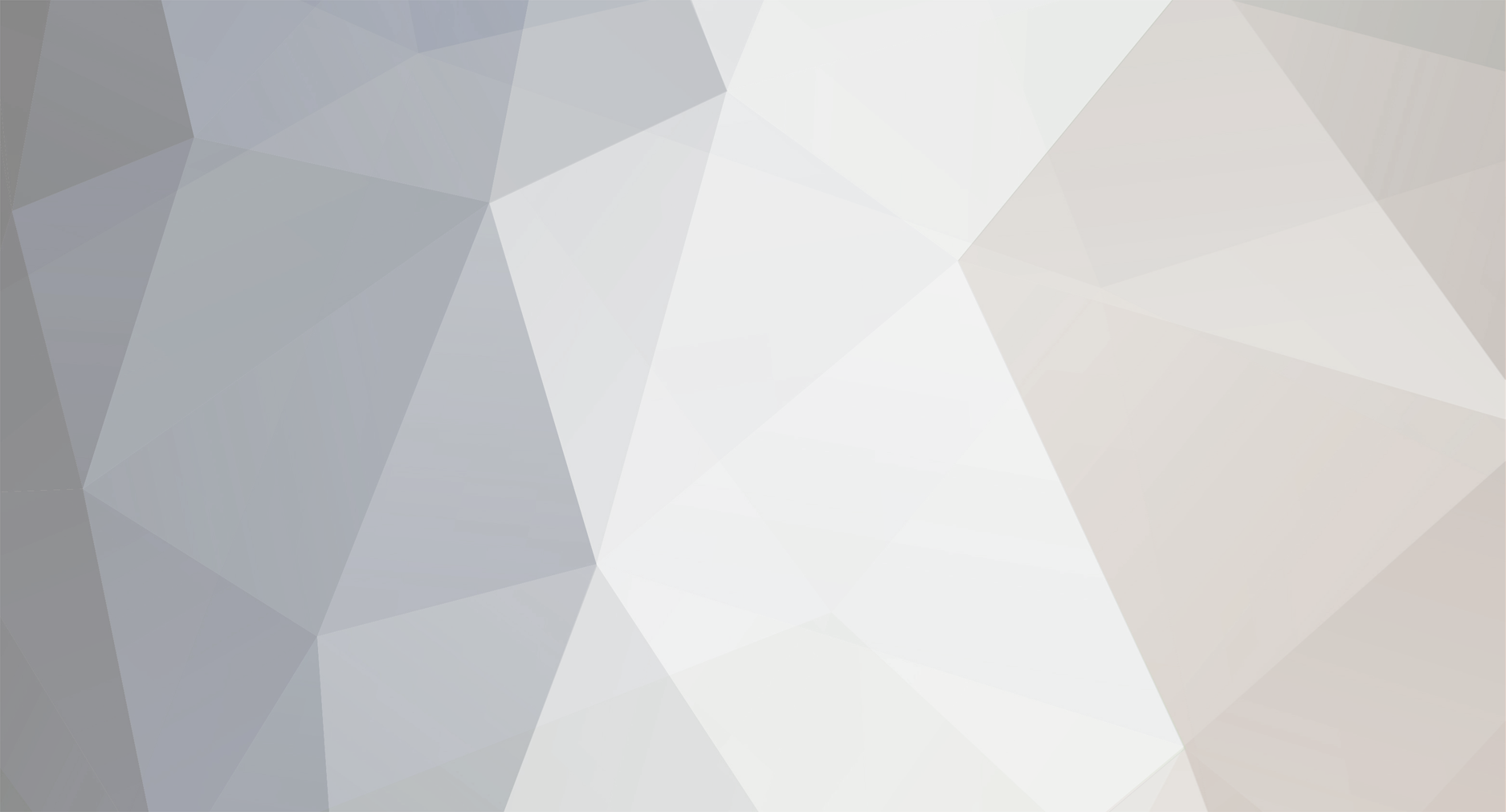
RichardRotterdam
Members-
Posts
1,509 -
Joined
-
Last visited
Everything posted by RichardRotterdam
-
Let me explain why this error occurs. In the following code you check the variable $scale when it doesn't exist yet. <?php if ($scale == "celcius") ?> What do you mean by check submit? You are using a form. You need to check if a form was submitted before you can use the values that were send by the form. This is because there are no form values to begin with. You can check if a form was send like so: <?php // using the post method if(isset($_POST['submit'])){ // your form submit code here } // using the get method if(isset($_GET['submit'])){ // your form submit code here }
-
Then the previous code should work I guess haven't tested it so it might produce errors <?php if(isset($_POST['save'])) { include 'config.php'; include 'opendb.php'; $query = stripslashes ($_POST['run']); mysql_query($query) or die(mysql_error()); } ?> <html> <head> <title></title> </head> <body> <form method="post"> <table width="80%" border="0" align="center"> <tr> <td width="100">Query</td> <td><textarea name="run" cols="50" rows="10"></textarea></td> </tr> <tr> <td width="100"> </td> <td> </td> </tr> <tr> <td colspan="2" align="center"><input name="save" type="submit" value="Run query"></td> </tr> </table> </form> </body> </html>
-
Do you mean you just want to enter that query and it should be executed? It is indeed a query that will create a new table
-
Ugh re-reading the comments you made I think I just missunderstood. I'm grabbing another coffee. You're simply trying to execute sql commands using a form. Similar to running a query on a command line. Just don't use it on any public server that would be just too risky <?php if(isset($_POST['save'])) { include 'config.php'; include 'opendb.php'; $query = stripslashess ($_POST['run']); mysql_query($query) or die(mysql_error()); } ?> edit Hmmm or did you mean you want to fill in a table name to create a new table? Now I am not sure what you mean could you elaborate a little more?
