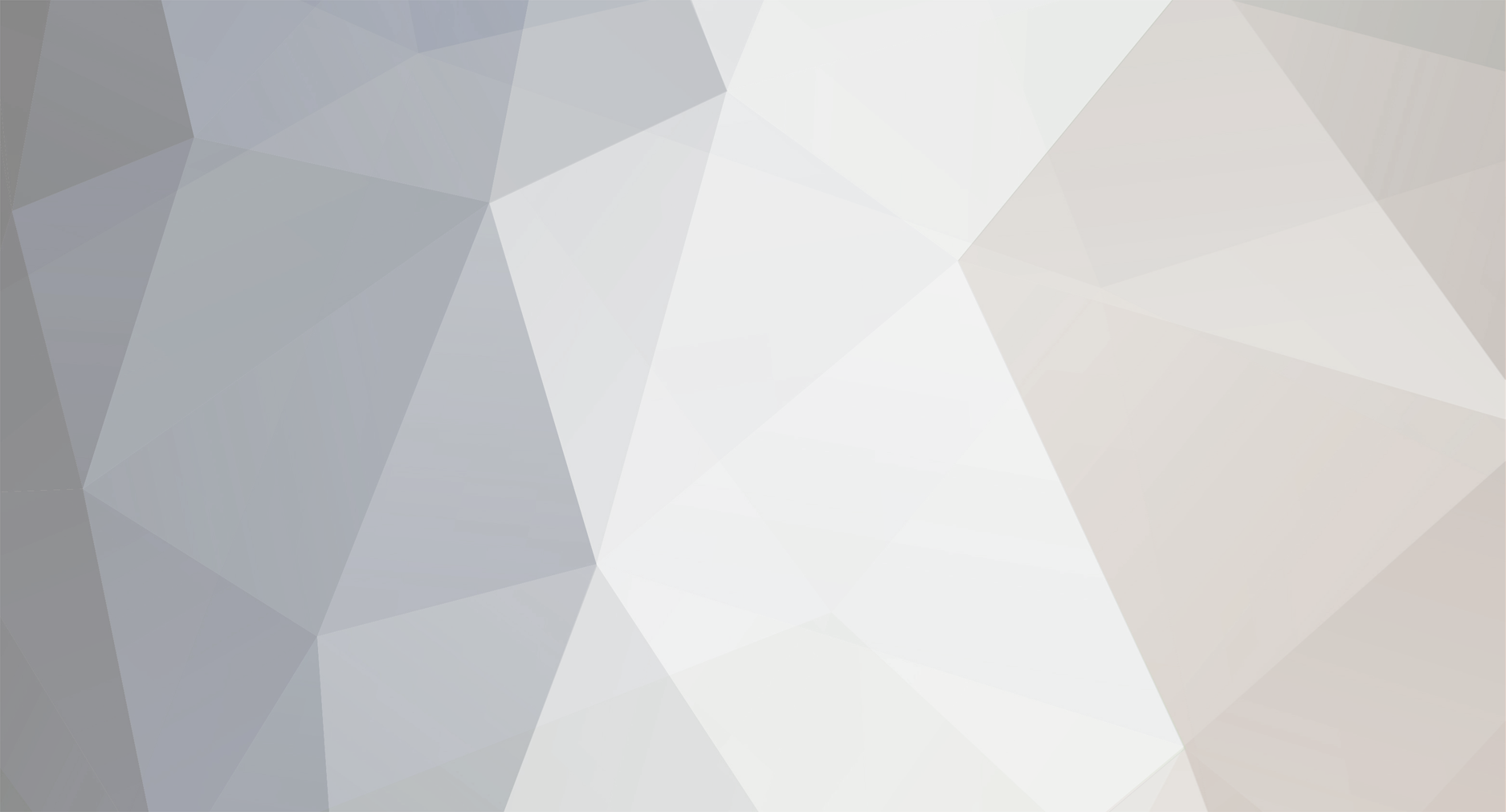
KevinM1
Moderators-
Posts
5,222 -
Joined
-
Last visited
-
Days Won
26
Everything posted by KevinM1
-
The problem with HTTP_REFERER is that you're not guaranteed that it'll work 100% of the time (see: http://us3.php.net/manual/en/reserved.variables.server.php). So, you essentially have two options: 1. Use JavaScript to go back, like you have above 2. Pass some sort of value/flag that represents the originating page to the login page via a query string or hidden field, then use that to determine what page to redirect to
-
Exactly right. window.onload is used when someone writes JavaScript in an unobtrusive manner to ensure all of the HTML document's elements are present in the DOM before the script attempts to access them. That, in turn, gets rid of those pesky "something-or-another is not an object" errors that tend to crop up. It's pretty much the same thing as using jQuery's $(document).ready(), except it isn't quite as fast.
