visited
Everything posted by Daniel0
-
What is wrong with the snippet I provided you with? http://www.phpfreaks.com/forums/index.php/topic,273565.msg1293765.html#msg1293765
-
[SOLVED] Bitwise, base-2 categorization system acting oddly.
Daniel0 replied to Goldeneye's topic in PHP Coding Help
I suppose I would just store them in a tree like this: <?php $tree = array( array( 'name' => 'Category 1', 'children' => array( array( 'name' => 'Child 1', 'children' => array(), ), array( 'name' => 'Child 2', 'children' => array(), ), ), ), array( 'name' => 'Category 2', 'children' => array( array( 'name' => 'Child 3', 'children' => array( array( 'name' => 'Child 3-1', 'children' => array(), ), ), ), ), ), array( 'name' => 'Category 3', 'children' => array(), ), ); Writing it out it would look something like this: Category 1 --Child 1 --Child 2 Category 2 --Child 3 ----Child 3-1 Category 3 That would also support infinite depth (though still bounded by the computer's memory of course). -
[SOLVED] Bitwise, base-2 categorization system acting oddly.
Daniel0 replied to Goldeneye's topic in PHP Coding Help
I suppose I just don't see the point in using only powers of 2. I don't really see why you couldn't use a regular enumerated array. Oh yeah (and I know this might be seen as nitpicking), but it's still base 10. Base 2 is just using only two distinct digits that are raised to a power of 2. Effectively, (9)10 = (1001)2. You're not using base 10 only when using the numbers 1, 10, 100, 1000 etc. either. Of course the numbers are always stored internally in base 2 because that makes the circuits easier to make. Given any number in any base, you can always convert it to base b for some integer b > 1 (also called doing a base b expansion). Just wanted to get the nomenclature straight -
[SOLVED] Bitwise, base-2 categorization system acting oddly.
Daniel0 replied to Goldeneye's topic in PHP Coding Help
Why are you storing things like that in the first place? Anyways, seeing as using a string is a good enough compromise for you, you can just use bcpow. daniel@daniel-laptop:~$ php -r "echo bcpow("2", "1000") . PHP_EOL;" 10715086071862673209484250490600018105614048117055336074437503883703510511249361224931983788156958581275946729175531468251871452856923140435984577574698574803934567774824230985421074605062371141877954182153046474983581941267398767559165543946077062914571196477686542167660429831652624386837205668069376 -
[SOLVED] Bitwise, base-2 categorization system acting oddly.
Daniel0 replied to Goldeneye's topic in PHP Coding Help
Not on a 32-bit system, no. You'd have to use a version of PHP compiled for 64-bit on a machine and operating system that supports it. Even then you would just hit the barrier only a little higher. -
Something like foreach ($matches[1] as $link) { // do stuff }
-
Well, that's not really helpful. Post some sample input and output.
-
I suppose that depends on the kind of data you already have.
-
If you need to access the fourth element in an array called $array you'll do like this: $array[3] Note that many things in programming and computer science are 0 indexed whereas English generally is 1 indexed.
-
What is that supposed to mean?
-
Not necessarily. Iterating over an associate array would look ugly using e.g. a while loop. Assuming you have the following array: $array = array( 'foo' => 'bar', 'test' => 'hello', ); Iterating over it using a while loop would look something like this: $arrayKeys = array_keys($array); $arrayValues = array_values($array); $i = 0; $arraySize = sizeof($array); while ($i < $arraySize) { echo $arrayKeys[$i] . ' => ' . $arrayValues[$i] . PHP_EOL; ++$i; } Compare that to the following (which is much easier to read): foreach ($array as $key => $value) { echo $key . ' => ' . $value . PHP_EOL; } Another example would be creating an infinite loop (that you of course break out of somehow within the body). Using a while loop you would do this: while (true) { doSomething; } and the for loop equivalent would look like this: for (; { doSomething; } The for loop in this case would be most efficient (though not by much) because it doesn't need to evaluate a statement all the time.
-
Generally, any for loop will have the following structure: for (startingStatement; condition; iterationStatement) { body; } That can be written like this using a while loop: startingStatement; while (condition) { body; iterationStatement; } So it will never really matter if you use a while loop instead of a for loop. It's just two ways of writing the same thing. Thought it might be useful to know. Actually, in a for loop, all the things within the parentheses are optional, so you can also do this: for (; { something; }
-
"Keep looping until true" would pretty much be a while loop with a negated conditional statement because a while "keeps looping until false".
