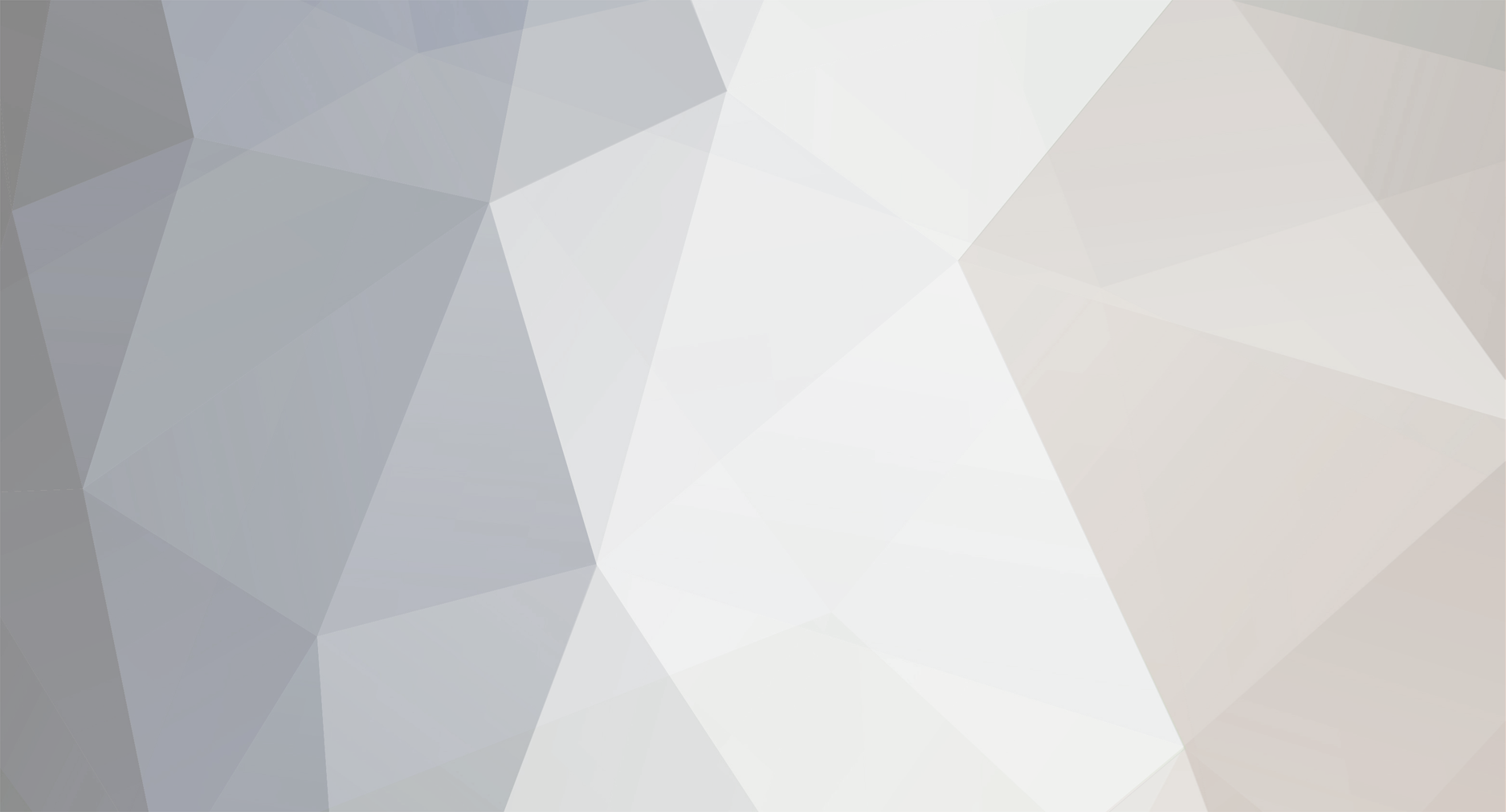
KevinM1
Moderators-
Posts
5,222 -
Joined
-
Last visited
-
Days Won
26
Everything posted by KevinM1
-
You should probably google non-disclosure and non-compete agreements.
-
Classes Static Methods VS Instance Methods WAR!
