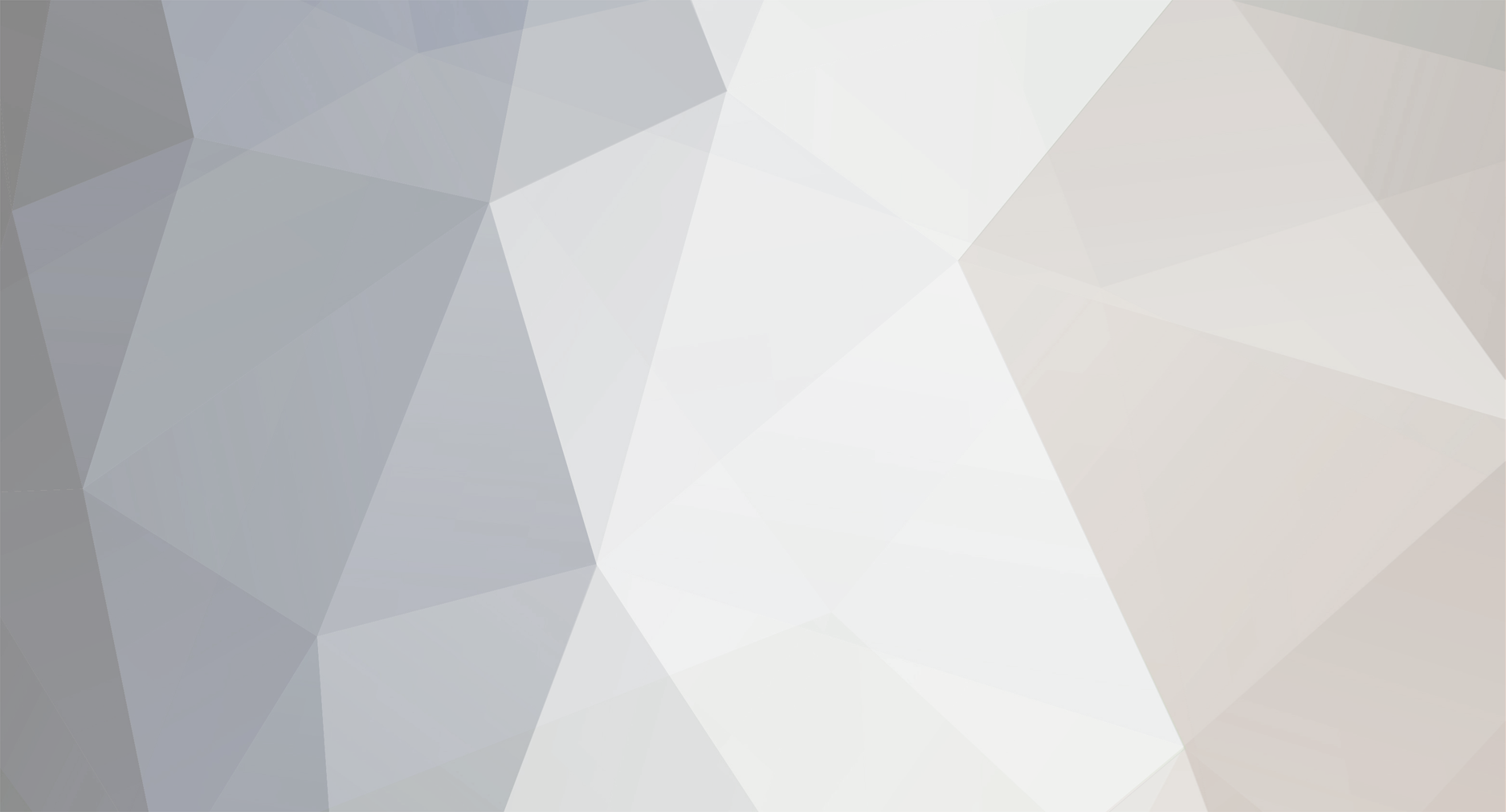
HuggieBear
Members-
Posts
1,899 -
Joined
-
Last visited
Everything posted by HuggieBear
-
The queries are going to need changing a little I'm sure, but I just wanted a basic example to show you the principle of the way it could work using a simple structure. Regards Huggie
-
Yes, it's actually quite simple. 1. User goes to login.php and provides his username and password in a form. 2. Submitting the form posts back to itself and connects to the database to retrieve details, use something like this: [code] <?php // Start session session_start() // Connect to the database include_once('connect.php') // Execute the query $sql = "SELECT id FROM users WHERE username = '{$_POST['username']}' AND password = '$_POST['password']'"; $result = mysql_query($sql); if (!$result){ // If query didn't execute echo "Unable to execute:<br>\n$sql<br>\n" . mysql_error(); } else { if (mysql_num_rows($result) == 1){ // Assign the unique ID to a session variable $_SESSION['id'] = mysql_result($result, 0); echo "You have been authenticated\n"; } else { echo "Unable to authenticate you\n"; } } ?> [/code] This should authenticate you if you exist in the database and now has your unique id stored in a session variable for use when editing. 3. User goes to profile.php which has the following code... [code] <?php // Start session session_start(); // Connect to the database include_once('connect.php'); // Execute the query $sql = "SELECT * FROM users WHERE id = '{$_SESSION['id']}'"; $result = mysql_query($sql); if (!$result){ // If query didn't execute echo "Unable to execute:<br>\n$sql<br>\n" . mysql_error(); } else { if (mysql_num_rows($result) == 1){ $row = mysql_fetch_array($result, MYSQL_ASSOC); // Echo the form here with the default values like so echo "<input type=\"text\" name=\"firstname\" value=\"{$row['firstname']}\">"; } else { echo "Unable to retrieve your profile\n"; } } ?> [/code] This should be enough to get you started and on track. Regards Huggie
-
Remove this condition [code=php:0]if (!isset($_SESSION)) { session_start(); } [/code] As any page that uses session variables must output it. Regards Huggie
-
Need help with adding a value to a stored value in database
HuggieBear replied to Varma69's topic in PHP Coding Help
Try this, and please use code tags [b][nobbc][code] [/code][/nobbc][/b] when posting your code... [code] <?php // Select and execute the query $query = "SELECT * FROM Class Where ClassId = '$ClassId'"; $result = mssql_query($query, $db) or die ("Error with Query:<br>\n$query<br>\n"); $row = mssql_fetch_array($result); // Increase the count by 1 $row['NoEnrolled']++; // Update the database with the new value $query = "UPDATE Class SET NoEnrolled = '{$row['NoEnrolled']}' WHERE ClassId='$ClassId'"; $result = mssql_query($query) or die ("Error with Query:<br>\n$query<br>\n"); ?> [/code] Regards Huggie -
$_SERVER['PHP_SELF'] and validation & redirection
HuggieBear replied to rbragg's topic in PHP Coding Help
ok, for ease of use and speed in the example, I used this code: [code=php:0]foreach ($_POST as $key => $value){ $_SESSION[$key] = $value; }[/code] Without the condition that would actually have given me 4 session variables, so when I echoed them all on the display page using a foreach loop: [code=php:0]echo "The following details were added to our records:<br>\n"; foreach ($_SESSION as $key => $value){ echo "$key: $value<br>\n"; }[/code] I'd have got: [pre]firstname: richard lastname: jones username: huggybear submit: submit[/pre] So this line [code=php:0]if ($key != "submit")[/code] says, if my $key is not equal (!=) to submit (The name of my button) then carry out the selected action, which in our case was to add the value to a session variable. So by adding that line in, I didn't actually create a session valiable called submit. I hope this makes sense. Regards Huggie -
