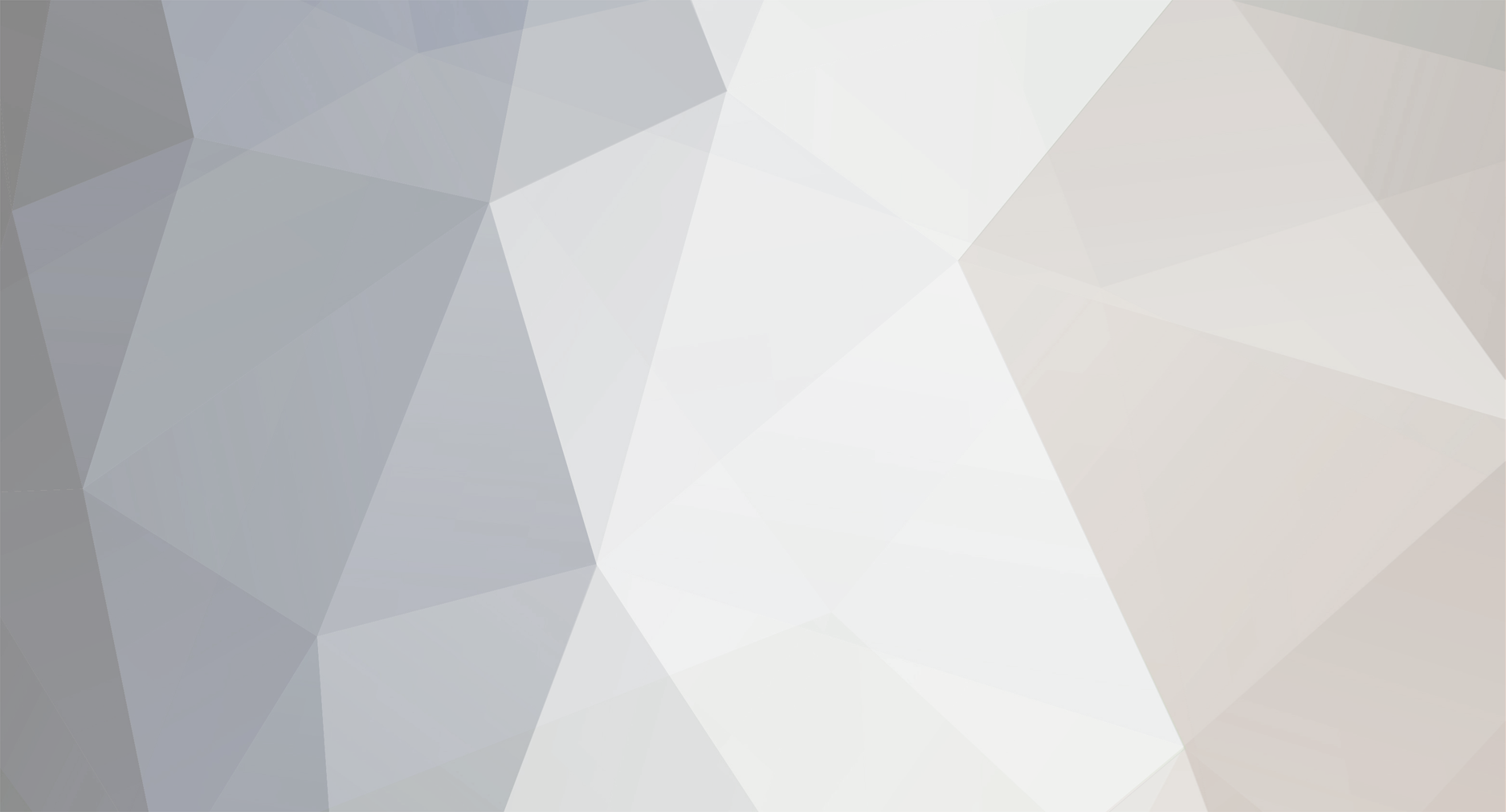
s0c0
Members-
Posts
422 -
Joined
-
Last visited
Never
Everything posted by s0c0
-
I can't see anything immediately wrong in the code, but I could be missing something. Have you tried running that query on its own to verify it returns 6 records?
-
When I've had to do this I used an array. In fact I'll have two arrays. One contains the days of the month (ie. cellArr[1]) for displaying the actual days on the calendar. As it loops through, my second array's key is the day of the month as a number, and its element is the data I want displayed. So when I'm looping through the cell array to show the actual cells I just do something like $dataArr[cellArr[$i]] to display the data I want. Does that make sense?
