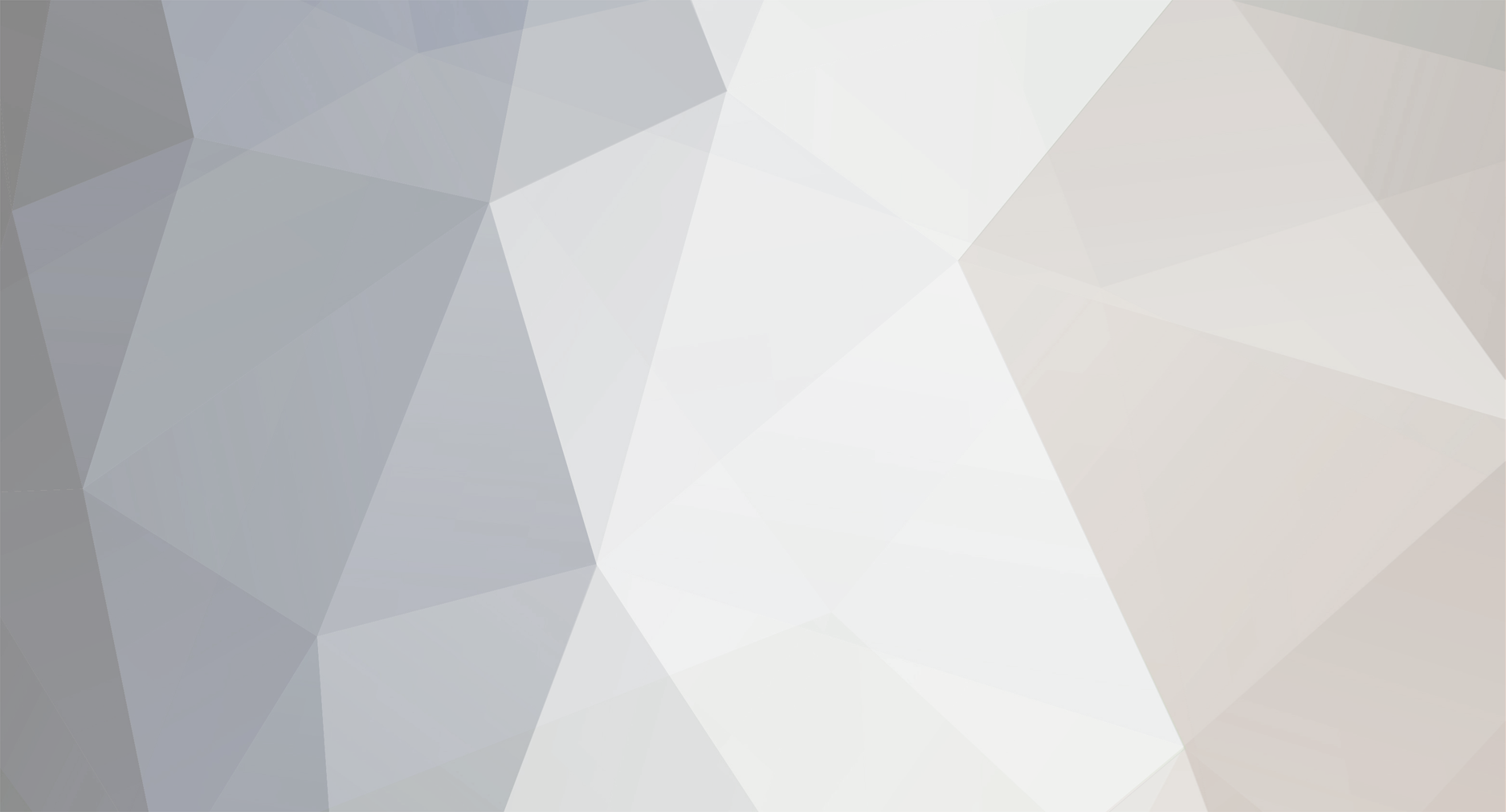
KevinM1
-
Posts
5,222 -
Joined
-
Last visited
-
Days Won
26
Posts posted by KevinM1
-
-
I've read that a lot of people use the __autoload() function to dynamically include whatever class files are needed. I'm just wondering if I'm understanding how that's done:
You put your defined __autoload() function inside of a file that's included in every other page (like a config file), right? And the definition would be something along the lines of the following code, correct?
