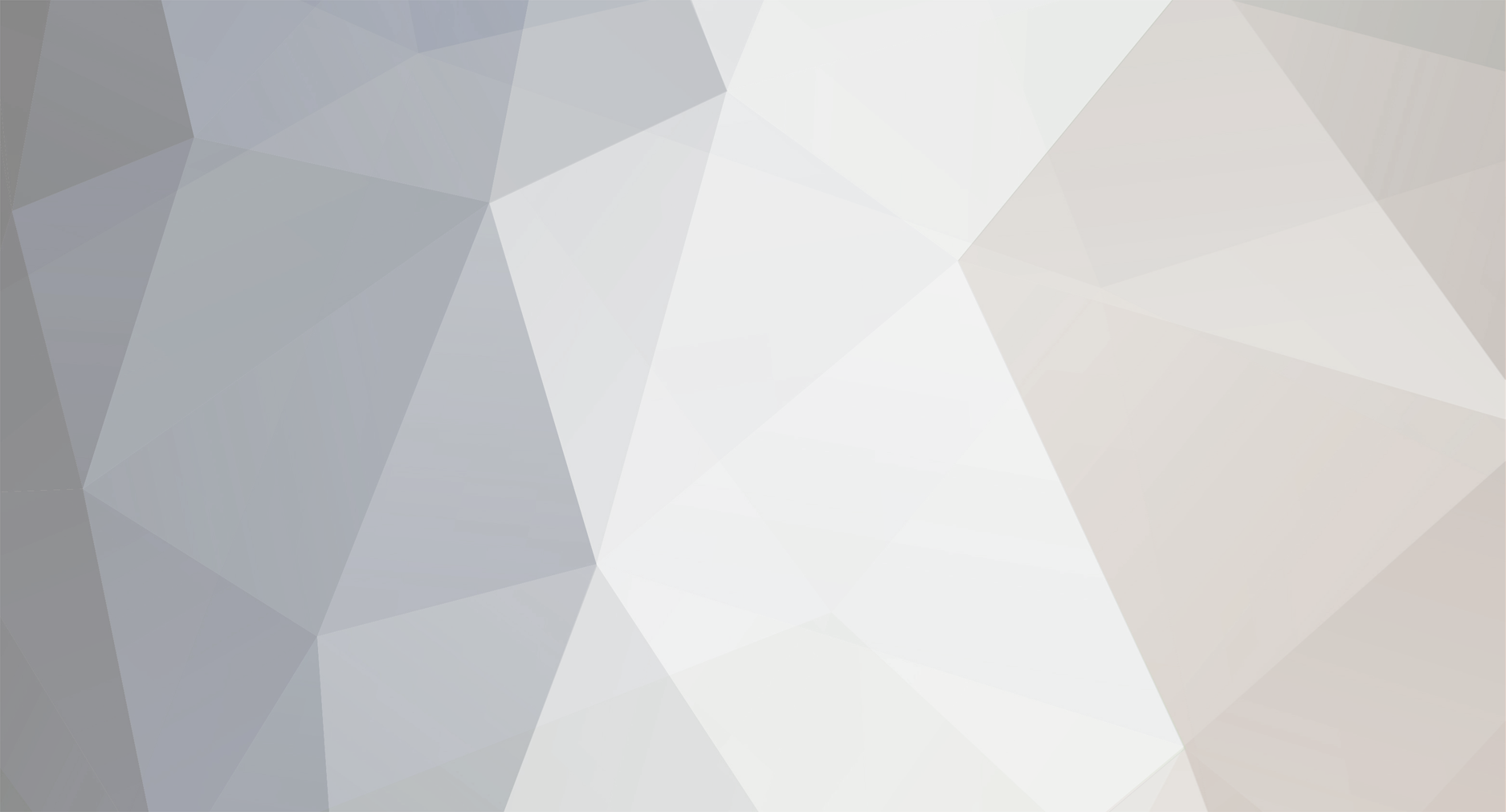
Daniel0
Staff Alumni-
Posts
11,885 -
Joined
-
Last visited
Everything posted by Daniel0
-
In your httpd.conf file or in a file called ".htaccess" in the document root (if you've got AllowOverride on).
