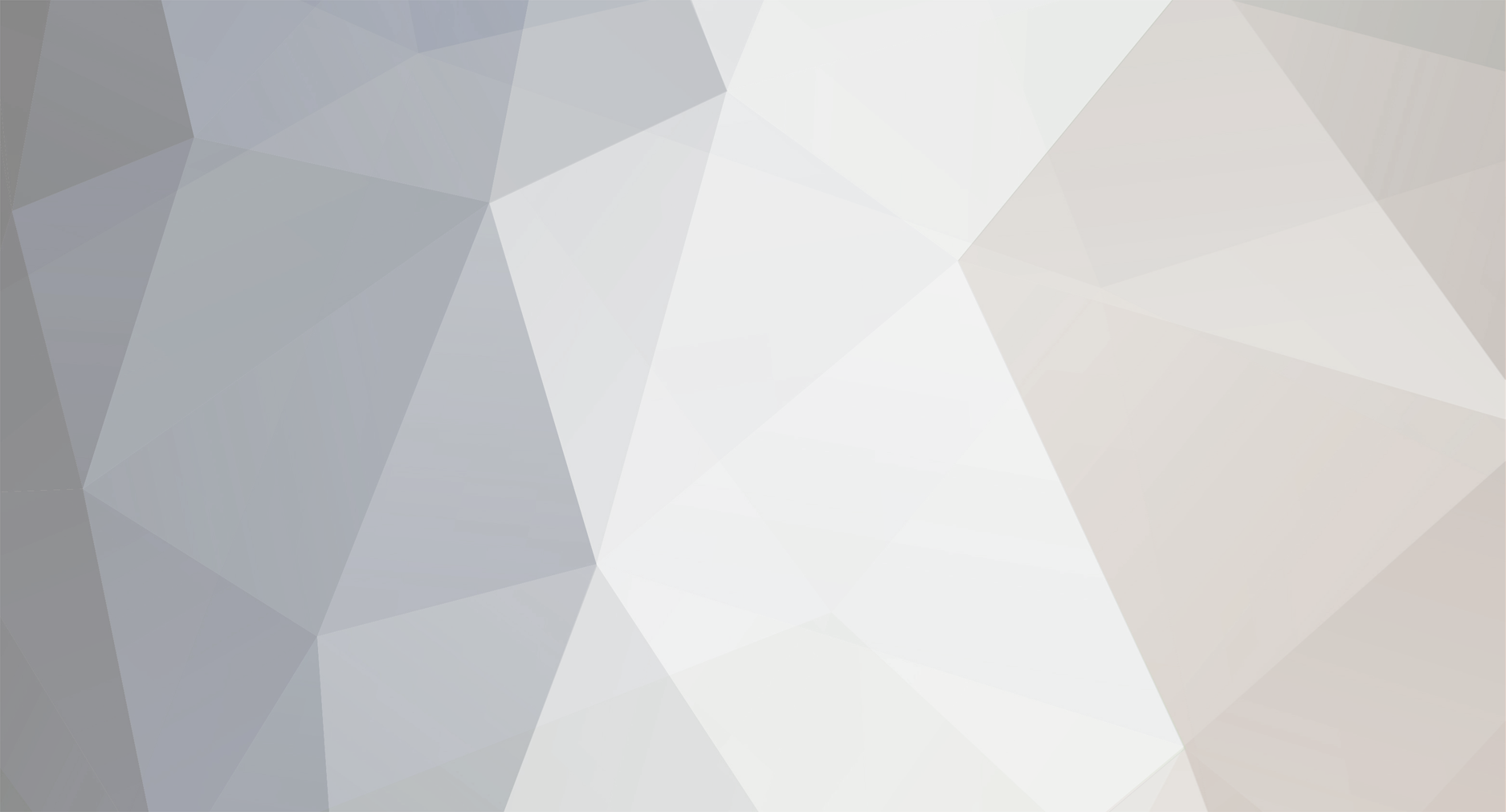
KevinM1
Moderators-
Posts
5,222 -
Joined
-
Last visited
-
Days Won
26
Everything posted by KevinM1
-
It's probably do to you not waiting for the HTML to be finished rendering before your script tries to get the necessary element references. But, I can't tell for sure until you show more code. Specifically, how/where the checkBothTotals() function is called.
-
If it's a form field, attaching an event handler that resets the default search value to onblur should do the trick.
-
Price * Quantity input field, and auto update subtotal on onkeyup???
