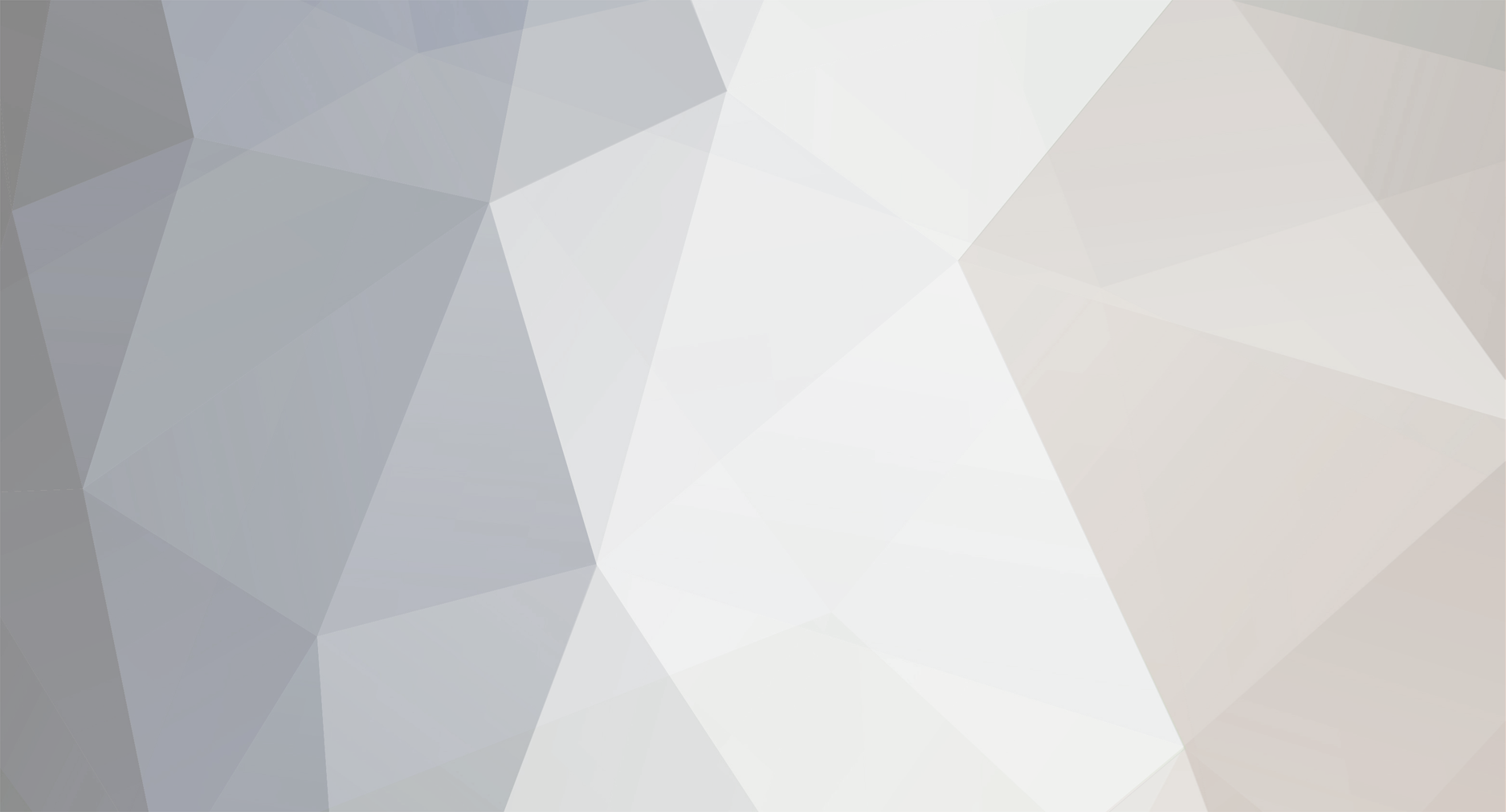
The Little Guy
-
Posts
6,675 -
Joined
-
Last visited
Posts posted by The Little Guy
-
-
I once worked at a company that created a database for every user.... wow....
If you create a table for every user or in my case it was a database, every time you change the table/database you will need to change ALL of them, not just one, and if you forget to change one then the person that owns that table/database will get lots of errors, and you will have a mess on you hands.
-
-
also, what is $invites?