-
I do this on my server, but since its my server I just use image magick's convert command. My code looks like this: function imgResize($img) { exec('convert -size 125x125 '.$img.' -resize 125x125 '.$img); return true; } Where image is the full path to the file.
-
You take OOP too far when it makes it difficult for another programmer to jump in and start modifying your code. I've seen developers try and turn PHP in Java. PHP is far from Java and at some point you have to stop. There is no need for gets and sets in PHP (okay I do use sets in objects), but there is absolutely no need for gets to simply get object data members. Just instantiate the object and grab the data member for crying out loud. We use this method for designing OOP at my work. Core object interacts with the database. For instance we would have an object called C_User that interacts with the user table. This objects job is to run queries and set object variables that is it. So it's constructor would look like this: function __construct($user_id='') { $dbConn = new C_DatabaseConnection(); $this->db = $dbConn->music(); if($user_id!='') { $sql = "SELECT * FROM users WHERE id='$user_id'"; $result = mysqli_query($this->db, $sql); while($row = mysqli_fetch_array($result)) { $this->username = $row[username]; $this->email = $row[email]; $this->status = $row[status]; $this->directory = $row[directory]; $this->traffic = $row[traffic]; $this->user_id = $user_id; } } } The class might also contain a query like this: public function getMusic() { $sql = "SELECT * FROM mp3 WHERE users_id='$this->user_id'"; return mysqli_query($this->db, $sql); } Notice I just return the query result. Now I can just call this method, get the query result and do whatever I want with that in other objects and methods. We then would have a T_User object which is used for displaying text to the screen. So in a very ugly and simplistic form it would look something like this: function __construct($user_id='') { $this->user = new C_User($user_id); } public function returnMusic() { $resultArr = $this->user->getMusic(); while($row = mysqli_fetch_array($resultArr, MYSQL_ASSOC)) { $retVal .= $row[song]; } return $retVal; } We also have H objects (ie. H_User) which are used for one-off tasks that don't fit into the core object or the text object. So you might have some number crunching functions in here or form validation in an H object. This is the sanest way I've found of implementing OOP in PHP.
-
I've been think about writing something fun as of late. My idea for an application is an online multiplayer browser based knock-off of the classic board game Risk. I would design it using PHP and JavaScript/Ajax and use MySQL as my backend. Is this something that you think would catch on if it had a ladder system, tournaments, unranked games, and stuff like that? Perhaps this is something that has been done too much and is not worth my time (in the interest of being original).
-
I would like to make a version of my site easily accessible via cell phones with internet access. If anyone could give me some information on how to do this and whether a cellphone would support something like flash or at the very least audio playback that would be very beneficial to me. I just don't know where to start.
-
Not sure exactly what you are asking so I'll take your question in a very literal sense: You can hide a checkbox from the users view using CSS, there is no easy way to do it though such as a hidden text field.
-
using a table in 2 seperate databases with a symbolic link
s0c0 replied to s0c0's topic in MySQL Help
My boss told me to make the new DB...6 weeks plus into development. Yeah, I was real happy about that. If I may ask, what is a federated table? -
I initially began a project with my tables in database A, but when the amount of tables needed grew to 20+ I was told to create a new database. This sucked because I am inner joining on tables within database A, of course moving my tables to a new database broke several queries. Rather than creating objects to extract the data out of database A and work with my existing code our network administrator came up with an interesting solution. Since mysql stores tables as files within the databases folder on the file system, why not make a symbolic link to the tables. This is working for me in a development environment, but I am worried about potential problems that could arise from this. Has anyone on here tried this approach before? Any ideas on the possible downside of this?
-
I have this query: SELECT (sum(wsale_cost)*sum(quantity)) as cost FROM wsale_orderedProd WHERE invoice='14277333' The wsale_cost datatype is float(5,2) and that query yeilds the following result: 170.50000190735 on a calculator it yields 170.50. I realized that I can wrap ROUND(x,2) where x is the formula and get it look like 170.50, but why the hell does float do this? Should I instead be using decimal? I've read that float has more efficient data storage. Strange...can't find a good answer on google, maybe one of you can help. For right now I'm switching to decimal(5,2) to avoid having to run an additional function inside my query.
-
So lets say I have an orders table with an invoice column as the primary key and several other columns. I'll auto-increment the invoice starting at the number 1 and I don't even anticipate there to be more than 1,000,000 records. So I set the type to medium int and assign it a value of 6. What I'm getting at is why is this a better practice than just assigning that column say a type of int with a value of 10? Is this even a best practice? Does this even matter? Please advise.
