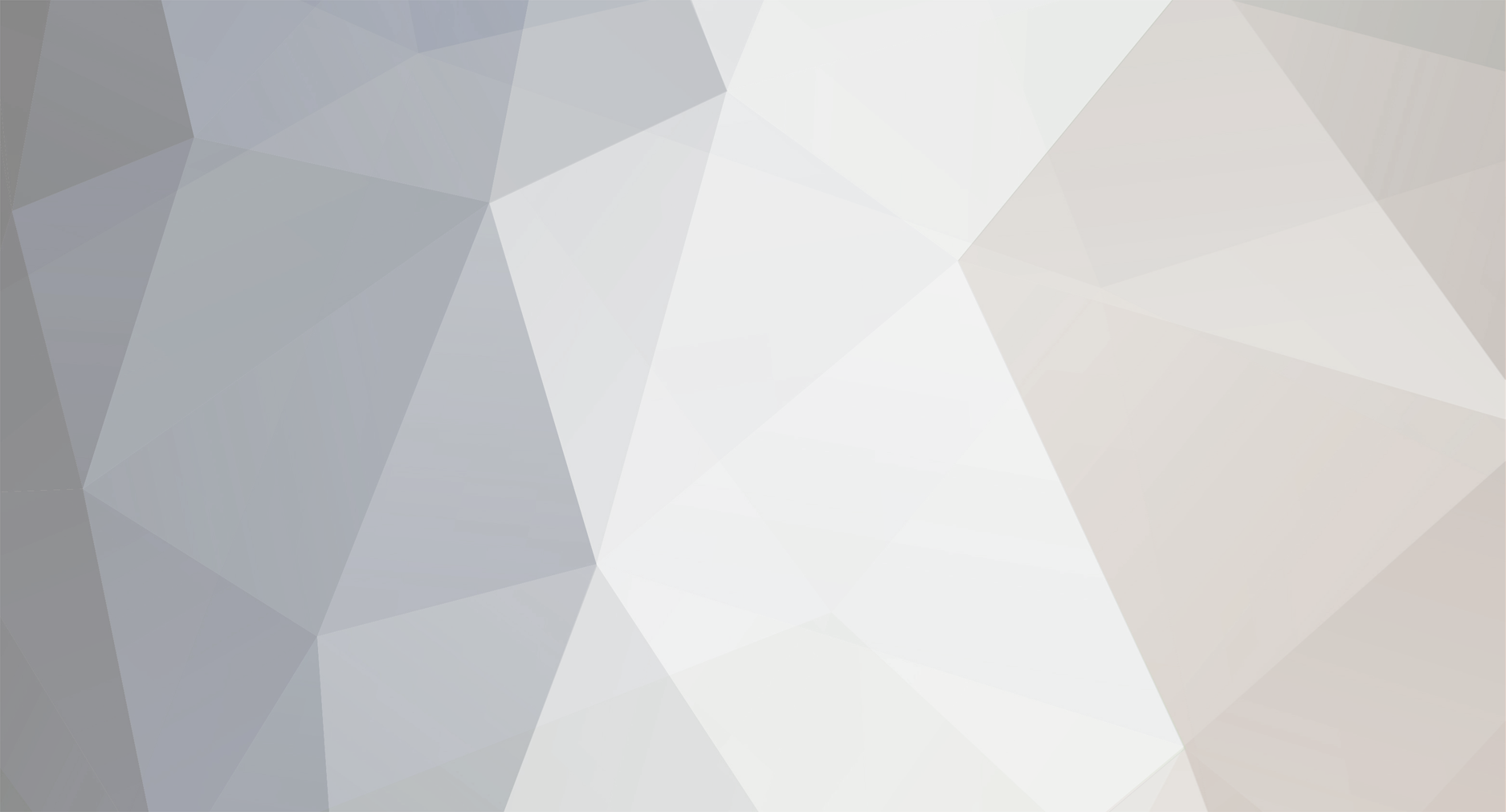
KevinM1
Moderators-
Posts
5,222 -
Joined
-
Last visited
-
Days Won
26
Everything posted by KevinM1
-
You can't echo in a function argument list. Echo literally means "echo this to the screen", so you can see how that won't make sense. If you need quotes around the variable, simply do: $uid = '"' . $uid . '"'; (that's a single quote, followed by a double quote, followed by another single quote) That said, are you sure you need the value of $uid to be wrapped in quotes?