KevinM1 replied to Rangel's topic in Application Design
Using static everywhere is like a carpenter using only a screwdriver. Yeah, they can probably hammer nails with it, but it's not the best tool for the job. In your (Rangel's) example, as canned as it may be, I can't help but think that each user should be its own object. It's just more efficient to have instance variables in cases like this. Something like: class User { private $name; private $category; public function __construct($name) { $this->name = $name; } public function getName() { return $this->name; } public function setCategory($cat) { $this->category = $cat; } } class UserList { private $users; public function __ construct($users) { $this->users = $users; } public function addUser($user) { $this->users[] = $user; } public function setCategory($userName, $cat) { for($i = 0; $i < count($this->users); i++) { if($this->users[$i]->getName() == $userName) { $this->users[$i]->setCategory($cat); return; } } echo "User not found"; } } $angelina = new User("Angelina Jolie"); $brad = new User("Brad Pitt"); $jealous = new User("Jennifer Aniston"); $users = array($angelina, $brad, $jealous); $userList = new UserList($users); $userList->setCategory("Jennifer Aniston", "stalker"); It's not hard to envision UserList having a method to remove individual users from its internal array, as well. To me, the code I wrote is far more clear than yours. It deals with concrete entities, each with its own set of properties. I can mix and match User objects whenever I want without compromising their integrity or the integrity of other components in the system. Something else I just caught: While __construct may be a magic method, its purpose is to set properties and invoke other methods during object creation. In other words, there's an implied usage expectation when using that method. The way you're using it is both confusing, as you never intend to use the object that's briefly created, and ultimately pointless, as you don't need a child object there at all. From what I can see, after looking at your code more while slowly waking up this morning, you pretty much miss the point of OOP. I mean, data encapsulation is a big part of it, yet your class properties are publicly accessible, meaning they can be changed at any time. You never create any objects (at least none that persist) - what if you wanted several arrays of users, based on their membership levels? What if users had more info attached to them than their name and membership level? There's a reason why it's termed Object Oriented Programming. -
Object instantiation and use is a key component to OOP. There's really no way around it in the real world. Even in OO languages like Java or C#, where there are libraries of code/objects available for you to use, you'll inevitably need to roll your own at some point.
-
Dunno, but the code is junk. You're using the for-loop wrong. Try this: <script type="text/javascript"> window.onload = function() { var toggles = new Array(); var panes = new Array(); var links = document.getElementsByTagName('a'); var divs = document.getElementsByTagName('div'); for(var i = 0; i < links.length; i++) { if(links.getElementById('toggle' + i)) { toggles[i] = links.getElementById('toggle' + i); } } for(var j = 0; j < divs.length; j++) { if(divs.getElementById('bld' + j)) { panes[j] = divs.getElementById('bld' + j); } } for(var k = 0; k < toggles.length; k++) { toggles[k].onclick = function() { panes[k].style.display = (panes[k].style.display == 'none') ? 'block' : 'none'; } } } </script> <!-- ... --> <table border='0' style='border-collapse: collapse;' width='500'> <tr style='border-bottom: 1px solid gray;'> <th width='100' align='left'>Armor Maker</th> <td align='right'> Levels: <a id='toggle31' href='#'>1</a> <a id='toggle32' href='#'>2</a> <a id='toggle33' href='#'>3</a> </td> </tr> <tr> <td colspan='2'> <div id='bld31' style='display: block;'>test1 1</div> <div id='bld32' style='display: none;'>test2 2</div> <div id='bld33' style='display: none;'>test3 3</div> </td> </tr> </table> Something like this should work, assuming you have the same number as links as you do divs, and it wouldn't hurt to keep their id's lined up (i.e., toggle31 is used for bld31, etc). Keep in mind that I haven't tested this, so don't expect it to work properly just by cutting and pasting. You may need to play with it.
-
Unfortunately, that doesn't really shed any light on the problem. It looks like you have several 'islands' of JS in your code, but without seeing exactly where it's located in the document, and any code that provides context for what's going on, I can't really diagnose the problem. Is this JS something you wrote yourself, or is it a 3rd party script?
-
Help with changing images and hiding divs with a script
KevinM1 replied to Darkmatter5's topic in Javascript Help
Part of the problem is that you have two images with the same name. Here's how I'd do it: <script type="text/javascript"> window.onload = function() { var images = document.images; var toggles = new Array(); for(var i = 0; i < images.length; i++) { if(images[i].className == 'toggle') { toggles.push(images[i]); } } for(var j = 0; j < toggles.length; j++) { toggles[j].onclick = function() { var oDiv = document.getElementById('div' + j); if(this.src.indexOf('images/minus.gif') != -1) { this.src = 'images/plus.gif'; oDiv.style.display = 'none'; } else { this.src = 'images/minus.gif'; oDiv.style.display = 'block'; } } } } </script> <!-- in your HTML --> <form method="post"> <img class="toggle" src="images/plus.gif" />Expand div 1 <br /> <img class="toggle" src="images/plus.gif" />Expand div 2 <br /><br /> <div id="div1" style="display: none;">Sections</div> <div id="div2" style="display: none;">Coords</div> </form> There are pros and cons doing it this way. Pros: -Your markup is free of JavaScript -It's dynamic -- you don't need to manually write the function call for each image, and if you're creating your markup from a server-side script, so long as you have an image with a class of 'toggle' and a corresponding <div>, it'll work. Cons: -Initial overhead. If you only have a couple of <div> elements that need to be toggled, then this solution is overkill -Loss of semantic meaning with the <div> elements themselves. Since each id is now simply 'div#', applying CSS is more difficult.* *There is a workaround. If you give all the divs you want to toggle a class name, then you can keep their more meaningful id's. Keep in mind, I haven't tested this, but it should work. -
Without being able to see the code, this could be the root of the problem. You need to ensure that the HTML is fully rendered before attempting to obtain element references. If you're using a lot of inline JavaScript, then it won't be much of an issue as it's written at the same time as the markup. But if you have element references placed within <script> tags, they may be null because JavaScript is attempting to obtain the elements before they exist. So, something like: <script type="text/javascript"> window.onload = function() { /* script goes here */ } </script> May fix the issue. Again, this is without seeing any code, so there's definitely no guarantee that this will fix the issue.