$_SERVER['PHP_SELF'] and validation & redirection
HuggieBear replied to rbragg's topic in PHP Coding Help
No it doesn't, so here's an example... page1.php [code]<?php // Start the session session_start(); // If the form's been submitted if (isset($_POST['submit'])){ // Put the values into session variables foreach ($_POST as $key => $value){ if ($key != "submit"){ $_SESSION[$key] = $value; } } // Include your validate code include('validate.php'); // This is just an example as I don't know how you're validating, but the header() part is the important part if ($validated == "yes"){ header("Location: page2.php"); } } // Echo the form echo <<<FORM <form name="register" action="{$_SERVER['PHP_SELF']}" method="post"> <input type="text" name="firstname" value="{$_SESSION['firstname']}">First Name<br> <input type="text" name="lastname" value="{$_SESSION['lastname']}">Last Name<br> <input type="text" name="username" value="{$_SESSION['username']}">Username<br> <input type="submit" name="submit" value="submit"> </form> FORM; ?> [/code] page2.php [code] <?php // Start the session session_start(); // Echo the code echo "Welcome {$_SESSION['firstname']}<br><br>\n"; echo "The following details were added to our records:<br>\n"; foreach ($_SESSION as $key => $value){ echo "$key: $value<br>\n"; } ?> [/code] I hope this helps. Regards Huggie -
No trouble... 1. Open phpMyAdmin 2. Click on your database name on the left hand side (above all the table names). 3. On the right hand side, click 'Export' 4. Select the tables to export in the list by using 'Ctrl' + clicking the ones you want 5. Put a dot in the box called SQL under the table list 6. Tick 'Structure', 'Add AUTO_INCREMENT value', 'Enclose table and field names with backquotes' and 'Data'. 7. Select 'INSERTS' in the drop down box. 8. Click 'Go' Then paste the output in the forum here. Don't forget to delete any sensitive data from it, if there is any. Regards Huggie
-
That's not correct. If you have an auto incrementing ID then the highest one will be the most recent. I'd use the following code to get the data... [code] <?php include("MySQL_Info.php"); // Query selects the last user added to the database and only returns one row $sql = "SELECT * FROM users ORDER BY id DESC LIMIT 1"; // I've changed $mysql_result to $result as there's a function called mysql_result() wouldn't want it getting confusing $result = mysql_query($sql) or die ("Couldn't execute:<br>\n$sql<br>\n". mysql_error()); $row = mysql_fetch_array($result, MYSQL_ASSOC)); //echo the code echo "<center><font color='#C0C0C0'>-Newest User-</font><br /><a href='View_Profile.php?Username={$row['Username']}'>{$row['Username']}</a><br /><font color='Black'>$Title</font></center>"; ?> [/code] Regards Huggie
-
$_SERVER['PHP_SELF'] and validation & redirection
HuggieBear replied to rbragg's topic in PHP Coding Help
I'm assuming you have a logical page progression like this... I go to page 1, I fill in the form, I hit submit, it submits to itself to validate information, assuming that the information is valid it sends me to page 2 with my details displayed, if not, it shows me page 1 again with the erros on it? Is this correct, if not, can you provide me with the logical flow? Regards Huggie -
