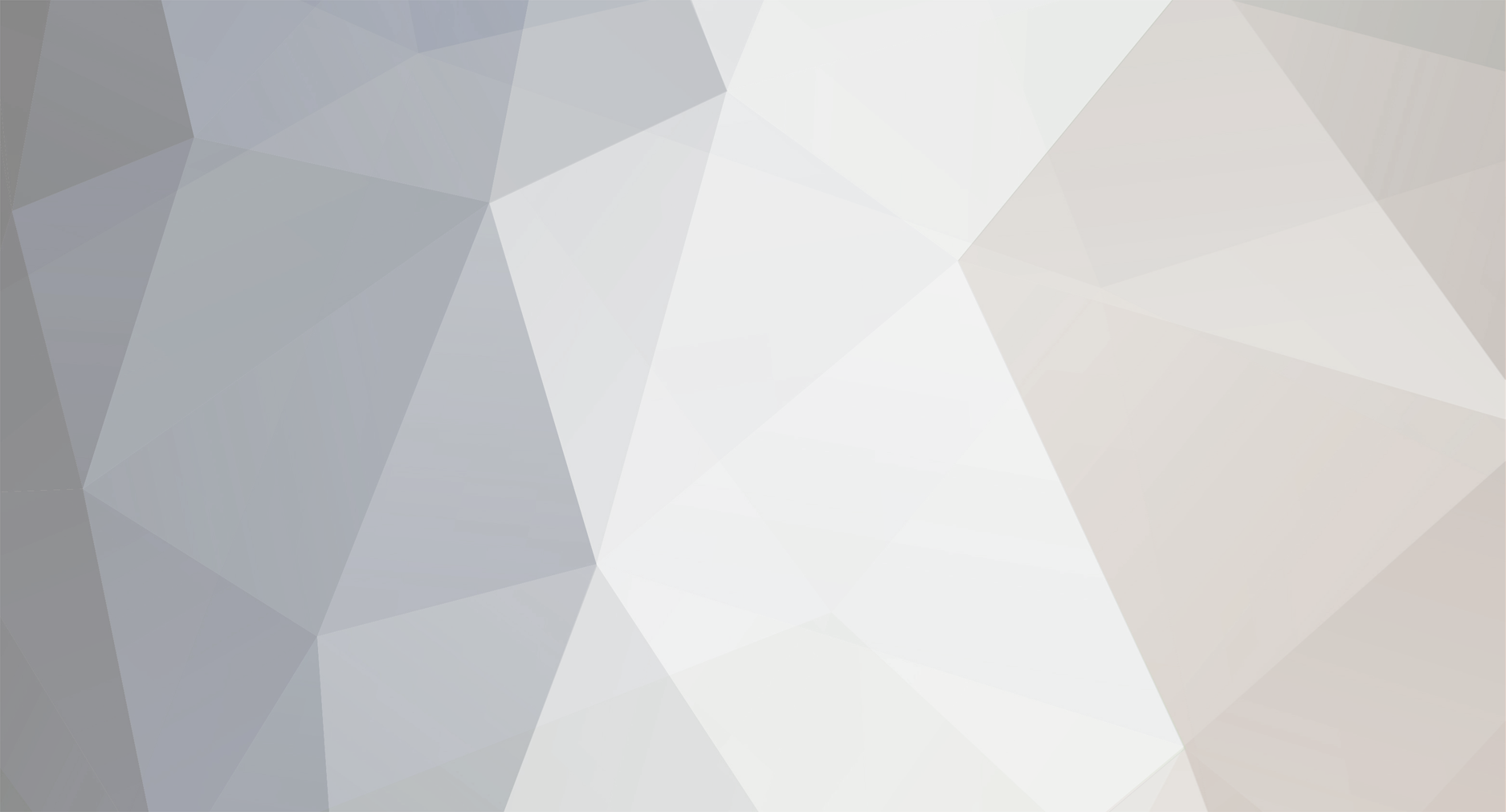
The Little Guy
-
Posts
6,675 -
Joined
-
Last visited
Posts posted by The Little Guy
-
-
I am not sure how to explain this, so I have some examples to go along.
So This is how classes are normally laid out (from everything I have seen).
Base Class / \ Class A Class B
You initiate class A or class B and inherits the base class functionality along with it.
What I would like to do is something like this:
Base Class / \ Class A Class B \ / Root Class
I would like to initiate the Root Class, and it inherits functionality from class A and class B and the base class.
Here are my thoughts:
You create an instance of the root class, this wouldn't do much other than tie everything together. Class A and B would have many similar functions such as process(), refund(), etc. (this whole ordeal is for credit cards, were going to be using 2 Gateways). So basically the root class will decide which gateway to use, were going to do 50/50 using mt_rand for now, this would be in the root class. Then we call Class A or B which would be able to use functions from the base class such as getting the card from the database, decoding the hashed cc number value back to a valid cc number, etc.
How should I make something like this?
Thanks!
-
horse and carriage or motor vehicle?
-
You should only have one ServerName directive er vhost.
Use:
ServerName weblyize.com ServerAlias www.weblyize.com
That works too!
-
Yeah I just got it working.
It was an Apache thing.
I had this in the VirtualHosts
ServerName www.weblyize.com
I then changed it to this:
ServerName weblyize.com ServerName www.weblyize.com
Thanks for every ones help!
-
Okay! I have gotten it to work!
One question I now have is why does this work:
and this not work:
Oops! Google Chrome could not find weblyize.comDid you mean: www.weblyize.com
Additional suggestions:
Access a cached copy of weblyize.com
Search on Google:
What would cause that? Apache? Bind? Godaddy?
