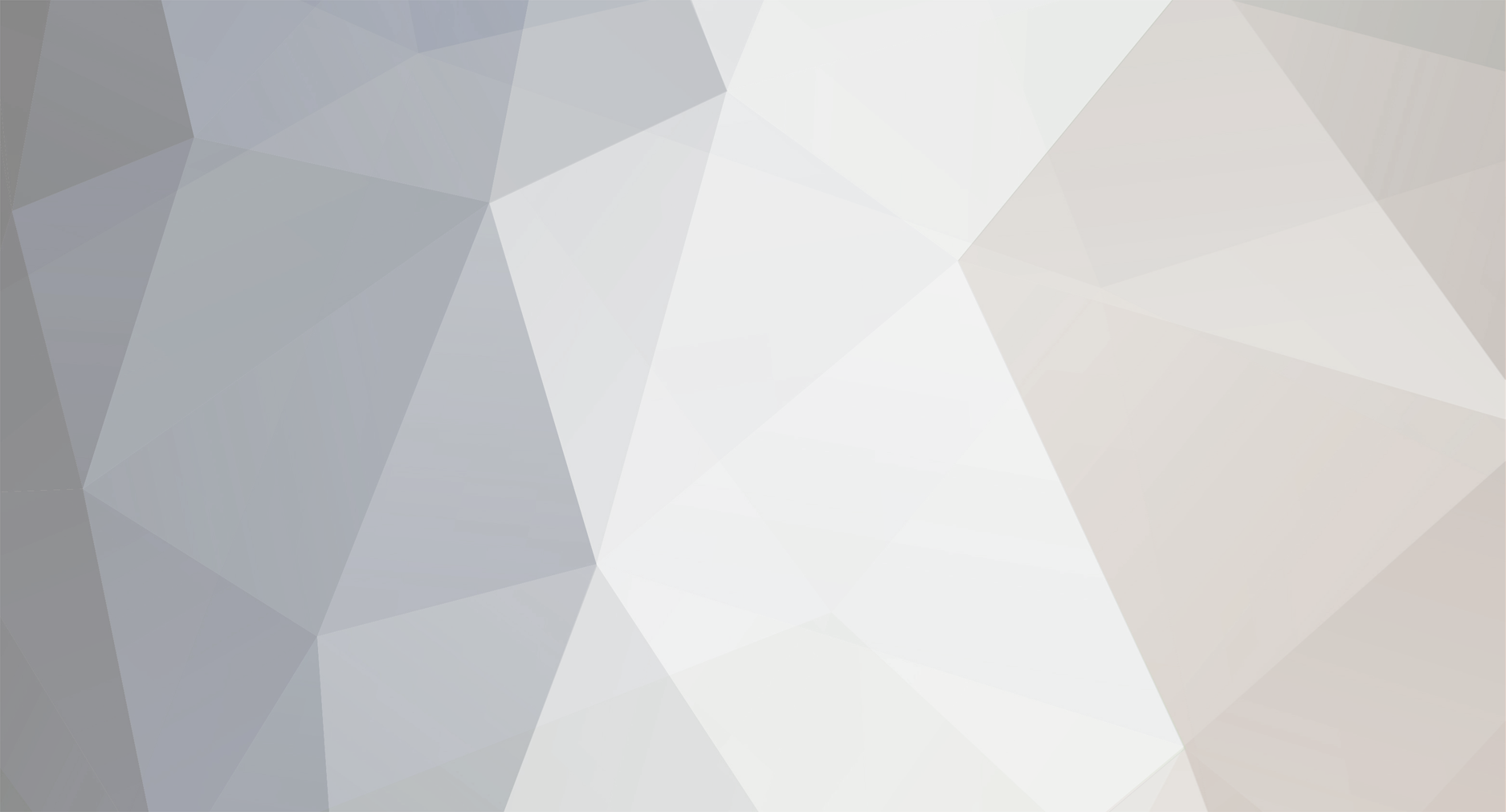
KevinM1
Moderators-
Posts
5,222 -
Joined
-
Last visited
-
Days Won
26
Everything posted by KevinM1
-
Extra credit way of getting it: $output = ''; $letters = array("A", "B", "C"); for($i = 1; $i <= 3; ++$i) { $output .= $letters[$i - 1]; } echo $output; The way that won't get you in trouble for cheating: $output = ''; for($i = 1; $i <= 3; ++$i) { if ($i == 1) { $output .= 'A'; } elseif ($i == 2) { $output .= 'B'; } else { $output .= 'C'; } } echo $output;
-
Yup, same with me. Happened again this morning after seemingly fixing itself for a couple days.
-
Don't send him to w3schools. See the following for why: http://w3fools.com/ When in doubt, go straight to the source. The PHP manual: http://www.php.net/manual/en/langref.php
-
Really take the time to read through the link I gave you. To use your bookstore example, ISBNs would not be listed as a comma separated string in a member's row. Instead, it would be part of a book's row. The link between book and member would come from a foreign key. The placement of that foreign key would depend on what kind of relationship should exist between the two. To use another example, blog posts and their comments represent a one-to-many relationship. One blog post can have many comments, but each comment is meant for one particular blog post. In this case, the primary key of the blog post would be the foreign key for each comment relating to it. So, you'd have something like: Table Comments comment_id blog_id comment_text 1 55 "Awesome!" 2 55 "I agree!" 3 55 "But what about Snoopy?" 4 90 "Thanks for the link." 5 02 "I really can't agree with this at all." 6 02 "Me neither." For many-to-many relationships, like I said before, a pivot table is used. This is simply a table that contains the primary key of each related item and maps them together. Think of video games and platforms - each platform (PlayStation, XBox, etc.) has a library of games, and a lot of games are available on multiple platforms (Grand Theft Auto is available on PlayStation, XBox, and PC). To relate them, you need a purpose-built table: Table Games_Platforms id game_id platform_id 1 04 55 2 04 77 3 04 94 4 10 77
-
The whole point of the link I gave you was to illustrate that you really don't want to have multiple entries per column. You need multiple database tables. Activities should have their own table. And if the relationship between them and members is many-to-many (activities can have multiple members, and members can take part in multiple activities), then you'll need another table (often called a pivot table) to map that relationship.
