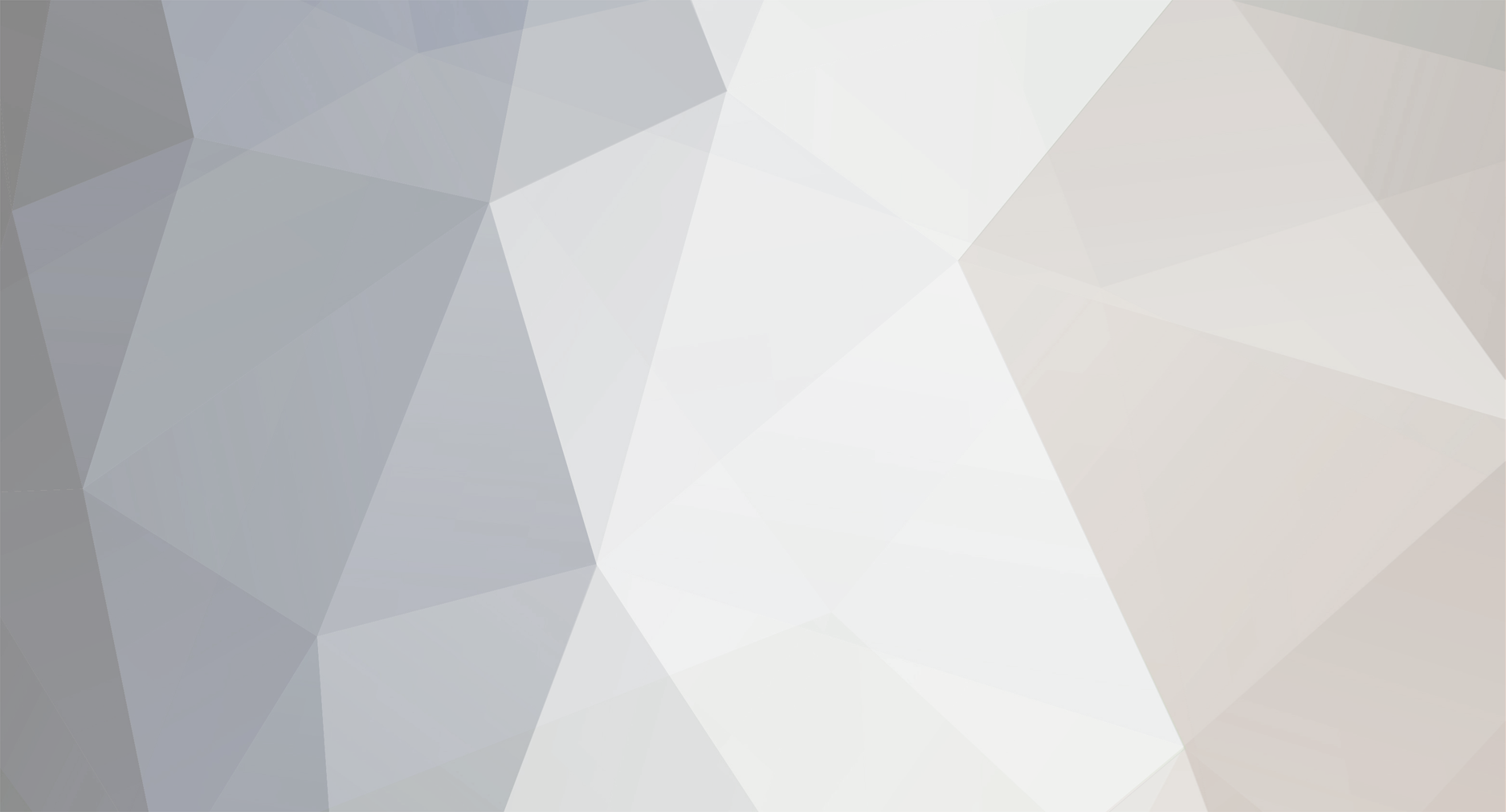
KevinM1
Moderators-
Posts
5,222 -
Joined
-
Last visited
-
Days Won
26
Everything posted by KevinM1
-
I don't see why there'd be much of a difference between using a div as a toggle switch and using a radio button. The process is essentially the same: Click the toggle (div/radio button) Check to see which toggle was clicked Display/hide proper div. Have you tried simply substituting a radio input for the header div while keeping the id the same?
