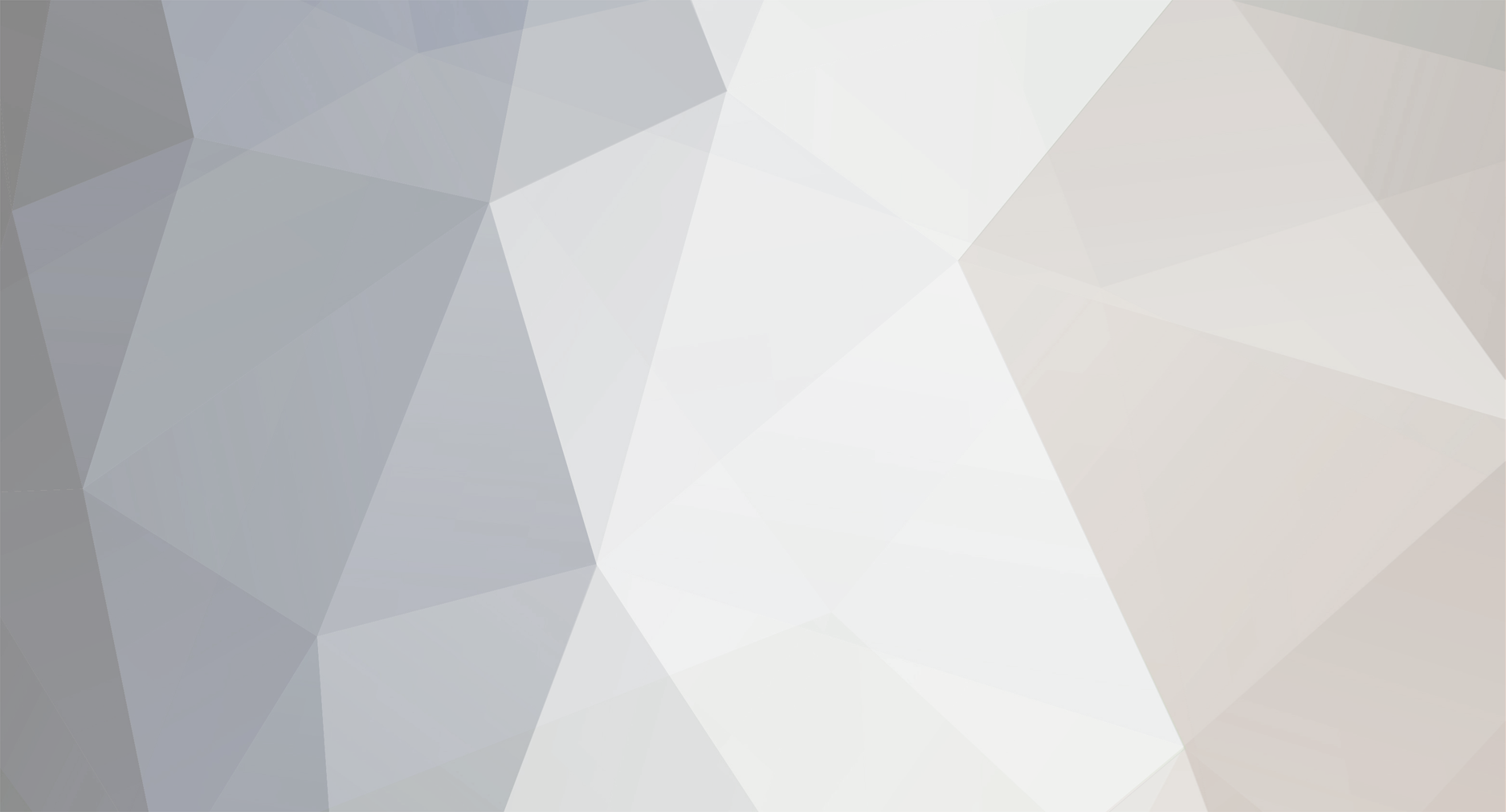
The Little Guy
-
Posts
6,675 -
Joined
-
Last visited
Posts posted by The Little Guy
-
-
in your table, I assume you have a column that tells which product the image belongs to; if not, add one.
