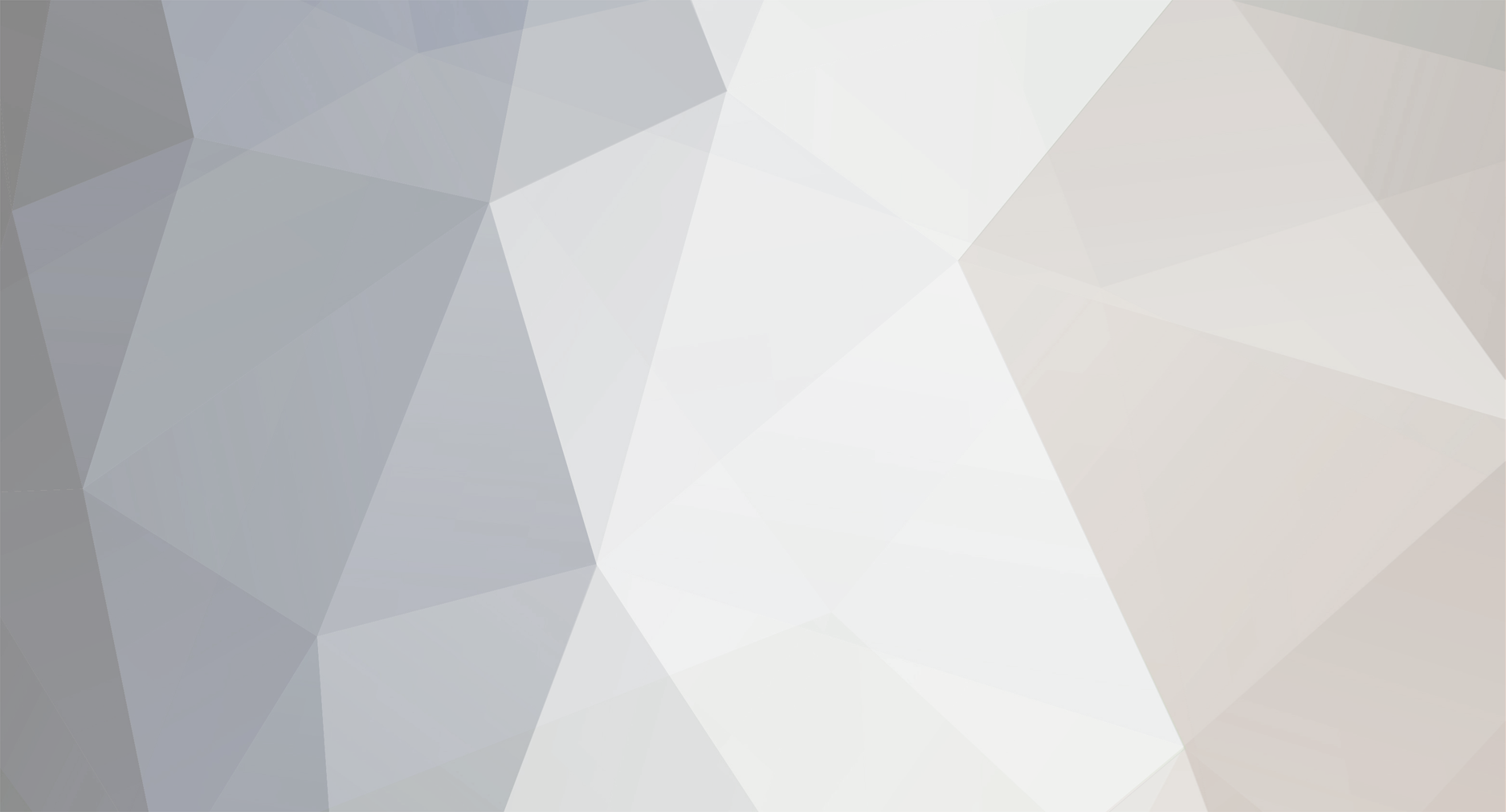
KevinM1
-
Posts
5,222 -
Joined
-
Last visited
-
Days Won
26
Posts posted by KevinM1
-
-
Never mind. I figured it out. Apparently, I'm an idiot. :lol:
-
Hey, fenway, thanks for your help and patience. I know it must get frustrating dealing with newbs like me, so I just wanted to let you know that I really do appreciate it. :)
I managed to get the calendars to print, but I seem to have a logic problem. In my testing, my months beginning on any day but Sunday all printed fine. But the months that start on a Sunday (April and I believe July) kept being printed as though they started on a Monday, and I'm not 100% sure why. I have a while-loop (which you'll see below) that prints off the blank days at the beginning of each month, if there are any. It appears that whenever a month starts on a Sunday, that while-loop still fires one time. I've tried different conditionals in there (start != 1 && pos < start, pos < (start - 1)), but none have worked so far. Any ideas?
