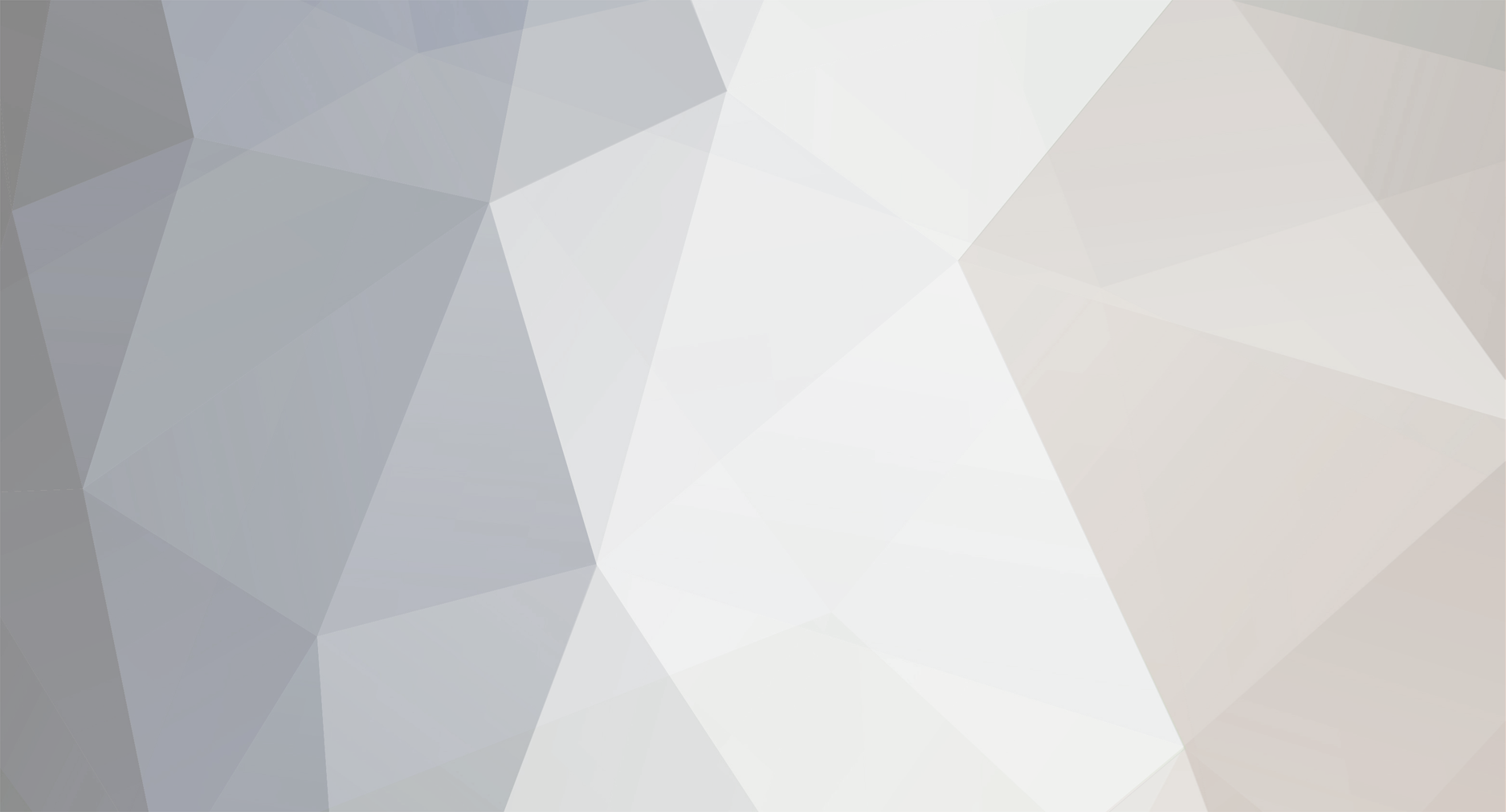
KevinM1
Moderators-
Posts
5,222 -
Joined
-
Last visited
-
Days Won
26
Everything posted by KevinM1
-
Something else to consider is that while learning the ins and outs of a language is useful, having the mindset of essentially memorizing a language's built-in capabilities is the wrong way to go. Memorizing functions won't turn you into a better programmer. Like neil said, learning from a book is a good way to start. They tend to merge language-specific details with generalized programming techniques. I like the various Visual Quickstart Guides as they're friendly to beginners without being completely patronizing.