[code]
<?php
function __autoload($className){
include ("../classes/{$className}.php");
}
?>
[/code] -
Yeah, OO is pretty tricky in that regard. If you were programming a game, then those examples would make more sense. When I was at UNH, we basically used the OO capabilities of C++ to create our own abstract data types/structures. For PHP, I think that's a better way to look at it. A C++ OO example would be a dynamically allocated list, queue, or stack. Or maybe an improved string data type.
So, don't worry so much about what the examples create. The important thing is knowing how classes are created and how you can use them. They're basically just a way to make your own data structures. -
[quote author=ToonMariner link=topic=114122.msg464297#msg464297 date=1162916655]
can it be done woithout paragraph tags?
[/quote]
I dunno..maybe. You could do the same thing by putting them into their own div if you want, but I believe they need some kind of containing element to get the floats to clear properly with everything properly positioned on each line. And what difference does it make, anyway? You can change the separation between inputs by playing around with the paragraph's margins. It's not that much more markup (about the same as what you originally posted with some extraneous divs) and it behaves properly, with the exception of your radio button text being not quite level with the button itself. -
This should work. The 'Use as correspondee' text isn't quite even with the radio button, though.
CSS:
[code]
form#delreg{
width: 765px;
}
fieldset{
border: 1px solid #939598;
}
#delreg label, #delreg input, #delreg textarea{
margin: 5px 10px;
float: left;
}
#delreg label{
float: left;
width: 130px;
text-align: right;
}
#delreg .bttn{
margin: 10px;
}
p {
clear: both;
margin: 5px;
}
[/code]
HTML:
[code]
<?php
//to get around the security measures that make it hard to post html code on the board
?>
<form action="/delegate_reg.php" method="post" enctype="application/x-www-form-urlencoded" name="delreg" id="delreg">
<div id="dcont">
<fieldset>
<p><label for="name[0]"><span class="req">*</span>Delegate Name:</label><input type="text" name="name[0]" id="name[0]" value="" /></p>
<p><label for="phone[0]"><span class="req">*</span>Telephone:</label><input type="text" name="phone[0]" id="phone[0]" value="" /></p>
<p><label for="fax[0]">Fax:</label><input type="text" name="fax[0]" id="fax[0]" value="" /></p>
<p><label for="email[0]"><span class="req">*</span>Email:</label><input type="text" name="email[0]" id="email[0]" value="" /></p>
<p><label for="address[0]"><span class="req">*</span>Postal Address:</label><textarea name="address[0]" id="address[0]"></textarea>
<input type="radio" name="correspondee" value="0" checked="checked" />Use as Correspondee</p>
<p><label for="postcode[0]"><span class="req">*</span>Postcode:</label><input type="text" name="postcode[0]" id="postcode[0]" value="" /></p>
</fieldset>
</div>
<a href="javascript:tccAddDel();" title="Add Image">Add Another Delegate</a><br />
<input type="submit" class="bttn" name="submit" id="submit" value="Next" />
</form>
<?php
//one more time for good measure
?>
[/code] -
[quote author=thorpe link=topic=114011.msg463682#msg463682 date=1162829305]
[quote]Is the 'arrow' notation used in the first example always just representative of a truncated function name?[/quote]
No. In the example mysqli is an object, not a function.
[/quote]
There doesn't seem to be much difference, though. I mean, there are two ways to initialize it:
[code]
<?php
$stmt = $mysqli->stmt_init(); //the OO way
$stmt = mysqli_stmt_init($link); //the procedural way
?>
[/code]
The former uses an object...is there a mysql object where it could be used in the way I theorized in my last post (code repeated below)?
[code]
<?php
$dbc = $mysql -> connect($username, $host, $password);
$db = $mysql -> select_db('dbname', $dbc);
?>
[/code] -
Why do you have a php file as a source in your second image tag?
