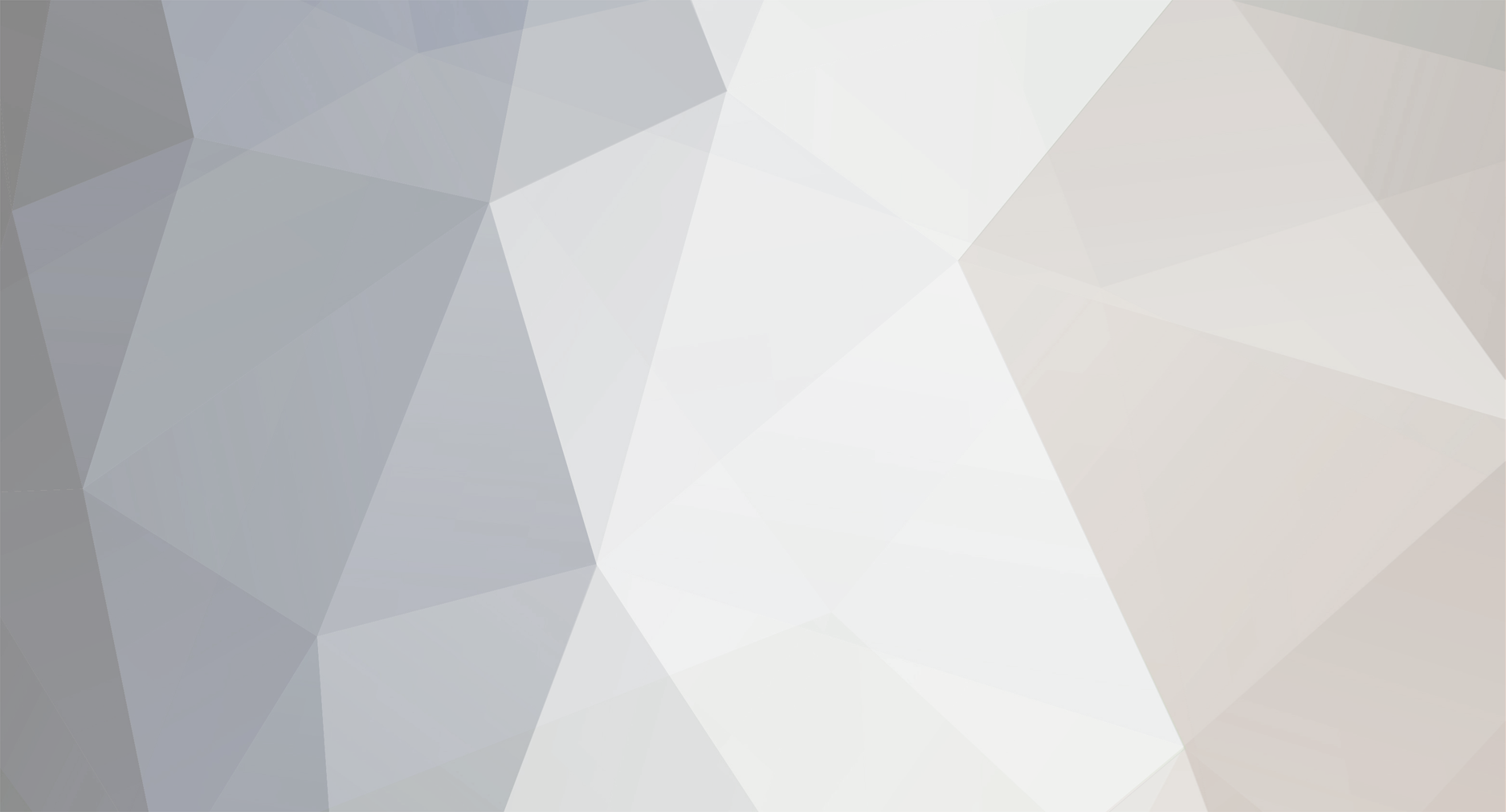
HuggieBear
Members-
Posts
1,899 -
Joined
-
Last visited
Everything posted by HuggieBear
-
Apologies for the delay... Here's the code, with comments, give it a try. [code]<?php // Connect to DB include('connect.php'); // Select total results for pagination $result = mysql_query('SELECT count(countryName) FROM tblCountry'); // This needs to be your query $num_records = mysql_result($result,0,0); // Set maximum number of rows and columns $max_num_rows = 2; $max_num_columns = 2; $per_page = $max_num_columns * $max_num_rows; // Work out how many pages there are going to be $total_pages = ceil($num_records / $per_page); // Get the current page number if (isset($_GET['page'])) $page = $_GET['page']; else $page = 1; // Work out the limit offset $start = ($page - 1) * $per_page; // Select the results we want including limit and offset $result = mysql_query("SELECT countryName FROM tblCountry ORDER BY countryName LIMIT $start, $per_page"); // Your query $num_columns = ceil(mysql_num_rows($result)/$max_num_rows); $num_rows = ceil(mysql_num_rows($result)/$num_columns); // Echo the results echo "<table border=\"2\">\n"; for ($r = 0; $r < $max_num_rows; $r++){ echo "<tr>\n"; for ($c = 0; $c < $max_num_columns; $c++){ $x = $r * $max_num_columns + $c; if ($x < mysql_num_rows($result)){ $y = mysql_result($result, $x, 0); // This line outputs the results from your query } else { $y = "Coming Soon"; // This is what I put in the empty spaces } echo "<td>$y</td>"; } echo "</tr>\n"; } // Echo page numbers echo "</table>\n"; for ($i=1;$i <= $total_pages;$i++) { if ($i == $page) echo " $i "; else echo " <a href=\"?page=$i\">$i</a> "; } ?>[/code] [size=8pt][color=red][b]Note:[/b][/color] If you have a 'where' clause in your main select statement, you should also include it in the pagination sql at the top of the page[/size] Regards Huggie
-
Morning all, I'm getting the following error when trying to create a thumbnail using createimagefromjpeg(): [quote] Fatal error: Allowed memory size of 8,388,608 bytes exhausted (tried to allocate 9216 bytes) [/quote] The file I'm trying to create a thumbnail of is 369,951 bytes in size, does anyone know why I'm getting this error, does it really allocate over 8MB of memory to create a thumbnail? I've tried to free any memory with imagedestroy() but still the same error, any ideas? Regards Huggie [size=8pt][color=red][b]Edit:[/b][/color] I corrected this by adding the following line to the top of the script[/size] [code=php:0]ini_set("memory_limit","12M");[/code]
-
Sorry dude, been busy, I'll get on the case now. Regards Huggie
-
Oh, so you want it to open in a new window... That's not a php issue, it's HTML/Javascript Try googling for [url=http://www.google.co.uk/search?hl=en&q=javascript+%2B%22open+new+window%22&meta=&btnG=Google+Search]javascript +"open new window"[/url] Regards Huggie
-
Writing to a text file instead of database [RESOLVED]
HuggieBear replied to AdRock's topic in PHP Coding Help
Yes, look at the second argument that fopen() accepts. Regards Huggie -