-
[SOLVED] Collapsing a div with a radio button
KevinM1 replied to Darkmatter5's topic in Javascript Help
Assuming that the only radio buttons on your page will be used to toggle divs, I've come up with the following: <html> <head> <title>Blah</title> <script type="text/javascript"> window.onload = function() { var inputs = document.getElementsByTagName('input'); var radios = new Array(); var divs = new Array(); for(var i = 0; i < inputs.length; i++) { if(inputs[i].type == "radio") { radios.push(inputs[i]); } } for(var j = 0; j < radios.length; j++) { radios[j].onclick = function() { divs[j] = document.getElementById(this.value); divs[j].style.display = (divs[j].style.display == 'none') ? 'block' : 'none'; } } } </script> </head> <body> <form> <div id="sections" style="float: left; width: 100px;"> <input type="radio" name="togglesecs" value="buildings" />Buildings<br> <input type="radio" name="togglesecs" value="units" />Units<br> <input type="radio" name="togglesecs" value="units1" />Units1<br> <input type="radio" name="togglesecs" value="units2" />Units2 </div> <div id="buildings" style="float: left; width: 150px; border-left: 1px gray dashed; display:none;"> <input type="radio" name="toggledivs" value="bldlvl1" />Level 1 buildings<br> <input type="radio" name="toggledivs" value="bldlvl2" />Level 2 buildings<br> <input type="radio" name="toggledivs" value="bldlvl3" />Level 3 buildings<br> <input type="radio" name="toggledivs" value="bldlvl4" />Level 4 buildings<br> <input type="radio" name="toggledivs" value="bldlvl5" />Level 5 buildings<br> <input type="radio" name="toggledivs" value="bldlvl6" />Level 6 buildings<br> <input type="radio" name="toggledivs" value="bldlvl7" />Level 7 buildings<br> <input type="radio" name="toggledivs" value="bldlvl8" />Level 8 buildings<br> <input type="radio" name="toggledivs" value="bldlvl9" />Level 9 buildings<br> <input type="radio" name="toggledivs" value="bldlvl10" />Level 10 buildings </div> <div id="units" style="float: left; width: 150px; border-left: 1px gray dashed; display: none;"> More stuff here </div> </form> </body> </html> It works in FF 3*. Haven't tried it in IE. Note that you don't need an inline function invocation in the markup. Simply create a radio button whose value is the id of the div it should toggle, and that div itself, and it'll work. *The first two radio buttons work. The others throw an error because their respective divs aren't actually written in the markup. -
Hmmm... not sure the Oxford dictionary (or any other for that matter) would recognize that.. but I understand it, and I like it [jotting this entry down in my Danielism Dictionary :-D ] Indeed, it's a perfectly cromulent word.
-
[SOLVED] Need Function to Work With Multiple Forms
KevinM1 replied to limitphp's topic in Javascript Help
Use something along the lines of: function myFunc(num) { document.forms["frmPlaylist" + num].elements["newName"].value = "blah"; } -
I haven't tried my hand at creating custom controls. So far, the pre-existing controls have been more than adequate at filling my needs. For C#, the biggest thing to remember is that everything is an object. That, and type is actually enforced. So you have to keep track of your object relationships, specifically the is-a relationships - is object1 a object2 as well? Also, polymorphism works in two ways: public class ParentObject { private int id; private string name; public ParentObject(int id, string name) { this.id = id; this.name = name; } } public class ChildObject : ParentObject { private string msg = "This is a private message"; public ChildObject(int id, string name) : base(id, name){ } public string MSG { get { return this.msg; } set { this.msg = value; } } } /* main code */ ParentObject parent = new ChildObject(1, "Bubba"); //<-- perfectly legitimate parent.MSG = "Hello Wisconsin!"; //<-- error, because parent doesn't have that property For ASP.NET itself, I think the most important thing to learn is the Page lifecycle and the event model. Other than that, it's pretty straight forward.
