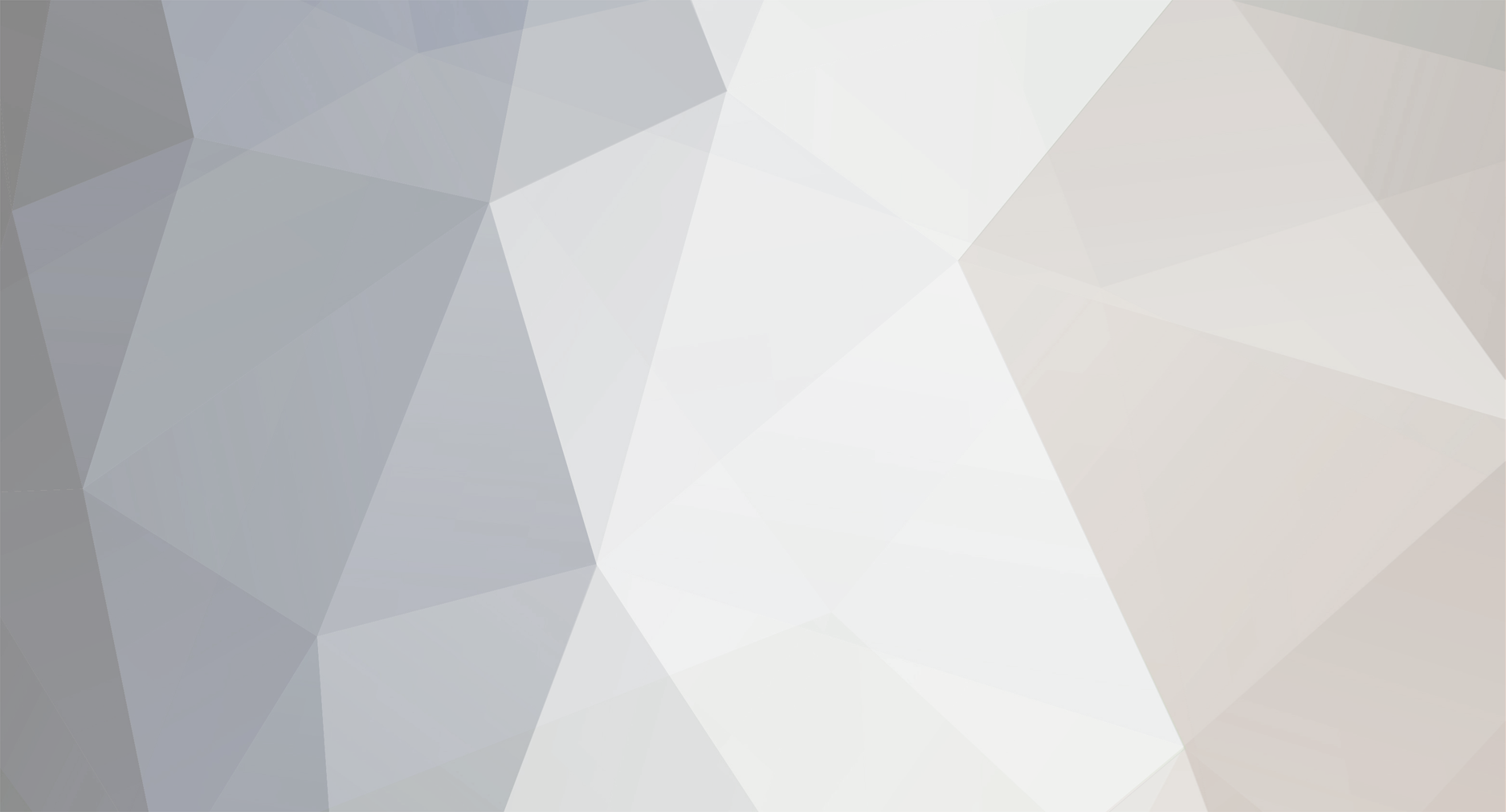
HuggieBear
Members-
Posts
1,899 -
Joined
-
Last visited
Everything posted by HuggieBear
-
redirecting a web page along with session info
HuggieBear replied to garry27's topic in PHP Coding Help
If the variable is set in the session then there's no need to do anything. Redirect using the header() function. [code] <?php if ($username == $validated){ // if the user is validated then redirect using header header("Location: redirect.php"); } ?> [/code] Assuming your user is saved in a session variable then you should be able to access like so... [code=php:0]echo $_SESSION['username'];[/code] assuming that your variable is called [color=green]username[/color] -
You need to query the database first. Try this: [code] <?php mysql_connect('10.0.35.4','testuser','test'); mysql_select_db('helpdesk'); $sql="SELECT * FROM login"; $result = mysql_query($sql); // This was what you were missing $row = mysql_fetch_array($result) [/code] Regards Huggie
-
In that case, just use the following... [code] <?php $username = $_SERVER['PHP_AUTH_USER']; // Notice the single quotes echo "Welcome {$username}<br>\n"; ?> [/code] Regards Huggie
-
getting error when trying to get row count for pagination
HuggieBear replied to simcoweb's topic in PHP Coding Help
[quote author=simcoweb link=topic=111171.msg453290#msg453290 date=1161111168] Acutally the category id IS being passed in the URL as you can see in my example links: [url=http://www.plateauprofessionals.com/category-page2a.php?categoryid=4]http://www.plateauprofessionals.com/category-page2a.php?categoryid=4[/url] [/quote] Actually it's NOT!!!... Although I appreciate that you may have included your category id in the link that you included in your post to the forum, you have not included it in your code... Your code has this... [code] echo "<a class=\"body\" href=\"".$_SERVER['PHP_SELF']."?page=$i\">Page $i</a> "; [/code] Your code should look something like this... [code] echo "<a class=\"body\" href=\"".$_SERVER['PHP_SELF']."?categoryid=$cat_id&page=$i\">Page $i</a> "; [/code] Although I'm extremely pissed, I've had a go at changing the code you pasted, so change what you pasted to: [code] include 'dbconfig.php'; include 'header.php'; //connect to db mysql_connect($dbhost, $dbuser, $dbpass) or die(mysql_error()); mysql_select_db($dbname) or die(mysql_error()); // Get category ID or set default $cat_id = is_numeric($_GET['categoryid']) ? $_GET['categoryid'] : 0; // Double check for a valid value // Get page ID or set default $page = isset($_GET['page']) ? $page = $_GET['page'] : $page = 1; // Define the number of results per page $max_results = 5; // Figure out the limit for the query based on the current page number. $from = (($page * $max_results) - $max_results); // Figure out the total number of results in DB: $sql = "SELECT COUNT(*) FROM members_cat WHERE categoryid='$cat_id'"; $result = mysql_query($sql); $total_results = mysql_num_results($result); // Figure out the total number of pages. Always round up using ceil() $total_pages = ceil($total_results / $max_results); // Grab data from plateau_pros, where the member has the specified category id $sql = "SELECT p.* FROM members_cat c, plateau_pros p WHERE c.categoryid='$cat_id' AND c.memberid=p.memberid LIMIT $from, $max_results"; $results = mysql_query($sql) or die(mysql_error()); $num_rows = mysql_num_rows($results); echo "<font class='bodytext'>There are <b>$num_rows</b> professionals in this category</font><br><br>\n"; // Build Page Number Hyperlinks echo "<center>Select a Page<br />"; for($i = 1; $i <= $total_pages; $i++){ echo " | "; if(($page) == $i){ echo "Page $i "; } else { echo "<a class=\"body\" href=\"".$_SERVER['PHP_SELF']."?categoryid=$cat_id&page=$i\">Page $i</a> "; } } echo "</center>"; if (empty($results)) { echo "No profiles found in this category."; } else { while ($a_row= mysql_fetch_array($results)){ [/code] Let me know if you get an error. Regards Huggie -
