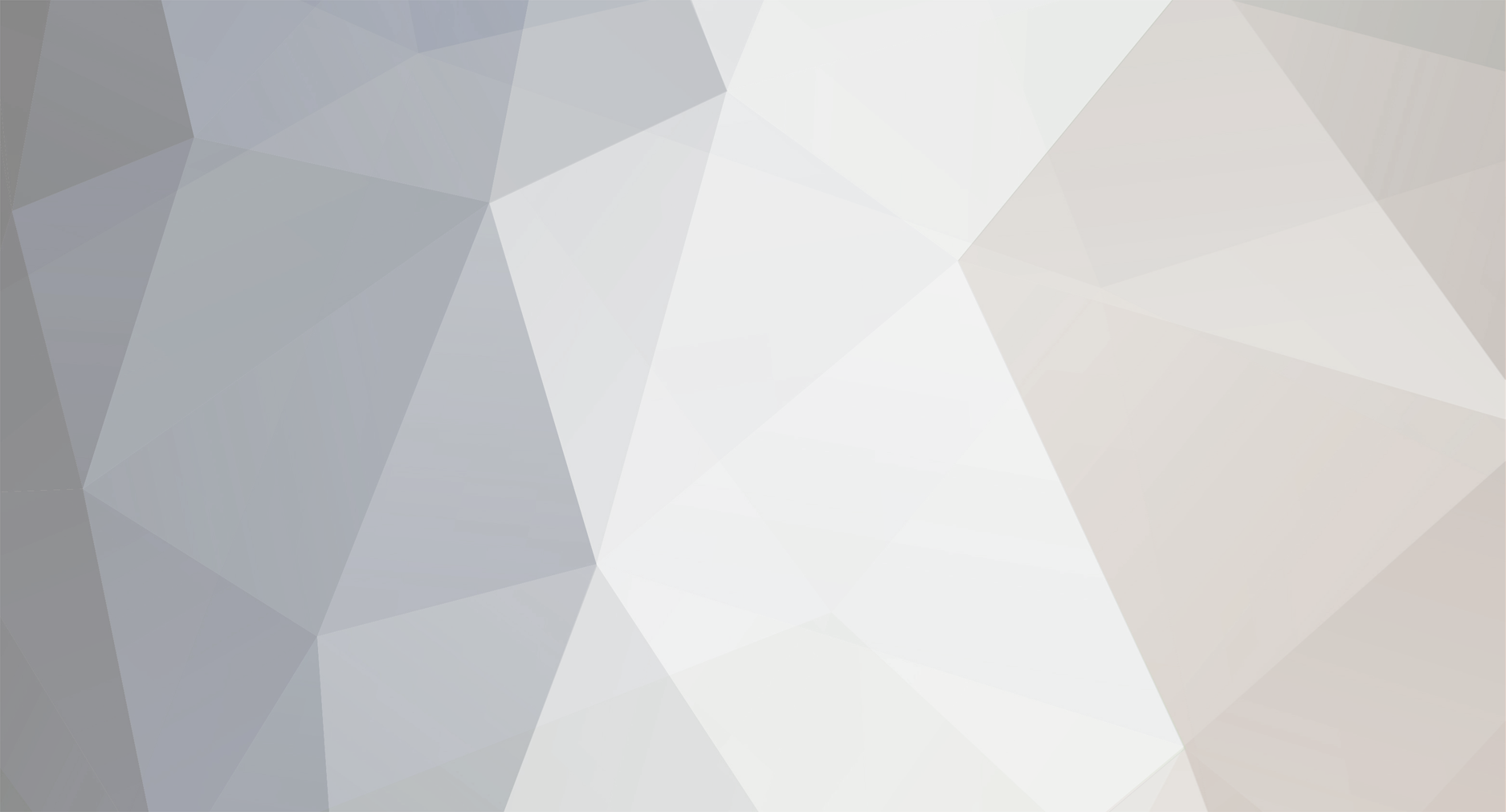
s0c0
Members-
Posts
422 -
Joined
-
Last visited
Never
Everything posted by s0c0
-
I recommend you run lamp, install an easy linux distribution such as fedora core, centos, or ubuntu and then just install apache, mysql, and php. Personally I suggest ubuntu. Seriously the AMP part was built for linux. But if windows works it works, just my 2 cents.
-
bump...response?
-
So let me get this straight. My classes are written in a page called actions.php, the user interacts with the game using index.php. I call these classes from index.php using the require function. So when I call these objects from index.php I am creating an instance of these objects. So I want to create the code that does the "battling" on index.php and store whatever variables in sessions within index.php's code. I want to do this because the classes I created on actions.php simply describes the object and some things the object will do, but what the object does in a given instance is performed within index.php. Am I getting this?