-
You can wait a billion years, but nothing will happen. You just placed an index.html file with the following contents: <HTML> <HEAD><META HTTP-EQUIV=Refresh CONTENT="0; url=http://brendansite1.startlogic.com/oddnerdrum/index.php"> </HEAD> </HTML> You'll need to actually send an HTTP 301 response code (that means "Moved Permanently") and a proper Location HTTP header. Using Apache mod_rewrite, something like this would do it: RewriteEngine on RewriteRule (.*) http://brendansite1.startlogic.com/oddnerdrum/$1 [R=301,L] Otherwise Google won't care about you. It'll just index an empty page on the oddnerdrum.info domain. Why exactly don't you use the domain name you've purchased though?
-
You can't. Arrays are just short hand notation for pointers. When you pass an array, you are passing the memory address of the first element. Say you have an array of 10 chars for instance. One char takes up exactly 1 byte, so an array of 10 chars take up 10 bytes. These are all stored sequentially in memory, so when you initialize a char array of size 10, you are allocating 10 byte somewhere in memory. This means the following things are equivalent for your char array: arr[0] arr* or: (arr + 0)* arr[1] (arr+1)* arr[2] (arr+2)* arr[n] (arr+n)* etc. So even though the highest index in this array is only 9, doing arr[1000] is valid because (arr+1000)* is valid even though it's out of bounds and you might really be reading anything. This is also why array indices start from 0 in case you ever wondered. The solution is to pass the size of your array as an extra argument to the function. So, something like char *argv[] and char **argv (which is often seen as argument in the main function) are entirely equivalent. This is also the reason why you have the argc argument: you don't know how long argv is otherwise!
-
Heh... that's what you get from blindly copy/pasting things without trying to understand it first I guess.
-
It does anything if it's an illegal filetype...
-
Replace or/xor with and...
-
When you're having trouble with propositional logic, it's often a good idea making a truth table If we say that: p: $uploaded_type=="image/jpg" q: $uploaded_type=="image/jpeg" Then the truth table looks like this: [pre]p | q | ~p ⊕ ~q --------------- T | T | F T | F | T F | T | T F | F | F[/pre]
-
This is a bit shorter: ^[0-9]*?(??:[13579][26]|(?(?<=^)[02468]?|[02468])[048])00|(?:[13579][26]|(?:[2468][048]|[02468][48]|(?<=^)[048])))$ Now I'm trying to get the >= 1582 into it. Edit: Okay... I can't be bothered to do that right now. I was thinking about either making something that matches numbers less than and then put it on the back with a negative lookbehind or creating one that matches greater than or equal with a positive lookbehind.
-
if (many_badged_members == CV){ $sarcasm = true; } else { $sarcasm = 'limited'; // which also happens to evaluate to true, doesn't it? ~ } Notice: Use of undefined constant many_badged_members - assumed 'many_badged_members' in /home/daniel/test3.php on line 2 Notice: Use of undefined constant CV - assumed 'CV' in /home/daniel/test3.php on line 2
-
Hmm... maybe you're not as 1337 as I am. I had invites from the start.
-
Oh hell yeah: ^0$|^[0-9]*?(?:[13579][26]|(?(?<=^)[02468]?|[02468])[048])00$|^[0-9]*?(?:[13579][26]|(?:[2468][048]|[02468][48]|(?<=^)[048]))$ I rule!!1one!1
-
Just use the "Invite others to Google Wave" wave in your inbox to do it.