[code]
var W3CDOM = (document.createElement && document.getElementsByTagName);
var months = ["January", "February", "March", "April", "May", "June", "July", "August", "September", "October", "November", "December"];
var numDays = [31, 28, 31, 30, 31, 30, 31, 31, 30, 31, 30, 31];
var firstDays = [2, 5, 5, 1, 3, 6, 1, 4, 7, 2, 5, 7]; //1 = Sunday, 2 = Monday, etc.
function init(){
if(!W3CDOM) return;
printMonth();
}
function printMonth(){
bod = document.getElementById('bod'); //My body element has an id of bod
var output = '<table><br /><th>Sun</th><th>Mon</th><th>Tue</th><th>Wed</th><th>Thu</th><th>Fri</th><th>Sat</th><br /><tr style="text-align: right;"><br />';
var start = firstDays[6];
var pos = 1;
while(pos < start){
output += '<td></td>';
pos++;
}
for(var i = 1; i <= numDays[6]; i++){
if ((pos / 6) <= 1){
output += '<td>' + i + '</td>';
pos++;
}
else{
output += '</tr><br /><tr style="text-align: right;"><td>' + i + '</td>';
pos = 1;
}
}
output += '</tr><br /></table>';
bod.innerHTML = output;
}
window.onload = init;
[/code] -
[quote author=fenway link=topic=111389.msg452173#msg452173 date=1160935282]
Well, to be honest, I have no idea why you have that if/else/switch logic... it's quite confusing. As for your problem, my guess would be that a variable isn't being reset.
[/quote]
Well, like I said before, the vast majority of the script was written before I got to it. All I did was try to append the switch statements as I was told that the rest worked fine. Unfortunately, I found that to not be the case (my previous message).
I'm now trying to re-write the script from scratch. I've written a basic test script to see if I can print out at least one month correctly. I figure I can modify that to print all months correctly once it works right. Unfortunately, nothing is being printed to the screen so far, and I'm not sure why. My code (which is in an external .js file) is:
[code]
var W3CDOM = (document.createElement && document.getElementsByTagName);
var months = ["January", "February", "March", "April", "May", "June", "July", "August", "September", "October", "November", "December"];
var numDays = [31, 28, 31, 30, 31, 30, 31, 31, 30, 31, 30, 31];
var firstDays = [1, 4, 4, 0, 2, 5, 0, 3, 6, 1, 4, 6];
function init(){
if(!W3CDOM) return;
printJanuary();
}
function printJanuary(){
document.write("<table><th>Sun</th><th>Mon</th><th>Tue</th><th>Wed</th><th>Thur</th><th>Fri</th><th>Sat</th><tr>");
var start = firstDays[0];
var offset = 0;
while(offset < start){
document.write("<td></td>");
offset++;
}
for(var i = 1; i < numDays[0]; i++){
if((i % 7) < 1){
document.write("<td>" + i + "</td>");
}
else{
document.write("</tr><tr><td>" + i + "</td>");
}
}
document.write("</tr></table>");
}
window.onload = init;
[/code]
I have other scripts that use the same basic setup. I've never had a problem with this kind of initialization function being used in the window's onload event handler before, so I'm pretty sure that's not where the problem lies. The XHTML is basically empty. There's no structure other than the body. Any ideas?
EDIT: I know I have a logic problem with the algorithm (the i % 7 bit), but I wouldn't think that would prevent anything from being printed. -
[quote author=fenway link=topic=111389.msg451898#msg451898 date=1160848089]
Your return statement for CheckDate() is inside the for loop, so it only runs once... move it one line down, and it'll work.