$product_id = (int)$_GET['id']; $sql = mysql_query("select * total from images where product_id = $product_id"); while($row = mysql_fetch_assoc($sql)){ echo <<<OPT <a href="/images/{$row["pics"]}"><img src="/images/{$row["pics"]}" /></a> OPT; }
-
Something like this:
$root = "/path/to/users/"; $username = $_POST["username"]; // A pre-validated username if(!is_dir($root.$username)) mkdir($root.$username); if(!is_dir($root.$username."/images")) mkdir($root.$username."/images"); if(!is_dir($root.$username."/images/thumbs")) mkdir($root.$username."/images/thumbs");
-
Some new Changes:
- The image file size is smaller
- The main image is now a slideshow
- The header has a new background texture
-
Okay, Scroll is gone
-
Apache is on server A, and MySQL is on server B, they now have the ability to take a higher load.
if MySQL is bogging down server B, it won't affect Apache on server A, unless you have a script trying to talk to MySQL on server B.
Lets say you are running a intensive script that is using 90+% CPU server B will/may become very slow and almost unresponsive. If this happens and Apache is on the same server, it will have a harder time trying to answer incoming requests. By splitting the two up, Apache can respond almost instantly in the same situation. But if your web script that is running from Apache (server A) needs to talk to MySQL (server B) it will slow down.
-
-
Thanks guys!
I fixed almost everything you guys suggested:
- I made it so the nav doesn't ever move, or hide
- The "About Suzies" link, works for me in IE/Chrome/Firefox, so I can't really fix it because I can't duplicate the problem.
- The menu, I can't figure out how to make fit to the screen, I read I needed to do this:
<object data="/media/pdf/menu.pdf#view=FitV" ...
- But that solution didn't work at all, so if anyone konws any solution to fix that, that would be great.
- In the places that don't have any content, I added some basic text to it.
- The logo is now clickable
Todo:
- Resize the file size of the club sandwich
- Fix the texture (I would like to make it look like a weave if anyone has a Photoshop tutorial let me know)
Thanks again!
-
I spent all day yesterday making this layout, I would like it if you could give me some feedback on what you think. Right now there isn't much content, but that isn't what I am currently after. I designed it with Chrome 16, I am still working on getting it to look like chome in IE, and there is a small almost unnoticeable bug in Firefox. Another thing I need to do is make the main image on the home page smaller (500k is too large).
Other than all that, what do you think?
-
I have the following custom font:
@font-face { font-family: ws; font-style: normal; src: url(/media/fonts/ws.eot); src: local("WebServeroff"), url(/media/fonts/ws.otf) format("opentype"); font-weight: normal; font-style: normal; }
But it doesn't work in IE any reason why?
-
you can use ftp to bypass php max file upload limit I believe (Not the only use though). You don't need to use it for download though.
php.net file upload:
<?php $file = 'somefile.txt'; $remote_file = 'readme.txt'; // set up basic connection $conn_id = ftp_connect($ftp_server); // login with username and password $login_result = ftp_login($conn_id, $ftp_user_name, $ftp_user_pass); // upload a file if (ftp_put($conn_id, $remote_file, $file, FTP_ASCII)) { echo "successfully uploaded $file\n"; } else { echo "There was a problem while uploading $file\n"; } // close the connection ftp_close($conn_id); ?>
php.net file download:
<?php // define some variables $local_file = 'local.zip'; $server_file = 'server.zip'; // set up basic connection $conn_id = ftp_connect($ftp_server); // login with username and password $login_result = ftp_login($conn_id, $ftp_user_name, $ftp_user_pass); // try to download $server_file and save to $local_file if (ftp_get($conn_id, $local_file, $server_file, FTP_BINARY)) { echo "Successfully written to $local_file\n"; } else { echo "There was a problem\n"; } // close the connection ftp_close($conn_id); ?>
-
add an extra quote at the end of the query.