-
I understand sessions, but have only used them in username type scenario, what do you mean when you say serialize them? Also, what do you mean by creating new instances of my classes? If you think I need to do more reading please point me at a good book.
-
I am trying to learn object oriented programming in PHP 5. In doing so I am writing a very simplistic game. There is a Dragon object and a Hero object. The too should be able to exchange damage between eachother by passing eachothers hitpoints to the other and subtracting from their life. So how do I pass one objects variable to the other. I have tried including one class in the other and a bunch of other stuff, the only way I've been able to do it is on the actually page the user sees, but thats not what I want to do. Below is my code. <?php class Hero { public $herolife; public $herohitpoints; public $heroaction; public $herodamage; ///////////////////////////////////////////////////////// // public functions ///////////////////////////////////////////////////////// //// Action - calls additional functions based on user action public function Action($action){ $this->action = $action; if($action == "sword"){ $this->Sword(); } elseif ($action == "arrow"){ $this->Arrow(); } if($action == "evade"){ //Evade(); } else { //Shield(); } } //// Sword - attack with sword yields 5 to 10 hit points public function Sword(){ $this->herohitpoints = rand(5,10); } //// Arrow - attack with bow & arrow yields 0 to 15 hit points public function Arrow(){ $this->herohitpoints = rand(0,15); } } class Dragon { public $dragonlife; public $dragondamage; public $herohitpoints; public function Life(){ //$this->dragondamage = $dragondamage = $herostuff->herohitpoints; //$this->dragonlife = $herohitpoints - $this->dragonlife; if(!isset($this->dragonlife)){ $this->dragonlife = "50"; } } } ?> Question 2: What would be the best way to store each objects life after each round of play? Should these be stored in a url string and then somehow passed back into the class each round, should I use a mysql db?
-
Form validation: What dangerous characters should I look for?
s0c0 replied to MrCat's topic in PHP Coding Help
I would get rid of all < and > that should do it. If you are posting these fields to a sql query string you may want to block other stuff like DROP, SELECT, etc... -
I may be wrong be don't you need to specify the true command that will open the movie? For example <?php $command = "moviecommand /movie/Without a Paddle.avi"; exec($command); ?> Also notice I removed the echo from infront of exec. I don't think you can echo a function. So maybe first try w/o the echo, then explore a command that will actually open the movie.
-
You're right, this is in the wrong forum I had meant to post this in the help section. My apologies I was tired when I posted this last night. Mod's, can you please move my post? To answer your question, a web spider is a program that crawls the web and pulls back information. All search engines (google, yahoo, msn) use a spider to crawl the web looking for new sites to index. I need to bring my self up to speed on several aspects of PHP for a job I will be taking soon and they have asked that I develop a basic spider.
-
Can anyone point me to a how to on building a web spider or at least something that covers the concepts?
-
A search of this forum yielded this: http://developer.amazonwebservices.com/connect/kbcategory.jspa?categoryID=59 But, let me know if there is anything else out there.
-
Does anyone have experience with using php to interact with amazon.com's search service? I would like to be able to make calls to the service for artist and album information. Unfortunately most of the decent documentation (there's not much) is for .Net. My googling yielded information a pear class, but the documentation is poor. So can anyone point me in the right direction? Just looking for some good documentation or how to.
-
I have a javascript/html form that allows a user to specify multiple files for upload. This is passed into a php script that loops through each files and uploads them. The caveat with this is my program needs to have certain meta-data regarding the files stored in a mysql database. When this was just a one file upload form it was fine as the user would be taken to another form after submitting the file that would update the files record in mysql. Since I am now taking multiple files I am grappling with the many different ways of doing this and deciding on which is the best way. What is the best way to accomplish this? I can think of two right off the top of my head. 1. Once the files are uploaded store the files path in variables and take the user to the record update form, each time the user submits a record pass the next files path in a URL string until there are no more files left. 2. Every time a user adds a file to be uploaded (it has not been uploaded at this point) show the record update fields next it. Then pass all the information at once. Solution 1 seems like the easier and more half-assed solution. Solution 2 seems like the more elegant/web 2.0 solution, but will take more much longer to implement. Since I am not in the business of half-assing stuff I would go with solution 2, but I am very interested in your ideas and the possibility of more solutions.
-
In the settings.php file is the password just stored in there? Why are you not using a database?
-
Yes, I am using sessions, but none of what I have googled is solving the problem. Anyone have an exact how to on this or specific code? If not then I'll eventually figure it out for myself, thanks.