[/quote]
Thanks, that helped a lot! :)
Unfortunately, now I have a logic problem. None of the appointments are displaying correctly. For instance, the second appointment is just supposed to highlight June 12 in red. Instead, it highlights from June 1 to June 12. The others show the same kind of thing -- highlighting the entire month in the appropriate color until the value stored in the today variable. I'm not exactly sure where the problem lies. I'm having a hard time following the for-loop and the myriad if-statements it has. I thought the problem lied where if-3 and else-1 are in the loop, as both check to see if dayno >= apps[app][0], but commenting it out didn't help. Any ideas? -
[quote author=fenway link=topic=111389.msg451710#msg451710 date=1160779550]
I think I understand, but I really need to see that page... can you put it up somewhere?
[/quote]
I put it up at http://www.nightslyr.com/calendar.html -- all of the code is in that file. -
[quote author=fenway link=topic=111389.msg451617#msg451617 date=1160768107]
And what calls are you issuing to write out the page itself?
[/quote]
Every time I try posting HTML within the code brackets I get an error, so I can't rewrite the code. But I can describe it! :)
The page's layout is a table, four rows, three columns. In every table cell, they used a script element to call PrintMonth(x), where x is the index of the array (0 = January, 1 = February, etc). Nothing else is within those cells.
Sloppy, and definitely not the way I'd do it. Once I get the calendars to print the set appointments correctly, I'll try to convince the guy I'm helping to let me change how all of that is handled. -
I fixed it. My problem wasn't with creating the feed. The feed came as part of PHP-Fusion. The problem came from the pre-existing code using & instead of its HTML entity equivalent whenever the post appeared on any page but the first. My original tweak (swapping & for its entity), months ago, worked on posts that were on the first page so I thought it would work for all posts, and I didn't notice that $rstart would contain the &. Oops. :blush:
-
I'm weary of the Wii because of its controller. Due to my physical disability, I'm not sure if I'd be able to use the controller well. On the one hand, it would be a great source of exercise. On the other, I wouldn't want to use the motion detection if I just wanted to have some easy, mindless fun. Since I'm not sure if the Wii's games will allow for a more normal control scheme (read: no motion detection), I'll reserve any potential purchace until that info becomes available.
X-Box 360 looks nice, but I'm not really into its franchises. Halo just doesn't look all that interesting to me (I have my lovable Half-Life 2 to fill that niche). Bioshock looks interesting, but there's a possibility it's coming out for the PS3 as well. The only franchise I feel I miss out on is Project Gotham Racing.
The PS3 is my choice. I've already owned both of the previous PlayStations, so its backwards compatability is a nice feature. I like that it comes with a huge hard drive. I'm used to the Dual Shock style controller, so there's no need to adjust there. Sony has historically had the big Square-Enix games, which is nice (even more so if Final Fantasy ever gets out of the emo cloud (no pun intended) its been in for the last decade). Gran Turismo is always pretty fun (except for the AI), and the F1 game it has looks incredible. All the other big titles (Assassin's Creed, Grand Theft Auto) are multi-platform. And, to top it all off, Tekken. -
Name: Kevin
Gender: Male (don't know too many female Kevin's....)
Age: 26
Â
I remember 'programming' on my Commodore VIC-20 when I was a little kid. Basically, we -- my brothers and I -- would just copy the instructions from one of the books we had of fun things to do with Basic. It was pretty cool making rockets out of *'s and watch them move up the screen. And, btw, Gorf and Radar Rat Race still own.
Â
I went to UNH to get my CS degree. I did alright until I got to assembly, and basically was stuck for two years before I figured I should at least get some sort of degree from there. So I went from Hell's Kitchen to Easy Bake Oven -- a communication degree. Gotta love liberal arts.
Â
Virtually all of my web development knowledge has been self-taught, either by me going to message boards like this one or by buying books and learning that way. I'm still a newb, but I'm making relatively steady progress. My university experience with C++ has helped in that regard, so variables, arrays, dynamic structures (lists, queues, stacks), and even simple objects are familiar territory. It's just a matter of learning how to implement them and when to use them.
-
Should I be using htmlspecialchars_decode for the ampersand? Or will it print correctly because it's within double-quotes?
-
Unfortunately, since I'm not 100% sure where my problem lies, I'll have to post all of the script so you can see how each of the functions are called. CheckDate is the most important function, and the one that I modified (the switch blocks). The only other thing I modified was the apps 2-d array. I merely added a flag as the last element for each array.