-
Say I have the following html document:
[code]
<?php
//using php in my code block because the board always breaks on me if I try using just html
?>
<html>
<head><title>Blah</title></head>
<body>
<div>This chicken tastes <span style="font-weight: bold;">really</span> good.</div>
</body>
</html>
<?php
//more php just to make sure the board doesn't break
?>
[/code]
What would be the div's text node(s)? Would it be "This chicken tastes good?" Or would there be two text nodes because of the span ("This chicken tastes," and "good")? -
While visiting the PHP.net site after following a link from one of the other posts here, I found something that highlights something that confuses me regarding the language. The function in question isn't of importance (mysqli_stmt_prepare), but the examples are:
OO style of using the function:
[code]
<?php
$mysqli = new mysqli("localhost", "my_user", "my_password", "world");
/* check connection */
if (mysqli_connect_errno()) {
printf("Connect failed: %s\n", mysqli_connect_error());
exit();
}
$city = "Amersfoort";
/* create a prepared statement */
$stmt = $mysqli->stmt_init();
if ($stmt->prepare("SELECT District FROM City WHERE Name=?")) {
/* bind parameters for markers */
$stmt->bind_param("s", $city);
/* execute query */
$stmt->execute();
/* bind result variables */
$stmt->bind_result($district);
/* fetch value */
$stmt->fetch();
printf("%s is in district %s\n", $city, $district);
/* close statement */
$stmt->close();
}
/* close connection */
$mysqli->close();
?>
[/code]
Procedural style of using the function:
[code]
<?php
$link = mysqli_connect("localhost", "my_user", "my_password", "world");
/* check connection */
if (mysqli_connect_errno()) {
printf("Connect failed: %s\n", mysqli_connect_error());
exit();
}
$city = "Amersfoort";
/* create a prepared statement */
$stmt = mysqli_stmt_init($link);
if ($stmt = mysqli_stmt_prepare($stmt, "SELECT District FROM City WHERE Name=?")) {
/* bind parameters for markers */
mysqli_stmt_bind_param($stmt, "s", $city);
/* execute query */
mysqli_stmt_execute($stmt);
/* bind result variables */
mysqli_stmt_bind_result($stmt, $district);
/* fetch value */
mysqli_stmt_fetch($stmt);
printf("%s is in district %s\n", $city, $district);
/* close statement */
mysqli_stmt_close($stmt);
}
/* close connection */
mysqli_close($link);
?>
[/code]
Is the 'arrow' notation used in the first example always just representative of a truncated function name? Could I do something like the following?
[code]
<?php
$dbc = $mysql -> connect($username, $host, $password);
$db = $mysql -> select_db('dbname', $dbc);
?>
[/code] -
Once you have a form's id, you don't need an id for each input. So long as the inputs have a name (which, in your case, they must), you can still access them.
[code]
var form = document.getElementById('formId');
var name = form.name;
var email = form.email;
var password = form.password;
.
.
.
//this example assumes your form has inputs with the names name, email, and password
[/code] -
[quote author=AndieB link=topic=113967.msg463508#msg463508 date=1162799478]
Hello all,
perhaps someone of you are able to provide me with a solution.
I'm having a TABLE which is created when I'm querying a database. The result is displayed in the TABLE. Now, the thing is that I want people to be able to get more details about the "displayed" information by clicking a ROW ([i]the TR in the table[/i]) in the table, no matter in which TD they click.
Also I want the ROW ([i]TR[/i]) to change background-color when I put the mousepointer over a ROW, to show that is being selected. I do not want to use the <a href...> tag, I want to use: onMouseOver and onClick...
Anyone knows HOW I should code this??
Thank you in advance for any kind of help!!
Sincerely,
Andreas
[/quote]
Well, if those table rows are the only ones in your html, you could do something along the lines of:
[code]
var rows = document.getElementsByTagName('tr');
for(var i = 0; i < rows.length; i++){
rows[i].onmouseover = mouseOverFunction;
rows[i].onmouseout = mouseOutFunction; //you probably don't want the row to stay highlighted if the mouse isn't hovering over it
rows[i].onclick = mouseClickFunction;
}
[/code]
If you have multiple tables, you can still do the same thing, with an extra step:
[code]
var table = document.getElementById('tableId');
var rows = table.getElementsByTagName('tr');
for(var i = 0; i < rows.length; i++){
rows[i].onmouseover = mouseOverFunction;
rows[i].onmouseout = mouseOutFunction;
rows[i].onclick = mouseClickFunction;
}
[/code]
I'm not 100% sure about what you want to have displayed when a user clicks on a row, but changing the background color is pretty easy:
[code]
function mouseOverFunction(evt){
evt = (evt) ? evt : ((event) ? event : NULL);
if(evt){
var elem = (evt.target) ? evt.target : ((evt.srcElement) ? evt.srcElement : NULL);
if(elem){
elem.style.backgroundColor = 'red';
}
}
}
function mouseOutFunction(evt){
evt = (evt) ? evt : ((event) ? event : NULL);
if(evt){
var elem = (evt.target) ? evt.target : ((evt.srcElement) ? evt.srcElement : NULL);
if(elem){
elem.style.backgroundColor = 'blue';
}
}
}
[/code]
Keep in mind that the event handler function names are intentionally generic...you probably shouldn't name them the way I have.