-
It's obvious you've never tried .NET. Your ignorance on the subject speaks volumes. Does .NET make things easier for the programmer because of its large library of code? Of course. But how is that different than using PEAR? Or even one of the many PHP frameworks? Even C++ has its own standard library. Yes, the Visual Studio IDE has a design view. How is that any different than using something like Dreamweaver? One could easily say that it's also like using a paint program, given that you can drag and drop elements right onto the screen. And I haven't had many troubles with the free version of Visual Web Developer. It does hang occasionally, when first loading a project or opening a database table, but 90% of the time it's just as fast as Notepad++. And I don't have a super computer. And, have you actually tried C#? It sounds like you haven't. .NET is plenty stable. Take a look here: http://www.ektron.com/customers/ Ektron is a local company that created a very flexible CMS in .NET/C#. I think that both the quantity and quality of their customers show that .NET is a viable solution. So, yeah...you don't know what you're talking about.
-
PHP is a good place to start, but you should also try your hand at other languages/technologies to make yourself more marketable. Java, Perl, .NET with C#.... Regardless of whether you stick with PHP or not, you'll need a good grasp of JavaScript. As far as a degree goes, corbin is correct - there are no PHP degrees. That said, most technical colleges have Associate Degree (2 year) programs that focus on web development. Their courses run the gamut from image creation/editing (Photoshop) to Flash to back end programming (PHP and whatnot). If you want to do more, then a full-fledged Bachelor's Degree in computer science may be the way to go. Keep in mind that most colleges and universities consider a CS degree an engineering degree, so you'll be forced to take several math classes (calculus+) and a year of physics. Other science classes may be required as well, such as electrical engineering. And that's in addition to at least one programming class per semester, and trying to cram in all the general education requirements as well. It's not for the timid. I hope this helps.
-
Public: can be invoked by code outside of the class itself. Private: can only be invoked within the class. Example: class Example { private $SSN; //Social Security Number...it shouldn't be freely available to the rest of the system private $name; public function __construct($SSN, $name) { $this->SSN = $SSN; $this->name = $name; } public function getSSN() { return $this->SSN; } public function getName() { return $this->name; } public function setName($name) { $this->name = $name; } } So, let's say the main code is: $example = new Example("000-00-0000", "John Doe"); $example->SSN = "123-45-6789"; //<-- ERROR $mySSN = $example->SSN; //<-- ERROR $example->name = "Angelina Jolie"; //<-- ERROR $myName = $example->name; //<-- ERROR $mySSN = $example->getSSN(); //<-- SUCCESS! $example->setName("Angelina Jolie"); //<-- SUCCESS! $myName = $example->getName(); //<-- SUCCESS! So, you're probably wondering what the point is. One of the core tenets of OOP is of encapsulation. Objects should encapsulate data. This data should only be accessed through clear lines of communication to ensure that no ambiguity or errors creep into the data itself. To put it bluntly, it's dangerous to keep things publicly accessible because you're not guaranteed that the data is safe. By forcing your (or another's) code to use these clearly defined rules of communication, you help ensure that the object is used in the proper way. The same applies to private methods, as well. These tend to be bookkeeping methods, or methods that do a lot of the grunt work that the rest of the public world shouldn't concern itself with.
