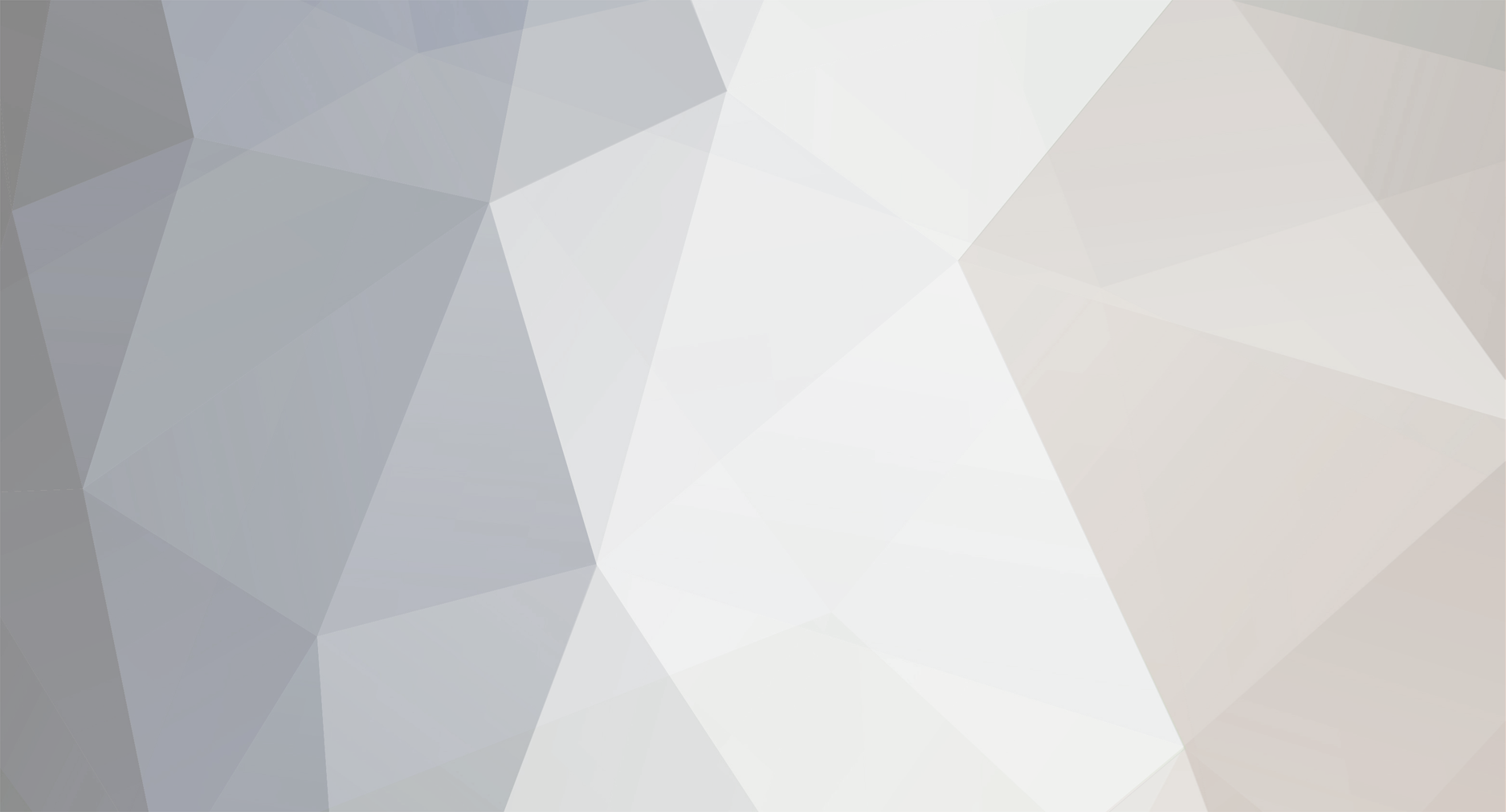
KevinM1
Moderators-
Posts
5,222 -
Joined
-
Last visited
-
Days Won
26
Everything posted by KevinM1
-
You don't need to make it static to do that. Simply override it in the child class: abstract class DB { public function create(array $fields, $tableName, $validFields) { $keys = ""; $values = ""; foreach($fields as $key => $value) { if(in_array("$key", $validFields) && !empty($value)) { $keys .= "$key,"; $values .="'$value',"; } } $keys = rtrim($keys, ","); $values = rtrim($values, ","); $mysql = new Mysql_Wrapper(); return $mysql->query("INSERT INTO $tableName($keys) VALUES($values)"); } } class Cat extends DB { private $tableName = "cats"; public function create(array $fields) { parent::create($fields, $this->tableName, /* whatever $validFields are supposed to be */); } } I could be misreading your question, but it seems like you merely want to invoke the abstract class' method with parameters specific to a child class.