Stick the form code inside the 'else' part of the 'if' statement... [code] <form name="myform" method="post" action="<?=$_SERVER['PHP_SELF']?>"> <div class="home_text"> <?php if ($sMode == 'complete') { echo '<div class="complete"><div class="formwrapper">Thank You for submitting your details.</div>'; } else { ?> <? if(is_array($aErrors) && count($aErrors) > 0) { ?> <ul><div class="formwrapper"> <? foreach($aErrors as $sError) { echo '<li>'.$sError.'<br></li>'; } // end foreach ?><br /></ul><? } else { ?> <div class="formwrapper"> <div class="textlabel">Name*</div><div class="formlabel"> <input name="Name" type="text" class="dataform" value="<?=(stripslashes($_POST['Name']))?>" /> </div> <div class="textlabel">Email*</div> <div class="formlabel"> <input name="Email" type="text" class="dataform" value="<?=(stripslashes($_POST['Email']))?>"/> </div> <div class="textlabel">Address*</div> <div class="formlabel"> <textarea name="Address" rows="2" wrap="virtual" class="dataform"><?=(stripslashes($_POST['Address']))?></textarea> </div> <div class="textlabel">Business Name*</div> <div class="formlabel"> <input name="Business_Name" type="text" class="dataform" value="<?=(stripslashes($_POST['Business_Name']))?>"/> </div> <div class="textlabel">Type of Business*</div> <div class="formlabel"> <input name="Type_of_Business" type="text" class="dataform" value="<?=(stripslashes($_POST['Type_of_Business']))?>"/> </div> <div class="textlabel">Comments</div> <div class="formlabel"> <textarea name="Comments" rows="2" wrap="virtual" class="dataform"><?=(stripslashes($_POST['Comments']))?></textarea> </div> <div class="textlabel"> </div><div class="formlabel"> <input name="Submit" type="submit" class="databut" value="Submit" /> <input type="hidden" name="mode" value="apply" /> <br class="clear"/> </form> <?php } ?> [/code] Regards Huggie
-
My advice would be to do this... 1. Create a serverparams.php page that looks like this: [code] <?php foreach ($_SERVER as $k => $v){ echo "$k - $v<br>\n"; } ?> [/code] 2. Upload that to an area within your secured folder. 3. Open the serverparams.php file in your browser, you'll be asked to login. 4. Check that the PHP_AUTH_USER and REMOTE_USER actually contain values. Let me know the outcome. Regards Huggie
-
On your processing page, just process all of the values like this, that way you have no need to know what the values are: [code] <?php echo "Please order me the following:\n"; foreach ($_POST as $part => $qty){ echo "$part - $qty\n"; } ?> [/code] Regards Huggie
-
getting error when trying to get row count for pagination
HuggieBear replied to simcoweb's topic in PHP Coding Help
OK, you're getting no results as you're not passing the category through the address. Change this at the bottom of the page: [code] echo "<a class=\"body\" href=\"".$_SERVER['PHP_SELF']."?page=$i\">Page $i</a> "; [/code] To this: [code] echo "<a class=\"body\" href=\"".$_SERVER['PHP_SELF']."?categoryid=$cat_id&page=$i\">Page $i</a> "; [/code] You must pass the category id through the URL too if you're using it in your query. Regards Huggie -
Maybe it's the rest of the code... Are you able to provide us with it? Regards Huggie
-
Glad to have been of some assistance... I'll put those Oracle books back to the bottom of the dust pile now ;) Huggie
-
It can be done by using fopen() on the html files and then using preg_match() or preg_match_all() or something similar to extract the data. You'd need to read up on the preg_* functions first. If someone else has a better idea, I'm sure they'll post it soon. Maybe something XML related. Regards Huggie
