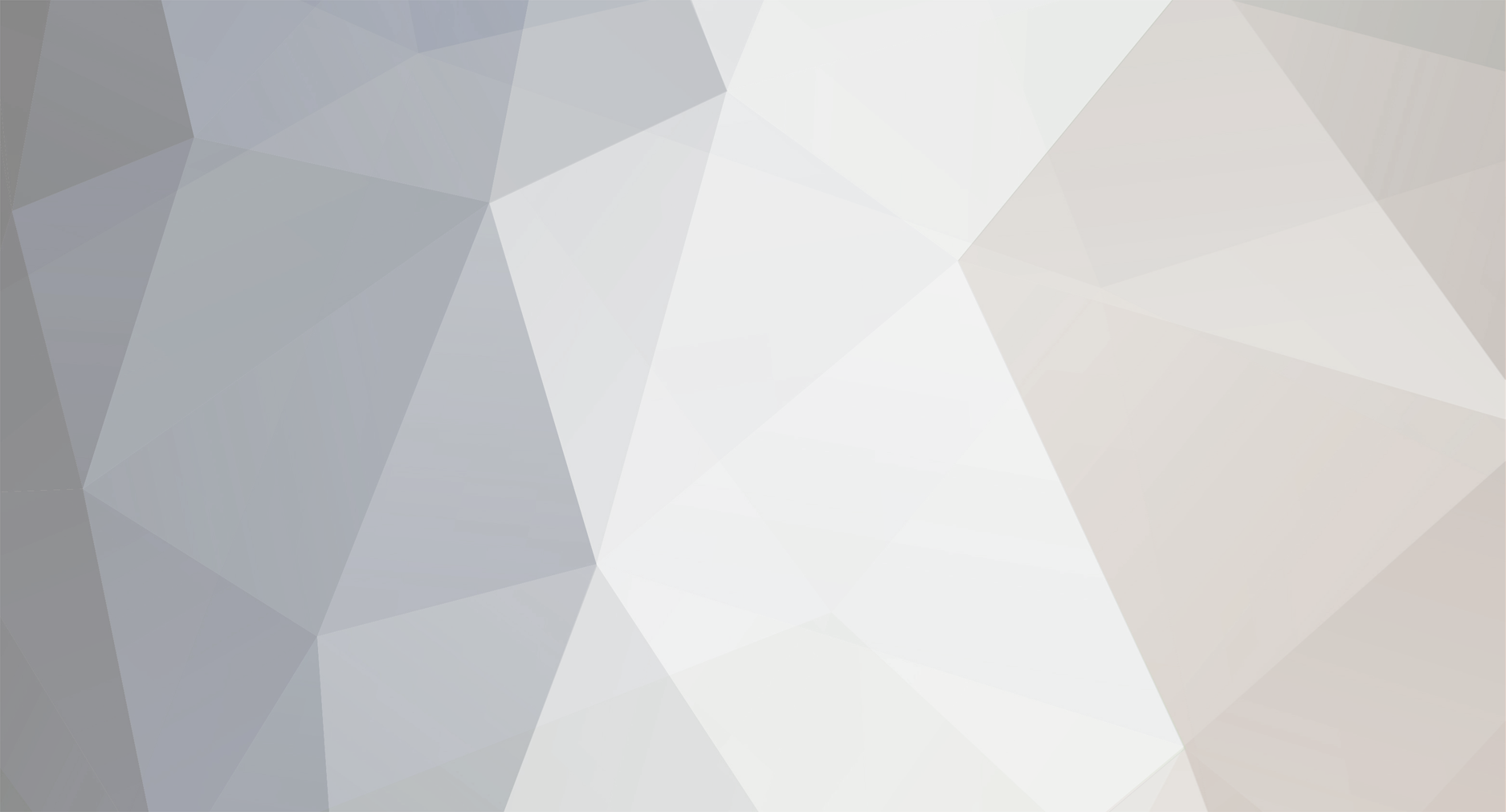
KevinM1
Moderators-
Posts
5,222 -
Joined
-
Last visited
-
Days Won
26
Everything posted by KevinM1
-
$GLOBALS is a superglobal array (like $_GET and $_POST) of all variables currently in global scope. Chances are, your design has an issue if you're using it.
-
I typically use some sort of factory class in order to construct/populate a User object. So, the process would be something along the lines of: User submits login info. User Factory takes the info, and returns a populated User object*. The factory method is static since an object does not need to exist for it to work. Through the User object, the end user can modify their profile info and save it. $user = UserFactory::LogIn($_POST['username'], $_POST['password']); $user->ChangePassword($_POST['newPass']); $user->SaveChanges(); *You may not want to lug around a complete User object from page to page via sessions. You only really need the full object when work needs to be done on it. Instead, you could simply pass around the username through sessions until you really need the full object.
