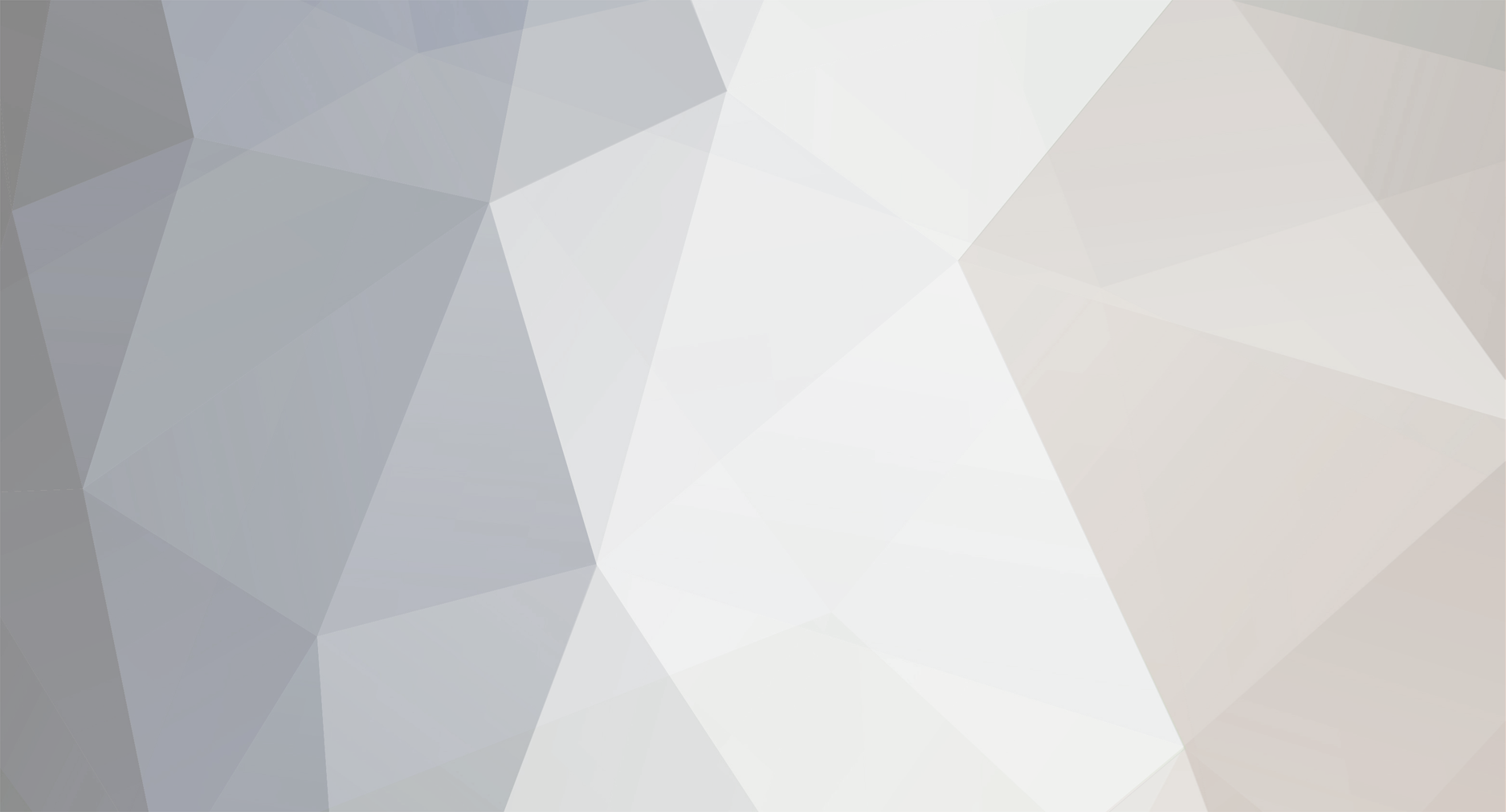
KevinM1
Moderators-
Posts
5,222 -
Joined
-
Last visited
-
Days Won
26
Everything posted by KevinM1
-
Okay, so like virtually every young wannabe PHP developer, I'm attempting to make my own web-based RPG. Right now I'm still in the planning stage, so feel free to move this to the App Design forum if need be. Right now, my biggest stumbling block is handling different weapon abilities. Say I have a basic weapon type of Firearm: abstract class Firearm { private $ammoType; //obvious private $ammoAmount; //number of bullets/shells/whatever per reload private $shotType = "single"; //single shot, burst, or full-auto private $baseDmg = 5; private $maxRng = 50; abstract function getShotType(); abstract function getBaseDmg(); abstract function getMaxRng(); } And a generic decorator setup: abstract class FirearmDecorator extends Firearm { protected $firearm; public function __construct($firearm) { $this->firearm = $firearm; } } class Glock extends FirearmDectorator { public function getShotType() { return $this->firearm->shotType; } public function getBaseDmg() { return $this->firearm->baseDmg * 1.25; } public function getMaxRng() { return $this->firearm->maxRng * 0.75; } } What could/should I do if I wanted to add a special attack for each concrete weapon? Or, even better, based on a character's skills? So, say my Glock has an attack that increases accuracy, or my character has trained in something that causes splash damage to other enemies? I'm thinking that some sort of strategy object (or objects, depending on the number of special attacks available) would be the way to go, but I'm having difficulty seeing how to implement it cleanly. Any ideas?
-
What is it that your select element is supposed to be doing, exactly?
-
Is there a neat,clean way to avoid code repetition like this?
KevinM1 replied to MelodyMaker's topic in PHP Coding Help
Maybe I'm looking at this wrong, but isn't this a clear case of where delegation should be used? It seems like the most obvious solution to me. I mean, MM wants a User to own (contain) Photos associated with them. This User, logically, should be able to modify their own Photos. Why not just have an array of Photos as a property of the User class, then a series of methods that delegate to the Photo's methods? Something like: class User { private $photos = array(); . . . public function addCaption($key, $caption) { $this->photos[$key]->addCaption($caption); } } class Photo { private $caption; . . . public function addCaption($caption) { $this->caption = $caption; } } $Bob = new User(); . . . $Bob->addCaption("vacation", "My summer vacation"); //remember, array keys (in this case, "vacation") can be strings -
