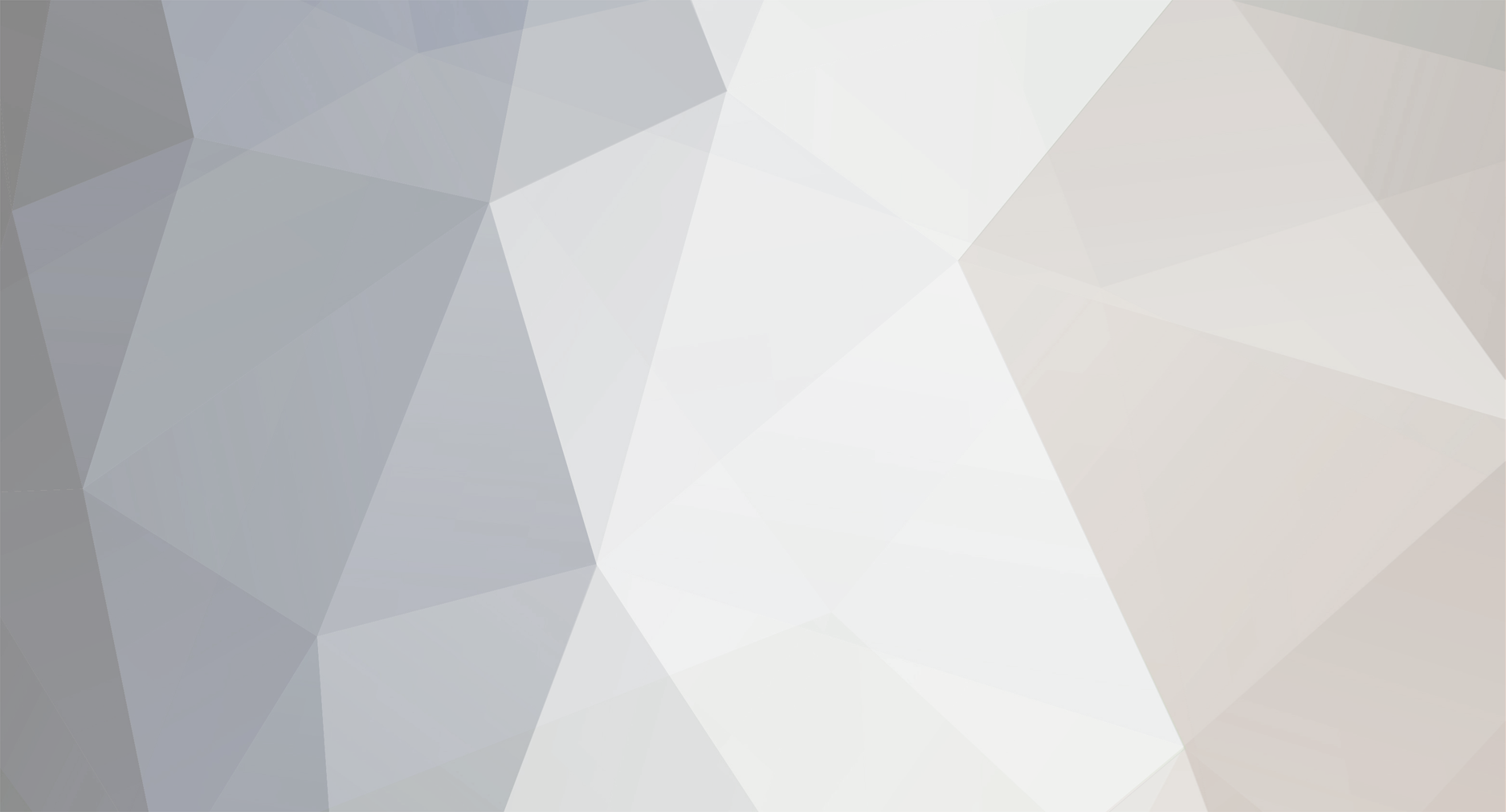
The Little Guy
-
Posts
6,675 -
Joined
-
Last visited
Posts posted by The Little Guy
-
-
With an md5, you have:
63,340,286,662,973,277,706,162,286,946,812,000,000,000,000,000,000
different combinations.