-
I've been getting strange output in my url string within my app lately. It adds stuff like ?1174406969965 when I'm not even posting a url string on my forms. This doesn't seem like a big issue, but I was wondering if anyone has ran into this before. I used the following to post to the page: <form action="<?php echo $_SERVER['PHP_SELF'];?>" method="post"> <?php /* If session variable is null then tell the user to login again */ if(!isset($user)) { echo "<p><strong>Your session has timed out, please login again.</strong></p> \n"; } /* begin html table for listing users mp3s */ echo "<table border='0' cellspacing='2' class='bubbletbl'> \n"; echo '<tr>'; echo "<td> </td> \n"; echo "<td width='130'><strong>Song</strong></td> \n"; echo "<td width='130'><strong>Artist</strong></td> \n"; echo "<td><strong> </strong></td> \n"; //echo "<td><strong>Genre</strong></td> \n"; //echo "<td><strong>Length</strong></td> \n"; //echo "<th bgcolor='#CCCCCC'>Playlist</th>"; echo "</tr> \n"; /* loop throgh user's mp3s and create html table row for each */ /* checkbox is given the songs mysql table id */ for($i=0; $i<$num_tracks; $i++) { $row = $trackresult->fetch_assoc(); echo '<tr>'; echo "<td><input type=\"checkbox\" name=\"track[]\" value=\"". $row['id'] ."\"></td> \n"; // radio value is mp3.id echo "<td><a href='" . $row['file'] . "'>" . $row['song'] . "</a></td> \n"; //link is mp3 file echo "<td>" . $row['artist'] . "</td> \n"; echo "<td><a href=\"javascript:popUp('/popup/update/index.php?id=". $row['id'] ."&uid=". $uid ."')\">Update</a></td> \n"; //echo "<td>" . $row['genre'] . "</td> \n" ; //echo "<td>" . $row['playtime'] . "</td> \n"; //echo "<td>" . $row['playlist'] . "</td>"; echo '</tr>'; } echo "</table> \n"; /* post checkbox variables to self, this will delete the selected records */ /* the code for this is at the top of the script around line 30-50 */ echo "<p><input type=\"submit\" name=\"delete\" value=\"Delete Tracks\"> \n"; echo "</form> \n"; ?>
-
I don't know what options godaddy gives you so ask them if you can update your A record. You will need to point your DNS A record at your home computers IP address. I assume you know that you'll need to forward all port 80 requests to the web servers local IP or at least setup the web server as a DMZ host.
-
I don't get it. There is no auto completion, no intellisense, and nothing remotely like eclipse or .net. So what makes it different then all the other text editors and stuff out there? Not to be harsh or anything, but tell me if there is something I am missing. If you just created this for personal use and to learn a new language, than congratulations, it's more than I can do. It's fast and has no bloat, which I like. If it had auto completion and intellisense than I'd be using right now.
-
It's like a 13 yr old built a website in 1995 and then puked all over his design. Why can't these people pull their heads out of these 1990s-no skill sites. For christ sake, go spend $40 on a template from templatemonster.com
-
I like it. You can easily tell it's a mouse now (which is not a bad logo idea to begin with). In your first logo design I thought it was like a hammer sickle or something. No one wants to get pc support from the commies lol. I also think the font style is appealing.
-
http://www.templatemonster.com/ look at all the templates in the religion category and see if you can get some ideas. There is nothing wrong with gaining inspiration from web designs and taking ideas away from them, but there is something wrong with copying code word for word. Perform searches via google on topics such as religion, church etc... to get further site design ideas. It doesn't all have to be about looking at religion sites for inspiration though, since your site is about issues, then look at issues sites to get an idea of the overall layout. You also may want to consider looking at a wordpress blog as your site design. There are numerous themes, all of which can be modified.
-
Overall layout is good, logo is good, maybe change how the links like on hover for your navbar, and look at other backgrounds.
-
Where did you get your flash-music player? Is this something you purchased, developed yourself, or is it freeware? Btw, I like the site, but don't settle there is always room for improvement.
-
Well in that case as you know the customer is always.... If you get paid and the customer is satisfied then that's all that matters in the end, but if I had to pages open, yours and the one with the changes I recommended, I would look at the later first.
-
If I was designing this site and I had settled on this particular layout (which is fine) I would change your body background to white and get rid of the red. I'm not sure that I dig the green either. I think you just need to play around with your color schemes at this point.
-
Holy crap! It looks horrible in 1024x768. I work in 1440x900 res. A good lesson learned now is to test in multiple resolutions. The nav bar is supposed to appear in the main white bubble to left of the text. I will fix this immediately...thanks!