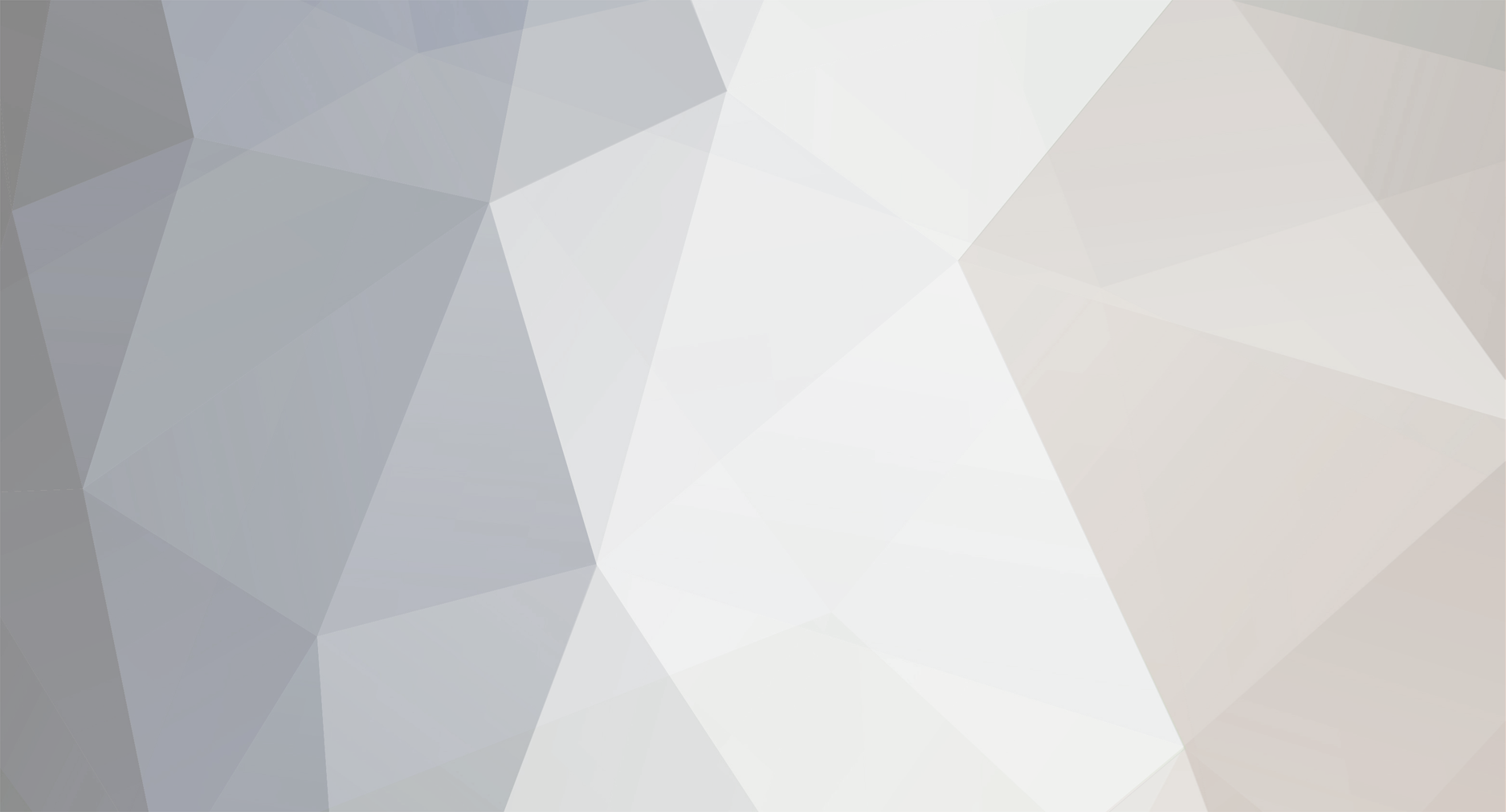
KevinM1
Moderators-
Posts
5,222 -
Joined
-
Last visited
-
Days Won
26
Everything posted by KevinM1
-
C++ I bought a time machine and want to learn it
KevinM1 replied to cssfreakie's topic in Other Programming Languages
I think it's well worth it. Both languages really highlight what goes on under the hood, especially when it comes to memory management. There's an epiphany that almost everyone gets when they build their first dynamically linked list. Seeing the memory allocation/de-allocation at play, pointers doing their thing, what references and addresses actually mean.... You'll also see one reason why the idea of type is important. PHP is a dynamically typed language, but type definitely does exist in the language, even if it's not explicit. OOP looks at type in a more abstract way, but at least you'll be forced to deal with it from the outset. You'll start to see why I and everyone else trying to do OOP the right way harps on it. I recommend the two Thinking in C++ books. They're both fairly big, but do a great job exploring the language in an engaging way. If you get nothing else out of it, you'll at least see how simple PHP is in comparison. -
Was just going to post something like this myself. Definitely the way to go.