EDIT: fixed the last two functions as I forgot about handling the event...d'oh! -
[quote author=obsidian link=topic=113609.msg462511#msg462511 date=1162581079]
[quote author=Nightslyr link=topic=113609.msg462505#msg462505 date=1162580564]
[quote author=448191 link=topic=113609.msg462496#msg462496 date=1162579287]
It's not recursive. The second parse method is the modules' method.
[/quote]
??
Okay, now I'm completely lost.
[/quote]
Take a look at the line that [i]appears[/i] to be recursive. It's actually calling:
[code]
<?php
// this
$module->parse();
// not this (recursive)
$this->parse();
?>
[/code]
It is calling the parse() function of each module you have loaded.
[/quote]
With each defined in its own class? -
[quote author=448191 link=topic=113609.msg462496#msg462496 date=1162579287]
It's not recursive. The second parse method is the modules' method.
[/quote]
??
Okay, now I'm completely lost. -
I hope you don't mind answering this newb question, but I'm a bit confused on the following bit of code:
[code]
<?php
public function parse($input) {
foreach($this->modules as $module){
$input = $module->parse($string);
}
return $input;
}
?>
[/code]
I know it's recursive, but I can't see what it actually does. So, uh...what does it do? -
As far as I know, the statements in the creation of my positions table that have REFERENCES in them are supposed to create the foreign keys (see my original post for how I created them).
You're right about the second part. I did some more research and found that I do have to manually put them there. D'oh! -
I was able to force them into InnoDB by putting 'ENGINE=INNODB' at the end of my CREATE statements.
According to phpMyAdmin, my tables are InnoDB, but the database is MyISAM. Screenshot link:
http://www.nightslyr.com/hockey/dbstructure.jpg
My goalies table is filled out (I used this query so you could see all of the columns). Screenshot link:
http://www.nightslyr.com/hockey/goalies.gif
But the goalie_id in my positions table still isn't being set. Screenshot link:
http://www.nightslyr.com/hockey/positions.jpg
And I populated the positions table [b]after[/b] I populated the goalies table, just in case that makes a difference. -
Well, I was able to change the tables to InnoDB, but my hosting won't let me change the actual database, which is still MyISAM. Despite my hosting telling me that changing just the tables would work, I'm still not seeing the goalie_id's in the positions table (more precisely, everything is still 0 in that column), so I guess they were wrong.
Any ideas on what I can do? -
I'm using phpMyAdmin to update my database. I decided to rebuild some of the tables from scratch. Unfortunately, the table that's supposed to have foreign keys isn't 'seeing' them.