-
OOP Databases Singletons and Registries
KevinM1 replied to black.horizons's topic in Application Design
Well, one of the points of OOP is to make code modular. So, you should do what you can to make it so you can plug your db class into a variety of projects. That's why I suggest passing in some form of settings data as an argument during instantiation. In most real world scenarios, info like db connection settings are read in from a separate file. That way, instead of having to muck with actual system code in order to get this class to work on separate projects, all you'd need to modify is a non-system settings file. -
OOP Databases Singletons and Registries
KevinM1 replied to black.horizons's topic in Application Design
Using a singleton for a db object is pretty common practice. Whenever you want to use it, you simply store a reference to it in a variable by calling getInstance: $myDBO = Database::getInstance(); $myDBO->query(/* some query */); Since there can be only one instance of any given singleton class in the system, you'll have only one connection to the database. Something to consider - db initialization. When you first instantiate your database singleton, you'll probably want to pass in the relevant database connection info. There are a few ways to do it, but I think the simplest would be to store them in an associative array, and pass that into getInstance. We can make it (and the constructor) flexible by using default argument values: private function __construct($settings = null) { if($settings) { /* store each setting in the data member variables */ } } public static function getInstance($settings = null) { if(!self::$instance) { self::$instance = new self($settings); } return self::$instance; } With this, you're not forced into passing db setting info to getInstance every time you want to manipulate the db. Instead, you'll only need to do it in the first invocation of getInstance, when the object is actually constructed. -
An easier (and better designed) way of doing it is to do the following: 1. Instead of using <input> tags, use <button> tags. This will make it easier for us to grab a hold of all the buttons within the form. 2. Use unobtrusive JavaScript. There's no reason to embed JavaScript function calls within your HTML. So, let's do things the right way. <html> <head> <title>Calculator</title> <script type="text/javascript"> window.onload = function() { var buttons = document.getElementsByTagName('button'); for(var i = 0; i < buttons.length; i++) { buttons[i].onclick = function() { alert(this.value + " was clicked."); } } } </script> </head> <body> <!-- someplace later, within your calculator form --> <button type="button" value="1">1</button><button type="button" value="2">2</button><button type="button" value="3">3</button> <!-- etc. --> </body> </html>
-
OOP Databases Singletons and Registries
KevinM1 replied to black.horizons's topic in Application Design
A singleton is an object with two primary characteristics: 1. It's a global 2. Only one instance of that particular singleton can exist at any one time If you've been reading these forums, you've probably read the mantra "globals are bad." Why are they bad? They're bad because they tend to break encapsulation, and they promote tight coupling of an object to the greater system it resides in. In other words, relying on globals can make code harder to debug, less modular, and just a pain to deal with in general. Raw globals can be rewritten at any time, and there's no way to ensure that the global you want is the one you're currently using. Singletons mitigate this somewhat. Their structure (which you'll see below) only allows one to exist. Also, since they're objects, you can enforce a public interface so that their internal values aren't overwritten by accident. In the most general sense, a singleton looks like: class Singleton { private static $instance; private function __construct() {} public static function getInstance() { if(!self::$instance) { self::$instance = new self(); } return self::$instance; } } Obviously, other methods can be added to this, and both getInstance and the constructor can take arguments if you need them to. Registries are somewhat different as they serve a different purpose. The whole point of a registry is for you (or the system itself) to record and store values for future use. Think of a site that allows 3rd party plugins - they need to be registered with the system in order for the system to realize they're available for use. Where does a singleton enter the picture? Registries are often represented as singletons. The reason being that you can have different types of registries (one that stores request data, one that stores references to 3rd party plugins, etc), but you most likely only want one of each of those, as any more would be redundant. And, you probably want to be able to access those registries at any time as there's no telling exactly when you'll be reading/writing to a registry, so having them globally available is desirable. I hope this helps. -
[SOLVED] Of database design, world of warcraft and antipatterns