-
This worked for me:
<?php $blacklistArr = array("email@email.com", "email2@email.com", "email3@email.com", "@gmail.com"); function isBL($e){ global $blacklistArr; $isBlackListed = false; foreach($blacklistArr as $email){ $email = preg_quote($email); if(preg_match("/$email/i", $e)){ $isBlackListed = true; break; } } return $isBlackListed; } $tests = array("email@email.com", "myemail@gmail.com", "email23@email.com", "email2@email.com", "mike@yahoo.com"); foreach($tests as $email){ echo "$email: "; var_dump(isBL($email)); echo "<br>"; } ?>
-
the easiest way to generate a unique number is to use a myql auto_increment.
-
Just moved this to the end of the <body> block
$("img#ximage").load(function(){ $(this).fadeIn("fast"); });
-
Why doesn't my image load() fire?
The image loads, but the function doesn't fire. This part works: $("#main").fadeIn(3000);
<script type="text/javascript"> $(window).load(function(){ $("#main").fadeIn(3000); $("img#ximage").load(function(){ $(this).fadeIn("fast"); }); }); </script>
<body> <div id="main" style="display: none;"> <div id="X"><img src="/images/x.png" alt="X" id="ximage" style="display: none;" /></div> <p>Some text goes here</p> </div> </body>
-
did you grant that IP the correct permissions?
-
Try this:
$q = "SELECT song_id, song_title, added_date, datediff(now() - interval 7 day, added_date) as date, (downloaded / datediff(now() - interval 7 day, added_date)) as average FROM songs ORDER BY downloaded DESC";
-
what is the error?
-
I actually take back my previous post, and think you should do this:
set @m:=0; select * from (SELECT member_id, (v1+v2+v3) AS total, @m:=@m+1 AS rank from members order by total desc) as tbl where member_id = 2;
-
Try this:
SELECT `username`, (`strike`+`defence`+`covert`) AS rank FROM `accounts` ORDER BY rank
-
wamp requires phpmyadmin to be called from localhost.
-
This should work: mb_strlen
-
I have 2 indexes on the table and ~130 row in it.
+---------------+------------------+------+-----+-------------------+----------------+ | Field | Type | Null | Key | Default | Extra | +---------------+------------------+------+-----+-------------------+----------------+ | search_id | int(10) unsigned | NO | PRI | NULL | auto_increment | | domain_id | int(10) unsigned | NO | MUL | NULL | | | ip | int(10) unsigned | YES | | NULL | | | search_string | char(50) | NO | | NULL | | | search_date | timestamp | NO | | CURRENT_TIMESTAMP | | +---------------+------------------+------+-----+-------------------+----------------+
+----------+------------+-----------+--------------+-------------+-----------+-------------+----------+--------+------+------------+---------+---------------+ | Table | Non_unique | Key_name | Seq_in_index | Column_name | Collation | Cardinality | Sub_part | Packed | Null | Index_type | Comment | Index_comment | +----------+------------+-----------+--------------+-------------+-----------+-------------+----------+--------+------+------------+---------+---------------+ | searches | 0 | PRIMARY | 1 | search_id | A | 134 | NULL | NULL | | BTREE | | | | searches | 1 | domain_id | 1 | domain_id | A | 2 | NULL | NULL | | BTREE | | | +----------+------------+-----------+--------------+-------------+-----------+-------------+----------+--------+------+------------+---------+---------------+
-
What would cause this line:
insert into searches (domain_id, ip, search_string) values ($domain_id, inet_aton('$ip'), '$string');
To take 0.06 seconds to run? It is only run one time. That takes up half the time it takes my 8 other queries to run (inserts and selects).
