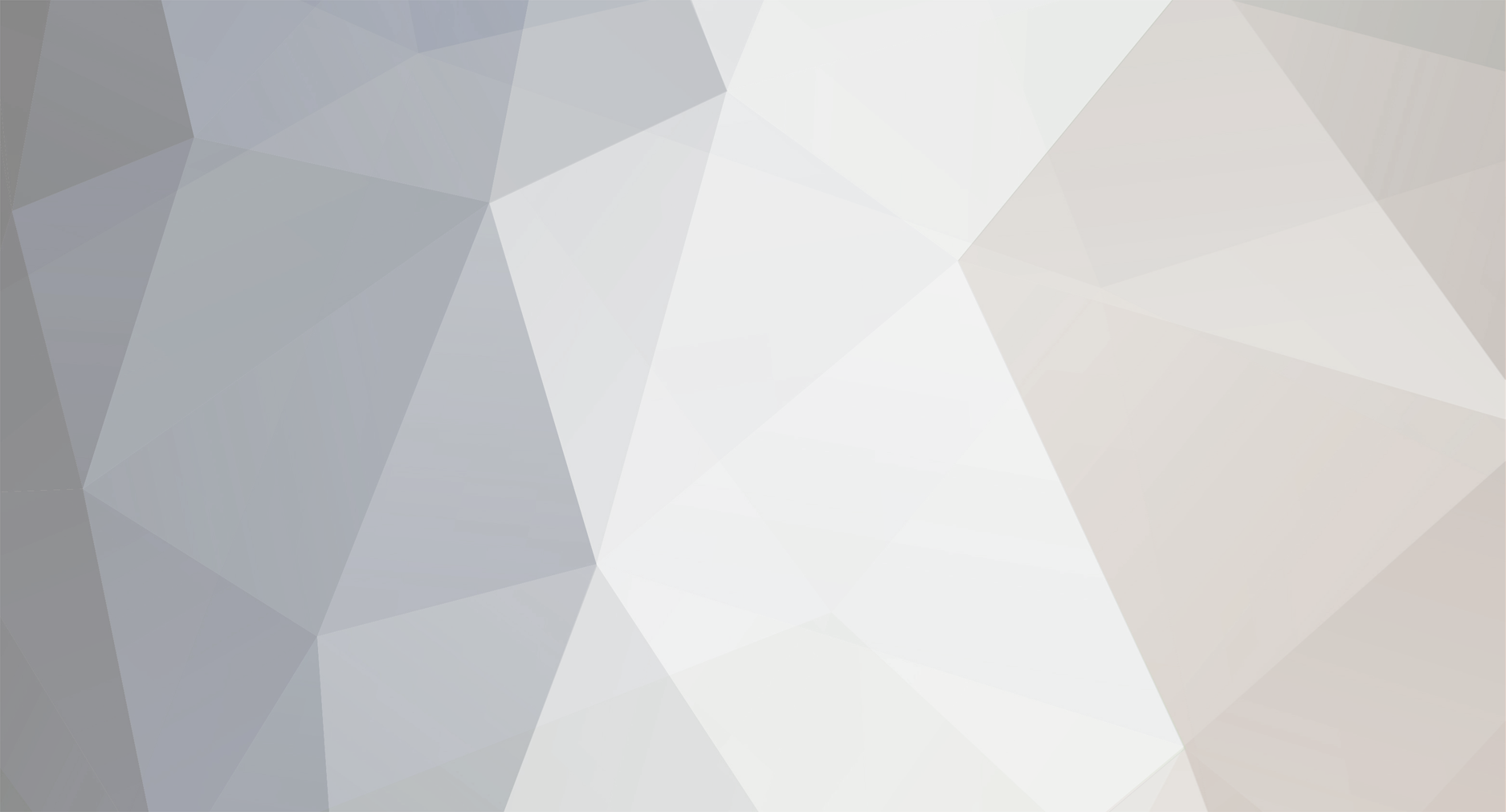
KevinM1
Moderators-
Posts
5,222 -
Joined
-
Last visited
-
Days Won
26
Everything posted by KevinM1
-
Generally, most people try to keep their controllers thin. The controller is the crossing guard - it should pass data along to where it should go (the model, the view), but it shouldn't be responsible for actually manipulating it beyond that. Even with ORMs, many people create Repository objects to act as a facade in order to keep the model details hidden from the controller.