-
I was more thinking of something like this <?php if(isset($_POST['save'])) { include 'config.php'; // probably your db connect vars such as host dbname user and password include 'opendb.php'; // Don't know what's in here is this your database connection script? $run = mysql_real_escape_string ($_POST['run']); // sql injection prevention $query =" INSERT INTO TABLE survivalist ( run ) VALUES( '{$run}', )"; mysql_query($query) or die(mysql_error()); } ?> <html> <head> <title></title> </head> <body> <form method="post"> <table width="80%" border="0" align="center"> <tr> <td width="100">Query</td> <td><textarea name="run" cols="50" rows="10"></textarea></td> </tr> <tr> <td width="100"> </td> <td> </td> </tr> <tr> <td colspan="2" align="center"><input name="save" type="submit" value="Run query"></td> </tr> </table> </form> </body> </html>
-
To be honest I just think that script is plain wrong. the following just should not be in there $query = 'CREATE DATABASE survivalist'; That's why I mentioned the basic SQL commands. You need a insert query such as <?php $sql=" INSERT INTO TABLE table_name ( val1, val2, val3, etc ) VALUES( '{$val1}', '{$val2}', '{$val3}', '{$etc}', )"; mysql_query($sql) or die(mysql_error());
-
It seems like you are creating a database each time you want to insert something new. This is not how a webapp works. You need to create a database before you insert something. When you have created a database you need a table inside your database. Tables inside a database are used to store data. You can use PHP to manipulate data in your database. I suggest you look into the following basic SQL commands: select insert update delete
-
Yeah I hear you on that. Also manipulating the DOM with just vanilla javascript is just a whole lot less fun in comparison with using a JS framework for that task. He meant wrap your code between [ code ] [ /code ] (without the spaces). It will highlight php code and put it inside a codebox. Whoaah!!! hang on right there buddy you lost me there. I thought you meant dynamically add form fields using javascript but you meant adding fields with PHP I think. Could you post a sample of the HTML that is generated by the php with the multiple forms? Maybe that way a part of your interface is visible which makes it more clear what you are trying to accomplish.
-
Can you show the code that generates these multiple fields? Also I agree with roopur18 that using a js framework for this would recommended. Just not necessarily dojo. Dojo, jQuery, mootools, prototype YUI are all fine frameworks.
-
I indendented your create query for better readability CREATE TABLE `bob`.`address` ( `Address_ID` VARCHAR(12) NOT NULL, `Address_Line1` VARCHAR(45), `Address_Line2` VARCHAR(45), `Address_Line3` VARCHAR(45), `ZipPostalCode` VARCHAR(10) NOT NULL, `City` VARCHAR(45) NOT NULL, `State` VARCHAR(45), `Country` VARCHAR(45) NOT NULL, PRIMARY KEY (`Address_ID`), CONSTRAINT `FK_Address_1` FOREIGN KEY `FK_Address_1` REFERENCES `country` (`Country`) ON DELETE RESTRICT ON UPDATE RESTRICT ) ENGINE = InnoDB; Ok here's my critique on it: `Address_ID` VARCHAR(12) NOT NULL Why is your adress_id of a varchar type? Is there a reason for this? CONSTRAINT `FK_Address_1` FOREIGN KEY `FK_Address_1` REFERENCES `country` (`Country`) You could use the CONSTRAINT `FK_Address_1` but you don't really need it. It's just a name added to your constraint. FOREIGN KEY `FK_Address_1` Here you are trying to create a foreign key on the FK_adress_1 field, howerver you dont have a field named `FK_Address_1`. Also it's common for the foreign key type to be an int is there a reason your using varchar here? REFERENCES `country` (`Country`) Here you are refering to the field named `Country` within table named `country` I recommed refereing to an id field such as country_id Here is how I would do it CREATE TABLE `bob`.`addresses` ( `Address_id` INT(12) NOT NULL AUTO_INCREMENT, `country_id` INT(12) NOT NULL, `Address_Line1` VARCHAR(45), `Address_Line2` VARCHAR(45), `Address_Line3` VARCHAR(45), `ZipPostalCode` VARCHAR(10) NOT NULL, `City` VARCHAR(45) NOT NULL, `State` VARCHAR(45), PRIMARY KEY (`Address_ID`), FOREIGN KEY (`country_id`) REFERENCES `countries`(`country_id`) ON DELETE RESTRICT ON UPDATE RESTRICT ) ENGINE = InnoDB; edit You might also want to set up a foreign key for the states and the cities.