-
Unfortunately, your request is a bit vague. Is the error occurring with a certain version of IE? Do you have any code that's supposed to fire when the page is unloaded? Have you checked it with FireFox and FireBug? Can you generate the error yourself? Are you waiting to load the JS until after the HTML is fully rendered?
-
[SOLVED] How can i get get a variable from a while loop
KevinM1 replied to madspof's topic in PHP Coding Help
Not only is it possible, but it's the preferred way to structure PHP applications. While it's possible to switch from PHP to HTML and back on the fly, it's best to keep the two as separate as you can. Process, then output. -
[SOLVED] How can i get get a variable from a while loop
KevinM1 replied to madspof's topic in PHP Coding Help
Rethink your design. Instead of switching between PHP and HTML in a haphazard fashion, do all of your PHP processing first, then write the HTML output. It'll save you headaches like these, and make your code easier to maintain in the long run. -
Try changing it to: <?php if($scriptName == 'thispage.php' || $scriptName == 'thatpage.php') { //do stuff } ?>
-
Well, you have several problems, the biggest of which is your confusion about how values are passed to the script by a form. First, 'link' is not a valid form method. You have two choices - post and get. Post sends values to the server behind the scenes. Get sends values as a query string appended to the end of a URL. In most cases, you want to use post. Sending form values to PHP is ridiculously easy. In your form, simply choose a method (post or get), and give each input a name. Then, in the PHP script (given by the action attribute of the form), you can access your inputs by simply writing: <?php $formInput = $_POST['inputName']; //Or $formInput = $_GET['inputName']; ?> Where inputName is the name you gave your form input in the HTML, and $_POST/$_GET is the form method you used.
-
Hmm... how are the shirts themselves loaded? Because IMO the simplest solution (without knowing how the rest of your system works) would be to set the shirt image as the background image for the element, then have the shirt design/logo that's selected by the dropdown fill the content of that element. Since the shirt image is set as the background, you should get your overlay effect.
-
Wow I didn't realize prepared statements are auto escaped! Thanks for the links as well! would this work ? <?php # database connection scripts # the next 4 lines you can modify $dbhost = 'localhost'; $dbusername = 'root'; $dbpasswd = ''; $database_name = 'share'; #under here, don't touch! $connection = mysql_connect("$dbhost","$dbusername","$dbpasswd") or die ("Couldn't connect to server."); $db = mysql_select_db("$database_name", $connection) or die("Couldn't select database."); if(!get_magic_quotes_gpc()) { $_GET = array_map('mysql_real_escape_string', $_GET); $_POST = array_map('mysql_real_escape_string', $_POST); $_COOKIE = array_map('mysql_real_escape_string', $_COOKIE); } else { $_GET = array_map('stripslashes', $_GET); $_POST = array_map('stripslashes', $_POST); $_COOKIE = array_map('stripslashes', $_COOKIE); $_GET = array_map('mysql_real_escape_string', $_GET); $_POST = array_map('mysql_real_escape_string', $_POST); $_COOKIE = array_map('mysql_real_escape_string', $_COOKIE); } function clean($value) { if (is_array($value)) { foreach($value as $k => $v) { $value[$k] = clean($v); } } else { if(get_magic_quotes_gpc() == 1) { $value = stripslashes($value); } $value = trim(htmlspecialchars($value, ENT_QUOTES, "utf-8")); $value = mysql_real_escape_string($value); } return $value; } ?> Change it around to: <?php function clean($value) { if (is_array($value)) { foreach($value as $k => $v) { $value[$k] = clean($v); } } else { if(get_magic_quotes_gpc() == 1) { $value = stripslashes($value); } $value = trim(htmlspecialchars($value, ENT_QUOTES, "utf-8")); $value = mysql_real_escape_string($value); } return $value; } # database connection scripts # the next 4 lines you can modify $dbhost = 'localhost'; $dbusername = 'root'; $dbpasswd = ''; $database_name = 'share'; #under here, don't touch! $connection = mysql_connect("$dbhost","$dbusername","$dbpasswd") or die ("Couldn't connect to server."); $db = mysql_select_db("$database_name", $connection) or die("Couldn't select database."); $_POST = clean($_POST); $_GET = clean($_GET); $_COOKIE = clean($_COOKIE); ?>
-
So MySQLi protects against that then? With prepared statements, type is enforced. You can see how to construct them here: http://us.php.net/manual/en/mysqli.prepare.php And, the kind of datatypes it accepts here: http://us.php.net/manual/en/mysqli-stmt.bind-param.php Prepared statements are also automatically escaped, so you don't need to run something like mysql_real_escape_string().