-
Really? The previews looked horrible. I saw it a few weeks ago. Aside from a few action parts, I didn't really care for it. The beginning dragged, and the ending, in particular, ended on an odd tone. And no, I'm not referring to the violence against a particular character.
-
How much of a performance impact would it be if PHP used an overload of + as its string concatenation operator?
-
Can you fix your formatting? Some of your indents are out of control. Also, consolidate your code. For example, you can manipulate the form data in a mere two lines. $username = mysql_real_escape_string(stripslashes($_POST['username'])); Do the same for friend. You already obtain the number of db rows and assign it to $rows. You don't need to calculate it again. Finally, your second if-conditional has a redundancy in it. Remember, equality goes both ways. You don't need an 'or' there. Only write what you need to get something to work, don't repeat code, and trim the unneeded. It'll make it easier for anyone trying to help you to get to the heart of what you're trying to do. Good code is terse and readable.
-
It works though. True. I just greatly prefer the dot syntax used by the other big players.
-
...and the syntax is ugly as sin.
-
What part of programming challenges you the most?
KevinM1 replied to Anti-Moronic's topic in Miscellaneous
Hardest thing about coding for me is dealing with other web devs. Best case scenario is they are good but have their own style of doing things..which is mostly okay, as long as they know wtf they are doing. Worst case scenario (which I should really call average case scenario) is they have no business being a web developer in the first place...client too stingy to put real money into that sort of resource so I'm stuck dealing with c/p cowboys. You know what, I never used to have a problem with this but it is a pet peeve now. People taking on PHP who don't even like programming or have an interest in developing themselves as decent web developers. You can see it sometimes in the other help forums. Asking questions which they should already know because they've taken on work they can't even understand or want to understand. ugh..I feel dirty. Well, sometimes it isn't their fault. It's not uncommon for a small time graphic designer, or, hell, even an administrative assistant to be forced to dabble in PHP because their boss wants a small tweak done to their site. We actually had a few posts like that here a couple months ago. You can't always tell your boss 'no'. -
1. You can't mix and match MySQLi and MySQL. Use one or the other. 2. Why use a hidden input when you can simply check $_POST if the submit input exists in the array? 3. Validate and escape incoming data. 4. Don't use die as an error handler. At the very least, use trigger_error instead. 5. The error message itself is useless for the end user.
-
Comment protection against sql injection help need to be secure!
KevinM1 replied to Minimeallolla's topic in PHP Coding Help
Where does $username come from? Echoing row data isn't the same as constructing a variable. Also, your assignment statement dealing with the comments is backwards. The escape function will protect you from injection, but you should validate all incoming data. When you display your comments, you'll need to combat against unwanted HTML and XSS attacks. -
Yes. An underscore cannot be the first character, but can be anywhere else in the name.
-
Comment protection against sql injection help need to be secure!
KevinM1 replied to Minimeallolla's topic in PHP Coding Help
Don't use addslashes. And what is real_escape_string supposed to be? Use mysql_real_escape_string on string data you want to insert into the db, or better yet, use MySQLi and parameterized statements. -
What part of programming challenges you the most?
KevinM1 replied to Anti-Moronic's topic in Miscellaneous
As a freelancer, I hate dealing with clients. Living in rural New England, most other small business owners are a frustrating mix of cheap, ignorant, and stubborn. Not fun to deal with at all. I'm actually happy I've found some success as a subcontractor. Doing work for people who at least have some idea of various parts of development is much more rewarding, especially if they've dabbled in PHP before. They know enough to not want to touch what you write. -
Just to add to this, the most popular convention is for public controller methods to map to view scripts of the same name. So, a method named index would use a view script named index,php. A method named showCart would use a view script named showCart.php. And so on. CodeIgniter, Kohana, I believe Zend Framework, Ruby on Rails, and ASP.NET MVC all follow this convention. For clarity, let's say you had a couple of controllers: class HomeController extends Controller { public function index() { // get data from model and render } } public class CartController extends Controller { public function index() { // obtain data from model and render } } Two controllers, each with a method named index. Surely they can't both use the same view script. This is where the naming convention pays off. Look at the class names. They follow a convention as well. SomethingController. The something is the important part. It informs the framework where to look for a particular controller's view scripts. So, the path to the first view script would be path/to/Views/Home/index.php. Similarly, the second would be path/to/Views/Cart/index.php. You'll notice that the path supplied to Thorpe's explicit invocation of render is essentially the same. The exact path name convention is dependent on the framework - I've been doing a lot of work in ASP.NET MVC lately which is why mine's a hair different than his. The overall point remains, however.