-
[SOLVED] 2 Questions: Locking/Unlocking a table and LAST_INSERT_ID()
s0c0 replied to s0c0's topic in MySQL Help
Thanks, and yes I am aware of the php function. -
I have two questions. I lock writing to a table so I can grab the last inserted auto increment primary key value. The entire code looks like this: mysql_query("LOCK TABLES wsale_products WRITE"); $sql = "SELECT LAST_INSERT_ID(prod_id) as id FROM wsale_products ORDER BY id DESC LIMIT 1"; $result = mysql_query($sql); $row = mysql_fetch_array($result); mysql_query("UNLOCK TABLES"); 1: I have found no way to unlock this specific wsale_products table. I have found only a generic UNLOCK TABLES query. It seems to me that this would unlock every table in the databases rather than just the wsale_products table. Is there a way to specify which table I want to unlock? My worry is that this method is conflicting with other database transactions. 2: Notice in my select last insert id statement that I have to order by and limit to 1 to retrieve that id. Without those parameters the query returns every id in the table. Am I utilizing this mysql function incorrectly? We are running MySQL 4.x and PHP 4.x, thanks in advanced. (Yes I am aware of the PHP mysql_insert_id function).
-
[SOLVED] setting text using innerHTML not working in Internet Explorer
s0c0 replied to s0c0's topic in Javascript Help
Wow no replies on this. I figured out a solution on my own. My problem was I was setting up ID names on TD tags which for some reason causes IE to error out. I got rid of those, and replaced them with span ID names, this resolved the problem. For instance if you have the following code: <script type="text/javascript"> var str = "hello world"; document.getElementById("text").innerHTML = str <script> And the following HTML: <table> <td id="text"></td> </table> This will not work, you must instead use <span id="text">, <div id="text">, or something like that to remain IE compatible. -
liebs19, can you post the code you were having problems with and then post the code with the solution. I am having the a very similar problem. Thanks.
-
I'm sure this has been asked before, but I'm asking again. What is the work around for this apparent bug? I have this snippet of code that works fine in FireFox, but throws an error in IE. /* try { var txtDocument = XMLHttpRequestObject.responseText; var totalsArr = txtDocument.split('-'); document.posfrm.total.value = totalsArr[0]; document.getElementById("total").innerHTML = totalsArr[0]; subTotalFrm.value = totalsArr[1]; taxFrm.value = totalsArr[2]; taxDiv.innerHTML = totalsArr[2]; shippingFrm.value = totalsArr[3]; shippingDiv.innerHTML = totalsArr[3]; infoDiv.innerHTML = totalsArr[4]; setShippingValues(totalsArr[4]); } catch(err) { alert("error getting order total amount"); } I've read several blogs, and even this KB (http://support.microsoft.com/kb/276228), but I can't seem to get any of the work arounds for this to work. Is there any easy work-around, please say yes, because I really don't want to "fix" over 1000 lines of JavaScript. P.s. Your boss tells you not to worry about IE compatibility, once you complete the project he comes back and says we need it to be IE compatible....go figure, thanks!
-
Using AJAX to inject and run Javascript on a page
s0c0 replied to noochies's topic in Javascript Help
I do something similiar on one of my Ajax pages. When a user does a product search it returns this to the div tag: $txt .= '<tr style="background-color:'.$this->returnRowColor($x).'"> <td id="'.$row[iD].'">'.$row[iD].'</td> <td id="'.$row[iD].'">'.$row[name].'</td> <td id="'.$row[iD].'">'.$row[price].'</td> <td><acronym title="add" style="color:#003F87; border:1px solid #ccc; cursor:pointer;" onclick=\'addProduct("'.$row[iD].'", "'.preg_replace($badCharArr, "", $row[name]).'", "'.$row[price].'", "1", "1", "'.round($row[cost],2).'");\'>add</acronym></td> <td><acronym title="description" style="color:#003F87; border:1px solid #ccc; cursor:pointer;" onclick=getDescription("'.$row[iD].'");>info</acronym></td> </tr>; Now it's still a user event that will actually add that product to the cart by clicking the acronym tag and this calling the addProduct function. Another bit of a code I have allows a user to import a shopping cart. Here is the code on my front-end page: /* virtual shopping cart import */ if($_GET[action]=='vsc_import') { $vscImportArr = $T_POS->virtualShoppingCartImport($_GET[cart_id]); $custArr = $vscImportArr[cust]; // contains customer information, used to pop. text fields $prodArr = $vscImportArr[prod]; // contains javascript functions calls, see bottom of page } Back-end code: function virtualShoppingCartImport($cartId) { /* set cart info, customer and shopping cart arrays */ $infoArr = $this->VscAdmin->get_cart_info($cartId); $itemsArr = $this->VscAdmin->get_cart_items($cartId); $custArr = $this->importCustomer($infoArr[customer_id]); /* create an array of javascript function calls of products in the customers virtual shopping cart */ foreach($itemsArr as $i) { $prodArr[] = 'addProduct("'.