-
You're trying to re-design a site's layout, but you don't know CSS? Good lord.... CSS stands for Cascading Style Sheets. It's the technology standard for formatting HTML documents. Like HTML, CSS is simply text that the browser reads. This text is essentially a bunch of formatting rules in order to modify the look and feel of HTML elements. Instead of using horribly outdated code, like <font> tags or what not, all of the formatting is placed in an external CSS file that the HTML file loads. There are three main selectors that one can use in order to specify what element(s) they want to format: 1. Tag name. The following code will turn the text of ALL paragraph tags (<p>) red: p { color: red; } 2. Unique id. If you give an element a unique id, then you can format just that one element: #firstName { font-weight: bold; } All id's begin with a '#' character within the CSS. In order for this to work in the HTML document, there needs to be an element with that id: <span id="firstName">Bubba</span> 3. Class name. You can group elements together by making them a member of a class. This is simple to do: .bookTitle { font-style: italic; } Classes are denoted by the '.' character in the CSS. In order for this rule to be applied to HTML elements, you must give them a class attribute: <span class="bookTitle">Pro JavaScript Techniques</span> by John Resig <br /> <span class="bookTitle">PHP For the World Wide Web: 3rd Edition</span> by Larry Ullman Where do these rules go? The simplest thing to do is create a .css file and put them all there. Then, within the <head> element of your HTML document, you can simply write: <link rel="stylesheet" type="text/css" href="styles.css" /> So long as you have a file named 'styles.css', and it's in the same directory as your HTML document, it'll all link up.
-
You essentially want a sticky form. Something like: <?php if(isset($_POST['submit'])) { if(isset($_POST['in1'])) { $in1 = $_POST['in1']; } /* validate and assign the other inputs */ if(/* inputs all are valid */) { /* redirect the user to the file download page */ } else { /* errors - generate error output, and store in a variable */ } } else //form not submitted, or submitted with bad values { if(/* error output variable exists */) { /* output error */ } ?> <!-- HTML FORM --> <form name="myForm" action="<?php echo $_SERVER['PHP_SELF']; ?>" method="post"> <input type="text" name="in1" value="<?php if(isset($in1)) { echo $in1; } ?> /> <!-- repeat with other inputs --> </form> <?php } //end else ?>
-
Onmouseover and onmouseout not working???
KevinM1 replied to Skipjackrick's topic in Javascript Help
Your JavaScript created images and the images within your HTML are not the same thing. Look at your code. You explicitly create new images within your JavaScript. These images, despite having a source, aren't displayed. Instead, you recreate them within your HTML. Your JavaScript most likely is swapping the values of the src attribute. The problem is that it's doing it to the wrong images. Instead, you should do something like: <script type="text/javascript"> window.onload = function() //<-- ensures that the script won't run until the entire HTML document is made { var image1 = document.images["image1"]; //<-- don't need to specify the src...simply use the one originally given in the HTML var image2 = document.images["image2"]; /* ... */ } </script> Another solution is to forget using JavaScript for rollovers and simply use CSS. You can tie image movement to CSS' a:hover pseudo-element. For more info, do a google search for CSS rollovers. -
Why are you using a span for the dropdown? A span's default display is inline. You should be using a div instead, as it's a block element by default. From what I can see, your dropdown images are wider than your table cell. You specify that the images should be 170px wide, but the cell is only specified to be 93px wide. This is breaking your layout. The 'Countries' image is moving up because you set the display to none, then to block. Setting an element's display to none takes it out of the document flow completely. Instead of using the display property, use the visibility property. Have it set to hidden as the default, and visible during the mouse event. When attempting to create a toggled element (in this case, your dropdown menu), it's best to create the layout with the element visible, at least during the planning stages. That way, you can see how things look when in the activated state. Once that's all set to your liking, then you can hide the element and write the JavaScript to display it during a mouse event. I hope this helps.
-
I haven't found any books on the subject myself. With that said, the best thing to keep in mind is to resist the temptation to switch between PHP and HTML a lot in your files. Given the PHP file's lifecycle, it's best to put all request handling and data processing at the beginning of the file, building your output as you go. Then, only at the end, should you actually render it to the screen. I say this because it's a common problem I've encountered with other people on here. Switching between PHP and HTML on the fly is tempting because it gives fast results, especially when looping through db results that need to be placed in tables. Unfortunately, problems arise when other considerations enter the picture - JavaScript, if-else conditionals, etc. The code tends to get messy and hard to maintain. So, self-discipline is more or less the key. You should probably check out PHP's OOP capabilities. They're not as potent as, say, C#'s, but they're useful. I'm hoping that you're learning PHP 5+. PHP 4's attempt at OOP was pretty laughable.
