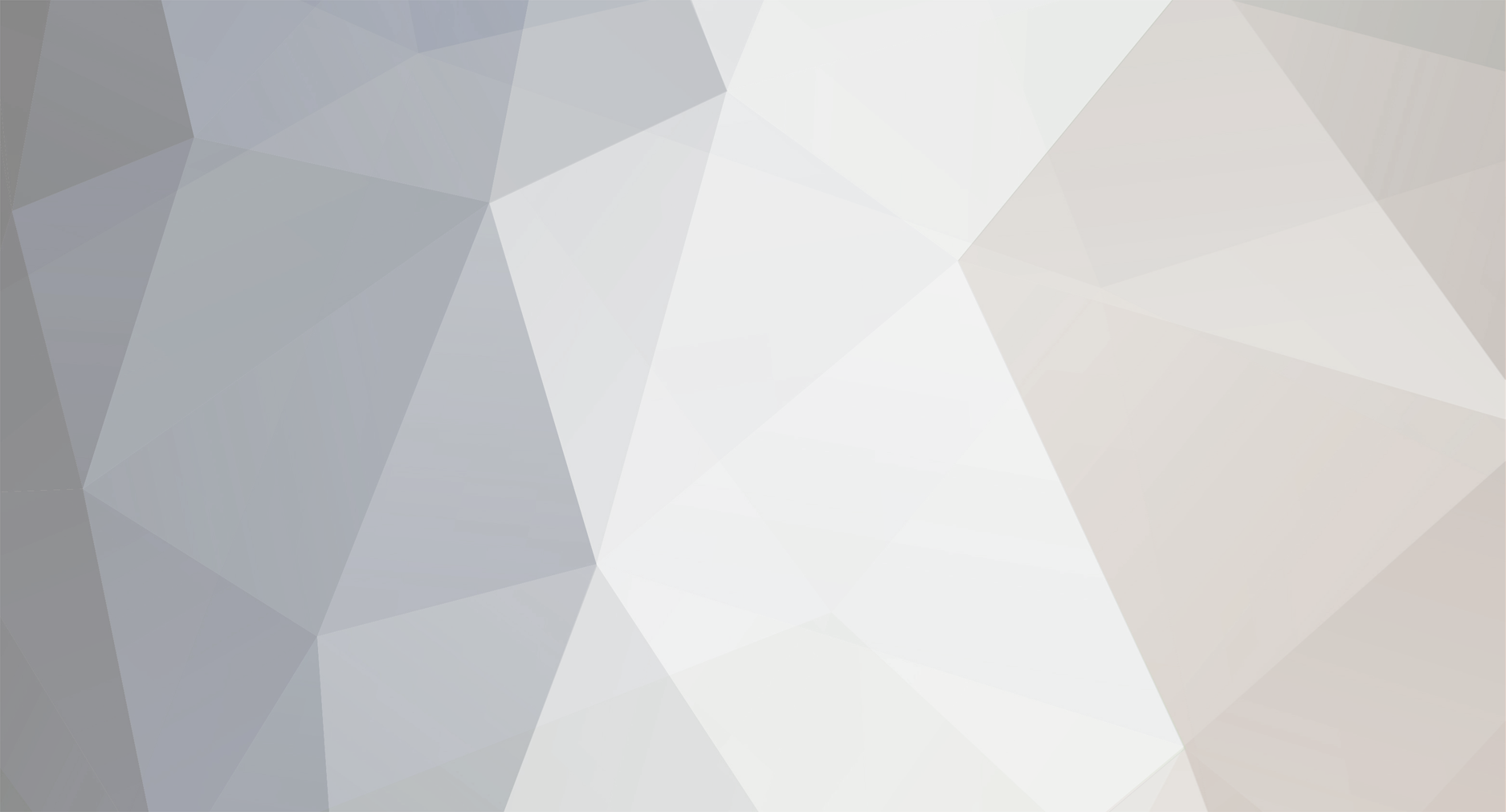
KevinM1
Moderators-
Posts
5,222 -
Joined
-
Last visited
-
Days Won
26
Everything posted by KevinM1
-
Are you certain that $_GET['categoryurl'] exists?
-
The newline character is a string literal, which means it must be placed in quotes. It's also \n, not /n.
-
You should always attempt to keep your components as separate as possible. There's actually a term for this - separation of concerns. Your PHP shouldn't be concerned with your JavaScript, and vice versa. The same goes for JavaScript and HTML - your HTML should be free from inline JavaScript (look up 'unobtrusive JavaScript'). It's impossible to keep things 100% separate as the various layers of your app have to communicate with one another (JavaScript may need to manipulate HTML elements, you may need to send some JavaScript data to your PHP script, etc.), but the goal is to do what you can to maximize separation and when you do need to have cross-layer communication, to only create the minimum necessary amount of clear, concise lanes of communication you need. There are many benefits to this. Your components are centralized, so if there's an issue, you don't need to dive through a bunch of unrelated code to fix it. If written well, you can swap out entire portions of your app for something else. It allows you to understand that, in essence, your HTML/CSS/JavaScript is really just a skin laying on top of the actual app. Trying to code with separation of concerns in mind from the beginning is a great habit to get into. It's how the pros do it, and if you start early with that mentality, you'll be ahead of the game.