-
I don't know what $user is, so maybe there is a better way, but based off what you posted
<?php $i = 1; while($data = mysql_fetch_array($user)){ $sql = mysql_query("SELECT COUNT(*) as total FROM `interested` WHERE `invites` = '".$invites['invites']."'")or die(mysql_error()); // remove "or die" after testing $count = mysql_fetch_array($sql); ?> <tr> <td><?php echo $i; ?></td> <td><?php echo $data['email']; ?></td> <td><?php echo $count['total']; ?></td> <td><?php echo $data['signup_time']; ?></td> <td><?php echo $data['signup_date']; ?></td> </tr> <?php $i++; } ?>
-
I think a query like this will give you what your looking for:
echo "<table>"; $sql = mysql_query("select * from favorites f left join books b on(f.favorites_id = b.genre) where f.user = $user_ID"); while($row = mysql_fetch_assoc($sql)){ echo <<<ROWS <tr> <td>{$row["book_title"]}</td> <td>{$row["book_genre"]}</td> </tr> ROWS; } echo "</table>";
-
Look in your php.ini file, you should see something that looks like this:
session.save_path = "c:/wamp/tmp"
Note: your location will obviously be different than mine
-
update some_table set link = concat('http://', link);
-
If you want to add me, here is my info:
Username: TheColorRed
ID Number: #494
-
This will work too:
CREATE TABLE newTable SELECT f1, f2, f3 FROM links
-
I would do these modifiers:
$desc_pattern = '/\<div class=\"on\">.*<p>(.*)<\/p><\/div>/isU';
-
You could choose one of these patterns:
$desc_pattern = '/\<div class=\"on\">.*<p>(.*)<\/p><\/div>/s';
$desc_pattern = '/\<div class=\"on\"> <p>(.*)<\/p><\/div>/s';
-
I play!
"Nexus Wars" is the best custom map!
-
because your string has a space between your opening div/p, but your regular expression doesn't allow for that.
-
echo date("t", strtotime("march 2011")); echo "<br />"; echo date("t", strtotime("previous month")); echo "<br />"; echo date("t", strtotime("now - 2 months")); echo "<br />"; echo date("t", strtotime("now + 2 years")); echo "<br />"; echo date("t", strtotime("now + 2 years 1 month"));
-
When this comes out will will need to have 3D glasses to browse the web.
-
No, you need to select it first, and put the results into an array, then update it to the new value and then have the function return the array.
-
Run this query:
alter table members change updated_on updated_on timestamp;
This will change the column to an auto update field, so every time a field gets inserted/updated this field will reflect the date/time that it happened.
Your select query makes no sense. If you already know the email why are you using a query to select it?
Does it really matter that I am doing the UPDATE based on "email"??
Only if your 100% sure the email is unique.
I would then run this query:
alter table members add unique (email);
That will enforce unique emails, here is a tutorial I wrote on it:
http://tutorials.phpsnips.com/MySQL/Manipulate_Table/Insert_unique_values
-
MySQL has a built in update, so you wouldn't even need to add that to your query.
You could then run this, and the updated field will update automatically:
UPDATE member SET pass=md5('somePassword') WHERE member_id = 123 LIMIT 1
You should also update a member based on member id.
-
can you post the results of this mysql query:
describe members;
-
I was able to come up with something like this (untested):
create temporary table myFriends select friend_id from partners where user_id = 123; -- List of your friends create temporary table myFriendsFriends select friend_id from myFriends left join partners on (myFriends.friend_id = partners.friend_id); -- List of your friends friends select mff.friend_id mff_id, partners.friend_id from myFriendsFriends mff left join partners on(mff.friend_id = partners.friend_id) having friend_id null; -- List of your friends friends who are not your friends
-
like this:
mysql -u root --password=mypassword --max_allowed_packet=32M
-
include "config.php"; $sorgu = "select * from table where id=$id"; $sonuc = mysql_query($sorgu) or die(mysql_error()); if(mysql_num_rows($sonuc) == 0){ header("HTTP/1.0 404 Not Found"); header("Status: 404 Not Found"); readfile("/errors/404.html"); exit; } $baslik1 = mysql_fetch_row($sonuc);
-
If there are no results, give a 404 header:
header("HTTP/1.0 404 Not Found");
Google will eventually remove it from their DB. You'll also want to give a message saying it wasn't found.
That wont always work, when you throw an error page, you should do it like so:
header("HTTP/1.0 404 Not Found"); header("Status: 404 Not Found");
you will also want to make a custom 404 page, and in that case you would do this:
// If the file is not found run this: header("HTTP/1.0 404 Not Found"); header("Status: 404 Not Found"); readfile("/errors/404.html"); exit;
-
you should add a limit to your sub-query, it should only return one result. I assume your warnings will be telling you that.
Anyone know where I can get a font/tutorial to make something like this
in Miscellaneous
Posted
why not just use the pencil tool?