-
Need to change select options to buttons (with JS form expand)
KevinM1 replied to richrock's topic in Javascript Help
Well, you could still do it with the select input. The problem you had with that attempt was that you didn't grab the selected index of the select. You need that value for anything to work. Not only that, but you don't need the 'javascript:' pseudo-attribute to get it to work. So, something like: <script type="text/javascript"> window.onload = function() { var accType = document.getElementById('acctype'); accType.onchange = function() { var selIndex = this.selectedIndex; if(selIndex != 0) //make sure you're not trying to expand the menu with the non-value option { ShowMenu(this.options[selIndex].value, 'divColor', 4); } } } </script> <!-- ... --> <select id="acctype" name="acctype" mosReq="1" mosLabel="Account Type"> <option value="">Select Account Type</option> <option value="1">Candidate</option> <option value="2">Employer</option> <option value="3">Recruiter</option> <option value="4">Referrer</option> </select> If you still want to do it by buttons, then the first thing you need to remember is that an id is supposed to be unique. It shouldn't be applied to more than one element. That's one of the reasons why your version wasn't working - you use the same id for each button. Also, once again, you don't need the 'javascript:' pseudo-attribute. That should only be used if you're dealing with a link's href attribute. So, the buttons... <script type="text/javascript"> window.onload = function() { /* NOTE: this code assumes that these buttons are the only buttons on your page */ var buttons = document.getElementsByTagName('button'); for(var i = 0; i < buttons.length; i++) { buttons[i].onclick = function() { ShowMenu(this.value, 'divColor' + (i + 1), 4); } } } </script> <!-- ... --> <button value="Candidate" mosReq="1" mosLabel="Account Type">Candidate</button> <button value="Employer" mosReq="1" mosLabel="Account Type">Employer</button> <button value="Recruiter" mosReq="1" mosLabel="Account Type">Recruiter</button> <button value="Referrer" mosReq="1" mosLabel="Account Type">Referrer</button> Keep in mind that I haven't tested any of this code. With that said, it should at least help you get on the right track. -
A couple things popped out at me after a quick glance: 1. There's a typo in your preloadImages() function. In your for-loop, you have 'var i=o'. You need it to be 'var i=0.' 2. It looks like your preloadArray variable is going out of scope. You declare it and populate it in the preloadImages() function, but after that function ends, it will go away. You should declare it in the global scope as an empty array (i.e., var preloadArray = new Array(); ), then fill it in the preloadImages() function. That way, the preloaded images will always be available. Are you calling displayMenu() anywhere? I can't see it in your HTML.
-
I've said it before, and I'll say it again: I'm not a big fan of w3schools for the new learner. Why? Because it merely teaches syntax in a vacuum. There's nothing about thinking how to structure code properly, nothing about best practices, or any of that. Syntax is only part of the equation. I mean, how many horribly designed code snippets have we all seen on here? How many failures are a result of shoddy logic rather than syntax? If you're going to learn PHP as a career move, you should invest in it early. Getting a good introductory book is a good way to go. You'll find that the financial cost is mitigated by the lack of frustration and wasted time you'd face by going the w3schools/tutorials route. I recommend Larry Ullman's Visual Quickstart Guide (http://www.amazon.com/World-Third-Visual-QuickStart-Guide/dp/0321442490/ref=sr_1_1?ie=UTF8&s=books&qid=1234217850&sr=1-1). He writes in a clear, easy to understand style. And, even better, his code works. I also can't say enough about this forum. It's the best free PHP resource available. Even if you're not directly involved with certain threads, trying to create your own solution to the problems posted here is a great way to practice.
-
It worked that way for my Xbox 360 version. After the game update, you'll need to go into RB1's options and choose 'Export Songs', or something similar. Keep in mind, there's an additional $4 fee in order to export the songs. After that, they'll be available in RB2.
-
*sigh* You kids today.... They were characters in the original Transformers cartoon/toyline of the 1980's. You know... Optimus Prime? Starscream? Megatron? http://www.youtube.com/watch?v=CccbBwyDAdk When I was a kid, the Transformers were more or less my religion, along with Voltron and Robotech.