If you're using phpMyAdmin you can use that, it's a file that you can run to add data into a database, it would allow me to create the tables and insert the data that you have in order for me to be able to test your code. A dump looks like this: [code] -- phpMyAdmin SQL Dump -- version 2.6.1 -- http://www.phpmyadmin.net -- -- Host: ***.***.***.*** -- Generation Time: Oct 19, 2006 at 07:55 PM -- Server version: 4.1.19 -- PHP Version: 4.3.2 -- -- Database: `osCom` -- -- -------------------------------------------------------- -- -- Table structure for table `banners` -- CREATE TABLE `banners` ( `banners_id` int(11) NOT NULL auto_increment, `banners_title` varchar(64) NOT NULL default '', `banners_url` varchar(255) NOT NULL default '', `banners_image` varchar(64) NOT NULL default '', `banners_group` varchar(10) NOT NULL default '', `banners_html_text` text, `expires_impressions` int(7) default '0', `expires_date` datetime default NULL, `date_scheduled` datetime default NULL, `date_added` datetime NOT NULL default '0000-00-00 00:00:00', `date_status_change` datetime default NULL, `status` int(1) NOT NULL default '1', PRIMARY KEY (`banners_id`) ) ENGINE=MyISAM DEFAULT CHARSET=latin1 AUTO_INCREMENT=2 ; -- -- Dumping data for table `banners` -- INSERT INTO `banners` VALUES (1, 'osCommerce', 'http://www.oscommerce.com', 'banners/oscommerce.gif', '468x50', '', 0, NULL, NULL, '2006-08-29 20:23:40', NULL, 1); -- -------------------------------------------------------- -- -- Table structure for table `banners_history` -- CREATE TABLE `banners_history` ( `banners_history_id` int(11) NOT NULL auto_increment, `banners_id` int(11) NOT NULL default '0', `banners_shown` int(5) NOT NULL default '0', `banners_clicked` int(5) NOT NULL default '0', `banners_history_date` datetime NOT NULL default '0000-00-00 00:00:00', PRIMARY KEY (`banners_history_id`) ) ENGINE=MyISAM DEFAULT CHARSET=latin1 AUTO_INCREMENT=8 ; -- -- Dumping data for table `banners_history` -- INSERT INTO `banners_history` VALUES (1, 1, 146, 0, '2006-08-29 20:24:09'); INSERT INTO `banners_history` VALUES (2, 1, 18, 0, '2006-09-06 11:51:41'); INSERT INTO `banners_history` VALUES (3, 1, 12, 0, '2006-09-12 00:47:56'); INSERT INTO `banners_history` VALUES (4, 1, 1, 0, '2006-09-15 23:52:14'); INSERT INTO `banners_history` VALUES (5, 1, 5, 0, '2006-09-16 00:00:55'); INSERT INTO `banners_history` VALUES (6, 1, 13, 0, '2006-09-28 16:32:29'); INSERT INTO `banners_history` VALUES (7, 1, 18, 0, '2006-10-01 11:30:47'); [/code] Regards Huggie
-
$_SERVER['PHP_SELF'] and validation & redirection
HuggieBear replied to rbragg's topic in PHP Coding Help
Your echoing the wrong thing. Echo the $_SESSION variables, not the $_POST variables and don't forget session_start() at the top of the page. Regards Huggie -
[quote author=Vatik link=topic=111941.msg453965#msg453965 date=1161201431] on my mysql table i have a column called User_level [/quote] Don't forget case-sensitivity for table names, so thorpe's code needs to have this: [code=php:0]if ($row['User_level'] == 2) [/code] not [code=php:0]if ($row['user_level'] == 2) [/code] Regards Huggie
-
Yes, it can be done using [url=http://uk.php.net/manual/en/ref.curl.php]CURL[/url] Regards Huggie
-
$_SERVER['PHP_SELF'] and validation & redirection
HuggieBear replied to rbragg's topic in PHP Coding Help
OK, post the code for page2... Regards Huggie -
Creating different pages for database values?