The easiest way to do it would probably be to create a popup window with three buttons. Those buttons would each have an onclick event handler which would execute option1, option2, or just merely close the window, depending on which button was clicked. Do you need the actual code for this, or can you work it out from the general algorithm I gave above?
-
How do those of you who loathe singletons handle things typically associated with a registry? Any globals I can remove, the better.
-
Turns out I had a typo. D'oh! My non-jQuery version also works perfectly now, so I'm not sure why yours wouldn't, unless it takes time in IE to apply new styles.
-
I ran into a strange DOM-loading issue with my tests. I had the following code: <html> <head> <title>CSS Selector Test</title> <script type="text/javascript"> window.onload = function() { var mySwitch = document.forms["myForm"].elements["mySwitch"]; mySwitch.onchange = function() { var styles = document.getElementById("styles"); styles.href = mySwitch.value; } } </script> <link id="styles" rel="stylesheet" href="original.css"> </head> <body> <div>This is the thing we're going to change, among others</div> <form name="myform" action="#" method="post"> <select name="mySwitch"> <option value="original.css">Original</option> <option value="black.css">Black</option> <option value="bold.css">Bold</option> </select> </form> </body> </html> But it kept telling me that document.forms["myForm"].elements["mySwitch"] was undefined, despite using window.onload to wait for the DOM to load. I did get it to work using jQuery: <html> <head> <title>CSS Selector Test</title> <script type="text/javascript" src="js/jquery-1.2.6.min.js"></script> <script type="text/javascript"> $(document).ready(function() { $("#mySwitch").change(function() { $("#styles").attr("href", $(this).attr("value")); }); }); </script> <link id="styles" rel="stylesheet" href="original.css"> </head> <body> <div>This is the thing we're going to change, among others</div> <form name="myform" action="#" method="post"> <select name="mySwitch" id="mySwitch"> <option value="original.css">Original</option> <option value="black.css">Black</option> <option value="bold.css">Bold</option> </select> </form> </body> </html> But since it's not pure JavaScript, it may not be the solution you're looking for. Hopefully someone like Aaron (rhodesa) or Fenway will chime in, to both give you the solution to your problem, and to tell me why my window.onload attempt didn't work. EDIT: Just to be clear, the jQuery solution returns an almost-instantaneous css change in IE7 (as well as FF2).