KevinM1 replied to simpli's topic in Application Design
Yup, that's about right. BTW, it would be trivial to integrate it with AJAX. I mean, since the user would have to send requests to the server manipulate data anyway, there's no reason why it couldn't be combined with immediate DOM interaction. Once again, though, if the data is as critical as you mentioned, this may not be the best approach from a security standpoint. -
[SOLVED] Of database design, world of warcraft and antipatterns
KevinM1 replied to simpli's topic in Application Design
Well, the prototype design pattern is a creational pattern in which the object(s) you want to construct provide a copy of themselves for the client to use. Typcially, there will be an object registry which is just an associative store of objects available to the client. When a request for an object is received by this store, it finds the appropriate object and returns a clone of it to the client. With your system, a user would request their db info. To facilitate that request, your system could return a clone of a generic database object populated with the correct data based on the user's id, or some other identifier. Then, they could manipulate this data in a disconnected way by dealing with the database object rather than directly using the db. Once they decide to save their work or otherwise end their session, you could then run the database object through whatever security checks you want to use before saving everything back in the db. So long as your system doesn't require new tables to be added to the db, it shouldn't be hard to construct an object that mirrors your db's structure. As far as normalization goes, I'm just not sure how you want to set up your tables. It was something of a stray idea/comment I had. More info on the prototype pattern (read the last paragraph, as it's essentially what my idea is): http://en.wikipedia.org/wiki/Prototype_pattern -
[SOLVED] Of database design, world of warcraft and antipatterns
KevinM1 replied to simpli's topic in Application Design
I've never been great when it comes to large database-driven apps, so please forgive me if this is dense, but.... Why not just have one database and normalize them like normal, then in the middleware tier of the app (PHP), use the prototype pattern to give each user 'their own' db object to play with? So long as the basic structure of each company's db is the same (that is, they all rely on the same tables to do business), you can simply give each user a cloned db object parameterized with their specific info. I actually like the 'give them each their own db' idea, given the security concerns, but if that's not an option I think prototyping may be the way to go. -
[SOLVED] PHP and Javascript: Just How Similar are they?
KevinM1 replied to Fluoresce's topic in Miscellaneous
Like Mchl said, their similarities lie in their syntax. Other languages have a similar syntax as well (C, C++, C#, Java, Perl, etc.). The two are completely different, otherwise. PHP is a server-side language. Therefore, it can't read and respond to browser events (button presses, mouse movement or clicks, etc.) directly. JavaScript is client-side. It runs in the browser and allows sites to be more interactive by responding to the kinds of browser events I mentioned earlier. -
Adding stuff in innerHTML, without clearing current fields data?
KevinM1 replied to JesuZ's topic in Javascript Help
Hmm...that's probably because the values written into the fields aren't considered to be a part of the existing HTML. So, when you append the new HTML to the form, the values are lost because, as far as innerHTML is concerned, they don't exist anyway. An easy fix is to temporarily store all the form's values in variables, then once the new HTML is appended to the old, reassign them to the proper fields. Something like: var myForm = document.getElementById('myForm'); var originalFormLength = myForm.elements.length; var tmpValues = new Array(); for(var i = 0; i < originalFormLength; i++) { tmpValues[i] = myForm.elements[i].value; } /* innerHTML assignment here */ for(var j = 0; j < originalFormLength; j++) { myForm.elements[i].value = tmpValues[i]; } It's a bit more difficult to do with a mix of form input types (i.e., text and/or radio buttons and/or a select element, etc), but you should get the idea. -
Adding stuff in innerHTML, without clearing current fields data?
KevinM1 replied to JesuZ's topic in Javascript Help
Hmm...I'm not sure. I just wrote a simple test script (code below) that works fine: <html> <head> <title>blah</title> <script type="text/javascript"> window.onload = function() { var myDiv = document.getElementById('myDiv'); var myButton = document.getElementById('myButton'); myButton.onclick = function() { myDiv.innerHTML += " button was clicked "; } } </script> </head> <body> <div id="myDiv"></div> <br /> <br /> <button id="myButton">Click Me</button> </body> </html> Question: when exactly is your div losing the new text? Because things set with innerHTML won't persist if you're refreshing the page due to a form submission. -
Adding stuff in innerHTML, without clearing current fields data?