-
Due to our lack of telepathy, you may want to clarify your problem by showing us some code, especially near where the error is occurring.
-
Instead of using JavaScript to make something 'closed' when the page loads, use CSS and set its display to 'none.' Then, have a JavaScript function tied to some sort of event elsewhere on the page (a button's onclick event, for example) toggle that table's display. Something like: <!DOCTYPE html> <html> <head> <title>Blah</title> <style> #second { display: none; } </style> </head> <body> <table id="second"> <!-- table data --> </table> <button id="toggle">Click to toggle the display of the second table</button> </body> <script type="text/javascript"> var oButton = document.getElementById('toggle'); var oTable = document.getElementById('second'); oButton.onclick = function() { oTable.style.display = (oTable.style.display == 'none') ? '' : 'none'; } </script> </html> If you still insist on tying the JavaScript to the elements directly inside the HTML (which I do not recommend, but that's not really the topic here), then be sure to put quotes around the id you pass into the function.
-
This just made me lol. Good show.
-
Remove the 10% and 15% from the loop? You're practically there, as you test the modulus of 'i' during the loop in order to output the results every 10 years. Simply remove the unneeded information and calculations.
-
?? You can get this result by playing with the rendering option in IE8's web development window. I'm a FF guy myself. Despite the improvements IE has made in the recent years, it still takes forever and a day to start, and just feels clunky, like it's held together with chewing gum and bailing wire. Chrome is nice, but I want better extension/add-on support before considering making the move. I'm also not a fan of its UI. I just think its anglular tabs look ugly and take too much room. I love FF because of how customizable it is. I'm currently rocking a good-looking minimalist UI that keeps my viewing space nice and large. Despite that, information like local weather, just about everything you'd want to know about a site (FireBug), and my Twitter feed are all available to me with a mouseover or single click of a status bar icon. Once they add Chrome-like transparency and a Chrome-inspired Stop/Refresh combo button, it'll have the nicest look of all the browsers. And good looks are VERY important to me given how long I stare at the thing.
-
Was it SnagIt? My mother has used it both at her work and at home for 15+ years and swears by it. I've been trying to get her to convert to the built-in Windows 7 variant, but no luck so far.
-
To be honest, I'm not sure what you're asking. The program takes in some input, and calculates the money earned on that data via interest for 39 years. There's no real formatting per se, as everything is simply spewed back out to standard output. Each line has some formatting - to currency, or a specifically given floating point precision - but that's it. You should ensure your conversions from string to int to double all work. The compiler can get picky with that sort of thing.
-
function closeTable(id) { var oTable = document.getElementById(id); oTable.style.display = 'none'; }
-
Okay, here's what's going on: Your library code (countdown.js) dynamically creates a <span> element to stuff the countdown into. Each loop your PHP code executes means a new <span> is created for a countdown. The problem is that each <span> has the same id - cntdwn. So, to be absolutely clear, your test has 3 <span> elements that look like: <span id="cntdwn"></span> Semantically, this is wrong, as an id means a unique value, and thus should be applied to one and ONLY one element in the document. JavaScript knows this, and simply stuffs the countdown into the first 'cntdwn' <span> it finds, ignoring the others. So, the countdown that's displayed is for the last wave, even though it looks like it's for the first. Unfortunately, there's no simple, presto-change-o fix for this. Even if you simply changed the <span> elements from having an id of 'cntdwn' to a class of the same name, you'd still need to figure out which <span> gets the right countdown. I suggest a complete rewrite of the JavaScript. You can easily generate the conditions for each countdown, as you've already shown. And there's no need to actually display the countdown during the loop - one of the beauties of JavaScript is that since you can easily grab a hold of the various document elements at any time, you can deal with them whenever you want. So, why not generate the data during the loop, then, display all of the countdowns at once?