I have three tables (one of which is currently empty):
[code]
CREATE TABLE positions (
pos_id TINYINT UNSIGNED NOT NULL AUTO_INCREMENT,
goalie_id TINYINT UNSIGNED NOT NULL REFERENCES goalies(goalie_id),
skater_id TINYINT UNSIGNED NOT NULL REFERENCES skaters(skater_id),
position VARCHAR(2) NOT NULL,
jersey_num TINYINT UNSIGNED NOT NULL,
PRIMARY KEY(pos_id));
CREATE TABLE goalies (
goalie_id TINYINT UNSIGNED NOT NULL AUTO_INCREMENT,
first_name VARCHAR(25) NOT NULL,
last_name VARCHAR(40) NOT NULL,
gaa FLOAT UNSIGNED NOT NULL,
save_percent FLOAT UNSIGNED NOT NULL,
shots_faced SMALLINT UNSIGNED NOT NULL,
goals_allowed TINYINT UNSIGNED NOT NULL,
saves SMALLINT UNSIGNED NOT NULL,
shutouts TINYINT UNSIGNED NOT NULL,
games_played TINYINT UNSIGNED NOT NULL,
wins TINYINT UNSIGNED NOT NULL,
losses TINYINT UNSIGNED NOT NULL,
ties TINYINT UNSIGNED NOT NULL,
minutes MEDIUMINT UNSIGNED NOT NULL,
attributes TINYTEXT NOT NULL,
biography TEXT NOT NULL,
oldstats TEXT NOT NULL,
PRIMARY KEY(goalie_id));
CREATE TABLE skaters (
skater_id TINYINT UNSIGNED NOT NULL AUTO_INCREMENT,
first_name VARCHAR(25) NOT NULL,
last_name VARCHAR(40) NOT NULL,
goals TINYINT UNSIGNED NOT NULL,
assists TINYINT UNSIGNED NOT NULL,
points TINYINT UNSIGNED NOT NULL,
pim SMALLINT UNSIGNED NOT NULL,
plus_minus TINYINT(4) NOT NULL,
attributes TINYTEXT NOT NULL,
biography TEXT NOT NULL,
oldstats TEXT NOT NULL,
PRIMARY KEY(skater_id));
[/code]
As of right now, skaters is empty.
When checking the filled tables goalies and positions, the positions table has 0's in the goalie_id column where there should be an id number. The goalie_id is correctly created in the goalies table, however. Since the positions table references the other two, shouldn't its goalie_id column (in this case) automatically have the proper ids for those two rows?
EDIT: would my hosting company changing storing engines from InnoDB to MyISAM be the culprit? -
Well, right now you have the order backwards. The most logical thing to do is process the form if it's been submitted. If it [b]hasn't[/b] been submitted, then you output the form. I've rewritten it into a form that should work below:
[code]
<?php
$errorMessage = ''; //initialize our error message
if(isset($_POST['submit'])){ //if the form has been submitted...
if(!empty($_POST['company'])){ //if company isn't empty
$company = $_POST['company']; //set the local variable to what's been posted
$comp = TRUE; //set a boolean for future use
}
else{ //company IS empty
$errorMessage .= "Please input your company name!<br />"; //add to our error message
$comp = FALSE; //set a boolean for future use
}
if(!empty($_POST['name'])){
$name = $_POST['name'];
$n = TRUE;
}
else{
$errorMessage .= "Please input your name!<br />";
$n = FALSE;
}
if(!empty($_POST['email'])){
$email = $_POST['email'];
$e = TRUE;
}
else{
$errorMessage .= "Please input your e-mail address!<br />";
$e = FALSE;
}
if(!empty($_POST['contact'])){
$contact = $_POST['contact'];
$cont = TRUE;
}
else{
$errorMessage .= "Please input your contact number!<br />";
$cont = FALSE;
}
if(!empty($_POST['message'])){
$message = $_POST['message'];
$mess = TRUE;
}
else{
$errorMessage .= "Please input your message!";
$mess = FALSE;
}
if($comp && $n && $e && $cont && $mess){ //if everything's been filled out correctly, send the mail
$ip = $_SERVER['REMOTE_ADDR'];
$time = time();
$date_message = date("r", $time);
$subject="New Enquiry";
$email="me@mine.com";
//format the message
$msg .= "IP: $ip\n";
$msg .= "$date: $date_message\n";
$msg .= "$subject\n";
$msg .= "Company: $company\n";
$msg .= "Name: $name\n";
$msg .= "Email: $email\n";
$msg .= "Message: $message\n";
mail($email,$subject,$msg);
echo "Your message has been sent. We will contact you with a reply as soon as possible.";
}
else{ //something is wrong, so print our error message
echo "<span style="color: red;">Could not send e-mail for the following reasons:<br />$errorMessage";
}
else{ //the form HASN'T been submitted yet
?>
<form action="<?php echo $_SERVER['PHP_SELF']; ?>" method="post">
<div align="center">
<table width="0%" height="0" border="0" align="center" cellpadding="0" cellspacing="0" class="contacttable">
<tr>
<td colspan="2"><p align="center"><strong>Fill in the form below to make an Enquiry</strong></p></td>
</tr>
<tr>
<td><p align="left"><b>Company name:</b></p></td>