KevinM1 replied to Presto-X's topic in Javascript Help
I need to know how to grab the prices from the actual HTML. Knowing the PHP variables that they inhabit isn't useful, due to the way a web page is constructed. It goes: Backend processing (PHP) -> rendered page (HTML) -> dynamic behavior (JavaScript) So, what is stored in $price, exactly? Is it merely text? Are there any HTML elements that could hold the price, like a hidden input or something? -
Price * Quantity input field, and auto update subtotal on onkeyup???
KevinM1 replied to Presto-X's topic in Javascript Help
Need to know a couple of things: 1. Where, in the HTML, are the item prices located? Can't update a price without being able to grab the price of one product unit. 2. Which element will display the updated price? -
Well, there are several things going on here, none of them good, I'm afraid. First, I don't see why you're trying to create the rest of the form line-by-line in your JavaScript. It seems as though you want a 'button' (I'm using the term loosely...it could be any element) to toggle the display of that part of the form. Am I correct in that assumption? If so, there's a much easier way to do it. Secondly, your function calls aren't making any sense. In particular, you're attempting to set an element's HTML to another element's onclick handler...it's a sloppy way to do things, regardless of whether or not the quotes are right. Third, from an HTML point of view, you're putting block elements (<p>, <textarea>) within a inline element (<span>). That kind of defeats the point. I have the feeling you want something like: <script type="text/javascript"> window.onload = function() { var firstToggle = document.getElementById('firstToggle'); var firstQuestion = document.getElementById('firstQuestion'); firstToggle.onclick = function() { firstQuestion.style.display = (firstQuestion.style.display != 'none' ? 'none' : ''); //<--- two single quotes } } </script> <!-- in your HTML --> <form name="questions" action="#" method="post"> <h3 id="firstToggle">FIRST QUESTION:</h3> <div id="firstQuestion"> <p>Question:</p> <textarea name="question" rows="4" cols="80"></textarea> <p>Answer:</p> <textarea name="answer" rows="4" cols="80"></textarea><br /> <input type="submit" name="submit" value="Submit" /> <input type="submit" name="cancel" value="Cancel" /> </div> </form> I've taken the liberty of fixing the several HTML errors you had, as well.
-
I haven't read JavaScript Bible at all. If it's like the other Bible books I do have (Ubuntu, and Ajax), it may be a bit hit or miss. I learned JavaScript through two books: JavaScript & DHTML Cookbook by Danny Goodman. It's a decent primer for beginners, and how to start thinking in JavaScript. Pro JavaScript Techniques by John Resig. I love this book. I consider it to be the best book on JavaScript available. Regarding browser differences, a good rule of thumb is to ignore older browsers (IE 5 and whatnot). Instead, code for what Yahoo terms as 'A-grade' and the equivalent 'X-grade' browsers, such as FireFox 3 for Linux or Google Chrome (http://developer.yahoo.com/yui/articles/gbs/index.html). These are all considered to be modern browsers, and are what you should focus on when coding. There are still differences between even the modern browsers, but in my experience the kinks really only show themselves during testing. I haven't yet found a centralized place describing all the 'gotchas' that can show up when developing for modern browsers. Just be sure to test your code on different browsers, with IE and FireFox being the two key browsers. And burn your 'For Dummies' book. Those books all tend to suck. Not worth the paper they're printed on, IMO.
-
Are there any good, free Entity Relationship Diagram programs out there for Vista? My Google-fu leads me to freeware projects of questionable reliability and diagrams that look pretty craptacular. I just want a tool that I can use to help me plot out my database designs. Something I can leave up on the screen and refer to as I work on my apps.
-
I had this long reply all typed up, but as I re-read it, and saw all the caveats ("well, except for AJAX...except for animation...except for cross-browser issues...."), I realized that, yes, I was talking out of my ass. Not the first time, and probably won't be the last. Has anyone tried 1.3.1 yet? I'm still using 1.2.6, but am more than willing to upgrade if most of the bugs have been squashed.
-
I love jQuery. That said, I think that all developers should learn the basics of JavaScript. It's really not that hard. Hell the basic structure of using jQuery is the same as well-formed unobtrusive JavaScript. Vanilla JS: <script type="text/javascript"> window.onload = function() { var myButton = document.getElementById("myButton"); var myDiv = document.getElementById("myDiv"); myButton.onclick = function() { myDiv.innerHTML = "Button has been clicked"; } } </script> Using jQuery: <script type="text/javascript"> $(document).ready(function() { $("#myButton").click(function() { $("#myDiv").html("Button has been clicked"); }); }); </script> The only appreciable difference between the two is that jQuery allows you to select elements and manipulate them all in the same step. That's why I get baffled when people that can use jQuery just fine have issues with vanilla JavaScript - they should be written in the same manner.