-
When and why should I use session_regenerate_id?
Daniel0 replied to fluvly's topic in PHP Coding Help
Is there a function that tracks the session requests? You can just do like this: if (++$_SESSION['lastRegeneration'] > 10) { $_SESSION['lastRegeneration'] = 0; session_regenerate_id(); } That should regenerate it every 10 requests. I did read what it does, if set to TRUE it deletes the old session file. If I use session_regenerate_id just for security purposes, would it make sense to keep the old session file, or would it be pointless? If you delete it, you lose all information about the user. Effectively this would log the user out. -
Try adding a Content-type header with a charset to the email. E.g. like this: <?php $to = "[email protected]"; $subject = "Invitation"; $email = $_REQUEST['email'] ; $message = $_REQUEST['message'] ; $headers = "From: $email\r\nContent-type: text/plain; charset=utf-8"; $sent = mail($to, $subject, $message, $headers) ; if($sent) {print "your email was sent"; } else {print "En error has occurred, try again , "; } ?>
-
When and why should I use session_regenerate_id?
Daniel0 replied to fluvly's topic in PHP Coding Help
In terms of security it can help foil session fixation attacks. If you obtain someone's session ID you can effectively log in as them. If the session ID changes frequently, the chance of a compromised session ID still being active decreases. Doing it right after login would be pointless. You should do it every X requests. I suppose that depends on what you want to use the function for. Did you read what it does? -
This post is primarily targeted at people who have posted asking for help as these seem to be the people who most frequently ask these questions. All PMs/emails to staff members regarding deletion requests will henceforth be ignored as they are answered in this thread. Q: Will you delete my post/topic? No. Q: Why won't you delete my post/topic? Imagine if everybody requested their posts to be deleted. If everybody did this there would be no community left, which means you couldn't get the help you required. Secondly, at about 700 posts per day, we would be very busy deleting posts if that was the case. Time is not really an issue, however. There are other reasons why we deny your deletion request. You've come to this forum asking for free help. You don't pay anyone any money, neither PHP Freaks nor the volunteer who is spending his/her spare time on helping you. We think that when you are getting such an awesome service for free, it is only fair that the help you have received is publicly archived for anyone to use. We also think that is a reasonable and very small price to pay. There is also another reason. As already mentioned, people have been helping you for free. By deleting your topic we are also deleting their work. That would be unfair. Q: But I didn't even get sufficient help, so why can't you just delete it? We are not interested in forming a committee with the purpose of finding out whether someone received adequate help or not each time someone asks. Q: But what if my client finds it? So what if your client finds out? If the topic reveals that you are incompetent at doing the work your client pays you to do, then you should not have taken the job in the first place. At least be adult enough to admit you have received help instead of relying on lies and deception. Q: But what if my teacher finds it? If your teacher finds it and you were supposed to do it yourself, it's your own fault for cheating. Q: Aren't you guys human? While the real identity of all staff members hasn't been verified, we are pretty certain that all our staff members belong to the homo sapiens species (except for CV..whatever (s)he/it is, it's not human...). Really though, trying to shift the blame is pretty pointless. Before you registered, you agreed to our Terms of Service stating that you will grant us an irrevocable license to use content you post using our systems. For added convenience we added a big red notice on all post forms informing you that we will not delete the things you post. You posted knowing these terms. Q: But my post makes me look immature/foolish/stupid/unintelligent. Won't you delete it? "Think before you speak" is a good rule to remember that applies both in real life and on the internet. Q: Won't you make an exception just this time? Sure! Like we'll make an exception for everybody else who asks... No. Q: So what should I do? If you're unwilling to accept that your topics here will be archived publicly, you should hire a consultant to help you in private. This rule blows! Maybe it does, but we did not force you to visit this web site. Basically, think of your posts as emails. You cannot alter emails once they have been sent either. Nor can you take them back (delete them). Think of your post like a speech transmitted live on television. You cannot change what you say there either. Consider what you write before you hit the "post" button. Your post is probably not so important that it cannot wait one minute while you do that.