-
Block and unblock all ports on wireless router
HuggieBear replied to Grega_'s topic in PHP Coding Help
Again, this is functionality provided by most wireless routers already. I suggest reading the manual thoroughly before posting here. My Linksys Wireless router allows me to block traffic on specific ports, allow certain machines access to the Internet at certain times, monitor network traffic and who's connected and send what when etc. Regards Huggie -
I think that Jamie meant that he wanted to do it in one statement as opposed to two... This can be done in Oracle, I believe it can probably be done in MySQL too. Check this page out: http://dev.mysql.com/doc/refman/4.1/en/insert.html and then this one afterwards: http://dev.mysql.com/doc/refman/4.1/en/insert-select.html Regards Huggie
-
OK, php is probably dealing with the escaping slightly different to Perl which I'm used to... I'll take another look. Regards Huggie
-
passing php variable to javascript **SOLVED**
HuggieBear replied to scottybwoy's topic in PHP Coding Help
Sorry, got bogged down with my own project at the minute :( I'll take a look soon though, I've done something similar before, so it shouldn't be too hard to adapt the code. Huggie -
I didn't realise the single quotes... Try this sorry... [code=php:0]chdir("\\\\PCnameonnetwprk\\thefolder\\"); [/code] or this: [code=php:0]chdir('\\PCnameonnetwprk\thefolder\'); [/code] Regards Huggie
-
Block and unblock all ports on wireless router
HuggieBear replied to Grega_'s topic in PHP Coding Help
I'd suggest starting at the Router Manufacturer's website. Find out some technical information about your router first, like if it's even possible. Most wireless routers run a small webserver (usually built on a linux distribution of somekind) so they can run a web interface for configuration purposes. The likelihood of it running php I'd have thought are probably, minimal, but it's not beyond belief. Also, if you're new to PHP I wouldn't advise embarking on this as your first project. Regards Huggie -
You look like you've started to escape the back slashes, but not correctly for a network path. Try this: [code=php:0]chdir('\\\\PCnameonnetwprk\\thefolder\\');[/code] Regards Huggie
-
I don't think this is going to help anyone, as you haven't actually provided us with any of the functional code. Regards Huggie
-
It's due to password incompatibility.... Check out this link [url=http://www.digitalpeer.com/id/mysql]DigitalPeer[/url] and I hope you have root access! Regards Huggie
-
Try changing the error reporting level... [code]<?php error_reporting(E_ERROR); $v[0]=1; echo $v[2]; ?>[/code] Regards Huggie
-
Toon, Is the exact error: [quote] Could not connect to MySQL: Client does not support authentication protocol requested by server; [/quote] HTH ;) Huggie
-
getting error when trying to get row count for pagination
HuggieBear replied to simcoweb's topic in PHP Coding Help
OK, the link you provided doesn't work I'm afraid. As for the two variables in the SQL query, they're doing what they're meant to. It should only be pulling 5 rows from the database, not 14. Hence the pagination. Please can you post your whole code for that page as it currently stands. Also, if you're able to put the example back on your site that would be helpful too. Regards Huggie -
The reason for this is because everything between single-quotes is interpreted as a literal string, and not interpolated by php as it would be if it were enclosed in double-quotes. Regards Huggie
-
That code looks good to me... When the form loads, right click and view source and see what's being put in the 'action' parameter. Regards Huggie