-
Your right. and i never claimed to know the workings. but compiling the program was work enough in itself.....thats what open source is for REMEMBER!.....allows ppl to build off other scripts to make new programs. But getting back to my original point, anyone wanting to really test if i can decrypt zend files can post an attachment and ill decrypt it........now thats impressive! Peace homie You said you split its head. I don't consider compiling a program some ninja-hackery. I must be a goddamn Jedi-ninja-pirate master serving the wiles of Chuck Norris given how many times I've compiled C++ programs and my .NET projects. Phear my kewl h4x!
-
Well, I'm happy I was of some help. This little endeavor reminded me why I hate dealing with pop-ups.
-
You should remove 'myform.submit();' from the first function. You don't want the form to be submitted during the preview stage. Simply having a 'return false;' at the end should suffice. I don't think you need to change the form's action there, either, since you're not submitting the form at that time. You'll need to link the buttons to their respective functions. Assuming these are generic submit inputs, you can do something like: var formPreview = myform.elements["preview"]; //assuming the input is named 'preview' var formSubmit = myform.elements["submit"]; /* function definitions for OnButton1 and OnButton2 go here */ formPreview.onclick = OnButton1; formSubmit.onclick = OnButton2;
-
Hi, sorry for the delay. You have to remember that JavaScript is client side, and is executed before PHP. So, assuming you create a second 'Preview' button (which is probably the best way to go), POST data won't be sent to the pop-up. Why? Because the pop-up will be generated through onclick rather than onsubmit. Instead, the pop-up window's values will simply be populated by the parent window's form field values (i.e., document.forms["myForm"].elements["cf_name"].value). It can be confusing, since you can use HTML already stored on your server to act as the pop-up page. The thing to remember is that the JavaScript in the parent window is merely modifying the pop-up's output after that HTML has been loaded. It's not actually modifying the markup itself. I believe you can have the child/pop-up window modify things in the parent window. I'm not entirely sure how to do it, as I tend not to use pop-ups myself. You'd probably need to write a separate JavaScript script for the pop-up page which detects whether or not it was opened by a script itself, then have it write back to the parent window. This brings me to a larger question: Are you sure you want to use a pop-up for this? Many users have pop-up blockers, so you're not guaranteed that the pop-up will always be available. You might be better served to create an intermediary PHP script that will preview the finalized form info.
-
Well, there's two main ways to do it - to have the main script create a table (or another form) in the pop-up window dynamically, or to create a basic pop-up window page, then have the main script open a window containing that page, which it can then send the form info to. The second option is the easier of the two. Basically, you need to tell the form that rather than submit the info to the server upon submission, it needs to open up that pop-up window. In order to do that, you must write a function that hooks into the form's submit event. This is easy to do, provided you give your form a name. In the code below, I've given your form the name 'myForm. <html> <head> <title>blah</title> <script type="text/javascript"> window.onload = function() // <-- this line assures us that our script won't load until after the HTML { var myForm = document.forms["myForm"]; myForm.onsubmit = function() // <-- this creates a function tied to the form's submit event { var popup = window.open('http://www.mypopupurl.com', 'popup'); /* <-- this creates the popup. do a google search on javascript window.open to see all of the options available to you */ /* you can now populate the other window with values from the form. the code below assumes that you created a div in the popup window with an id of 'results' */ var results = popup.document.getElementById('results'); results.innerHTML = "Band name: " + this.elements["cf_band"].value + "<br />"; return false; // <-- this stops the form on the original page from being submitted } } </script> </head> Keep in mind that the code above isn't intended to be the entire solution to your problem. Instead, it's basically an outline the overall direction you should go in. A lot depends on how you structure the HTML that forms the pop-up page. The other component is actually submitting the values if the user agrees that everything is okay. So, you'll need to figure out a way to send the info from the pop-up page. You could recreate the form, or pass the values from the main page to the pop-up, and store them in hidden input fields. Either way, you'll need two buttons - one to submit the info (a normal form submit input), and one to close the current window, so the user can try again on the main page. Let me know if you need help with that, too.