[code]
var months = ["January","February","March","April","May","June","July","August","Se ptember","October","November","December"];
var daycounts = [31,28,31,30,31,30,31,31,30,31,30,31]; //for leap years, remember to set february to 29 days
//2002 firstdays = [1,4,4,0,2,5,0,3,6,1,4,6];
var firstdays = [0,3,3,6,1,4,6,2,5,0,3,5];
//2004 firstdays = [3,6,7,3,5,1,3,6,2,4,0,2];
// This is where you put in the appointments. follow pattern [fromday,frommonth,today,tomonth,message, flag]
var apps = [
[1,1,2,1,"Red Watch Days", "Holiday"],
[12,6,12,6,"my birthday", "Holiday"],
[28,8,2,9,"Trip to Paris", "Holiday"],
[22,11,22,11,"Party with colleagues", "Holiday"],
[20,12,30,12,"Christmas with family", "Holiday"],
[12, 10, 14, 10, "Watch 1 Test", "Watch1"],
[15, 10, 17, 10, "Watch 2 Test", "Watch2"],
[18, 10, 20, 10, "Watch 3 Test", "Watch3"]
];
function CheckDate(month,dayno){ //begin function
var retval = new String(dayno);
var m = month + 1;
for(var app = 0; app < apps.length; app++){ //begin for-loop
if(m == apps[app][1] ){ //first month (if 1)
if(apps[app][3] - apps[app][1] > 0){ //if 2
if(dayno >= apps[app][0]){ //if 3
switch (apps[app][5].toLowerCase()){ //switch
case "holiday":
retval = "<div class='hol' title='" + apps[app][4] + "'>" + dayno + "</div>";
break;
case "watch1":
retval = "<div class='watch1' title='" + apps[app][4] + "'>" + dayno + "</div>";
break;
case "watch2":
retval = "<div class='watch2' title='" + apps[app][4] + "'>" + dayno + "</div>";
break;
case "watch3":
retval = "<div class='watch3' title='" + apps[app][4] + "'>" + dayno + "</div>";
break;
default:
retval = "<div class='error' title='" + apps[app][4] + "'>" + dayno + "</div>";
break;
} //end switch
} //end if 3
else { //else 1
if(dayno >= apps[app][0] && dayno <= apps[app][2]){ //if 3
switch (apps[app][5].toLowerCase()){ //switch
case "holiday":
retval = "<div class='hol' title='" + apps[app][4] + "'>" + dayno + "</div>";
break;
case "watch1":
retval = "<div class='watch1' title='" + apps[app][4] + "'>" + dayno + "</div>";
break;
case "watch2":
retval = "<div class='watch2' title='" + apps[app][4] + "'>" + dayno + "</div>";
break;
case "watch3":
retval = "<div class='watch3' title='" + apps[app][4] + "'>" + dayno + "</div>";
break;
default:
retval = "<div class='error' title='" + apps[app][4] + "'>" + dayno + "</div>";
break;
} //end switch
} //end if 3
} //end else 1
} // end if 2
else if(m == apps[app][3]){ // second month (else if 1)
if(dayno <= apps[app][2]){ //if 2
switch (apps[app][5].toLowerCase()){ //switch
case "holiday":
retval = "<div class='hol' title='" + apps[app][4] + "'>" + dayno + "</div>";
break;
case "watch1":
retval = "<div class='watch1' title='" + apps[app][4] + "'>" + dayno + "</div>";
break;
case "watch2":
retval = "<div class='watch2' title='" + apps[app][4] + "'>" + dayno + "</div>";
break;
case "watch3":
retval = "<div class='watch3' title='" + apps[app][4] + "'>" + dayno + "</div>";
break;
default:
retval = "<div class='error' title='" + apps[app][4] + "'>" + dayno + "</div>";
break;
} //end switch
} //end if 2
} //end else if 1
else if( m > apps[app][1] && m < apps[app][3] ){ //else if 1
switch (apps[app][5].toLowerCase()){ //switch
case "holiday":
retval = "<div class='hol' title='" + apps[app][4] + "'>" + dayno + "</div>";
break;
case "watch1":
retval = "<div class='watch1' title='" + apps[app][4] + "'>" + dayno + "</div>";
break;
case "watch2":
retval = "<div class='watch2' title='" + apps[app][4] + "'>" + dayno + "</div>";
break;
case "watch3":
retval = "<div class='watch3' title='" + apps[app][4] + "'>" + dayno + "</div>";
break;
default:
retval = "<div class='error' title='" + apps[app][4] + "'>" + dayno + "</div>";
break;
} //end switch
} //end else if 1
} //end if 1
return retval;
} //end for-loop
}
function PrintMonth(month){
var done = false;
var day = 0;
document.write("<table class='inner'><caption><b>" + months[month] + "</b></caption><thead>");
document.write("<th>Mon</th><th>Tue</th><th>Wed</th><th>Thu</th><th>F r i</th><th>Sat</th><th>Sun</th></thead>");