KevinM1 replied to JesuZ's topic in Javascript Help
Try using the += operator, like so: var myDiv = document.getElementById('myDiv'); var moreText = "and, another thing.... "; myDiv.innerHTML += moreText; -
Regarding form handling: First you need to understand what a web form is. The easiest example is a website user registration form, like the one you used when you registered here. Forms can have a variety of fields, and they can have different attributes - text, checkbox, radio button, etc. These forms are created through simple HTML tags. The 'magic' happens when a user clicks on a button to submit the information they entered into the form. Processing form information is typically called 'form handling,' and that's where a server-side language like PHP comes into play. A form handling script, at the most basic level, takes the information that the user entered into the form and does something with it. What that something is depends on what the form is trying to accomplish. Our registration form example would require the script to ensure that the desired user name isn't already taken by someone else, verify that the potential new user's e-mail address is legitimate, and, if everything checks out, store their credentials in the database. And that's without thinking of other considerations like validating input and security. The most common language structures in play here are the superglobal arrays $_GET[] and $_POST[]. So, that could be a starting point for your own research.
-
There are some things you should look at as you begin: form handling and interacting with a database. If you're going to use PHP, chances are you're going to do a lot of both. If you're going to be doing a lot of database work, you should also look up some information on database normalization.
-
Actually, she said that instead of display: none, she was thinking of putting it off-screen (-999em) during the initial load. I assume height 0 would be to ensure it didn't screw up other elements' positioning, since it'll be absolutely positioned initially. Regarding jQuery, I like it because it has a good power-to-simplicity ratio - a small amount of code can do a lot - and its CSS selector engine is incredible. That code written in it is very readable, and that the framework as a whole has a small footprint is icing on the cake.
-
Hmm...a quick look at the brothercake link shows that it's similar to something like jQuery's ready method. Since I would follow John Resig into the bowels of hell simply because his code is beautiful, I recommend trying jQuery's version. Why? I trust his code, and I've never heard of brothercake before. You can find jQuery here: http://docs.jquery.com/Downloading_jQuery Since you probably don't want to port all of your code over to use jQuery's syntax, I suggest stuffing what you have now within the ready method like so: <script type="text/javascript"> $(document).ready(function() { /* rest of the script goes here */ }); </script> Obviously, there's no guarantee that it'll work, especially since you tried something similar, but it's worth a shot. If that doesn't work, you could perhaps have two menus - one for JavaScript users, one for those with JavaScript turned off. Have the entire JavaScript menu have a display attribute set to 'none' and put the other menu in a <noscript> tag. Then, have your script set the display of the menu to 'block' when the document loads. If JavaScript is disabled, the <noscript> version will be displayed. Otherwise, the script will make the more dynamic version visible.
-
Well, the problem with your example is that it's too simple. Something like adding two numbers probably would be better served by creating a simple function rather than an object. The entire point of OOP is to create a (relatively) simple interface that hides a more complex implementation underneath. Why is this important? If the interface remains the same, you can use a variety of different objects that implement that interface. Things become modular, and thus more reusable and flexible. Let's say you're creating a couple of sites, one for news, another for cooking recipes. Both need to render content to the screen, but need to do it in different ways. However, since you want your sites' structures to be uniform across the board, you don't want to have custom client/controller code for each one. The solution is to create objects to encapsulate the concept(s) that vary, in this case the news and recipes. Since everything should be uniform, you need to start with an abstract (non-instantiated) base class that the others derive from. So, something like: abstract class Content { protected $info; public function __construct($info = null) { $this->info = $info; } public function setInfo($info) { $this->info = $info; } protected abstract function render(); } Notice that the 'render' method is abstract. This informs PHP that the actual implementation of this method will be defined in the child classes. Let's define those now: class News extends Content { protected function render() { echo "<div style='font-weight: bold;'>$this->info</div>"; } } class Recipe extends Content { protected function render() { echo "<div style='color: blue;'>$this->info</div>"; } } Obviously, these examples are a bit canned, as more specific and complicated formatting should be applied, but it still illustrates my point. Let's create one more object that returns the correct child object to the system based on a value sent by a query string: class ContentFactory { public static function getContent($value) { if(strtolower($value) == "news") { return new News(); } else { return new Recipe(); } } } So, assuming we have a link in the form of www.example.com/info.php?contenttype=something we can get the right kind of object simply by writing: $contentType = $_GET['contenttype']; $contentObj = ContentFactory::getContent($contentType); $contentObj->setInfo("Hello World"); $contentObj->render(); Again, this example is a bit canned. The info should be retrieved from the database, and there should be error checking, but I believe the basic concepts are there. Hope this helps.
-
Properly designed tables don't change, at least not in terms of structure. You shouldn't be adding/removing entire table columns on the fly. I'm not sure if that's what you mean by 'dynamic tables' or not, but it sounds like you're overthinking it. Read up on database normalization (if you Google it, one of the top results is a great article on the MySQL site). I think that will solve your problem.