-
You're looking at your design in the wrong way. Your Database class should be a wrapper for MySQLi. It should handle preparing queries, binding and returning results. Your other classes should not inherit from the Database class, but rather contain a reference to it, which is passed in through the constructor. Why? Because right now your entire system is based on you using MySQLi. This is very inflexible, and not the way to do things in OOP. Also, inheritance describes an is-a relationship, where a child object is also a parent object, just with more functionality. It doesn't make sense to look at your content as a database, or your users as databases. In most cases, what you really want to do is compose objects - have one contain a reference to another. This allows you to swap out an object representing a particular kind of functionality (say, MySQLi) for an object representing another kind of functionality (say, PostgreSQL) with no (if written correctly) changes needed to the containing object. Inheritance has its place, but composition is typically the way to go. For your specific issue, simply store the data you want to return in an array, and return that. As in: $query->fetch(); $results = array($title, $heading, $content); return $results; And here's how I suggest you look at your design: class Content { private $db; public function __construct($mysqli) { $this->db = $mysqli; } public function pageInfo($page) { $query = "SELECT pageTitle, pageHeading, pageContent FROM pageInfo WHERE pageName = ?"; $results = $this->db->query($query, $page); return $results; } } class Database { private $mysqli; public function __construct() { // construct your db instance } public function query($query, $args) { // figure out a way to abstract your MySQLi statements return $results; } } $db = new Database(); $content = new Content($db); $pageInfo = $content->pageInfo($page); There are ways to further refine this (dependency injection, for one), but you should get the idea.
-
That means your index is messageid, not messages. In other words, try: $messageid = $_GET['messageid'];
-
My first PHP send form ... how about a review please?
KevinM1 replied to autoworld's topic in PHP Coding Help
This is the last time I fix your post. From now on, put your code inside tags. Example: // my code goes here You have been warned. -
Yeah, I'd use exceptions for those errors. Examples of errors would be if add() was invoked without one of the parameters, or if an illegal error type (if you feel like creating a list of acceptable errors) was passed in.
-
You need something like: $query2 = "SELECT team_id FROM vtp_members WHERE id = {$_GET['r']}"; $result2 = mysql_query($query2); $numrows2 = mysql_num_rows($result2); if ($numrows2 == 1) { $row = mysql_fetch_assoc($result2); $query3 = "UPDATE xxxxx SET yyyyy = zzzzz WHERE team_id = {$row['team_id']}"; // etc. }
-
Keep in mind, technically $age isn't unset by doing that. It still exists, and contains a value of an empty string.
-
You still need to fetch the data from $result2, using either mysql_fetch_array or mysql_fetch_assoc. For the rest, what do you mean by 'store'? Where do you want to use the variable?
-
You have a couple options: 1. Break this method into two methods - one for the ticker list, one for the more robust stock list information. 2. Return an array containing both the tickers and the fleshed out stock list.
-
My first PHP send form ... how about a review please?
KevinM1 replied to autoworld's topic in PHP Coding Help
Remember to put your code inside tags. I did it for you above. -
The problem is that each set of inputs you display in your loop have the same name per-loop-iteration. So, for each db row's worth of inputs you display, you get an input with a name isadmin, an input with a name vnsupUsername, etc. Names, like IDs, are supposed to be unique identifiers. PHP knows this, which is why it can only 'see' the most recent one. Each loop essentially overwrites what PHP can get a hold of. You'll need to either create a unique name for each input during each iteration of your loop, or you'll need to store the values in an array by setting their names to be isadmin[] and vnsupUsername[].
-
HTTP is stateless. Your loss of information isn't due to PHP, but to the nature of the web. The same problem exists in ASP.NET, Ruby, Python, etc. Each request made on the server invokes a new request cycle from HTTP. You need to store your values before making a request. You can, as said above, store these values in sessions. You could also use a database, a file, or even cookies (not recommended for anything non-trivial). Also, coming from a C++ and C# background, you should know that this: Can be a very bad thing. Just because PHP is lax with enforcing type and, except for the most recent version, lacks namespaces, that doesn't mean good practices should be thrown out the window. Take care with any config variables you need, and try not to break scope with them. And, just to nip it in the bud here, never use the 'global' keyword.
-
Your normalization has an issue - you don't need to have pivot tables (like bookAuthors) for a 1:many relationship. Simply put the primary key of the many as the foreign key of the 1, so Books would have an AuthorID column, but Authors would not have a BookID column. Pivot tables, by their very structure, denote many:many relationships. Why? Because each key can be associated with any number of other keys. Example: BookAuthors: BookID AuthorID 1 12 1 45 1 34 2 45 2 77 3 12 3 77 Notice the repeated key values on both sides. AuthorID 12 points to BookIDs 1 and 3, for example.