while(!done){
document.write("<tr>");
PrintWeek(month,day, firstdays[month], daycounts[month]);
document.write("</tr>");
day = day + 7;
if( day > daycounts[month] + firstdays[month]){
done = true;
}
} //end of while-loop
document.write("</tbody></table><p>");
} //end of function
function PrintWeek(monthno,start,min,max){
var d;
var desc;
for(var j = 0; j < 7; j++){
document.write("<td>");
d = start + j;
if(d >= min && d < max + min){
desc = CheckDate(monthno,d - min + 1);
document.write(desc);
}
document.write("</td>");
} //end of for-loop
} //end of function
[/code]
EDIT: Nope, fixing the first toLowerCase() call didn't help. -
The $rstart variable is fine...all it does is specify which table row to start on for pagination.
I put an ampersand before the forum_id, but I'm not sure if it worked yet. I use Google for reading my feeds, and sometimes it takes a while for any changes to become apparent. -
I have a script that creates an RSS feed for one of the sites I have. It was working fine, but now it's parsing the info wrong and I'm not sure why. All it's supposed to do is return a message board post. Below is the code that creates the link:
[code]
<?php //just to turn on the pretty colors
<link>".$base."forum/viewthread.php?".$rstart."forum_id=".$row['forum_id']."&thread_id=".$row['thread_id']."#post_".$row['post_id']."</link>\n
?>
[/code]
The problem lies at the forum_id - & segment of the code. Instead of returning something like:
website.com/forum/viewthread.php?rowstart=20[b]forum_id[/b]=4[b]&[/b]thread_id=25#post_66
I get:
website.com/forum/viewthread.php?rowstart=20=4thread_id=25#post_66
Any ideas on why this is screwing up? And I haven't changed the code in months, so it's not as though I screwed it up by tweaking it myself. -
[quote author=Jenk link=topic=111347.msg451328#msg451328 date=1160741510]
they may have been referring to short tag '<?'
Wihtout an example it's difficult to say :p
[/quote]
Yeah, and of course the post I originally referred to didn't have any examples, only a passing mention of old vs. new syntax.
Oh well, so long as I can get my scripts to work, it doesn't really matter. :) -
The forum doesn't seem to like the code, so I'll just link to where I posted it on the other message board:
[url=http://www.ozzu.com/ftopic69734.html]http://www.ozzu.com/ftopic69734.html[/url]
I can't link directly to my message, but it's the last one in that thread. -
I've been trying to help out someone on another message board with tweaking a calendar script they have. The script allows for appointments to be made by passing values into an array within the code itself (not the way I would've done it, but that's neither here nor there). Originally, any appointments made would turn the days selected red to highlight the span of time they would take. Since the user is a firefighter, he wants the ability to have different colors available for different watch shifts. I figured that the easiest way to adapt the existing script to that would be to add another element to the array as a flag, then have a series of switch statements that would see what flag (if any) were set and highlight those dates with the correct color. Unfortunately, the code is only displaying the first appointment and nothing else. I think the problem is in checkDate's for-loop, but I can't see it. I originally thought that the switchs' break statements were ending the loop, but I don't think that's it.
Below is all of the code...it's long. I don't like that all of the JavaScript is in the head rather than included as an external file. I also don't like how the calendar is created, but since I can't get the basic functionality of the script to work correctly I don't want to re-invent that part of the wheel just yet. The comments on every checkDate line were to help me match brackets. Well, anyway, here's the code:
EDIT: I think I have to put it in a separate post because of its length. -
[quote author=businessman332211 link=topic=110237.msg451333#msg451333 date=1160741675]
You must have everything wrong.