-
I see that your shops table isn't using an index, though I don't know if that will fix the speed because you say it is faster without the order by.
But, an order by is the last thing to run in your code, first it needs to select all the data that it needs, then it needs to go through all that data again to choose the order it needs to return it in, it is almost like running 2 queries, but then again you have 3 orders, so it needs to order it 3 times. order by is a slow operation, and there is nothing via MySQL to speed it up, so if you remove it and order it using php it could speed things up.
-
add an explain in front of your select, like this:
EXPLAIN SELECT p.*, shop FROM products p JOIN users u ON p.`date` >= u.prior_login and u.user_id = 22 JOIN shops s ON p.shop_id = s.shop_id ORDER BY shop, `date`, product_id;
Post the results.
-
the if statement has a value that will ALWAYS return true, $HourOn will ALWAYS equal $HourOn
Also, $MinuteOn will never be less that $MinuteOn
-
You can do this, then you don't have to escape your html quotes, and still use variables without having to concatinate:
echo <<<OPT <a href="#"><img src="$phpVariable" width="125" height="156" alt="some image" /></a> OPT;
-
ip2long should help you with what your looking for.
<?php $ip = $_SERVER['SERVER_NAME']; var_dump(ip2long($ip)); ?>
-
shouldn't it be calling it from the location of the file that has the require?
I am including:
"API/v1/class/Main.php"
Within that file I am including:
"../../media/php/classes/Mysql.inc.php"
shouldn't it be up two directories from where "Main.php" is, and not the current running file?
-
Why does this code:
require_once "../../media/php/classes/Mysql.inc.php";
Work when I go to:
http://mysite.com/API/v1/index.php
But when I go to:
it doesn't work.
The require is being called from another class, which I call from the two pages above. The class loads, but in the second link, I get failed to open stream: No such file or directory but the first one works fine. What would cause this?
could this be an htaccess thing?
htaccess:
Options +FollowSymlinks RewriteEngine On RewriteBase / RewriteCond %{REQUEST_FILENAME} !-f RewriteCond %{REQUEST_FILENAME} !-d RewriteCond %{REQUEST_FILENAME}.php -f RewriteRule ^(.+)$ /$1.php [L,QSA]
-
It was the margin on my <h1>
-
If you look on this page:
There is a gap between the search bar and the blue box below it, I can not find where that gap is coming from, so can someone please help me find what is creating that gap?
-
I am try to make a javascript API, and I only want the site that register on my site to be able to access the API.
So, for example:
http://example1.com - A pretend registered site
http://example2.com - A pretend non-registered site
so, example1 one signs up and creates 2 hash codes to use that get validated on my server against the domain name. To make sure their site is a valid site, they need to pass to my API: the domain, and the two hash codes, which must match in the database. The problem is that you can view the source so the owner of example2.com can get the hash codes really easy, so all you need to do is use those hash codes, and modify the domain it is coming from, and now example2.com can get data as if they were example1.com.
Any ideas how I can make it so only the domain that runs the code can access the data?
What this is:
The site is an indexer, it indexes site data and when you do a search from your domain with your two codes it get all pages related to the search that are registered under your domain.
Does that all make sense?
-
An example would be:
<?php $id = (int)$_GET['id']; $sql = mysql_query("select * from my_table where id = $id limit 1"); if(mysql_num_rows($sql) > 0){ $row = mysql_fetch_assoc($sql); echo <<<OPT <img src="{$row['photo']}" alt="Image" /> OPT; }else{ header("location: /error.php"); exit; } ?>
-
The following code will update all fields that don't have a space, if it does have a space it will ignore them.
Note: May want to back up the table before you run this
update hotels set est_postcode = if(name regexp ' ', name, concat(substring(name, 1, (length(name)-3)), ' ', substring(name, -3)));
-
when looping through a list of MySQL results, you should use a while loop. mysql_fetch_array will return a value every time, and if it doesn't return a value it will return false which will break out of the while loop.
Trouble with query
in MySQL Help
Posted
I would suggest removing the where an placing it in the join:
I have had a similar problem, and doing that has worked for me.