-
You don't need to redefine the method in the child class. Simply make it non-static in the abstract base class. That's the way abstract classes are typically used - non instantiated classes that contain all of the executable base functionality their children can use. This makes the child classes simple to create as one doesn't have to redefine within them what's already present in the parent.
-
Ah, sorry. I don't tend to use inline JavaScript (i.e., JavaScript that's placed inside HTML elements). I prefer an unobtrusive (JavaScript that's separate from the HTML) style as it's easier to maintain, debug, and alows sites to degrade gracefully if the user is viewing it with an incompatible browser. So, my example would apply to a page that looks like: <!DOCTYPE html> <html> <head> <title>Unobtrusive JavaScript Example</title> </head> <body> <form name ="myForm" action="<?php echo $_SERVER['PHP_SELF']; ?>" method="post"> <!-- Inputs go here --> <input type="submit" name="submit" value="submit" /> </form> </body> <script type="text/javascript"/> var submit = document.forms["myForm"].elements["submit"]; submit.onclick = function() { if (/* condition */) { /* Do something */ } else { /* Do other thing */ } } </script> </html>
-
Nope, that kind of thing isn't possible. Event handlers must be functions. What's stopping you from doing someElement.onclick = function() { if (condition) { /* do something */ } else { /* do other thing */ } } ??
-
I'm actually having a similar problem on my Mint machine. Apache 2 is running fine, as HTML works normally. I downloaded and installed both Apache and PHP 5.3.1 through the package program. I can see both php.conf and php.load in my modules-enabled folder. It looks like my httpd.conf is completely empty. At least, nothing shows up in gedit when I try to edit it as root.
-
When using the MYSQL_ASSOC flag, you need to reference column data by their column name rather than column number, i.e.: $row['columnName'] Where 'columnName' is the name of the MySQL table column your data resides in. More info - mysql_fetch_array
-
http://www.microsoft.com/sqlserver/2008/en/us/licensing-faq.aspx
-
Social Media as a Form of Advertising / Reaching out to customers
KevinM1 replied to stublackett's topic in Miscellaneous
I don't think that there are any hard and fast rules one could give you. It depends on what your business is, who your intended audience is, what your competition is doing, etc. I think it would be a wise move to poll your current customer base with these sort of things. Their feedback would be invaluable. Questions like: Would you like to follow us online? Do you prefer e-mail updates, or following people/entities on Facebook and/or Twitter? How often do you use social media sites? etc. -
Me neither. There will always be an open source db available for us to use. So long as it works well, I couldn't care less about what brand it is, whether MySQL, postgreSQL, or anything else.
-
A lot of Windows slowdown can also be attributed to having a bunch of programs running in the background. I recently went through all of my startup programs and stopped all but the essentials from running. I was amazed at the speed increase. I pretty much run with just anti-malware programs running, and it's smooth sailing for me.
-
Well, you could save the server info as part of the site configuration. That would allow you to save only the filename in the db, keeping things a bit simpler on both ends. This could work with multiple storage locations if you save their paths in an array. Loop through them while concatenating the filename to check if the file exists.
-
<!DOCTYPE html> <html> <head> <title>Blah</title> </head> <body> <div id="content0"></div><a id="link0"></a> <div id="content1"></div><a id="link1"></a> </body> <script type="text/javascript"> var divs = document.getElementsByTagName('div'); var links = document.getElementsByTagName('a'); var expandable = new Array(); var toggles = new Array(); for(var i = 0; i < divs.length; ++i) { if (divs[i].id == 'content' + i) { expandable.push(divs[i]); } } for(var j = 0; j < links.length; ++j) { if (links[j] == 'link' + i) { toggles.push(links[j]); } } for(var k = 0; k < toggles.length; ++k) { toggles[k].onclick = function() { if (expandable[k].style.display == 'block') { expandable[k].style.display = 'none'; this.innerHTML = 'Show'; } else { expandable[k].style.display == 'block'; this.innerHTML = 'Hide'; } } </script> </html> Not tested, but something like that should work.
-
It actually doesn't look all that impressive to me. Don't get me wrong, the CG looks awesome, but all the ads make it look a bit clunky when actual people interact with the CG environments and creatures. It's not as bad as the Star Wars prequels in that regard, but still noticeable enough to be jarring. That, and the plot seems to be paint-by-numbers white guilt. Humans are bad, natives are good, etc. Every preview gives me that 'been there, done that' vibe. If it gets good reviews, I may see it in the theater, but I predict it'll only be a rental.