-
Would I be a nerd if I said I still remember that Sideswipe and Sunstreaker were brothers? Or that Skywarp's power was teleportation? Or just a loser?
-
Unfortunately, I haven't found any real decent online sources that cover what I'd consider best JavaScript practices. At least, no one source. A few good sources are QuirksMode and Dustin Diaz's blog (a google search for each should yield the desired result). W3schools leaves a bad taste in my mouth. It's decent as a "What was the name of that function?" resource, but it shouldn't be used as an actual primer on any language. Unfortunately, many people do tend to use it as their primary learning source, most likely because they haven't found anything better. Your best bet is to get a good book or two. I always recommend John Resig's Pro JavaScript Techniques, but it may be too advanced for you at this stage. I started with Danny Goodman's JavaScript and DHTML Cookbook. It's not great, but it teaches the basics. It may be a bit outdated, now, though. A lot of it is focused on getting older browsers to behave properly. I'm not sure it that's much of a concern any more. Unless you're working for a charity or government site, I doubt you'd want to jump through the hoops necessary to get things working correctly in, say, IE 5.
-
JavaScript structure - a guide to better scripting
KevinM1 replied to KevinM1's topic in Javascript Help
I actually like the combination of using a custom attribute followed by a delegate. It makes things semantically clear while keeping the script separate from the markup. <script type="text/javascript"> window.onload = function() { var myButtons = document.getElementsByTagNames('button'); //assuming they're the only buttons we want..used here because I'm a lazy typist for(var i = 0; i < myButtons.length; i++) { myButtons[i].onclick = function() { executeCommand(this); } } function executeCommand(button) { switch(button.cmd) { case "something": /* do something */ break; case "another thing": /* do another thing */ break; /* etc */ } } } </script> <!-- generic HTML here --> <button cmd="something">Do something</button> <button cmd="another thing">Do another thing</button> The only fishy 'smell' I'm getting is wondering whether or not that would validate. That said, this is pretty much how ASP.NET solves this problem. Its Button server control has an attribute aptly named CommandName that does the same thing as shown above. I know that mentioning .NET is taboo on a PHP board, but if it works, why not? -
Still vague.... I need to see code in order to help you. There could be several issues at play here, but without seeing exactly how you've written things, I can't diagnose the problem(s) nor suggest a cure.
-
Hopefully this will get stickied. Being a member of this board for a couple years, I've noticed a trend with those seeking JavaScript help. Most people, despite their good intentions, are unaware of the best way to structure their scripts. This leads to convoluted code, and even errors, which can stymie the best script writer. It's not their fault - the internet is filled with horrible tutorials based on outdated methodolgy and free scripts that are, literally, worthless. Attempting to learn from these sources tends to cause confusion rather than reduce it. Properly structured JavaScript is easy to write, easy to maintain, and easy to debug. It allows the developer to gracefully add features to their site while ensuring that the core content is available to all. So, with that said, let us begin. A website is typically developed and arranged in tiers: There's the server-side processing that handles requests and processes forms. It stores and retrieves values from the database. It even serves up the proper page to the user itself. Next, there's the actual HTML document. Regardless of where it came from, and what server-side black magic was used to create the document, this is the one constant for all sites. Finally, there's the client-side processing - JavaScript. Used correctly, JavaScript is bolted on top of the HTML, facilitating greater user interaction and feedback. It adds dynamism to what would otherwise be a static presentation. When looked through the lens of designing websites in tiers, it becomes apparent that these tiers, these layers should have clearly defined boundaries. They should lay on top of one another, and talk with each other through specific lines of communication. They should not be mixed haphazardly. HTML writers are already familiar with this thought process. After all, this kind of separation of duties is what resulted in CSS being developed. One is for structure, the other for formatting, and both are at their most useful when separated from each other. The same applies to JavaScript. How is this separation achieved? By using what is commonly termed unobtrusive JavaScript. It's a unique term that means a simple premise - there should be NO JavaScript code embedded in HTML code, with the exception of the <script> tags. Even inline event handlers are not allowed. Why? Because embedding