-
I am trying to add a primary key on a temporary table, but it isn't working.
Here are the following queries:
create temporary table rt_results (document_id int, weight int, primary key(document_id)); insert into rt_results values(1, 123); explain select dom.domain_id, domain, url, title, content from rt_results rt left join documents doc on (rt.document_id = doc.document_id) left join urls u on(doc.document_id = u.document_id) left join domains dom on(doc.domain_id = dom.domain_id) order by rt.weight desc;
And here is the explain result:
+----+-------------+-------+--------+---------------+-------------+---------+------------------------+------+----------------+ | id | select_type | table | type | possible_keys | key | key_len | ref | rows | Extra | +----+-------------+-------+--------+---------------+-------------+---------+------------------------+------+----------------+ | 1 | SIMPLE | rt | ALL | NULL | NULL | NULL | NULL | 1 | Using filesort | | 1 | SIMPLE | doc | eq_ref | PRIMARY | PRIMARY | 4 | search.rt.document_id | 1 | | | 1 | SIMPLE | u | ref | document_id | document_id | 4 | search.doc.document_id | 1 | | | 1 | SIMPLE | dom | eq_ref | PRIMARY | PRIMARY | 4 | search.doc.domain_id | 1 | | +----+-------------+-------+--------+---------------+-------------+---------+------------------------+------+----------------+ 4 rows in set (0.00 sec)
Why does it say "NULL" for the key? I added a primary key to rt.document_id so shouldn't it use it? It seem logical that it would to me.
Thanks!
-
Here is another way:
<?php $string = "012345678912"; echo substr_replace($string," ", 7, -strlen($string)); ?>
-
I am doing this:
$fields = array( "code" => $this->code, "secret" => $this->secret, "domain" => $_SERVER['HTTP_HOST'], ); $this->ch = curl_init(); curl_setopt($this->ch, CURLOPT_URL, "http://weblyize.com/API/v1"); curl_setopt($this->ch, CURLOPT_FOLLOWLOCATION, true); curl_setopt($this->ch, CURLOPT_BINARYTRANSFER, true); curl_setopt($this->ch, CURLOPT_RETURNTRANSFER, true); curl_setopt($this->ch, CURLOPT_POSTFIELDS, $fields); curl_setopt($this->ch, CURLOPT_POST, true); $opt = curl_exec($this->ch); return $opt;
But the post fields are not being passed for some reason, and I can't figure out why. I do a print_r($_POST); on the url above, and it is empty.
Do you see anything wrong with my curl?
Here is the page:
-
-
select * from husbands join wives using(sid) join children using(sid) where husbands.sid = 4003 group by husbands.sid;
-
I got this to work for me:
select * from husbands join wives using(sid) join children using(sid) where husbands.sid = 4003;
output with 2 children (see attachment)
[attachment deleted by admin]
-
from what I am aware of, is that if you think there will be better performance, you are wrong (maybe I am but I don't think that will improve permormance).
-
the company doesn't have 5.4, so cant do that, but it was a good read!
-
Thanks everyone! Looks like I will be making a Factory!
-
js and php are just as comparable:
- Both are scripting languages; Both are modes of transportation
- One works with the file system one doesn't; One poops one doesn't
- One requires a server one doesn't; One is fast one is slow
- One can do ajax one can't; One needs an oil change one doesn't
- One same code works in all browsers other doesn't; One has built in satellite radio one doesn't
- Both are fun to program; Both die
- Both have similar functionality (Math, Strings, Classes); Both need food to keep it moving
- One runs in the browser one doesn't; One has hooves one doesn't
- One is as fast as the browser it is run in one is as fast as the server it is run on; One needs to be brushed one doesn't
See lots of similarities, and differences.
Get visitors ip & store it.
in MySQL Help
Posted
::1 is the IPv6 equivalent of IPv4 127.0.0.1 address.
You can convert IPv6 address to IPv4 address using function inet_ntop()
http://php.net/manual/en/function.inet-ntop.php