-
can someone tell me why my php code wont process
KevinM1 replied to racercam's topic in PHP Coding Help
A more readable way of doing it would be to place the array variables within brackets inside the string. e.g.: $message = "Full Name: {$_POST['NAME']}\n Address: {$_POST['ADDRESS']}\n Town: {$_POST['TOWN']}\n Post Code: {$_POST['POSTCODE']}\n Phone Number: {$_POST['PHONE']}\n Email: {$_POST['email']}\n\n"; It keeps things in a format most expect, and eliminates little squabbles like this one. -
I think you're both over thinking it: <?php $n = array('81.0', '80.5', '70.5', '70', '71.569', '67.0', '65.5', '90'); foreach($n as $v) { $fv = (float)$v; // cast them as floats $iv = (int)$v; // also cast them as ints $decimal = ($iv - $fv); // obtain the decimal portion by finding the difference if($decimal < 0) // the difference is the fractional part, always 0 or lower { echo number_format($fv, 1) . "<br />"; } else { echo $iv . "<br />"; } } ?>
-
Even better if the methods are static, as you probably wouldn't have to worry about maintaining state in most cases.
-
Imagine if the currency code was used quite a few times. Putting it in say 4 classes would be duplicating. If I put it in one standalone function then these classes can use it as they need (can't they?) Surely there must be a need for a standalone function on some occasions - even in full OOP designs? Why would you need to put currency code in 4 classes to begin with? Properly designed code (forget about OOP for a moment) follows the DRY principle - Don't Repeat Yourself.
-
Then you don't really understand OOP. The currency of OOP is objects, which are instances of a class. You're supposed to have a lot of objects. Well, like you say, your hypothetical currency code will be part of a larger section.... Using classes to simply group together similar methods is NOT OOP. All you're doing is creating custom data types and using them procedurally. It's not much different than having library files filled with functions. The bulk of OOP is objects communicating with each other, containing references to one another, and using each other to do work. Yes. You should look at design patterns to get a good idea of how OOP differs from procedural programming. Wrapping things in a class and calling it a day isn't legit. Don't worry about optimization at the beginning. Create your system first, test it, then trim where necessary.
-
Or use a well established framework. That's a difference, .Net is a framework not a language itself. Exactly. When people say .NET, they generally mean ASP.NET with either VB (yuck) or C# (yay) as the actual programming language. For the web itself it's usually ASP.NET MVC 2 with C# and Entity Framework 4.
-
Even if you freelance there's a good chance you'll be working with existing code at some point. Also, if a particular area leans towards one or the other, your clients will expect a certain price point and interoperability with other existing systems. Finally, depending where you live, it may not be feasible for you to live solely on freelance work. For a lot of devs freelancing is a means of generating extra income, not a career in and of itself. If you're truly aiming to be self-sufficient, you'll need to understand your local economic environment.
-
There's no set answer. What is more popular in your area? PHP generally has a much easier learning curve, but that doesn't matter much if there aren't any PHP jobs in your area.
-
ASP.NET isn't more secure than PHP. Web security is 9/10ths how a site is written, not which language was used to write it. Also, PHP is free and ubiquitous - it's not going anywhere. The smart thing to do is learn both. That way you can earn a living regardless of the server side technologies used.
-
The best free reference is the PHP site itself, especially its function reference: http://www.php.net/quickref.php
-
What up, dawg?
-
If you're going to go Dell, I suggest their Studio line. Their Inspirons always felt a bit cheap to me.