-
First of all, this is a JavaScript validator you're trying to write, not a PHP one. Secondly, you put "return checkForm(this)" in the HTML of the form, but your function doesn't accept an argument (i.e., it's written as "checkForm(){ /*... */}"). Third, where does $company come from? If it's supposed to be from the PHP script, then, put simply, you're doing it wrong. JavaScript execution always occurs before the page is reloaded, and the form values are sent to the PHP script. So, right now, $company = '' If $company is supposed to be supplied by the form itself to the JavaScript, then, again, you're doing it wrong. You need to grab a hold of that form field, then retrieve its value. Here's the best solution, assuming your validator is JavaScript-only: <html> <head> <title>blah</title> <script type="text/javascript"> window.onload = function() { var myForm = document.forms['myForm']; myForm.onsubmit = function() { if(this.elements['company'].value == '') { alert("Please enter company name"); return false; } else { return true; } } } </script> </head> <body> <form name="myForm" action="post.php" method="POST"> <table align=center width="575" border=0 cellspacing=10> <tr> <td align=right><b>Name of Company</b> </td> <td><input type="text" name="company" size="50"> </td> </tr> <tr> <td align=center colspan="2"><input type="submit" name="Submit" value="Submit Form"> </td> </tr> </table> </form> </body> </html>
-
I learned JavaScript from two books: The JavaScript and DHTML Cookbook by Danny Goodman (http://www.amazon.com/JavaScript-DHTML-Cookbook-Danny-Goodman/dp/0596514085/ref=sr_1_1?ie=UTF8&s=books&qid=1231850230&sr=1-1). It's a decent primer on the basics, but some of his event handling leaves a lot to be desired. Still, if you need to grasp the basics, this is a good place to start. The other book was Pro JavaScript Techniques by John Resig (http://www.amazon.com/Pro-JavaScript-Techniques-John-Resig/dp/1590597273/ref=sr_1_1?ie=UTF8&s=books&qid=1231850340&sr=1-1). Resig is the man who developed the jQuery framework, so he definitely knows what he's doing. This book was nothing short of a revelation to me. Once you get the basics down, this should be the book you use. It's my JavaScript bible.
-
Hate to tell you, but the dropdowns aren't working with FireFox 3, either. At least, if they're supposed to be on the site you linked to in your first message. You're attempting to use a 3rd party, pre-written solution, correct? From what I can tell, from both this thread and the other one, it's a junk script. You'd be better off starting from scratch. I used a simple dropdown solution in one of my sights called Son of Suckerfish. It's a simple CSS and JavaScript method of creating dropdowns. The JavaScript is simply: // This code is to be used in conjunction with the Son of Suckerfish // navigation menu system. This code was provided by HTMLDog // (http://www.htmldog.com/articles/suckerfish/dropdowns/). sfHover = function() { var sfEls = document.getElementById("nav").getElementsByTagName("LI"); for (var i=0; i0? " ": "") "sfhover"; } sfEls[i].onmouseout=function() { this.className=this.className.replace(new RegExp("( ?|^)sfhover\\b"), ""); } } } mcAccessible = function() { var mcEls = document.getElementById("nav").getElementsByTagName("A"); for (var i=0; i0? " ": "") "sffocus"; //a:focus this.parentNode.className =(this.parentNode.className.length>0? " ": "") "sfhover"; //li < a:focus if(this.parentNode.parentNode.parentNode.nodeName == "LI") { this.parentNode.parentNode.parentNode.className =(this.parentNode.parentNode.parentNode.className.length>0? " ": "") "sfhover"; //li < ul < li < a:focus if(this.parentNode.parentNode.parentNode.parentNode.parentNode.nodeName == "LI") { this.parentNode.parentNode.parentNode.parentNode.parentNode.className =(this.parentNode.parentNode.parentNode.parentNode.parentNode.className.length>0? " ": "") "sfhover"; //li < ul < li < ul < li < a:focus } } } mcEls[i].onblur=function() { this.className=this.className.replace(new RegExp("( ?|^)sffocus\\b"), ""); this.parentNode.className=this.parentNode.className.replace(new RegExp("( ?|^)sfhover\\b"), ""); if(this.parentNode.parentNode.parentNode.nodeName == "LI") { this.parentNode.parentNode.parentNode.className=this.parentNode.parentNode.parentNode.className.replace(new RegExp("( ?|^)sfhover\\b"), ""); if(this.parentNode.parentNode.parentNode.parentNode.parentNode.nodeName == "LI") { this.parentNode.parentNode.parentNode.parentNode.parentNode.className=this.parentNode.parentNode.parentNode.parentNode.parentNode.className.replace(new RegExp("( ?|^)sfhover\\b"), ""); } } } } } // only ie needs the sfHover script. all need the accessibility script... // thanks http://www.brothercake.com/site/resources/scripts/onload/ if(window.addEventListener) window.addEventListener('load', mcAccessible, false); // gecko, safari, konqueror and standard else if(document.addEventListener) document.addEventListener('load', mcAccessible, false); // opera 7 else if(window.attachEvent) { // win/ie window.attachEvent('onload', sfHover); window.attachEvent('onload', mcAccessible); } else { // mac/ie5 if(typeof window.onload == 'function') { var existing = onload; window.onload = function() { existing(); sfHover(); mcAccessible(); } } else { window.onload = function() { sfHover(); mcAccessible(); } } } Put that in an external file and link to it like so: <html> <head> <title>Blah</title> <script type="text/javascript" src="my_dropdown_file.js"></script> </head> The CSS can get a bit more complicated, but is explained here: http://www.htmldog.com/articles/suckerfish/dropdowns/ Ignore the JavaScript there. If memory serves, the script I provided above was the one that everyone figured out to be the best. The original version didn't work for various Mac browsers.