-
Me -> new_to_oop(); Can't declare new variable in a method?
RichardRotterdam replied to br3nn4n's topic in PHP Coding Help
Anyone correct me if i am wrong. I think it's a style preference to make the difference more clear between public variables and protected + private vars. You don't have to use the underscores it will work fine without it. -
dynamic menu data from mysql with php
RichardRotterdam replied to nehapuniani's topic in PHP Coding Help
It is not the reason why I say you are better of using a different table for categories. You might want to change a category name in the future. With the current database design you would have to manually rename all of the category names instead of doing it only once. Besides that you might want to fit a category in a category as in use subcategories. What code do you have so far for selecting the results from the database? Are you using PHP? -
[SOLVED] function with implode() won't work
RichardRotterdam replied to Miko's topic in PHP Coding Help
Looking at the bigger picture it seems like you are trying to fetch the id's of the categories where it matches an array of names. I think your implode isn't the issue here I think your query is. You could write it in one query instead of a couple of queries in a loop. <?php /** * get a string with id's seperated by commas * @params array names * @return string */ function implodeId($names){ if(is_array($names)){ $sql = "SELECT * FROM categories "; $ids = array(); for($i=0; $i < sizeof($names); $i++){ if($i==0){ $sql .= "WHERE name = '{$names[$i]}' "; }else{ $sql .= "OR name = '{$names[$i]}' "; } } $result = mysql_query($sql); while($row = mysql_fetch_array( $result )) { $ids[]= $row['id']; } return implode(",",$ids); } } ?> -
You can do that with a query what do you have so far? You will need a cron job for this or scheduled task what ever you call it on windows
-
I know the feeling I totally hate presentations the larger the audience the more nervous I get. It does depends a little who the audience is though. It used to be the same with performing in a band or on piano for me, but that's a little different since it's a bit like being in your own world where there is just an instrument and yourself at least for me.
-
dynamic menu data from mysql with php
RichardRotterdam replied to nehapuniani's topic in PHP Coding Help
I wasn't able to run the query the quotes needed to be removed. I indented the create query so it's more readable and you might have a better response in the future create table Recipe ( Auto int(10) default 0, Category VARCHAR(255) default null, Name VARCHAR (255) default null, Recipe Text ) ENGINE = MYISAM; I have a bit of critique here on your table. 1. Why is your first field named auto??? shouldn't this be id or something similar. 2. You have category as text i'd create a different table for categories and set a relation with a foreign key 3. You have a field recipe in your table recipe. Maybe you should just name this field description so it's less confusing. -
Java - How to make a window open?
RichardRotterdam replied to plznty's topic in Other Programming Languages
function myPopup() { for(var i=0; i<1000; i++){ alert("you must click this button a 1000 times before seeing a smashing popup!"); } window.open( "http://www.google.com/" ); } I made some improvement to the script now it should work. Hmm then again that's not java. Java should be like class Popup { public static void main(String args[]) { // your window code here } } -
What does this script exactly do? Somehow I get the impression that this is a low level process.
-
Those are not errors but recommendations. The description on those recommendations are pretty obvious though. Those are just text highlighting it shouldn't really matter. Have you tried setting an xml header? header ("content-type: text/xml");
-
To give you a valid answer which I prob should have done before. What webserver are you using? If you are running apache have you looked up mod_rewrite? I'ts pointless to dive into these rewrite rules when you're not able to have mod_rewrite. Maybe you're running IIS and you want to run ISAPI Rewrite
-
dynamic menu data from mysql with php
RichardRotterdam replied to nehapuniani's topic in PHP Coding Help
To start we need to see what your database tables look like. Do you have some code so far? -
Me -> new_to_oop(); Can't declare new variable in a method?
RichardRotterdam replied to br3nn4n's topic in PHP Coding Help
What version of php are you running? perhaps php 4? I dont get this error "Parse error: syntax error, unexpected T_VAR in ..." I do see something wrong in the following function query($query) { var $q; $q = mysql_query($query) or die("Error, please try later"); $q = mysql_fetch_array($q); return $q; } The var infront of the $q shouldnt be inside a function remove var here -
RSS is nothing more then a XML. Do a select query and echo it out as RSS. What part are you stuck on?
-
From time to time I'm trying to catch up on my French. A penpal would be handy for this however I do not really find the right trigger to sign myself up. Here are a couple of reasons: Think of what people would be looking / what are their wishes. I'd like to filter by age and interest. Looking through the site I found this thing you name age cloud. Why is this named a cloud when all the sizes are equally large? Other then that I want them in order I'ts like creating a tag cloud of the alphabet when I want to search for every user under letter a A. That doesn't make much sense huh? Maybe I'd like to go on vacation to a country I haven't been to before and would like it to have someone guide me around. Let's see which countries are available. Hmmmm how can I see a list of all countries Now it might also be nice to have a picture of that person you might find interesting to have as penpal maybe there is a picture of a girl wearing a hat with a propellor on top that catches my interest. But yeah from what I see I should be centered a little more around the Penpals available. It needs more functionality around that. Hope you will find it usefull, Richard