I am a web developer, you seem to want to stunt people who seek knowledge.
I don't care what anyone say's there is nothing wrong with continuing to learn, if you had not of heard me in my career, I made a lot of major career choices lately.
I stopped trying to do graphic/design, layout/design, content writing
I have a friend who has ton's of experience, we are helping each other in a partner sort of way.
As for the rest I am interested in other forms of web development, coding in css/xhtml
programming
general web design, coding, programming
I still do everything, I get the project, do all the planning, send the specs to him for a picture of a logo/layout
I code/program organize everything, adn I do the content, the send it to him to proofread. Granted it might have been hard trying to master graphic design/photography and still keep my knowledge in other areas mastered.
I also know full well everything you said I didn' tknow, I sometimes get back into research, I study I ask questions to see what other people know, maybe there were things about something i didn't know.
[/quote]
Am I the only one thinking there's a language barrier problem here?
And, businessman, with all due respect, how can anyone here be categorized as wanting to stunt people who seek knowledge when they're volunteering their time to post on this board? I've seen nothing but great patience with the other members here as they've given you a lot of advice over and over again. That you very rarely follow through with their advice, resulting in frustrating the people who are trying to help you, is [b]your[/b] problem, not theirs.
No one is saying that you shouldn't learn. What they're (we're) saying is that you should pace yourself. A professional knows their strengths and limitations, not just with their skillset, but with [b]how[/b] they learn and [b]how much[/b] they can learn in a given time. I think it's pretty obvious that you're pushing yourself too hard. Like I said before, you don't need to learn all of this stuff all at once.
Since you call yourself a businessman, acting like a professional is in your best interest. Ironically, you've acted anything but professional to this point. Yes, you've asked questions. Many questions. And others have given you advice as a response. What do you do in return? You ignore it. They repeat their advice, and you still ignore it. When they offer constructive, if occassionally blunt, criticism you either ignore it outright or make statements (like the ones that started your last message) saying that they are mean and don't like you or that they're stifling your growth.
And no, none of this has [b]anything[/b] to do with your graphic design problems. I know others have said that they're not particularly good at graphic design. I'm not good at it either. It's a moot point. What this [b]is[/b] about is your insistance in thinking that everyone here is either wrong or mean when they don't pat you on the back or when they get frustrated with you asking the [b]same[/b] questions over and over again.
Being a businessman, a true businessman, does not mean acting like a petulant child. Yes, criticism can hurt, especially when you know you put a 100% effort into something. But this is the real world now. It's either sink or swim. If you cannot handle criticism, if you will not heed the advice of your contemporaries, then you're not a businessman. You're a guy with a hobby who has a fragile ego.
Please take this advice in the spirit in which it is intended. Like I said before, I'm in a similar situation as you, so I do want to see you succeed. -
[quote author=MrLarkins.com link=topic=111381.msg451313#msg451313 date=1160739628]
[code]$main = mysql_query("SELECT id FROM ibf_members WHERE mgroup=4");
$main = mysql_fetch_array($main);
$id = $main['id'];[/code]
here is my code. I know for a fact that there are five entries in the database in column id where mgroup=4, however when i do
[code]$count = count($id);
print("$count");[/code]
i get 1
isn't $id an array here? or did i do something wrong?
[/quote]
$id isn't an array...$main is an array, with each element being a column in your table. Right now, you're getting 1 because you've only accessed the id from the one record you've accessed. Try doing this:
[code]
<?php
$query = "SELECT id FROM ibf_members WHERE mgroup=4";
$result = mysql_query($query);
$ids = mysql_num_rows($result); //returns the number of rows returned by your SELECT query
echo "$ids\n";
?>
[/code]
If you want to get to info from each returned row, you'll have to loop through them as the mysql_fetch functions only return one row at a time. So something like:
[code]
<?php
$query = "SELECT * FROM ibf_members WHERE mgroup=4";
$result = mysql_query($query);
while($row = mysql_fetch_array($result)){ //while you have records available from the SELECT query
/* process the record, typically with something like:
$somevar = $row['somecolumn'];
do something with $somevar */
} //loop automatically ends when there are no more records available from your SELECT query
?>
[/code] -
You're trying to insert both $country (which is defined) and [b]$countryString[/b] (which [b]isn't[/b] defined) into the database. You don't seem to have a countrystring column to put it into, so you're overrunning the boundary of your table by trying to insert a record that's one column too large.