-
Hmm...I can't see anything that would be causing this issue. I mean, it's a simple value swap, so it should be happening instantaneously. I'm going to run a few tests. I'll let you know if the same thing happens to me.
-
Mind showing the relevant code? It's impossible to suggest a fix based on one function definition and a host of link tags.
-
What is "document.reg.theme", and where is it defined? How is preview() being called? It's possibly a matter of just waiting for the DOM to load, but it's hard to tell just by this vague snippet of code. Also, JavaScript is supposed to be inserted as so: <script type="text/javascript"> //JS CODE </script> Using HTML comments isn't necessary, unless you're intentionally scripting for older browsers. All modern browsers understand JavaScript.
-
It looks like you have a syntax error. Specifically, you're trying to assign a string ("this.select()") to an event, which is illogical. Select() is the name of the callback function you're trying to attach, right? If so, try: for(var i = 0; i < textInput.length; i++) { textInput[i].onclick = select; }
-
Show us how a window such as this is typically created.
-
Try: <script type="text/javascript"> window.onload = function() { var forms = document.forms; var focusedForm = ''; for(var i = 0; i < forms.length; i++) { forms[i].onfocus = function() { focusedForm = this.name; // or this.id, depending on your identification scheme } } // do something with focusedForm } </script>
-
Agreed, on all counts. Also, there are many good libraries available that virtually eliminate cross-platform issues anyway, at least where JavaScript is concerned.
-
<script type="text/javascript"> window.onload = function() { //code to display the window } </script>
-
Warning: does not always return a value
KevinM1 replied to The Little Guy's topic in Javascript Help
Not just for AJAX functions, but for any JavaScript function embedded in a link. 'return false' tells the browser to not perform the default action which, in this case, is to follow the hyperlink's href property. -
I used to code like: function myFunction(){ if($me == "awesome"){ echo "Damn straight."; } else{ echo "You suck."; } } In the last month or so, I've changed to: function myFunction() { if($me == "awesome") { echo "Damn straight."; } else { echo "You suck."; } } It seems more readable to me, especially when using something like Notepad++ which highlights matching braces.
-
Some general hints: 1. Figure out the current time and value of the category, and the experation time 2. If time has expired, the last person who clicked the button wins 3. If button is clicked, increase experation time/value of the category You should be able to figure it out from there.
-
[SOLVED] Help with validating form email addresses
KevinM1 replied to Sportbob's topic in PHP Coding Help
I believe you can just put a | between the two domains: (domain1.edu | domain2.edu) That should tell it to accept either domain1 or domain2. You can test regular expressions here: http://regexlib.com/RETester.aspx -
No one's stopping you from having opinions or feelings. I am suggesting, however, that you employ some tact. Professionalism begins there. And, I'll let you in on something: I hate it when people who don't know what they're doing are paid by unsuspecting clients to do a project for them. It's unfair to the clients, who just paid a lot of money for shoddy work, and it's unfair to those of us in the profession, as it negatively affects our overall reputation. So, yes, I do get where you were coming from. That said, I'm not arrogant to ASSume that the people asking for help here are shady charletans scrambling to finish a project that's way over their ability, or students who didn't pay attention in class because they were partying, or stayed up all night playing TF2 with their buddies. And I'm certainly not going to look down on the people asking questions here. Why? Because I was a newbie too. I started coming here at least a year ago, if not earlier. I asked the simple questions. I didn't know what escaping meant. I thought sessions were completely mysterious. I could barely get a sticky form to work right. But the people here were both generally kind and helpful, and I progressed very quickly, in part because of them. And, you know what? I still ask questions on here. Things slip my mind. I have an OOP idea that I could design in several ways that I'd like feedback on. I ask about other languages/technologies I'm not entirely comfortable with. Does that make me inherently deficient, or 'bad' somehow? Should I be treated with less respect because I want a second opinion? Or because I had a brain fart? Or because I still may have trouble wrapping my head around something? tldr; You want to be a professional? Try acting like an adult first.