Ooops, I forgot the while loop... [code]<?php $sql = "SHOW FULL COLUMNS FROM table_name"; $result = mysql_query($sql); while ($row = mysql_fetch_array($result, MYSQL_ASSOC)){ echo "$row['Field'] - $row['Comment']<br>\n"; } ?>[/code] I also added the 'field' value so that you get the column name too. Regards Huggie
-
mysql query output not displaying in foreach loop
HuggieBear replied to apheli0n's topic in PHP Coding Help
No problem, assuming the column that holds the track name is called Track_Name then give this a try [code]<?php //Discog query $sqldisog = "SELECT Albums.*, Tracks.* FROM Albums LEFT JOIN Tracks on Albums.Album_Name = Tracks.AlbumName WHERE ArtistName = '$aName' ORDER BY Albums.Album_Name"; $resultdiscog = mysql_query($sqldisog) or die("Query failed: " . mysql_error()); $album_title = "null"; while ($rowdiscog = mysql_fetch_array($resultdiscog)){ if (is_null($album_title) || strcmp($album_title,$rowdiscog['Album_Name']) !=0 ){ $album_title = $rowdiscog['Album_Name']; // Album header info echo "<br>$album_title - $rowdiscog['Album_Year']<br>\n"; } // Track listing echo "$rowdiscog['Track_Name']<br>\n"; // Change this variable to the name of your column } ?>[/code] Regards Huggie -
Give this a try: [code]SELECT m.id, m.name, m.email, m.joined, m.bday_day, m.bday_month, m.bday_year, i.icq_number, c.field_1, c.field_2, c.field_3, c.field_4 FROM ibf_members m, ibf_member_extra i, ibf_pfields_content c WHERE m.id = i.id AND i.id = c.id AND m.mgroup = 4 ORDER BY m.id[/code] Echo all the results to see if it's what you expect, then go about your formatting. Regards Huggie [size=8pt][color=red][b]Note:[/b][/color] Barand beat me to it, and I'm not even sure mine would work, but they look pretty similar :)[/size]
-
You my friend, are in need of AJAX. This will allow you to update the content on the page without reloading it. I'd also recommend having your select list created dynamically too, this way, you can add additional currencies to the database without having to amend the php pages at all. I'll try to post an example for you (using your code) when I get home from work this evening. Regards Huggie
-
mysql query output not displaying in foreach loop
HuggieBear replied to apheli0n's topic in PHP Coding Help
Try changing this: [code] while ($rowdiscog = mysql_fetch_array($resultdiscog)) $category[$rowdiscog["Album_Name"]][] = $rowdiscog["title"]; //album header info foreach ($category as $cat=>$title_array) { echo("<br/><b>$cat</b> ($resultdiscog[Album_Year]) <br/>"); echo("Label link <br/>"); //album tracklist foreach ($title_array as $title) echo("$rowdiscog[TrackOrder]) asdf<br>"); } [/code] To this: [code] while ($rowdiscog = mysql_fetch_array($resultdiscog)){ $category[$rowdiscog['Album_Name']] = $rowdiscog['title']; //album header info foreach ($category as $cat => $title_array){ echo "<br/><b>$cat</b> {$resultdiscog['Album_Year']} <br/>"; echo "Label link <br/>"; //album tracklist foreach ($title_array as $title){ echo "$rowdiscog['TrackOrder'])asdf<br>"; } } } [/code] All I've done is tidy up your code. I'll post and easier way for you shortly. Regards Huggie -
What do you mean by 'into javascript page' do you have a working example on a site somewhere? Where did the idea come from? Regards Huggie
-