$i[pid].'", "'.$this->returnProductName($i[pid]).'", "'.$i[sellPrice].'", "'.$i[qty].'", "0");'; } /* append the javascript calcTotals function to the end of the array */ $prodArr[] = 'calcTotals();'; /* return arrays to be broken apart and processed by front-end code */ $retArr[cust] = $custArr; $retArr[prod] = $prodArr; return $retArr; } And at the very bottom of my front end page I have this: /* echo out javascript function calls for virtual shopping cart import */ if(is_array($prodArr)) { foreach($prodArr as $prod) { echo $prod; } } Now what makes this easy is, this is all done on page load. Hopefully these examples help. You probably need to return you're javascript with php and somehow an event will trigger these functions. Good luck. -
I'm looking for a good book on ajax that covers design patterns and security. I have the DOM down, along with GET and POST HTTP requests, so I'm looking primarily at ideas on optimizing code, making it more scalable, and learning a lot about securing Ajax from stuff like xss attacks etc. I took a look at the Head Rush Ajax book, but it looks like its for a beginner, I'm more of an intermediate developer.
-
Using AJAX to inject and run Javascript on a page
s0c0 replied to noochies's topic in Javascript Help
Do you have FireFox w/ the web developer and firebug extensions? If not, get it, and install those. Reload firefox, and run your code, there will be either a green circle with a white check mark in it or red circle with a white X in it. Click on that, its like a debugger basically for your javascript, see what the error is and post back what it says. It should show what your PHP responded back with and any javascript errors. Looking at your code doesn't throw up any red flags to me, so this is your best bet IMO. -
[SOLVED] Switched from GET to POST, but my PHP Get code still works?
s0c0 replied to s0c0's topic in Javascript Help
Figured out the problem. I was not doing a true POST. My JavaScript code was executing an HTTP POST, but the URL I was posting to contained all the old URL strings. So it posted nothing and when my PHP code saw all those GET values it got excited and did its thing. I've now fully switched over and things are working great. -
Much easier to secure ajax on an intranet, now on the internet, thats when you have to really be careful. Thanks for your comments, anyone else have input on this?
-
Word Press freaks out when I put in html and php tags, even when I paste these into its "code" blocks. I use my blog for storing technical information (code examples, linux configurations) to compensate for a horrible memory. I'm sure plenty of you have word press blogs out there, any ideas?
-
Don't really have time to check it out, but I'm looking at migrating away from WordPress. What can you tell me about the install, spam control, and if any skins/themes are available for it?
-
released my third beta, online music storage and playback
s0c0 replied to s0c0's topic in Beta Test Your Stuff!
Really? Can you please tell me your username so I can check the logs and see what happened. This was a problem a while back, hopefully it has not returned. -
Hello, I recently implemented what you are trying to do as a solution for a product order form for our customer service reps. Basically when they add a product, that product and all of its information is stored in a series of hidden fields. Perhaps this snippet of code will help: var prodsDiv = document.getElementById("addedProdsDiv"); prodsDiv.innerHTML += '<input type="hidden" name="idX'+prodCount+'" value="'+id+'" id="idX'+prodCount+'" readonly>'; prodsDiv.innerHTML += '<input type="hidden" name="prodX'+prodCount+'" value="'+product+'" id="prodX'+prodCount+'" readonly>'; prodsDiv.innerHTML += '<input type="hidden" name="priceX'+prodCount+'" value="'+price+'" id="priceX'+prodCount+'" readonly>'; prodsDiv.innerHTML += '<input type="hidden" name="qtyX'+prodCount+'" value="'+qty+'" id="qtyX'+prodCount+'" readonly>'; prodsDiv.innerHTML += '<input type="hidden" name="totalX'+prodCount+'" value="'+total+'" id="totalX'+prodCount+'" readonly>'; Don't worry about the prodCount, price, qty, and other variables in there. Those are just passed into the function. Specifically you may want to use the prodCounter idea though. Basically each time this function is called it increments this prodCount hidden field by one, so I can have unique names for my hidden fields. In case you are wondering about the readonly parameter, that prevents the user from modifying these hidden fields, even with something like FireFox web developer which gives you direct access to hidden fields. Not sure if its IE compatible code, since the company I work for standardized on FireFox