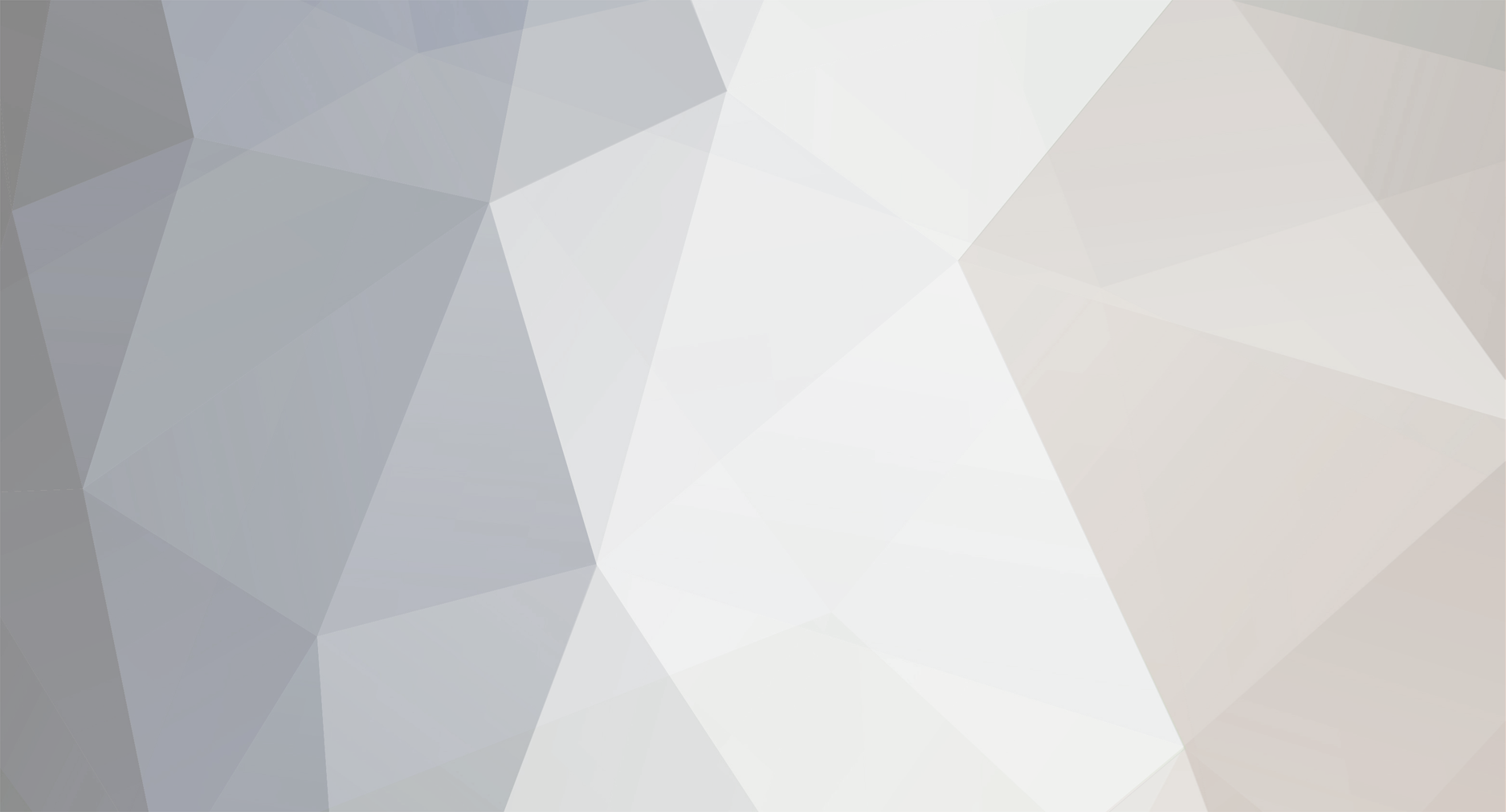
KevinM1
Moderators-
Posts
5,222 -
Joined
-
Last visited
-
Days Won
26
Everything posted by KevinM1
-
Tutorials are generally a bad way to learn as they're often only concerned with creating a certain thing. They're not designed to teach you how to program, but rather only to complete a certain task. It's like expecting to be able to build a house when all you've learned is how to build a table. The same goes for the PHP manual, which has value in showcasing the parts of the language, but not much in terms of script/site design or best practices. So, how do you learn to do it? Solid resources (good books) and lots of practice, all while being plugged into the community for guidance. And, programming does build on itself like math. Primitive types -> arrays -> functions -> objects. Conditionals -> loops. For books, here are the three I used. Read them in order: PHP for the Web: Visual QuickStart Guide (4th Edition) PHP 5: Objects, Patterns, and Practice Design Patterns: Elements of Reusable Object Oriented Software They're not all-encompassing, but they, along with constant practice and research on your end, will get you comfortable with PHP and basic OOP.