-
You shouldn't pass your objects by reference. PHP 5 automatically does it for you, and, really, there's no good reason to not use PHP 5 at this point. It's at least 5 years old now. Also, you're relying way too heavily on the Singleton pattern. Singletons should be used sparingly, if at all, as they're global. There's no need to break scope for this. What you should do is start with your routing system. You need a front controller sitting on top of your system to intercept requests. MVC controllers are really command objects - they're instantiated, and invoke certain functionality, based on the incoming HTTP request. They obtain the correct model from a repository based on GET values, and essentially act as a DI container for both the model and the view.
-
You have categories and sub-categories, right? Logically, a sub-category is a category. parent_id stores the id of that particular category's parent, if it has one. id name parent_id 1 Apparel 0 2 Men's 1 3 Women's 1 In this example, you have Apparel as a top-level category. It has two sub-categories, Men's and Women's. Apparel's parent_id column contains 0 as that's not a valid id in this table (remember, categories.id auto-increments and starts at 1). That tells us it's a top-level category. None of this has anything to do with a product per se. Products enter the mix when you use its foreign key. So, let's say you want to look at a product in Men's Apparel. A t-shirt or something. Your first query, when you process the incoming category id, would be: "SELECT * FROM products, categories WHERE categories.id = 2 AND products.category_id = categories.id" That would get you everything except the parent category (in this case, Apparel) info. So, you'd need to pass the parent_id value obtained from the previous query into a new query: "SELECT name FROM categories WHERE parent_id = 1" Remember, the 1 comes from the result of the previous query. Like I said, there may be a way to get it all in one query. But this is the general process I'd use. It allows you to nest categories, and keeps product data from being tangled with category data, which is what you want.
-
Has anyone tried it? I love LINQ, so seeing a basic version ported over to PHP is great, but I can't help but think it would add a horrible performance hit. I'm wondering if anyone has worked with it, or if I'll need to benchmark it myself. JSLINQ is awesome, especially when you need to drill into deep JSON results.
-
Okay, before you go much further, you need to strengthen your db design. Your tables aren't normalized, which is causing confusion and will make it progressively harder to insert and retrieve data. Here's what I'd do for tables: Products: id name price details location date_added category_id Categories: id parent_id name Then, you could do something like: "SELECT * FROM products, categories WHERE categories.id = $idc AND products.category_id = categories.id" This will give you everything except the parent category name. You can get that from an additional query: "SELECT name FROM categories WHERE parent_id = {$row['parent_id']}" There may be a better query which could do all of that in one statement. SQL isn't one of my strengths, so you may want to ask in our MySQL sub-forum. Still, you need to normalize your tables, else you'll keep getting into these situations where you need to contort your queries in order to get some simple data sets. Read: http://dev.mysql.com/tech-resources/articles/intro-to-normalization.html
-
I'm not entirely sure what you want to display. Can you list your tables, and highlight which columns you want from each?
-
I might do that. I don't know if I'll ever get around to finishing it, so someone else may as well take my half-baked ideas and see what they can do. It's an ASP.NET project, but aside from the hoops I had to jump through to successfully hook into its Page object's lifecycle (which was a PITA), and some LINQ to read in the data from the XML file, the majority is straight OOP code. Anyone should be able to lift most of it with minimal translation.
-
I think you mean RPG.... I was making my own a little while ago. Single player, so it wasn't a MMO, but the combat engine was the heart of it. Here's the gist of it: Both PlayerCharacters (PCs) and Enemies derived from a base Character class. PCs could be one of three classes - melee DPS, hybrid/status effect class, ranged DPS/mage. Characters had a list of Attacks, which were objects. Every class had access to the basic attack. Specialized attacks were class-dependent. New attacks were gained every 3 character levels. Note that 'Attack' was a generic class... some of them weren't attacks in actuality, but buffs/debuffs. Attacks could also have status effects tied to them. These effects would attach themselves to the targeted Character (on a successful hit) via the Observer pattern, and each effect had a duration. They would detach themselves from the Character when the duration reached 0. All of this class/attack/status effect data was saved as XML files so I could add new classes, attacks, and effects without having to hard code much into the system. It was as simple as simply dropping a new XML file into the correct directory. Enemies had their own, simpler XML files. Combat itself was turn-based. 50/50 chance of the Enemy attacking first. All of the rest of the attacks happened in sequence. I forgot what math I used, but I made sure there was a sliding miss percentage based on the Character's level. That way, good equipment wouldn't totally make one's level useless. I never got around to finishing it, but I had the basics of the combat system working. I stopped during the status effect design phase due to real life things.
-
If check0 is supposed to represent an array of values, you most likely have to put its name as check0[] in your HTML form. That will tell PHP to treat it as an array on the back end.