-
[quote author=wildteen88 link=topic=111347.msg451290#msg451290 date=1160733889]
I guess what they mean by "old syntax" is the use of register_globals.
For example if your PHP setup has register_globals enabled, instead of using $_GET['url_var_here'] you used $url_var_here
The "new syntax" is to disable register_gloabls (which is off by default as of PHP4.2.x) and use the new superglobals which are $_GET, $_POST, $_SESSION, $_COOKIE etc
Another new sytax change is the old superglobals which are the $HTTP_*_VARS. For example instead of using $HTTP_GET_VARS you use $_GET instead for accessing vars in the URL. Or instead of $HTTP_POST_VARS your use $_POST etc.
[/quote]
I dunno if that's what the other person was talking about as my version of the book only uses the current superglobals. I think it may have something to do with 'arrow' syntax ($something -> something) that I see in some people's code. How does that kind of thing work? -
I don't know if it's my place to say anything, but I'm bored, so why not? ;P
businessman, I have two very important words that I think you should heed: [b]Chill. Out.[/b]
You don't need to cram your head silly with everything under the sun all at once. Hell, I'm in a similar boat as you, learning on my own at home. I fell into the same trap you're in now...a lot of these technologies rely or use other technologies to work, so where should I go next? What's XHTML without CSS? PHP without MySQL? Or AJAX without JSON? And, like you, I tried cramming my head with everything all at once. And, ultimately, I learned nothing.
You need to structure your learning. What do you know? What do you know [b]well[/b]? If you know something really well, then learn the technologies/languages associated with it. If you're comfortable with MySQL, try PHP. Good at JavaScript? Try doing some object oriented stuff with it.
I think it's also important that you realize that memorization is not the same as learning. Being able to recite all the built-in PHP functions in alphabetical order may be a neat trick, but what's really important is knowing what they do and when to use them. I doubt anyone here has memorized every bit of PHP, but I don't doubt that, if they run into a problem, they can either lookup the proper function to use or create one themselves.
Finally, you've got to pace yourself. Like others have said, doing nothing but studying and coding in the hopes of becoming Master of the Universe is self-defeating. You've got to give your brain a chance to rest. Something that helps me is playing video games on my PS2 (I like to stay away from the PC during my relaxing). I find that I not only become reinvigorated while kicking the snot out of the AI in Tekken (gotta release that frustration), but my brain still works on whatever I'm learning in the background during play anyway.
In closing, just relax, man. The world won't stop spinning if you don't become an ace programmer tomorrow. -
I asked this in the miscellaneous category a while back and never got a response, so I figured I'd ask here as it seems like a better section in which to post this.
I have the Larry Ullman/Peachpit book (the one up to PHP4 and MySQL4). I saw someone make a comment that the book uses the 'old' PHP syntax. What is an example of the 'old' synatx, and what is an example of the 'new' syntax?
Thanks :) -
[quote author=Jenk link=topic=111314.msg451115#msg451115 date=1160684806]
[code]<?php
abstract class A
{
public function fooBar()
{
echo 'Foobar!';
}
}
class B extends A
{
}
$obj = new B;
$obj->fooBar();
?>[/code]
[/quote]
...duh, I should've known that. :D
Thanks. :) -
[quote author=Jenk link=topic=111314.msg451021#msg451021 date=1160674626]
When building a set of classes that share common functionality, it's useful to bundle that functionality into one class. Sometimes that functionality is useless on it's own and must be extended to complete the class; thus abstract comes into play :)
Not every method has to be abstract within an abstract class, infact you don't have to have any abstract methods at all.
[/quote]
So how would an abstract class be 'connected' to a non-abstract class that needs the functionality the former has?
innerHTML + XHTML = bug
in Javascript Help
Posted
I know there are createElement and appendChild methods. But I need to be able to write within the elements my script creates as well. Is there a way to do something like a document.write within my body element? Is write available for an element retrieved with a getElementByID?