-
Remember: an ID is supposed to be a unique value. Using the same ID on two elements is semantically incorrect. This is why your script only modifies the text of the first link - JavaScript only looks for one instance of that ID because there should only be one.
-
Hey thanks for the reply. I'm not 100% sure what you mean by passing it to a form. If you don't mind explaining that that would be great. Thanks You need to retain the old $partial value when the page reloads. Why is this necessary? Work through your current form: User visits the site, and a CAPTCHA value is created. User submits the form. Page reloads. A new CAPTCHA value is created. The script tests the submitted value against the newly generated value. For all intents and purposes, they can never be the same. So, you need to force your script to remember the old CAPTCHA value for testing purposes. Normally you'd be able to simply stick that value in a hidden form input, but since this is for security purposes, that's a bad idea. Use sessions instead.
-
Can you tell us which specific line is throwing the error?
-
Your if-statement is wrong - = means assignment == means test for equality In your case, your if-statement says "if $row['category_name'] can be assigned to $user, do the following: ..."
-
Around here, a lot of company intranets are written in Java. There's definitely a demand.
-
Remember - an id is supposed to be unique. It doesn't make any sense for multiple elements to have the same id. JavaScript knows this, and will stop at the first instance of the id you tell it to find. You have a couple of options: 1. Give each item that should be toggled its own unique id. 2. Give each group of items that should be toggled (i.e., all 'A' items, all 'B' items, etc.) their own class name. How the script is written will depend on your choice.
-
Or, you could break things up into various files and include them where necessary....
-
No, it's not the only language that can do that. To the OP, I'd suggest Java as well. If your school offers C#, that would be a good second choice. But stay far away from all forms of VB. Its syntax is enough to make the strongest person cry.
-
Just set its display to 'none' in your CSS. If JavaScript is enabled, you can change it to 'block' on page load.
-
FWIW, Adobe AIR stands for Adobe Integrated Runtime. Essentially, it's a runtime that allows Flash apps to be deployed in a desktop environment instead of a web browser. The JavaScript framework most likely works with Flash because ActionScript is a derivative of ECMAScript.
-
Something to keep in mind is that sessions don't persist. If a user clicks on a prayer, logs out of the system, then logs in again sometime later, the session that recorded that click no longer exists, meaning the user can click on the same prayer again. If you want to permanently ban multiple clicks, you'll need to store the info some place permanent, like a db table. Thankfully, the db solution is simple. Create a new table that will contain only a user's id and a prayer's id. Whenever a prayer is clicked, check that table to see if that user already clicked on that prayer. If so, alert the user that they already clicked that prayer. Else, do whatever processing you normally do and add that user-prayer pair to that db table.
-
Interesting article, http://www.computerworld.com/s/article/9141465/Microsoft_s_top_developers_prefer_old_school_coding_methods The 'visual' aspect isn't the same as the 'managed' aspect. The visual aspect is using the design mode of the IDE to do the hard work for you. I, myself, avoid this as it feels more restrictive and time consuming trying to tell the IDE to do what I want to do via diagrams and other graphical interface objects instead of actually writing code myself. Of course, I find that the article overstates the danger of visual learning. Ever been taught how an array works, or a more complex structure like a list or queue? Chances are, you saw a diagram of what the structure conceptually looks like. Of course, no programmer worth their salt would work primarily in design mode. The 'danger' stems from those developers - independent or otherwise - who think they're hot shit because they can point-and-click their way to success. This is no different than those Photoshop pros who think they're developers because they can export their layouts as HTML. These self-deluded people will always exist. The managed portion handles all the little 'gotchas' associated with dynamic memory allocation. Instead of manually needing to free memory obtained from the heap and dance around memory leaks and other problems, the runtime does it for you. This isn't a bad thing - even professional C++ developers often use framework classes that do the same sort of thing. Why? Because memory management is a tedious pain in the ass, and if the grunt work can be taken out of it, so much the better. There's nothing wrong with using tools to make your life easier if you can understand what's going on under the hood. I know what pointers are and how to use them. If I can get identical results without having to manually go through the hoops of memory management, which, as an added benefit, will make my code more readable, then why wouldn't I want to do it that way? I'm wondering if PHP could be considered a managed language or not. Specifically, whether or not its objects are actually created from heap memory, or, since PHP scripts are a one-shot affair, if they're allocated on the stack.