Here, this should work... [code]<?php $MyTableName = "username"; //Your table name goes here $sql = "SHOW COLUMNS FROM table_name WHERE Field = '$MyTableName'"; $result = mysql_query($sql); $count = mysql_num_rows($result); if ($count > 0){ echo "The column name already exists"; } else { echo "There is no column with that name"; } ?>[/code] Regards Huggie
-
DESCRIBE doesn't show it... Use SHOW with the 'FULL' keyword... Change table_name for your table [code]<?php $sql = "SHOW FULL COLUMNS FROM table_name"; $result = mysql_query($sql); $row = mysql_fetch_array($result, MYSQL_ASSOC); echo $row['Comment']; ?>[/code] Regards Huggie
-
Are comments a feature of MySQL or phpMyAdmin though? Regards Huggie
-
passing php variable to javascript **SOLVED**
HuggieBear replied to scottybwoy's topic in PHP Coding Help
Perfect, I'll take a look at this a little later. A brief look would indicate that it's not going to be too difficult. Regards Huggie -
shipment calculation problem with orginating and destination zipcodes
HuggieBear replied to josejp's topic in PHP Coding Help
Give this a try: http://www.ups.com/content/us/en/resources/service/hardware/free.html?WT.svl=SubNav Regards Huggie -
OK, I have a huge deal on at work at the moment, but luckily for you, it's almost the weekend :) I'll post the code for you tomorrow, I might even try to integrate some of your code for you. Regards Huggie
-
passing php variable to javascript **SOLVED**
HuggieBear replied to scottybwoy's topic in PHP Coding Help
You provided the link to the .zip file, but as already stated, I'm not a member at codeproject.com If you provide me with the zip file that you downloaded from that site, I'll set it up. Regards Huggie -
As stated, this isn't a php problem, more an html issue. You'd be better off using tables for something like that I'd imagine. Try posting in the HTML forum on this one. Regards Huggie
-
How about showing us the code for the related pages... Regards Huggie
-
Stuart, I've replied to this in your [url=http://www.phpfreaks.com/forums/index.php/topic,111054.0.html]other post[/url]... Please don't double post! Regards Huggie
-
[quote author=GremlinP1R link=topic=111316.msg451029#msg451029 date=1160675212] I use the [color=red]While ($row = $results)[/color] Comand to pull all data from the database. Now about 100 lines down I want to display that data. [/quote] About 100 lines down what? Do you mean you have say 300 rows in your database and you want to display rows 100 to 300 and not 1 to 99? I'm not sure that you mean by that? Regards Huggie
-
[quote author=Barand link=topic=111280.msg451111#msg451111 date=1160684536] Depends on the tools you use. My editor (PHPEd) would display it as $mystring = "the [b]$animal[/b] sat on the mat"; [/quote] My editor (Notepad) isn't that clever I'm afraid ;) Regards Huggie
-
You have a couple of rogue characters there. Remove the '[color=green][b]{[/b][/color]' from the end of the following lines... [code=php:0] $main = trunc($row['Main'], 200);{ $heading = trunc($row['Heading'], 75);{ [/code] Let me know if you still have issues after this :) Regards Huggie
-
passing php variable to javascript **SOLVED**
HuggieBear replied to scottybwoy's topic in PHP Coding Help
Can you attach the zip file you got from the site link that you posted? Regards Huggie