-
website design and communication between PHP and C++
KevinM1 replied to geantbrun's topic in Application Design
I've never used C++ and PHP together, so these suggestions are a bit of a shot in the dark. First, I'd try to keep the web component (the Joomla site) as separate from the form processing (the C++) as possible. Other than some simple communication ("here's the user-submitted info", "thanks, the process has started"), they shouldn't interact with one another. Somehow, the C++ code needs to know when the form submission happened. Can C++ handle/read HTTP requests? If so, you could forgo the database all together as those values will be sent via POST. You could intercept them right at form submission instead of storing them (unless, of course, you need to take into consideration any run-time collisions). Once the process begins, the C++ code will need to send word to the website. The easiest way would be to send another HTTP request - either through POST or GET - with a boolean value to represent the success/fail of the process initializing. On the PHP side, you just need to make sure the form points to the C++ function, and that you have another bit of code than can handle the incoming success/fail value. Once again, this is a bit of a shot in the dark, so take these suggestions with a pitcher of salt. -
What do you mean by 'dynamic table'? Database normalization is the process of creating new tables in order to eliminate anomalies in storing, editing, and retrieving data. So, an aversion to having many tables stems from not wanting to do the tedious work rather than a good design decision. I can't say I blame you, as DB work is incredibly boring, but you'll wind up tanking your entire site if you don't bite the bullet and attempt to do it right the first time. I'm wary about mapping objects to tables, especially in the way you're looking at it now. You're not saving yourself any work by mirroring the DB in objects. Let the DB handle the heavy lifting - that's what it's there for, and it's much more efficient to keep the data manipulation there. A DB object should, instead, be: 1. Generalized. It should be able to handle any database and any tables within that database. 2. A convenient way to access the database. It should be used as a simple proxy API to keep the client code as simple and readable as possible. So, instead of having a class db_users, and db_cars, and db_computers, and others, you should have one class that can handle queries and retrieve results. Also, unless you have reason for the client code to have direct access to your object's data members, you should declare them as private, and create accessor functions to manipulate them should the need arise. Otherwise, you kill your object's encapsulation.
-
Something that I hope the OP has figured out is that the term 'dynamic' means something different whether it's used to describe client-side or server-side design. With the client-side (read: JavaScript), 'dynamic' tends to mean interactive. You click on a button, or move the mouse over a certain element, and something changes immediately without a page refresh. With the server-side (read: PHP, Python, Perl, ASP.NET, Ruby, etc), 'dynamic' means being able to serve content based on some user-submitted value. It's not immediately interactive, as it requires a request to be sent to the server, that request to be analyzed and processed by the script running on the server, and the results sent back to the browser. This requires (in most cases) the page to be refreshed, or another page to be loaded. Database-driven sites are considered 'dynamic' because they facilitate this behavior. The user clicks a link whose URL has a query string, or submits a form, and the proper information is retrieved from the database to populate the next page. An example of this behavior can be seen with this forum. So, why is that termed 'dynamic' if the results aren't immediate? In the dark ages of the internet most sites were static HTML pages with the data hard-coded into each page's markup. These were mostly unpleasant times that we should never speak of again.
-
Doubtful. I wouldn't recommend one anyway. Most generators and wizards and whatnot generate awful code. And that's if they're not malware to begin with. What kind of records, and who needs to be generating/retrieving them? Easy enough to do. Slightly harder, depending on how in-depth this performance tracking needs to be. How were the other sites you made structured? Can you lift parts/ideas from them to incorporate in your quiz site? If you already have a login system, building a registration system off of that won't be difficult. As for the rest, have you tried using Google to find free tutorials? There are a ton of user registration tutorials and general PHP/MySQL/database normalization tutorials out there. I'd start with those.
-
Well, your second example is essentially just a wrapper to a function. If you're going to do that, you might as well just access the function directly. OO doesn't mean "stuff everything into an object." The whole point is to hide how things are implemented behind a clear, concise interface in order to promote modular, extensible, reusable code. The interface remains the same, allowing the underlying bits to be modified without completely breaking the system. In your specific email case, I'd only use an object if you need to maintain that list of recipients and keep them associated with the rest of the mail details (subject, body). If it's a one-shot deal, and no other objects need to pass that mail info around, then I'd just use the built-in function without an object wrapper.