-
The test isn't difficult if you have a solid grasp of OOP fundamentals. Not syntax, but the real fundamentals. The test is asking a few things: 1. Does the person know how to use abstract classes? 2. Does the person know the Factory Method, perhaps the most common creational pattern in use? 3. Can the person use objects in a loop while testing for certain edge cases (the special abilities of each warrior)? OOP is the dominant programming paradigm. That's not a value statement, merely an observation. It's something new developers need to learn if they want to work for someone else.
-
Do you know any OOP? This project is perfect for some basic OOP. It can still be done without it by using associative arrays. Here's how I'd approach the problem: 1. Your first page would be a simple form with two <select> elements and a submit button. The <select> options would be the kinds of warriors the player could pick - Ninja, Samurai, or Beserker. So, just to be absolutely clear, two drop down elements, each having the names of the kinds of warriors a player can choose. 2. That data would then be submitted to another page. This page would take the values of the drop downs and either create the appropriate objects (which is really the way to go) or associative arrays based on those values. 3. Once it's determined what kind of warriors to create, you need to go about filling their properties. For the random numbers in a range, you can use mt_rand like so: $ninja = array(); $ninja['health'] = mt_rand(40, 60); $ninja['attack'] = mt_rand(60, 70); // etc. For the evade property, you can fudge a decimal by dividing the result of the random number generator by 100: $ninja['evade'] = mt_rand(30, 50)/100; 4. From there, it's a matter of figuring out the game engine. a. Figure out which warrior goes first (you can get a 50/50 chance by using mt_rand and basic math). b. Have them attack each other for 30 turns (sounds like a loop...) c. Check if they meet the criteria for one of the special abilities/functions (if statements in the loop...) d. Echo the result of each turn to the screen You should be able to figure it out from here.
-
Sounds like your university took a "Survey of Web Development" approach to things, which is really pretty limiting. Professionals tend to start with one core non-HTML language, then branch out to others as their skills improve and they become better programmers in general. Look no further than the "How long have you been writing code for?" thread for proof of that. Did you go to school for four years, or two? Generally speaking, 'university' means four year bachelor's program, while 'college' means two year associate's program, although there are exceptions (Boston College, for one). In any event, there's no real way to get better than to roll up your sleeves and work on your deficiencies. I'm sure that's not the answer you want to hear, but that's the reality of the situation. To help you identify your weak areas, here's a general checklist I'd look for if I was hiring an intern/entry level coder (no particular order): 1. Form handling, including sticky forms, and input validation/escaping/general security 2. Array creation and manipulation 3. Basic database manipulation/CRUD 4. Comfort with logical structures - conditionals and loops 5. Decently written code (good variable names, good script structure, DRY, Separation of Concerns) 6. Comfort with creating custom functions. *Basic OOP skills (not mandatory, but a bonus) **You'd get fired immediately for using 'global' So, look at that basic list, see where you're weakest, and work to make it a strength.
-
What areas are you struggling with? What did your courses at school cover?
-
You need to use the Factory Method here: abstract class WrapperFactory { public static function GetById($id) { switch($id) { case 1: return new Business_solutions_cat(); break; case 2: return new Blackberry_cat(); break; // etc. } } } $wrapper = WrapperFactory::GetById($id); $newParent = $wrapper::findAll();
-
getElementsByTagName returns an array of elements. Arrays don't have a href property. You need to access an individual element by supplying an index, like: console.log(link[0].href); // logs the href of the first element in the array
-
call a program stored in my hard disk from my website?
KevinM1 replied to mariam's topic in PHP Coding Help
I think they want a Java applet to run on the user's screen when someone interacts with a PHP-driven web page. -
Don't go to w3schools. Go here instead: https://developer.mozilla.org/en-US/learn/
-
It's just a simple JavaScript form submission that fires on the <select> element's onchange event. To do it without a page refresh, use AJAX during the event instead of a 'normal' submission. And, just to be clear about ASP.NET's postback/viewstate capabilities, postback works almost exactly how thorpe's suggestion works. Hidden inputs are injected into the page. The viewstate is simply a base-64 encoded string containing the values of the server control properties on the current page. This encoded viewstate meta-value is set as the value for one of the hidden inputs, where it is decoded and parsed on the back end during page rendering. For more, see: http://msdn.microsoft.com/en-us/library/ms972976.aspx You can do essentially the same thing with PHP and hidden inputs, with the added benefit of not having to concern yourself with ASP.NET's horrid page lifecycle.