-
Trying to do a 1:1 conversion from procedural to OOP won't work. The two methodologies just don't translate neatly into one another. Remember that objects can contain other objects. Instead of each class handling everything from instantiating a db, running queries on it, performing logic based on those queries, and destroying/closing/cleaning up the db afterward, you need to break it up and have at least one generalized db class that the others can reference, or contain a reference to. You should look into PDO, which is a generalized OO wrapper for a multitude of dbs (pdo, http://www.php.net/manual/en/intro.pdo.php) before wasting time reinventing the wheel. For the rest, go over the patterns again, especially the instantiation and structural patterns again.
-
help on designing a OOPs website, please look
KevinM1 replied to harrywolves's topic in Application Design
This is database interaction 101. INSERT, SELECT, and UPDATE queries. Why don't you look at your class wiki for inspiration? HTML and JavaScript here. Hint: your project should have the same back end regardless of whether it's being viewed on a mobile device or not. Applications are layered software. The part one sees and interacts with should be able to be changed without affecting the underlying system logic. Google RSS specs and look up SimpleXML. I'm sure you can find a relevant example in your class notes. Not to copy, as it says below, but to see how to do it in general. Again, see your class notes. If it's allowed, use jQuery to abstract away the nuts and bolts of Ajax so you can focus on the process not the minutia. Also, just so you know, asking for help on homework is technically against the rules here, and generally frowned upon. See: http://www.phpfreaks.com/page/rules-and-terms-of-service#toc_forum_do_nots -
You'll need to use an SqlDataReader: http://msdn.microsoft.com/en-us/library/system.data.sqlclient.sqldatareader.read.aspx Also, why VB.NET? C# is where it's at.
-
Regarding 'global', just because you can do something doesn't mean you should. 'Global' is frowned upon for the very reasons you're experiencing - it makes things incredibly difficult to debug because the value can be changed at any time. It also ties the proper execution of a function to the context in which it was invoked. Functions are supposed to be general purpose, where they take certain values from the outside, process them in a certain way, and return a result. 'Global' adds an extra, and hidden to the outside/main script, constraint. Good code adheres to explicit lines of communication. With functions, that means using their argument lists as intended and not adding extra, hidden caveats via 'global.' And, if you need to pass an unknown number of arguments into a function, use an array. Your algorithm is flawed because you're not taking into account HTTP's stateless nature. Values are not retained between page refreshes. Rather than stuffing results in a generic global variable, put them in sessions (also an array) to keep them between page refreshes.
-
$person = PersonFactory::findPerson($_SESSION['loggedin']); Is supposed to be used in your main script, or in which ever method needs to find a Person. It's a static method, so it's able to be invoked without needing an actual instantiated object. For resources, there are two: 1. http://www.amazon.com/Objects-Patterns-Practice-Experts-Source/dp/143022925X/ref=sr_1_1?ie=UTF8&qid=1296674773&sr=8-1 2. http://www.amazon.com/Design-Patterns-Elements-Reusable-Object-Oriented/dp/0201633612/ref=sr_1_1?s=books&ie=UTF8&qid=1296674826&sr=1-1 They're the two best. Read #1, then #2. Free tutorials tend to be shoddy, and many teach bad habits. You may as well pay some money to learn from the best sources possible if you want to do it right the first time.
-
1. The proper tags for PHP are <?php ?>. You're not guaranteed that the shorthand tags will work, so never use them. 2. Never use the 'global' keyword. Functions have an argument list for a reason. Pass all parameters necessary for a function to work through the argument list. 3. Your global is superfluous. You don't need it in your case at all. Simply use $searchInput. 4. Try basic debugging techniques. Write echo statements to track your variable through the form submission process.