-
$points = mysql_query("select sum(points) as points from tb_users"); $row = mysql_fetch_array($points); echo $row[0];
-
select sum(points) as points from my_table;
-
-
Try this, it converts 2+ spaces into 1 space. It will the split the words into an array, any empty item in the array was a punctuation mark.
<?php header("content-type: text/plain"); $str = "this is my string. It is awesome!"; $str = preg_replace("/\s\s+/", " ", $str); $arr = preg_split("/ |!|\./", $str); print_r($arr); echo $str; ?>
-
There has to be something else in the javascript that your not showing use, because that works perfectly fine for me. Somewhere you must be setting message to something empty.
-
I don't think that is true sockets, but I may be wrong.
-
JavaScript doesn't have socket support, but Flash does. Flash is your middle man here, it will listen to the server and tell javascript to do something. JavaScript can also tell flash to do something and flash will then tell the server.
As I can tell, this is your best solution.
-
I am going to make a JS API that connects to a PHP script, does anyone have any suggestions on how to make it so that the API only works on the site of the user who registered the site?
Here are/were my thoughts of doing this:
[*]create a unique code that is required to identify the site
[*]check the referrer of the site that is connecting to the API
[*]if the two values match up in the database allow usage of the API
[*]if the two values don't match up in the database don't allow usage of the API
That is what I have come up with, now I am not sure how secure this would be so does anyone have any suggestions to make it so that only the registered domain can use the API?
-
It also depends on what version of MySQL you are running, the has been a HUGE improvement in InnoDB in Mysql 5.5 in selecting and inserting.
-
Try this:
SELECT pid FROM campaign_details WHERE camp_id='$camp_id' GROUP BY pid LIMIT 7
-
First of all, this id should NOT be a user id, it should be like a username. Mysql has a built in auto increment feature that should be used to to create unique user id's. What you have should be used to create something like a username or user code. You should not be using this to create distinct member ids, because it is bad practice.
Take YouTube for example they use a unique string to define a video, but that string is not the actual way they uniquely identify the video through out the database, and if they did the site would be slow because matching on strings is much slower than matching on unique ids.
So, to create your user's code, could do this:
A. Make a id column, which is a primary key and auto_increment
B. Make a column for the members code (xxx-xxx-xxxx) and make it a Unique column
do{ $str = round(rand(100,999))."-".round(rand(100,999))."-".round(rand(1000,9999)); $sql = mysql_query("insert into my_table (col1, col2, user_code) values ('$c1', '$c2', '$str')"); $affr = mysql_affected_rows(); if($affr == 1) break; }while(true);
-
Here is my regexp method:
<?php $content = file_get_contents('my_file.txt'); preg_match("/north-east|north/i", $content, $matches); $north = 0; $ne = 0; foreach($matches as $match){ if(strtolower($match) == "north") $north++; if(strtolower($match) == "north-east") $ne++; } echo <<<OPT North: $north<br /> North-East: $ne OPT; ?>
-
select * from table order by alphabet
-
found this, which seems too work too:
function rel2abs($rel, $base){ /* return if already absolute URL */ if (parse_url($rel, PHP_URL_SCHEME) != '') return $rel; /* queries and anchors */ if ($rel[0]=='#' || $rel[0]=='?') return $base.$rel; /* parse base URL and convert to local variables: $scheme, $host, $path */ extract(parse_url($base)); /* remove non-directory element from path */ $path = preg_replace('#/[^/]*$#', '', $path); /* destroy path if relative url points to root */ if ($rel[0] == '/') $path = ''; /* dirty absolute URL */ $abs = "$host$path/$rel"; /* replace '//' or '/./' or '/foo/../' with '/' */ $re = array('#(/\.?/)#', '#/(?!\.\.)[^/]+/\.\./#'); for($n=1; $n>0; $abs=preg_replace($re, '/', $abs, -1, $n)) {} /* absolute URL is ready! */ return $scheme.'://'.$abs; }
-
mikesta707 is missing a "}" at the end of the while loop, and it and you should be good.
-
Any thoughts on this function? Basically what it does is take the current users location ($location) and a relative path ($link) and convert it to a valid URL. I do know that this does work on my example, but does anyone have any suggestions on this?
<?php $location = "http://mysite.com/pages/images/docs/myfile.html"; $link = "../../games/game.html"; function resolve_url($current, $link){ $ui = (object)parse_url($current); $domain = $ui->scheme."://".$ui->host; $levelsA = preg_split("/\.\.\//", $link); $levels = 0; foreach($levelsA as $l){ if(empty($l)) $levels++; } $currentA = preg_split("/\//", $current); $length = count($currentA); array_splice($currentA, -$levels - 1); $currentA[] = preg_replace("/\.\.\//", "", $link); return implode("/", $currentA); } echo resolve_url($location, $link); ?>
Thanks!
-
yes, you will want to look into simplexml_load_file, it will return an array of the nodes. I don't know how your xml is set up, so I can't really tell you how to do it, but hopefully this will help you.
-
the name attributes need to have the same values, see below:
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd"> <html xmlns="http://www.w3.org/1999/xhtml"> <head> <meta http-equiv="Content-Type" content="text/html; charset=utf-8" /> <title>Untitled Document</title> </head> <body> <form id="form1" name="form1" method="post" action=""> <p> <label> <input type="text" name="img[]" id="img[]" /> </label> </p> <p> <input type="text" name="img[]" id="img2[]" /> </p> <p> <input type="text" name="img[]" id="img3[] " /> <label> <input type="submit" name="Submit" id="Submit" value="Submit" /> </label> </p> </form> </body> </html>
-
Also, it would probably be helpful to let us know what your trying to achieve.
please help limit unix_timestamp by last 7 days
in PHP Coding Help
Posted