-
Use preg_split.
-
Pressing the # button is fine, but, like I said before, with long files you need to post only the relevant code. Your script was too long, which made the code you posted hit the character limit, thereby removing the closing [/code] tag. That, in turn, made your code appear as regular text, which created a mountain of vertical scroll.
-
HTML/CSS/JavaScript - 8 years (rusty with them, however) PHP - 5 years C#/ASP.NET MVC - 1.5 years
-
Also, if you're going to learn any .NET language, learn C#.
-
Isn't what you're asking for part of the assignment itself? Regardless, PHP Freaks isn't here to help with homework (see: http://www.phpfreaks.com/page/rules-and-terms-of-service#toc_forum_do_nots - specifically #11).
-
Your best bet is to stick the complicate to write data in variables, like so: var checkboxLength = document.forms["formName"].elements["checkbox[]"].length; var checkboxes = document.forms["formName"].elements["checkbox[]"]; for(var i = 0; i < checkboxLength; ++i) { if(checkboxes[i].checked) { // do stuff } } You not only make it easier to write doing it this way, you also make it more efficient in the long run as you store the checkboxes in a variable before the loop rather than walking down the DOM to obtain a new reference on each iteration.
-
So long as they're in scope when you invoke your function, you'll be fine. --- Stepping back a moment, there seems to be some confusion on your part regarding both defining and invoking a function. So, hopefully, I'll be able to clarify some things for you in that regard. A function definition is the body of the function. So, something like: function exampleFunc($arg1) { // do things with $arg1 } is a definition. The function is not invoked at that point. It's simply a blueprint. Invoking a function is relatively simple. All you need to do is write out the function name, put parameters into its argument list (if any are needed), and place a semicolon at the end. Example: $x = 5; exampleFunc($x); In your case, you define a function with several expected parameters: But you neglect to actually pass any parameters in when you actually try invoking your function: CheckCertStatus(); If you define your function to take parameters, you need to manually insert them when you actually invoke your function. So, you need to do: $hostName = 'localhost'; $user = 'me'; $pass = '******'; $quizDB = 'quizDB'; $DBTable = 'quiz'; CheckCertStatus($hostName, $user, $pass, $quizDB, $DBTable);
-
Please use either or tags to display code.
-
Interfaces define, well, an interface. Method signatures which must be fleshed out in any class which implements them. They're sort of a way around PHP (and other languages) not allowing true multiple inheritance. They're also used to foster polymorphism. Much like an abstract class, an interface denotes a is-a relationship. An object of a class that implements an interface is seen as an instance of that interface. So, something like: class Example extends Parent implements ISomethingElse {} $example = new Example(); Would be considered to be an instance of Example, Parent, and ISomethingElse to PHP. Really useful if you have something like: class CashRegister { public function calculateTotal(ISomethingElse $items) { $total = 0; foreach($items as $item) { $total += $item->getPrice(); } return $total; } } Examples are obviously canned, but interfaces definitely have their uses. Take a look at the Observer Pattern as another example.
-
The best validation strategy is to use JavaScript for responsiveness and to give the user feedback immediately, while using the back end language (likely PHP in this case) for security. That said, yes, you can use JavaScript to validate a textarea and stop a form from submitting it the textarea doesn't include any text. Just realize that this validation does nothing to secure your site. It's simply a user interface nicety. My JavaScript is a bit rusty, but try something along these lines: var form = document.forms["myForm"]; // substitute 'myForm' with whatever your form name actually is form.onsubmit = function() { var re = /.+/; var textExists = re.match(this.elements["textarea"].value); // replace 'textarea' with whatever your textarea's name actually is if(!textExists) { // highlight the textarea? return false; } }
-
Escaping works differently dev vs. production
KevinM1 replied to shamwowy's topic in PHP Coding Help
^ You can make it even simpler with array_map. -
The best solution would be to have all your form processing within the following conditional: if (isset($_POST['submit')) { $form_message = false; // more form processing } if (isset($form_message) && $form_message == true) { // display HTML }