-
Is it possible to make a $this var global for all classes?
KevinM1 replied to jamesxg1's topic in PHP Coding Help
Your example is hardly clear.... -
Notice: Undefined offset error - No idea why its happening...
KevinM1 replied to Russia's topic in PHP Coding Help
Why don't you have your icon data stored in another table? You have a one-to-many relationship there: one icon can have many related entities. Instead of manually creating the relationship with a PHP switch, put your icon info in a separate table containing an id and path to the icon itself, and run a JOIN to retrieve the aggregated info. That would save you a lot of work in your script, as you wouldn't have to build an entirely new array just to display the icons. So, you'd keep your current table, and create another for the icons where the 'icon' column of test_mysql is a foreign key containing the id/primary key value of the actual icon table. For more info, take a look at: http://dev.mysql.com/tech-resources/articles/intro-to-normalization.html -
Notice: Undefined offset error - No idea why its happening...
KevinM1 replied to Russia's topic in PHP Coding Help
I cleaned up your code a bit. You had some redundancy, and your update query needed tweaking (you were inadvertently overwriting values, and then trying to access scalar variables as array variables). I'm as baffled as you, to be honest. The only answer I can see is that you don't have an $id which equals 2. Double check your db to make sure you have a row whose id is 2. You could also do a simple test: $isIDGood = (isset($picture[2])) ? "yes" : "no"; echo 'Is $picture[2] valid? ' . $isIDGood; And, really, it's good practice to check to see if a particular array element is set before calling it directly anyway. --- Anyway, here's a slightly cleaned up version for you: <?php error_reporting(E_ALL); ini_set('display_errors', '1'); mysql_connect("localhost", "", "")or die("cannot connect"); mysql_select_db("test")or die("cannot select DB"); $tbl_name="test_mysql"; $sql="SELECT * FROM $tbl_name"; $result=mysql_query($sql); $count=mysql_num_rows($result); if (isset($_POST['Submit'])){ for($i=0;$i<$count;$i++){ $month[] = $_POST['month'][$i]; $date[] = $_POST['date'][$i]; $message[] = $_POST['message'][$i]; $title[] = $_POST['title'][$i]; $id[] = $_POST['id'][$i]; $icon[] = $_POST['icon'][$i]; $monthday = $month[$i] . "<br>" . $date[$i]; $sql1 = "UPDATE $tbl_name SET monthday='$monthday', month='{$month[$i]}', date='{$date[$i]}', message='" . mysql_real_escape_string($message[$i]) . "', title='" . mysql_real_escape_string($title[$i]) . "', icon='{$icon[$i]}' WHERE id='{$id[$i]}'"; if(!($result1 = mysql_query($sql1))){ "<BR>Error UPDATING $tbl_name "; exit(); } } } ?> <!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd"> <html xmlns="http://www.w3.org/1999/xhtml"> <head> <meta http-equiv="Content-Type" content="text/html; charset=utf-8" /> <title>Image Dropdown</title> <link rel="stylesheet" type="text/css" href="dd.css" /> <script type="text/javascript" src="jquery-1.3.2.min.js"></script> <script type="text/javascript" src="jquery.dd.js"></script> </head> <body> <form name="form1" method="post" action="updat5.php"> <table width="100%" border="0" cellspacing="1" cellpadding="0"> <tr> <td align="center"><strong>ID</strong></td> <td align="center"><strong>Month Date</strong></td> <td align="center"><strong>Message</strong></td> <td align="center"><strong>Title</strong></td> <td align="center"><strong>Icon</strong></td> </tr> <?php while($rows=mysql_fetch_array($result)){ ?