-
You, uh, might want to include the PHP loop, so everyone can see what it's doing....
-
I don't have time to go through all of it, but your submit button does not have a name. That may be causing the error. Also, that validation code is horrid. Eval isn't necessary for something like this, as you can simply pass element references into the appropriate functions, and access their attributes and tie into their events that way. I'm guessing (hoping) that you got it from a 3rd party.
-
I think you were active here before I was, so I don't remember you. Nevertheless, it's always nice to see a current/former member of the team. So, welcome back, and I hope you can stick around.
-
Because it ensures that any other code attempting to access that info cannot also overwrite it?
-
One of the pains that every beginner feels when trying to learn OOP is a lack in confidence that their class is 'right'. How simple is too simple? How complex is too complex? How much should an object try to do? A couple of (probably not very helpful) things to keep in mind: KISS - Keep It Simple, Silly/Stupid. Single responsibility. A class'/object's purpose should be describable in one sentence, with maybe one or two words like 'and' and 'or'. This helps keep things organized and clean, and facilitates the writing of good code. So, with that said, what do you envision a manage_student.php file to look like? And how would it differ from what you have now?
-
That's a really bad idea. Queries shouldn't be provided by the user.
-
Even though the code that's generating the links is written in PHP, its outputting HTML. Simply use what you would for straight HTML with CSS - an id or class that a stylesheet can grab a hold of, or an inline style. Example: while($val = mysql_fetch_assoc($values)) { echo "<a href='newpg.php?id=" . $val['key'] . "' class='links'>" . $val['itsType'] . "</a><br />"; }
-
Upon closer inspection, where is your query? Properly formed database queries look like: $dbc = mysqli_connect('localhost','root','','Project4'); $data = $_POST['entry']; $result = mysqli_query("SELECT * FROM tablename WHERE data = $data", $dbc); while($row = mysqli_fetch_assoc($result)) { //do something with the fetched db row } You should brush up on your basic db interaction code, because most of what you have right now is gibberish.
-
Form processing should be done before you display the form itself. <?php if(isset($_POST['submt'])) { //process data } ?> <!-- show form -->
-
[SOLVED] Couple of "sessions" related questions!
KevinM1 replied to andreasb's topic in PHP Coding Help
For the first question, remember that sessions are separate from whatever database scheme you're using to save user information. It's probably easiest to think of them as variables that persist between pages. So, you'll have to use the value in the session to query the database for more info, i.e.: session_start(); if(isset($_SESSION['username'])) { $result = mysql_query("SELECT * FROM users WHERE username = {$_SESSION['username']}"); } For the second question, something like: session_start(); if(isset($_SESSION['loggedIn'])) { header("Location: profile.php"); } else { //not logged in } EDIT: D'oh, beat by a hair! -
Start simple. Work on the basics first, like figuring out how to display the forum, any sub-forums, and the threads themselves. OOP is perfect for something like this, as a lot of forum components map naturally to objects (users, the posts themselves, etc).
-
AFAIK, list items aren't focusable, so they can't be tabbed. What can be tabbed are the elements inside them, depending on what they are. Try attaching the tracking to the items within the list items.
-
Wouldn't it make more sense to tie the event to the document as a whole?
-
A more eloquent solution to the OP that shows how passing the errors array into the function can work: $errors = array(); function formValidate($field, $msg, &$errorArray) { if(empty($_POST[$field])) { $errors[] = $msg; return false; //since we're returning a legit value later on in the function, I like to return a false/null value in an error condition } else { $field = mysqli_real_escape_string(trim($field)); return $field; } } $result = formValidate('name', 'You must enter your name!', $errors); if(count($errors) > 0) { //handle errors } else { //insert $result } I didn't mention it earlier since the OP had a problem with both return values and scope. I figured passing an argument by reference may be too advanced for their current skill level, but since the idea of using global to 'fix' the problem came up, I figured it's best to show the right way of doing it.