HuggieBear replied to Solarpitch's topic in PHP Coding Help
What you're talking about is pagination and there's a tutorial on this site in the [url=http://www.phpfreaks.com/tutorials/73/0.php]tutorials[/url] section. Regards Huggie -
I see no reference anywhere to $_SESSION variables of any kind. Regards Huggie
-
$_SERVER['PHP_SELF'] and validation & redirection
HuggieBear replied to rbragg's topic in PHP Coding Help
So is it all working now then? Regards Huggie -
$_SERVER['PHP_SELF'] and validation & redirection
HuggieBear replied to rbragg's topic in PHP Coding Help
Should be [code=php:0]$_SERVER['PHP_SELF'][/code] not [code=php:0]$_SESSION['PHP_SELF'][/code] by the way. The following code will assign all your form values to session variables, and then echo them to check they're being stored correctly, give it a try. [code]<?php // Start the session session_start(); // Loop through foreach ($_REQUEST as $key => $value){ $_SESSION[$key] = $value; echo "{$_SESSION[$key]}<br>\n"; } ?>[/code] Regards Huggie -
Give this a try: [code]<?php include('header1.tpl'); $email = $_POST['email']; // open connection to MySQL server $connection = mysql_pconnect('localhost', '', '') or die ('Unable to connect!'); // select database for use mysql_select_db('db') or die ('Unable to select database!'); // Run the query and get the result $query = "INSERT INTO newsletter(email) VALUES ('$email')"; $result = mysql_query($query) or die ("Unable to run the following query:<br>\n$query<br>\n" . mysql_error()); if ($result){ echo'<br/>'; echo'<center>'; echo 'Thank you for signing up for smeresources newsletter'; echo'</center>'; } else { echo'<br/>'; echo'<center>'; echo 'You cant be Registered,try again later'; echo'</center>'; } ?>[/code]
-
mail() sends one email but not the other...why?
HuggieBear replied to simcoweb's topic in PHP Coding Help
In that case it is a delivery issue, and the problem's with your host, not your script. Regards Huggie -
Fastest way to set loads of variables to null?
HuggieBear replied to Perad's topic in PHP Coding Help
How about a function? [code]<?php $user_id = set_to_null($userdata['user_id']); $f_name = set_to_null($_POST['first_name']); $l_name = set_to_null($_POST['last_name']); $gfx = set_to_null($_POST['hw_gfx']); $mb = set_to_null($_POST['hw_mb']); $pro = set_to_null($_POST['hw_pro']); $mem = set_to_null($_POST['hw_mem']); $mouse = set_to_null($_POST['hw_mouse']); $fgame = set_to_null($_POST['fav_game']); $ffilm = set_to_null($_POST['fav_film']); $fbook = set_to_null($_POST['fav_book']); $notes = set_to_null($_POST['notes']); function set_to_null($var){ if (empty($var)){ $var = NULL; } return $var; } ?>[/code] -
mail() sends one email but not the other...why?
HuggieBear replied to simcoweb's topic in PHP Coding Help
OK, so if you received it in your hotmail account, change both email addresses to your Hotmail account and see if both emails turn up. Regards Huggie -
Version 4 what? Version 4.1.0, or 4.3.2 or something else? Regards Huggie
-
You're not submitting the form! Try this: [code] <form method="post" action="test.php" enctype="multipart/form-data"> <select name="about"> <option selected="selected">General</option> <option>Lures</option> <option>Sales</option> <option>Other</option> </select> <input type="submit" name="submit" value="submit"> </form> <?php $selected = $_POST['about']; echo $selected; ?> [/code] Regards Huggie
-
mail() sends one email but not the other...why?
HuggieBear replied to simcoweb's topic in PHP Coding Help
ok, can you try something to humour me then... Can you change your database so that both the email addresses are the same, make the sending member's email address and the receiving member's email address the same as the one that successfully received the email, then see if they both turn up. This way, you'll know if it's the code or a mail delivery issue. Regards Huggie