-
PHP already is a template engine. Look at any decent MVC framework and how they handle their views. All Smarty provides is additional overhead and an extra language you have to learn. You're not gaining anything that you couldn't get with a well-planned PHP-only app. @btherl - It works because prompt() generates both the initial script, which it outputs directly, and the answer script, which is returned to the main code and then output immediately. In the browser, the output is simply: <script type='text/javascript'> var answer = prompt('Please enter your name'); </script>Hello there<script type='text/javascript'> document.write(answer); </script> @Zephni - no, that's actually a really, really bad way of doing it. Like bh said, it's ideal to keep the different components as separate as possible. Your prompt() function is badly thought out as it both echoes directly to the screen as a side effect and returns a value. And, really, why have the back end create AND process the front end code? JavaScript is designed to work on the front end. Keep it there. If you need data from JavaScript, there are better ways to obtain it.
-
What does this syntax mean in PHP?? Do you know ?
KevinM1 replied to aabid's topic in PHP Coding Help
In other words, objects can pass themselves as method parameters. Pretty wild, huh? -
$this->count doesn't exist. $count is local to the check() method. The 'this' keyword denotes a class member, which is either a pre-declared class field (like $dbconn was in my example), or a class method. Local variables (variables created within a method) don't count, and unless they're returned and stored in a variable outside of that method's scope, essentially disappear, which is what $count does. Before you go much further, you should brush up on the basics: http://php.net/manual/en/language.oop5.php
-
Do you understand GET, POST, and the differences between the two?
-
Yes.
-
Try: class DB_Handling { private $dbconn; public function __construct($dbname) { $conn = mysql_connect("localhost", "root", ""); if ($conn) { $this->dbconn = $conn; $recordset = mysql_select_db($dbname); if (!$recordset) { /* abort, error */ } } else { /* abort, error */ } } public function valueExists($value, $tablename) { $query = "SELECT * FROM $tablename WHERE username = $value"; $result = mysql_query($query, $this->dbconn); $count = mysql_num_rows($result); if ($count > 0) { return false; } else { return true; } } public function add($value, $tablename) { if (!$this->valueExists($value, $tablename)) { $query = "INSERT INTO $tablename VALUES ($value)"; $result = mysql_query($query, $this->dbconn); if ($result) { echo "Success!"; } else { /* abort, query error */ } } else { /* abort, value exists */ } } } $myDB = new DB_Handling("sample"); $myDB->add("Bubba", "testing"); NOT tested, so there may be some syntax errors, and you'll need to fill in the error handling.
-
You have a two dimensional array. You need to echo $count[i]['status'], where i is the index of the inner array you want.
-
In check, put: if ($count > 0) { return false; } else { return true; }
-
The 'undefined' means you have an error. Specifically, your JavaScript is most likely trying to manipulate a DOM object it cannot find/doesn't exist. It's a common error as JavaScript runs faster than HTML in the browser, so often scripts try to obtain references to HTML elements which haven't been rendered yet. The problem is trying to pinpoint the source of the error. Your best bet is to redesign your pages/site. Use unobtrusive JavaScript techniques (google it) and be sure to wait until the DOM loads fully before attempting to run any JS. Finally, develop using Firefox and the Firebug plugin. It's invaluable as a development tool, especially when it comes to debugging JS.
-
Is this all of it? As in, you don't have any code that defines a class?
-
Show me your actual code.
-
function add($value) { if (!check($value)) // when you translate this to a class, you'll have to use !$this->check($value) { // add the value } else { // abort, because the value already exists } } You may want to give check() a better name, like valueExists(), just so your code is more readable.
-
What results are you expecting to get?
-
Try: if (!check($value)) { add($value); }
-
Where is $carrier set?
-
You have a spelling error at the beginning of your PHP file. javascrypt should be javascript. Does the problem happen just after the OK button is pressed? After any button is pressed? Have you tried it with other browsers? Have you seen any JavaScript errors?
-
You should also check around locally and see if there are any small businesses that want a site. You may not make a lot of money at first, but at this stage of the game one's reputation tends to matter more.
-
In image1.php, you're attempting to echo the image data itself, but in image2.php you're trying to use that as the path of the image source. Raw image data is not the same as the image's path. You also have syntax errors in several places, including using the wrong value for your GET in image1.php. It should simply be $_GET['id']. That said, just so I'm completely clear, fixing those syntax errors won't fix your main problem. I'm not sure why you don't simply use a function to do what you want to do. It's simpler/cleaner than having a separate script. Try something like the following (all in one file - not tested): function displayImage($id){ $query = mysql_query("SELECT imgage FROM photos WHERE id = $id"); $row = mysql_fetch_array($query); $content = $row['image']; header('Content-type: image/jpg'); echo $content; } include("common.php"); error_reporting(E_ALL); $link = mysql_connect(host,username,password) or die("Could not connect: " . mysql_error()); mysql_select_db(db) or die(mysql_error()); $sql = "SELECT id FROM photos"; $result = mysql_query("$sql") or die("Invalid query: " . mysql_error()); ?> <table border="1"><tr><td>id</td><td>image</td></tr> <?php while($row=mysql_fetch_assoc($result)){ echo '<tr><td>'.$row['id'].'</td><td>'; displayImage($row['id']); } echo '</td></tr></table>'; At the very least, that's the approach you should be using.
-
A lot of tutorials, unfortunately, rely on 'global'. It's really the wrong way to go. Functions have argument/parameter lists for a reason. Pass everything your functions need to work through their lists. In any event, what do you mean by your form being filled in by a query? What's the process, step-by-step? And, when should your button(s) be enabled?
-
What's the code for DisableControls and SwitchDisabled? Also, never, ever, ever use 'global' in PHP. You're only hurting yourself when you do.
-
Figured this list should have the actual Mozilla Developer Center stuff: https://developer.mozilla.org/en/JavaScript