> <tr> <td align="center"> <?php $id[]=$rows['id']; ?><?php echo $rows['id']; ?><input type="hidden" id="id" name="id[]" value="<?php echo $rows['id']; ?>"> </td> <td align="center"> <input style="border: 1px solid #C3C3C3;height: 20px;" name="month[]" MAXLENGTH="3" size="3" type="text" id="month" value="<?php echo $rows['month']; ?>"> <input style="border: 1px solid #C3C3C3;height: 20px;" name="date[]" MAXLENGTH="2" size="2" type="text" id="date" value="<?php echo $rows['date']; ?>"> </td> <td align="center"> <input style="border: 1px solid #C3C3C3;height: 20px;" name="message[]" size="70" type="text" id="message" value="<?php echo $rows['message']; ?>"> </td> <td align="center"> <input style="border: 1px solid #C3C3C3;height: 20px;" name="title[]" size="70" type="text" id="title" value="<?php echo $rows['title']; ?>"> </td> <td align="center"> <select name="icon[]" style="width:200px" class="mydds"> <option value="1"<?php if ($rows['icon'] == 1) { echo 'selected="selected"'; } ?> title="icon/icon_phone.gif">Phone</option> <option value="2"<?php if ($rows['icon'] == 2) { echo 'selected="selected"'; } ?> title="icon/icon_sales.gif">Graph</option> <option value="3"<?php if ($rows['icon'] == 3) { echo 'selected="selected"'; } ?> title="icon/icon_faq.gif">Faq</option> </select> </td> </tr> <?php } ?> <tr> <td colspan="4" align="center"> <br><input type="submit" name="Submit" value="Submit"> </td> </tr> </table> </form> <hr> <?php $picture = array(); while($row = mysql_fetch_assoc($result)) { $id = $row['id']; switch ($row['icon']) { case 1: $picture[$id] = '<img src="img/apple.gif" title="apple" alt="apple" />'; echo $picture[$id]; break; case 2: $picture[$id] = '<img src="img/banana.gif" title="banana" alt="banana" />'; echo $picture[$id]; break; case 3: $picture[$id] = '<img src="img/orange.gif" title="orange" alt="orange" />'; echo $picture[$id]; break; default: $picture[$id] = ''; echo $row['icon'] . " is something other than 1 2 or 3"; break; } } ?> <?php echo $picture[2]; ?> <script language="javascript" type="text/javascript"> function showvalue(arg) { alert(arg); //arg.visible(false); } $(document).ready(function() { try { oHandler = $(".mydds").msDropDown().data("dd"); oHandler.visible(true); //alert($.msDropDown.version); //$.msDropDown.create("body select"); $("#ver").html($.msDropDown.version); } catch(e) { alert("Error: "+e.message); } }) </script> </body> </html> -
Notice: Undefined offset error - No idea why its happening...
KevinM1 replied to Russia's topic in PHP Coding Help
Do you have a link I could visit? I'd like to see the rendered HTML. Also, which code did you move, and to where? I can't tell if you're talking about the JavaScript, some of the PHP, or both. -
Notice: Undefined offset error - No idea why its happening...
KevinM1 replied to Russia's topic in PHP Coding Help
Can you show more code? It's hard to diagnose without seeing how things are called. -
Notice: Undefined offset error - No idea why its happening...
KevinM1 replied to Russia's topic in PHP Coding Help
Actually, I was wrong originally. Arrays in other languages act differently than PHP arrays. Still, the point remains, are you sure you have an $id of 2? -
Notice: Undefined offset error - No idea why its happening...