-
Why is this not ready for production? Because there is no error handling? Yes, and for the other reasons I specified. The class, currently, is merely a sack of errors. What would happen if the same type of error is triggered twice, like, say, the form has two empty fields? How would you want to handle it? Also, would you want to log these errors? Like I said, the class is incomplete. It's meant as a teaching tool, nothing more. Well, like I said above, you most likely want each type of error to be formatted in the same way, so you don't have "empty", "Empty", "EMPTY", etc. when trying to add them to the collection. Nothing special, but not something entirely trivial, either. Displaying an error message to the user is the obvious step. What about the error itself? Does the error matter? Would you want to track them to see if there's an issue with your form design? How would multiple errors on the same field work? Would an empty field also be considered one with invalid characters? How would multiple errors of the same type be handled?
-
Uh... there's a whole section in the manual devoted to OOP: http://www.php.net/manual/en/language.oop5.basic.php And, like I said before, simply return the array of errors and let your display code handle it: class Errors { private $errorMsgs = array(); public function add($type, $message) { if ($type != null && $message != null) { $this->errorMsgs[$type] = $message; } else { // error } } public function getAll() { return $this->errorMsgs; } } $errors = new Errors(); $errors->add("empty", "Empty input"); $errors->add("invalid", "Invalid characters"); foreach ($errors->getAll() as $error) { // echo $error } Keep in mind that this class is very bare-bones. You could add functionality to return the count of multiple errors of the same type, for example. And there's no error handling, or data normalization (error types should probably all pass through strtolower). This was just some code to show the basic idea. It's not ready for production. Exceptions aren't a good idea for something like this. They're used to encapsulate application errors, such as being unable to connect to a db. They also contain technical details that you wouldn't want to show the user. User errors don't qualify, and require better messaging so the user can remedy the problem.
-
class Errors { private $errorMsgs = array(); public function add($type, $message) { if ($type != null && $message != null) { $this->errorMsgs[$type] = $message; } else { // error } } public function show() // <-- not the best design, as it's best to separate processing from display { foreach ($this->errorMsgs as $msg) { // echo errors } } } $errors = new Errors(); $errors->add("empty", "Empty input"); $errors->add("invalid", "Invalid characters"); $errors->show(); This is essentially a 1:1 translation of what you want into a class. Like I say above, it's not the best idea, from a design standpoint, to have a class like this directly output its contents. It muddies up what the class is used for (storing errors and displaying them?). Instead, I'd simply return $errorMsgs from the object and iterate over them in your display code.
-
More to the point, your foreach is simply outputting each inner array as a whole, not their contents. I'm wondering why you're even passing an array into your object since you never seem to do anything with its indices. 'a' and 'b' are hardly descriptive.
-
In your storeMessage method, put: $this->messages[] = $messages; Keep in mind, this will create a 2d array since you're passing in an array to the method.
-
You don't need to turn your array into an object. You need to instantiate your BigClass class. To create an instance, you need to write the following outside of your class definition: $myObj = new BigClass(); // where myObj can have any name you want, since it's a variable $myObj->firstStep($myarray); // calls the firstStep method of BigClass, passing in $myarray as an argument I strongly suggest you read through examples of how OOP works in PHP. The online manual has great code examples. Start here: http://www.php.net/manual/en/language.oop5.basic.php and continue down the list on the left. Finally, 'global' isn't bad because of security issues*, it's bad because it leads to messy, unmaintainable, unextensible, inflexible code. It's a crutch used by beginners who start learning PHP by reading bad online tutorials. It's a real bad habit to get into, and should be remedied as soon as possible, not just for your own sake, but for anyone who has to look at or use your code. *The 'global' keyword is not the same thing as the Register_Globals directive that is now, thankfully, turned off by default in PHP. Register_Globals was very dangerous from a security standpoint because it simply transformed incoming request values into variables. This means that it was simple for outsiders to poison variables used to protect sensitive areas of a site by simply passing in new GET or POST values. See: http://php.net/manual/en/security.globals.php
-
'Global' should never be used, regardless of whether you're writing procedural code or OO code. Period. Argument lists exist for a reason. Also, to the OP, remember that classes are merely blueprints for objects. Classes, themselves, don't execute any code. You need to create a new object instance, and then use it to invoke its methods.
-
You have a ton of code posted... can you simply show the relevant parts? Also, you don't need to use a while-loop if you're only retrieving one row from the db.
-
'Headers already sent' errors usually indicate that you have some output being rendered before you attempted session_start, which is why I asked you if you if inc_theme.php rendered any output.... See also our clearly marked sticky topic at the top of this sub-forum: http://www.phpfreaks.com/forums/index.php?topic=37442.0