-
I don't see any dropdown menu at the site you provided a link to. In any event, image rollovers are very easy to do, especially if you're using the images as links. The big question, however, is how much JavaScript and CSS do you know?
-
Well, I have the feeling that it boils down to inefficient code. In your original script, you already had access to the image you wanted to change ('this'). But, instead of dealing with it directly, you pass its id to the function (this.id), then have the function retrieve the image element any way (document.getElementById(id)). You were just adding steps that weren't necessary to the actual process. My solution just deals with the images once. There's less processing overhead as you don't need to retrieve the correct image element within the function - it's already there as 'this' (linkImgs) while you iterate through all of the images.
-
Eh, you can do it an easier way: <script type="text/javascript"> window.onload = function() { var nav = document.getElementById('ulnav'); var linkImgs = nav.getElementsByTagName('img'); for(var i = 0; i < linkImgs.length; i++) { linkImgs[i].onmouseover = function() { var tmpSrc = this.src.replace('.jpg', '_over.jpg'); this.src = tmpSrc; } linkImgs[i].onmouseout = function() { var tmpSrc = this.src.replace('_over.jpg', '.jpg'); this.src = tmpSrc; } } } </script> . . . <ul id="ulnav"> <li><h2><a title="Doctors Housing Home" href="http://localhost/doctorshousing.com/site/home"><img alt="Doctors Housing Home" width="58" height="79" id="hnav" src="http://localhost/doctorshousing.com/site/images/nav/home.jpg" /></a></h2></li> <!-- other links go here --> </ul> This should make it easier on your PHP script as well, as you don't need to put a function reference in each image's HTML, as you can see in the example above. Instead, this script iterates over each image and adds mouse hover functions to each one.
-
Do you want the images to revert to their default state on a mouseout? Or are you intentionally keeping them in their hover state until the next mouseover?
-
Yup. That's what I get for rushing. The class should work fine, though, if __connect is changed to __construct.
-
Hmm...mind showing how you're calling this function?
-
The code in here makes me want to cry... Okay, first, why are you using PHP 4? PHP 4 is old, and not even officially supported by Zend any more, to say nothing of its crappy OOP 'features.' If you're trying to use objects, you're much better off using PHP 5. Second, even if your db connection info is located in another file (which is good), you don't want to include it directly into your class file. It's a sloppy technique that leads to coupling, which is a very bad thing in OOP. A better designed class would be: class mysql { private $name; private $user; private $password; private $tableName; private $dbc; public function __connect($name, $user, $password) { $this->dbc = mysql_connect($name, $user, $password); if($this->dbc) { $this->name = $name; $this->user = $user; $this->password = $password; } else { die("There was an error connecting to the database."); } } public function selectTable($tableName) { if($this->dbc) { $this->tableName = mysql_select_db($tableName, $this->dbc); } else { die("Could not connect to the database."); } } public function query($query) { $result = mysql_query($query); //notice it's NOT $this->result... return $result; } public function fetch($result) { if(is_resource($result)) { $fetchedRow = mysql_fetch_assoc($result); return $fetchedRow; } else { die("Bad query result."); } } } In your client code, you'd use the class like so: require_once('../database_settings.php'); $mysql = new mysql($db_host, $db_user, $db_password); //Note NO $this-> $mysql->selectTable($tableName); $query = "SELECT * FROM users"; $result = $mysql->query($query); while($row = $mysql->fetch($result)) { /* do stuff */ }
-
The problem lies here: public function fetchAssoc($query) { if(!is_resource($query)) { $this->query($query); // <--- right here } $assoc = mysql_fetch_assoc($query); return $assoc; } Your query function returns a mysql_result, yet you don't actually capure that result because you don't assign the return value to a variable. So, if the value passed into the function isn't a result, you're screwed, because mysql_fetch_assoc() is trying to fetch a result from the actual query text rather than a mysql_result. A solution would be: public function fetchAssoc($query) { if(!is_resource($query)) { $result = $this->query($query); } else { $result = $query; } $assoc = mysql_fetch_assoc($result); return $assoc; }