-
If $subject_data is defined before your loop, then you're overwriting it when you turn it into a blank array. In other words: $subject_data = array(); Is overwriting whatever you have. Also, where is $sql1 coming from?
-
http://dev.mysql.com/tech-resources/articles/intro-to-normalization.html
-
I don't mind the gray. What I don't like are: The blue blob/cloud behind your logo. It just seems haphazard, like you wanted something more distinct but couldn't figure out exactly what to do. The 2d/3d text on the menu. You should probably pick one style or the other. I'm also not sure if the 'naked' look works on the items on the end. Also, not sure if this is intentional, but the hard drop shadow on the edge is missing to the right of 'Contact' in its off state, but reappears on mouse over. Your margins are a bit jarring to me. The block in the middle has a clear margin to the left and right, but other elements (navigation, bottom block) stretch clear across the main block. Overall I like it, but there are some things that don't mesh.
-
You can't have any output written to the browser if you want to use a HTTP header command. Not even a space or new line. PHP apps tend to be written so all PHP processing is done before output is written. That way, you can determine if you need to redirect, or display something, and do either without interruption. Its a good habit to get into.
-
Is all the code in one file, or split up into multiple files? It's hard to tell since you didn't use code tags.
-
Like I said before, you NEED to use session_start at the top of each PHP file you want to access $_SESSION with. Otherwise, the session will not continue to exist in those files. It needs to be one of the very first lines of code you write. Not in a conditional, not in a loop, but right away in each file.
-
Show your code.
-
Just use a normal for-loop: $numConversations = count($conversations); for($i = 0; $i < $numConversations; ++$i) { if(($i + 1) == $numConversations) // adding 1 to the index because arrays are ZERO-indexed, so $conversations[0] is your first one { // add your CSS class } }
-
include('../Sub-Folder3/Sub3-IncludeFile1.php'); The '..' in the path means "Move up one level."
-
Are you using session_start at the beginning of each of your files?
-
The last two days have been fine for me. The previous few (4-5) were when the logouts were occurring. I haven't looked or touched my cookies ( ), so I'm not sure what caused the stability to return.
-
It's as simple as (pseudo-code): if (/* entered password is NOT correct */) { // display side bar } There's no else-clause because I'm assuming that the default state of the page wouldn't have the side bar. Now, you'll have to figure out how to check that the user is logged in, how to pass that info from page to page, and how to display the side bar itself.
-
Learning OOP - Need pointer in the right direction.
KevinM1 replied to iPixel's topic in PHP Coding Help
http://www.php.net/manual/en/language.oop5.static.php -
Learning OOP - Need pointer in the right direction.
KevinM1 replied to iPixel's topic in PHP Coding Help
I think you have the relationship backwards. Your authenticator should use/contain your db object, not the other way around. Why? Because a db object shouldn't care about authentication. It has a singular purpose - execute db queries and return their results and other metadata about the queries (like number of rows affected). The authenticator should handle the higher level stuff. I'd also make your authenticate method a pure boolean. Get rid of $ex/$ok - they're superfluous - and simply return true or false. Rename your method to isAuthenticated, and you can do something like: if($auth->isAuthenticated($user, $pass)) { echo "Welcome!"; } else { // handle it } Better yet, if you don't need the authenticator to maintain state, turn the function static and call it like: if(Authenticator::isAuthenticated($user, $pass)) { // etc. } For more of a general idea of how to determine good code, Google the following: Separation of Concerns Single Responsibility Principle DRY - Don't Repeat Yourself Tell, Don't Ask -
Defining a function is different than invoking a function. When you write: function myFunc($arg1) { // do something with $arg1 } You're not actually running (invoking) the function at that point. You're merely defining it. All you're really doing is telling the script "Here's a custom function I wrote." It makes sense to define functions outside of your script's execution flow in order to keep this distinction clear. Function definitions can still be placed in the same file as your script, but they don't belong in conditionals or loops.
-
Our first thread in this subforum deals with exactly this: http://www.phpfreaks.com/forums/php-coding-help/header-errors-read-here-before-posting-them/
-
$this is a PHP keyword, usable only inside of an object.
-
You should always use PHP regardless. Why? Because JavaScript can be turned off in the browser. That said, you can use JavaScript on top of PHP, as an extra layer to add more responsiveness for the user. The typical way to do it is to run JavaScript validation first. If things check out, then the PHP script will take a hold of the form data and do its own validation on it. If the JavaScript validation finds something wrong, it can alert the user immediately without sending the data on to the back end.
-
I haven't been able to stay logged into the site this week. I always have the 'forever' option highlighted, but I have to log in every morning. Just wondering if anyone else is having this issue or if I should clear my cookies.
-
Why not make a custom Exception object which inherits from Exception? Instead of storing a custom Exception within your static class (which isn't doing what you hope to do), simply throw your custom Exception when necessary and catch it like normal. In other words: Custom_Exception extends Exception { // custom properties and methods } class Autoloader { protected static $classes = array(); public static function load_library($class_name) { // ... try { self::load_class($class_name, $file); } catch(Custom_Exception $e) { echo $e->message; } } } This will allow you to catch various kinds of Exceptions, so if something doesn't fit your custom criteria, you could still handle more generic errors.