-
Disregarding that talking about leap years isn't applicable before 1582, this is what I came up with for matching leap years only: ^[0-9]*?(?:[02468]?[048]|[13579][26])(?:00)?$ I think it should work. Edit: Nevermind. It doesn't work. Crap. Edit 2: Okay, so this regex will match all natural numbers where mod 400 = 0: ^0$|^[0-9]*?(?:[13579][26]|(?(?<=^)[02468]?|[02468])[048])00$ To be continued... Edit 3: And I think this should match all natural numbers n where n mod 100 != 0 and n mod 4 == 0: ^[0-9]*?(?:[13579][26]|(?:[2468][048]|(?<=^)[048]))$ Edit 4: The above one doesn't work. This one almost works, but it matches all the hundreds which it isn't allowed to do: ^[0-9]*?(?:[13579][26]|(?:[02468][048]|(?<=^)[048]))$
-
Then why not use existing IDEs such as NetBeans (free) or Zend Studio (not free) that already have that?
-
That is against the IMDB terms of service. Locked. If you have one of their licenses to use their information, I'm sure they would have told you how to get the content.
-
The way a for loop works is fairly simple actually. It's always of this form: for (startStatement; condition; iterationStatement) { body } So when you start the for loop, the startStatement will be executed. Each time you do something in the loop is called an iteration and at the end of each iteration the iterationStatement will be executed. A new iteration will start as long as the condition evaluates to true. During an iteration, the body will be executed. So doing something like this: for ($i = 0; $i < 2; ++$i) { echo $i; } First you'll set $i = 0 and because 0 < 2 is true, we'll execute the body and print 0. Then we'll increment $i so now it holds the value 1. Because 1 < 2 is true, we'll execute the body again and so 1 is printed to the screen. Then $i is incremented again so it will hold the value 2. However, 2 < 2 is false, so the loop will terminate.
-
Unless they added it since the initial release, it doesn't have IE6's rendering engine.
-
[SOLVED] Calculating the sum of an HTML column
Daniel0 replied to Maracles's topic in PHP Coding Help
True, but I just wanted to point out that there were smarter ways of doing the same thing to all elements in an array than writing a million lines of repetitive code. -
[SOLVED] Calculating the sum of an HTML column
Daniel0 replied to Maracles's topic in PHP Coding Help
array_map() just applies a function to every element in an array and returns the result. -
[SOLVED] Calculating the sum of an HTML column
Daniel0 replied to Maracles's topic in PHP Coding Help
That entire monster block of code can be summarized into this: $daygross = array_values(array_map('stripslashes', $row)); And if it's important to you that your array indices are 1-indexed (which is odd, by the way) you could do this: $daygross = array_combine(range(1, count($row)), array_values(array_map('stripslashes', $row))); You should turn off magic quotes though. Then you won't need to stripslash() everything. -
http://www.phpfreaks.com/forums/index.php/topic,275775.msg1304066.html#msg1304066 Have a look at that. It's not the same, but conceptually it's more or less the same thing. By the way, how did you get to 24 combinations? Mathematically speaking there is only one combination if you use all of them, so I'll assume you mean permutations. Even then there are far more than 24 permutations when selecting 5 elements out of 5 elements. If you don't have any repeats there will be 5!=120 permutations and with repeats there will be 55=3125 permutations. How do you define "combinations"?
-
Ask, and thou shalt receive (granted, depending on your locale, this might not be an issue at all). http://www.phpfreaks.com/forums/index.php/topic,252010.msg1183648.html#msg1183648 Well, I never actually tested it and I've never needed it, but I looked it up in Mastering Regular Expressions: After researching a bit more:
-
Not in PCRE. There \w is always equivalent to [a-zA-Z0-9_] regardless of locale.
-
How would you write a regular expression that checks if a year is a leap year? It's a leap year if year mod 100 != 0 and year mod 4 = 0 or year mod 400 = 0. Then you also have to consider that leap years weren't invented up until the late 16th century (can't remember the exact year). Even if you could somehow craft a regex that would do that it would still be ridiculous and this would be way faster: $isLeap = ($year % 4 == 0 && $year % 100 != 0 || $year % 400 == 0) && $year >= 1582; Edit: So we started with this leap year business in 1582. Updated code.