-
Things like this are why I like using MySQLi and its prepared statements.
-
I don't believe that array_map used at the top-level will work on request data that is itself an array (checkbox results, for example). I use the following function, which I got from someone else on here: <?php function clean($value) { if (is_array($value)) { foreach($value as $k => $v) { $value[$k] = clean($v); } } else { if(get_magic_quotes_gpc() == 1) { $value = stripslashes($value); } $value = trim(htmlspecialchars($value, ENT_QUOTES, "utf-8")); //convert input into friendly characters to stop XSS $value = mysql_real_escape_string($value); } return $value; } ?> Other than that, make sure you validate incoming data...a name, for instance, shouldn't contain an integer.
-
Give your table a unique id. Then, modify the init function to be: function init() { var myTable = document.getElementById('tableId'); //<-- use whatever id you want var rowElements = myTable.getElementsByTagName('tr'); //<-- retrieve just that table's rows /* use the rest of the function as-is */ }
-
You seem to have a scope problem. Namely, the $result within your function is not the same as the $result in your while-loop. You need to store the returned $result from the function in a variable, then test against that. Just because you named your conditional variable $result doesn't mean it's magically being set/updated. Your best bet is to remove the while-loop altogether and run the function twice, storing the results in separate variables. If neither is equal to "Journey Not Available" then add the strings together, else, there's a scheduling conflict you need to handle.
-
Well, it's hard to tell from looking at a couple of code snippets.... Let's start with what you do know, and work from there.
-
Why would you want it to be public? In the vast, vast majority of cases, class properties should be private.
-
Class Design- objects/classes inside classes- Critique my idea
KevinM1 replied to JoeBuntu's topic in PHP Coding Help
But wait, PHP has some form of type hinting.... As far as I can tell, PHP's type hinting isn't available for object members/fields. Instead, it only works with method arguments. -
Class Design- objects/classes inside classes- Critique my idea
KevinM1 replied to JoeBuntu's topic in PHP Coding Help
Nested tables could be a problem, but nested HTML tags, in general, could be solved easily. Just save cell data as a string, tags included. Regarding JavaScript - this is where unobtrusive JavaScript comes in. In most cases, if you're using inline JavaScript calls, you're doing it wrong. There's no reason why JavaScript should be embedded in HTML when there are a variety of ways to get element references from outside the markup. What the OP is trying to do is limit the size and scope those PHP 'pockets'/islands have on a page template. Instead of having a big where-loop in the middle of a template, he can simply write: <?php $table->print(); ?> And the entire thing will be output properly. So, in short, the OP is trying to strengthen the divide between logic and output with his OO tables. It's unfortunate that PHP doesn't adopt a full code-behind model to compliment its inline model. It'd save some design headaches in the long run. -
Class Design- objects/classes inside classes- Critique my idea
KevinM1 replied to JoeBuntu's topic in PHP Coding Help
Heh, I'm currently doing an ASP.NET/C# project myself. With PHP, one of the things you need to keep in mind is that it doesn't have the property mechanism of VB.NET/C#. So, I'm a bit concerned seeing code like: $table->rows->addRow('body'); PHP doesn't check against type, so there's an inherent risk of losing encapsulation along the way. If I'm reading your example correctly, 'rows' is a public field of $table - most likely a Row object or an array of Row objects. This field can be overwritten by anything. Even something like: $table->rows = 1234; And PHP won't give an error. If you're already aware of this, then I suggest getting rid of the 'rows' portion altogether and having an interface like: $table->addRow('body'); Instead. -
Class Design- objects/classes inside classes- Critique my idea
KevinM1 replied to JoeBuntu's topic in PHP Coding Help
It sounds like a very .NET approach to PHP. The biggest hurdle is with data binding. How will you handle creating the table and storing values inside it? Doing something like: $table = new Table("ID", "Name"); $table->addValues(1, "Bubba"); Is a lot more palatable than doing something like: $table = new Table(); $headerRow = new Row(); $idColumn = new PlainTextColumn("ID"); $nameColumn = new PlainTextColumn("Name"); $headerRow->addColumn($idColumn); $headerRow->addColumn($nameColumn); $table->addRow($headerRow); And that's before any data is even added to the table.