<td><input name="company" type ="text" size="50" maxlength="30" />
<div align="center"></div></td>
</tr>
<tr>
<td><p align="left"><b>Your Name:</b></p></td>
<td><input name="name" type ="text" size="50" maxlength="30" />
<div align="center"></div></td>
</tr>
<tr>
<td><p align="left"><b>Your Email:</b></p></td>
<td><input name="email" type ="text" size="50" maxlength="50" /></td>
</tr>
<tr>
<td><p align="left"><b>Contact Number:</b></p></td>
<td><input name="contact" type ="text" size="50" maxlength="25" /></td>
</tr>
<tr>
<td width="150"><p align="left"><b>Your Messsage:</b></p></td>
<td><textarea name="message" cols="50" rows="8"></textarea></td>
</tr>
<tr>
<td class="bottomleft"> </td>
<td><input name="submit" type="submit" value="Send Message" /></td>
</tr>
</table>
</p>
</div>
</form>
<?php
} //ending bracket for our else-conditional
?>
[/code] -
[quote author=obsidian link=topic=113366.msg460601#msg460601 date=1162311235]
The order of your declared columns really only matters to you. Keep in mind that whatever order you create your columns in is going to be the default order of values for INSERT statements as well. With this in mind, order doesn't necessarily matter as long as you keep track of what order to insert you values.
[/quote]
Ah, I should've phrased my initial question better... ;D
I'm wondering about the [i]attributes[/i] (like auto_increment, not null, etc) -- is there a certain order they're supposed to go in after the column name and datatype have been declared? -
Well, you could combine your pages into one file (say contact.php):
[code]
<?php
if(isset($_POST['submit'])){ //has the form been submitted?
//check all of the inputs
if(!empty($_POST['company'])){ //if the company field of the form is not empty
//process the info
}
else{
//company field hasn't been filled -- error
}
.
.
.
} //end of if(isset(...
else{ //if it hasn't been submitted, display the form
?>
<form action="<?php echo $_SERVER['PHP_SELF']; ?>" method="post">
.
.
.
<?php
} //end of the else block
?>
[/code]
A script can refer to itself (which is what the echo statement in the form does), so you can have a script both display a form and process it upon submission, with the basic outline I showed above. I've also shown how to check if an input field is empty or not (only works in textfields, I believe).
For your e-mail structure, you'll probably have to use regular expressions. I'm not very familiar with PHP's regex functionality, so I'll leave that for someone else to handle. ;) -
When defining a table, does the order of column attributes matter? If so, is there a general syntax that column attrubutes follow (i.e. datatype, followed by it being null or not, followed by...)?
-
I think it all depends on how complex you want your attack system to be. Something simple would to have it merely retrieve the stats from the database and use them without any kind of formula. Example:
Character A has 20 Strength and 85 HP
Character B has 14 Strength and 67 HP
Each attack every other second, with the first character to attack determined randomly. Damage is just the target's HP - the attacker's strength. In a case like this one, Character A will always win. Not very exciting.
The reason why the people above me kept asking about what formula you've developed is because RPGs take a lot into account when it comes to combat. Is anyone wearing armor? Any stat bonuses? Any status effects (poison, blindness, mute)? Does positioning matter? Who has a greater chance to hit the other? Are they even in melee range? Or is one using a ranged weapon (bow, gun, crossbow, sling, etc)? Or magic? Every turn in combat in every RPG requires the manipulation of this kind of data in a specific way. It is up to you to create that specific way, either by liberally using the combat system of your favorite tabletop RPG or by creating one by yourself.
Good luck. :) -
Hehe, this is pretty interesting to read from a newb's point of view. Quick question: I'm a bit lost at
<?php class Guts implements ArrayAccess, Singleton ?>
As I don't see ArrayAccess defined anywhere. Am I missing something, or just blind?
-
I think something like:
[code]
<input name="demo" value="<?php if(isset($_POST['demo'])) echo $_POST['demo']; ?>" >
[/code]
Will work.
Latest owner query
in MySQL Help
Posted
Thanks. :)