JavaScript within HTML creates coupling. The two become so tied together that an edit in one requires an edit in the other. Not only that, but it muddies which responsibility lies with which component. Both of these, in turn, create code that is difficult to debug and maintain. Even inline event handlers create unnecessary ties to the HTML document. There's no reason to go down that road if a better alternative can be found. Thankfully, JavaScript itself gives developers a couple different ways to grab a hold of HTML elements: getElementById(), and getElementsByTagName(). With these two tools, the developer can obtain any element within their HTML document. So, with all that said, how is a proper JavaScript script structued? The first step is to ensure that the script won't be executed until the HTML is fully loaded. This is necessary, as JavaScript tends to be processed by the browser before the HTML is fully rendered, which makes it impossible for the script to obtain all of the HTML element references it needs in order to work. This is one of the primary causes for things being 'undefined' in a script. These elements are undefined because they don't actually exist when the JavaScript code attempts to access them. Fixing this is, thankfully, a simple matter: <script type="text/javascript"> window.onload = function() { /* script goes here */ } </script> The code won't fire until the document is fully loaded. What's next? Now, it's time to get a hold of the elements that need to be accessed by using one of the built-in JavaScript selectors: <script type="text/javascript"> window.onload = function() { var myButton = document.getElementById('myButton'); var mySpans = document.getElementsByTagName('span'); /* more script goes here */ } </script> Elements are stored within variables for two main reasons: 1. It gives the developer an easy way to access the element. Accessing myButton is far quicker than using getElementById() every time one wants to access that element. It saves typing, and improves readablity. 2. It's more efficient code. Rather than forcing JavaScript to traverse through multiple elements to find the right id every time one wants to access the element, a direct reference to the element is stored after the initial successful search. Now, let's do something with these elements: <script type="text/javascript"> window.onload = function() { var myButton = document.getElementById('myButton'); var mySpans = document.getElementsByTagName('span'); myButton.onclick = function() { for(var i = 0; i < mySpans.length; i++) { mySpans[i].style.color = 'red'; } } } </script> As you can see, adding an event handler is trivial. One could also use a named function: <script type="text/javascript"> window.onload = function() { var myButton = document.getElementById('myButton'); var mySpans = document.getElementsByTagName('span'); myButton.onclick = turnToRed; function turnToRed() { for(var i = 0; i < mySpans.length; i++) { mySpans[i].style.color = 'red'; } } } </script> The finalized code would look something like: <html> <head> <title>Unobtrusive JavaScript Example</title> <script type="text/javascript"> window.onload = function() { var myButton = document.getElementById('myButton'); var mySpans = document.getElementsByTagName('span'); myButton.onclick = function() { for(var i = 0; i < mySpans.length; i++) { mySpans[i].style.color = 'red'; } } } </script> </head> <body> <span>This sentence will turn red.</span><span> As will this one.</span> <br /><br /> <button id="myButton">Click</button> </body> </html> Admittedly, it's not the most interesting HTML document. But it serves its purpose as a simple example that unobtrusive JavaScript can work. Isn't that a lot of code to do something so simple? Yes, it is. Unobtrusive JavaScript tends to suffer when it comes to writing trivial code. There's a lot of work to do up-front in order to create that wedge between the script and the markup. In larger projects, however, unobtrusive techniques shine, especially when it comes to adding the same event handler to multiple elements. Beyond that, though, unobtrusive techniques just work. Given the amount of time people tend to spend beating their heads against the wall while trying to fix their meshed code, it seems that the extra few minutes writing in an unobtrusive manner more than makes up for the frustration. In the end, the entire point comes down to doing things right the first time. Writing scripts with structure in mind may not automatically ensure success, but it definitely helps. I hope this helps some people. If anyone is more curious about this, read through my post history. The code there is most likely far more illuminating than my canned example above.
-
Sorry, but I need to see more. Where do num's 1, 2, 3, and 4 come from? Where are these functions located? Are they merely between <script> tags? Is there any more context you can give me? EDIT: You should probably be doing something like: document.getElementById('total2').innerHTML = "$" + total2; Rather than trying to use the value attribute.