KevinM1 replied to Russia's topic in PHP Coding Help
The error is happening because you're attempting to access an array element that doesn't exist. Your array has one item, indexed by whatever value $id contains. $picture[2] is attempting to access the third (because arrays are zero-indexed) item in the array, which, again, doesn't exist. Hence an undefined offset. There's a simple fix: $picture['2'] -
Knowing OOP syntax is only half the battle. You need to understand the philosophy behind OOP to figure out how to structure things so they work properly. One of the main tenets of OOP is encapsulation - that objects are self-contained entities which have the potential to work in different contexts. Having an object look for a particular session variable breaks encapsulation because you're tying (coupling) the object to a particular system. It won't be able to work unless that session variable exists. Logically, your object shouldn't really care where its data comes from. So long as it has legit data, it should be able to function correctly. Use the constructor's argument list to pass in the correct values: class Person { private $id; private $name; public function __construct($id, $name = null) { $this->id = $id; $this->name = $name; } public function getName() { return $this->name; } } Now, what about constructing a person? I like to use a factory for something like this, as, really, a Person should represent a single person's data and the actions which can be performed on that data. Finding and constructing a new Person seems like a separate responsibility to me. So, take a look at this bare-bones Factory class: class PersonFactory { // db config info/settings/variables public static function findPerson($id) { $query = mysql_query("SELECT name FROM users WHERE id = $id"); $result = mysql_fetch_assoc($query); $person = new Person($id, $result['name']); return $person; } } Now, it's as simple as: session_start(); $person = PersonFactory::findPerson($_SESSION['loggedin']); You've now decoupled the Person from both sessions and the db. It can act, and be acted upon, in an individual and free manner. Keep in mind, this is a very rough sketch of how I would approach your problem. There's no error checking, autoloading, etc. Don't expect to be able to simply copy and paste this and have it work completely.
-
You can't use $id if it's undefined. Look at where you attempt to use it in comparison to where you assign a value to it.
-
Take a look at urlencode and urldecode, or htmlentities
-
Not having them can break encapsulation. Objects should be interacted with through clear lines of communication. Having an accessor method ensures that the entity interacting with them is, in fact, intending to interact with them and is doing so in a certain, explicit manner. Like someone else said in the thread, they're not for you but for others who will be working with your code.
-
Chances are, the value was generated by a hash algorithm. Hashes are one-way only encryption, meaning they can't be decrypted (it's why they're typically used for password protection). So, you're likely out of luck.
-
You're mixing single and double quotes on almost every line... that will cause problems.
-
Let's say you have something like: function makeForm() { $form = '<form action="" method="post"><input type="text" name="data" /><input type="submit" name="submit" value="Submit" />'; echo $form; } $numbers = (1, 4, 90, 33, 234); makeForm(); foreach($numbers as $num) { echo $num; } The form will be displayed, as well as the numbers in the $numbers array. However, if you try to do something like: echo $_POST['data']; It will fail unless you check to see if the form has been submitted. That's because although you've outputted a form, an incoming request with the form data hasn't yet been made, and you're trying to obtain a value from a variable that doesn't exist.
-
Not necessarily. Again, the script only runs until it runs out of code to execute (end of file). If you output your form at the end of the script, NOTHING will be running. Moreover, again, HTTP is stateless. Even if you have more code in your script, it can't obtain values entered into the form automatically. It takes a form submission, which is an HTTP request, which necessitates a new copy of the page being served. What are you trying to do? And, more importantly, what results are you expecting? I'm concerned that you're not grasping the fundamentals of what's really going on because the word 'running' is a loaded term.
-
do_it will output the form and the script will continue until the end of file. The form itself is merely rendered in the browser. Once it's displayed on the screen, it's done - there's nothing running in the background waiting for user interaction. When someone submits a form, a HTTP request is made to the server. The server reads the action URL and serves up the correct script based on that value. In all cases, it's a new copy of the script. Put another way, it looks like you're expecting do_it to be able to read user submitted form values and use them to continue processing. Neither PHP nor HTTP works that way. There's no persistent MyScript.php process running in the background.
-
Could someone maybe explain what happends here
KevinM1 replied to fortnox007's topic in Javascript Help
For the '\b', take a look here: http://www.regular-expressions.info/wordboundaries.html The mouseout function is removing the sfhover class from the elements that don't need them. For the last bit, it's simply attaching the sfHover function to the window's onload event. When the window is loaded, the hover functions will be loaded and ready to work. -
Or, the form is a sticky form, which uses the values sent via a HTTP request (get or post) to refill the form fields.