-
Is that really necessary? Also, by looking at the OP's posting history, it's pretty obvious that they're a student. 1) yes, it was necessary. Whether he's a student or a professional, getting answers from other people is never a good thing. So, why are you here then? This is a place where people ask questions and seek answers. Perhaps. Or perhaps they're just not suited to be a programmer, and, despite their efforts, are having trouble finding the solution. ASSuming why a person is requesting help speaks more about you than them, IMO. What is this, high school? You don't get extra leeway just because you supplied some help. One does not excuse/negate the other. In any event, if you feel the need to respond, please take it to PMs. I have the feeling we've polluted this thread enough.
-
Is that really necessary? Also, by looking at the OP's posting history, it's pretty obvious that they're a student.
-
Possible? Multiple image/text submits on form..
KevinM1 replied to monkeytooth's topic in PHP Coding Help
Yes, you can have multiple submits. However, it's best to give them different names. You can probably work around that by doing something like: <input type="submit" value="thing1" name="submit[]"> <input type="submit" value="thing2" name="submit[]"> <input type="submit" value="thing3" name="submit[]"> That may pass the submits to PHP/JavaScript as an array. I haven't tested it myself, so I can't say with 100% certainty. That said, for additional processing, you'll have to do some array manipulation in order to obtain the correct submit button. That's why I think it's just easier to give them different names. You can access them directly without having to jump through extra hoops. -
What do you mean, exactly, by 'time specified'? Are you referring to an assignment's due date/time, or are you referring to a timeout situation, where if a user is accessing the file upload page for too long a time you'll boot them to another page?
-
If use_potion() is defined within your Char class, the line in question should simply be: <?php $this->hp_up($row['HP_Bonus']); ?> The hp_up() function already updates the $hp value, from what you showed me, so there's no reason to assign it. Double-check your syntax. You've lost track of capital letters and even function names (up_hp()? or hp_up()?) in the examples you've written. I still think it's better to refactor the armor and weapon. Why? Well, for one thing, a Char object really shouldn't 'know' the inner workings of how armor and weapons are created/used. Yeah, it works, but it ties weapon and armor info (and behavior!) to a character. This tight coupling may pose problems down the road as your system expands. Another reason is flexability. It's much more difficult creating different weapons using an array than an object. Let's use swords as an example: <?php abstract class Sword { abstract public function getDura(); abstract public function getAttack(); abstract public function getParry(); abstract public function getHpMod(); abstract public function getMagic(); } abstract class SwordDecorator extends Sword { protected $sword; public function __construct(Sword $sword) { $this->sword = $sword; } } class ShortSword extends Sword { private $durability = 12; private $attack = 10; private $parry = 15; private $hpMod = 0; private $magic = "none"; public function getDura() { return $this->durability; } public function getAttack() { return $this->attack; } . . . } class Icebrand extends SwordDecorator { public function getDura() { return $this->sword->getDura() * 1.35; } public function getAttack() { return $this->sword->getAttack() * 1.5; } . . . public function getMagic() { return "ice"; } } $IceShortSword = new Icebrand(new ShortSword()); $Icingdeth = new Icebrand(new Scimitar()); $FireDagger = new Flametongue(new Dagger()); $HolyAvenger = new HolySword(new BastardSword()); //etc. ?> By breaking things down into their constituent parts, you make it far easier to increase the lifespan of your application. In an RPG (which I'm assuming your project is), loot is often key. Being able to come up with new items on the fly, like I illustrated above, greatly reduces the amount of grunt work you'll have to do in the long run. Still, it's just an idea. It's obviously your decision.