-
Also, unless you're using PHP 4, you shouldn't use the object's reference ('&') during assignment. In PHP 5, objects are automatically passed by reference.
-
1. It comes from this line: echo "print_r = " . print_r(true); True can also be represented as 1. In binary, 0 is false/off while 1 is true/on. As a bit of trivia, that's why power switches have a 1 superimposed over a 0 as their symbol. 2. Yes, it will cause the form to submit to itself via POST. 3. It depends on what you need to do. Forms that submit to themselves are pretty common as it makes it easy to handle/display form field errors.
-
In the future, please put your code within tags.
-
Like I said before, values appended to the end of a URL (web address) as a query string are being passed via GET. Let's say you have a URL like www.example.com/index.php?firstname=forrest&lastname=gump The '?' denotes the beginning of a query string. Query strings contain name/value pairs. So, as you can see from this example, there are two pairs - firstname, which has the value forrest, and lastname, which has the value gump. To grab a hold of these in PHP, you'd need to access them through the $_GET superglobal array, like so: echo $_GET['firstname']; POST is different in that the data sent is passed in the background. There's no query string with POST. To submit the form as POST, you need to specify it as the value of the method attribute of your form tag: <form action="" method="post"> Just be aware, like I said above, when you're passing data via POST, your address will simply be http://localhost/00_MyWebSite/zzEmptyPHP.php. You won't have a query string.
-
Values appended to the end of a URL as a query string are passed via GET. You're checking to see if $_POST['submit'] is set.
-
Actually, the link is visible if you expand the header.
-
I'm trying to help you by steering you in the right direction to learn what the code actually means. Copying code isn't going to teach you how to program. You need to be able to know what it is you want to do, and then figure out the code necessary to do it. I've gone just about as far as I can short of writing your code for you. Maq and I have explained exactly why you're getting an error, and what you should learn in order to fix it. Your issue is larger than something that can be solved by changing a line of code. Your script has a fundamental logic error. This error has been explained. It's up to you to take that explanation and figure out how to apply it to your script. We can't do it because we don't know what your id is supposed to represent nor how it's supposed to be generated. Finally, if you're going to learn PHP, the best free resource is the online manual: http://www.php.net/manual/en/langref.php If English isn't your native language, you can pick which locale is best for you: http://www.php.net/docs.php That said, you should still probably invest in a good book or two, just to see different ways to go about solving common problems, and to reinforce what you learn.
-
You really need to stop and take a look at the process. There is no id value. You have: <input name="id" type="hidden" value="<?php echo 'id'; ?>" /> Which if you look closely, will simply echo the word 'id'. This is probably not what you want, and is most likely a typo. That typo aside, you still wouldn't have an id value because you never set one in your code before the form is displayed. You need to slow down and map the process you're trying to create by hand before attempting to code it. Pen/pencil and paper. Really. You have a glaring logic error you need to fix. You also clearly need to learn about POST, GET, and what their differences are, as the lack of that fundamental understanding is part of your problem here.
-
In static functions, you need to use $self::member name rather than $this. Why? $this refers to an instance. Static applies to a class, not an instance of a class (object), so $this doesn't apply.
-
When you write an SQL query that tries to pull data from two or more tables, the WHERE clause will apply to ALL of them. So, you're really asking: SELECT * FROM products WHERE products.category_id = some id // <-- good query AND SELECT * FROM categories WHERE products.category_id = some id // <-- bad query, because products.category_id doesn't exist in the categories table When joining tables, your WHERE clauses are very important. They're what actually decides the returning data set. Since you want to join some results from one table with another, you need to specify the link between them in the WHERE clause. So, something like: SELECT * FROM products, categories WHERE products.category_id = categories.id AND products.name = 'Fork' Will work because of the AND. The first comparison ties the tables together (you're joining them on the category id). The second one narrows the result set down to just forks. I hope this makes some sense.
-
If that's the case, he has bigger problems than just a failing query. @co.ador: http://dev.mysql.com/tech-resources/articles/intro-to-normalization.html
-
The '@' squelches errors. Remove it and see if simplexml_load_file() is actually generating an error.
-
Why do you need to join them if everything you want is in products? Wouldn't: SELECT * FROM products WHERE category_id = $id Suffice? You join tables when you need to display or manipulate data from both of them. If you're simply spitting out the data of one based on it's foreign key, just supply a value for that foreign key.
-
You're not joining anything here. Your statement is saying: SELECT all categories from products and categories WHERE categories.category_id = something Where's the part that references the products table? You need something in your WHERE clause that ties one of the columns of products to one of the columns of categories.
-
The newline character is working in regard to your generated output (look at your source code in the browser). The 'problem' is that you're looking at it with the browser. To a browser, an HTML line break element (<br>) is what will make a new line visible on the screen. In other words, your generated code is: my string my other string But since browsers ignore white space, it's being displayed as: my stringmy other string To make it look right in the browser, swap \n for <br>