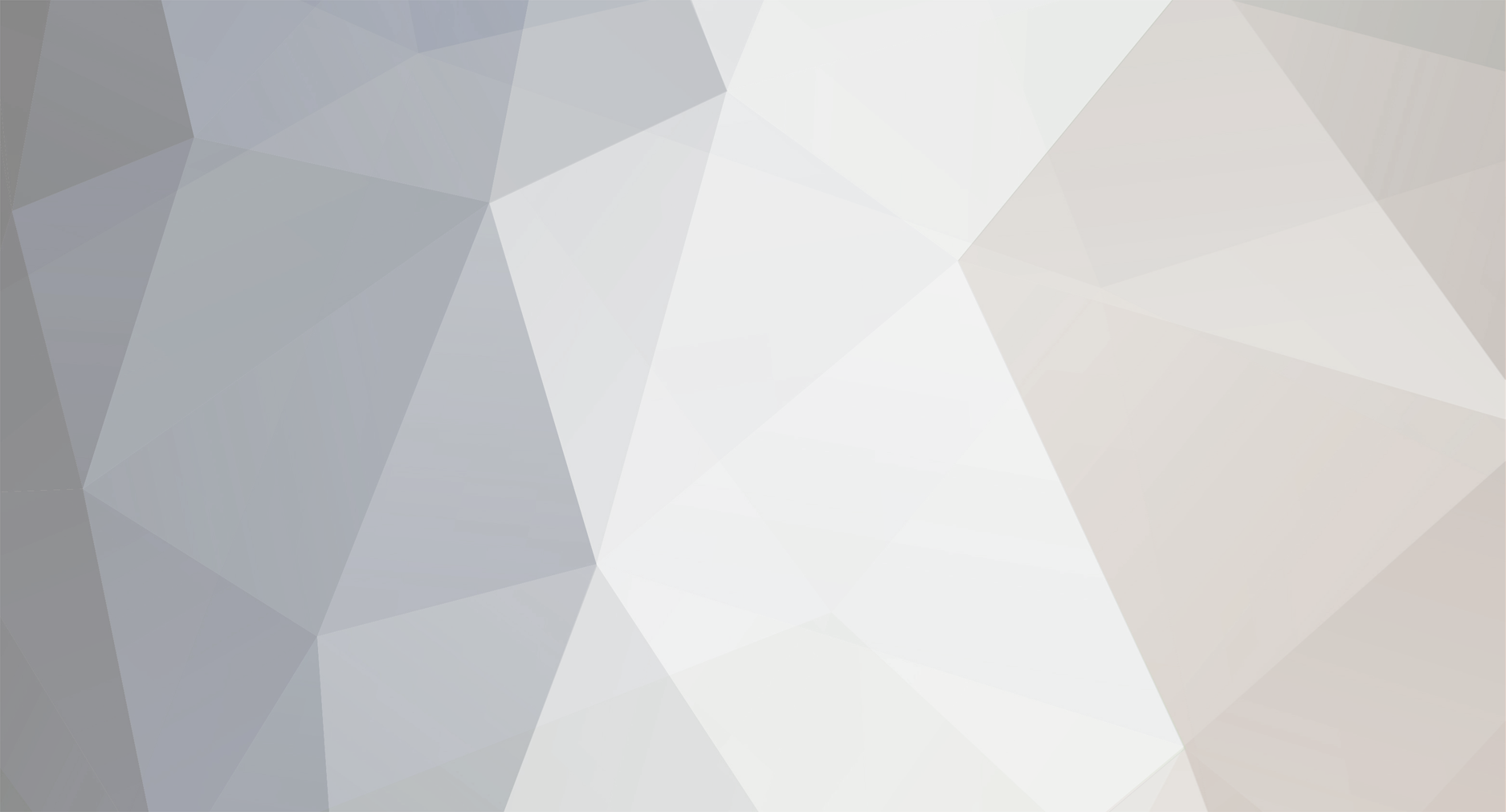
mga_ka_php
-
Posts
134 -
Joined
Posts posted by mga_ka_php
-
-
im trying to scrape the text of an image.
-
is this possible to get the text in an image?
-
thanks for all of your replies.
-
while loop is better?
-
i see that they use array in getting records from the database.
while ( $row = @mysql_fetch_object( $this->result ) ) { $this->last_result[$num_rows] = $row; $num_rows++; }
what if my records is 50,000. does array can hold that number of records, or does it use to much memory?
-
why does wordpress is using foreach rather than while in listing records?
common
while ($products = mysql_fetch_object($rs) { ... }
but wordpress is using foreach
foreach ($posts as $post) { ... }
which is better? because i'm trying to create my own code for database connection.
-
ok thanks
-
so mysql_real_escape_string removes the quotes?
-
i have a textarea and the content is description. how do i save this to database, because sometimes it has ' and " so it fails when i insert it into database, what should i use addslashes?
-
what is the for writing {
function myfunc {
or
function myfunc
{
it should be right after myfunc or below function myfunc?
-
i have a form submission and it saves in my database. but sometimes i got weird characters, so when i display it on my site. the text content got weird error symbols. how do you handle those? thanks.
-
how do you optimize this query?
SELECT SUBSTR(eventDetails, INSTR(eventDetails, '|#|Name') + 8, INSTR(eventDetails, '|#|StartDate') - INSTR(eventDetails, '|#|Name') - eventTitle, SUBSTR(eventDetails, INSTR(eventDetails, '|#|City') + 8, INSTR(eventDetails, '|#|State') - INSTR(eventDetails, '|#|City') - city, IF(UNIX_TIMESTAMP(SUBSTR(eventDetails, INSTR(eventDetails, '|#|StartDate') + 13, 10)) = 0, SUBSTR(eventDetails, INSTR(eventDetails, '|#|StartDate') + 13, 10), UNIX_TIMESTAMP(SUBSTR(eventDetails, INSTR(eventDetails, '|#|StartDate') + 13, 10))) eventStartDate, IF(UNIX_TIMESTAMP(SUBSTR(eventDetails, INSTR(eventDetails, '|#|EndDate') + 11, 10)) = 0, SUBSTR(eventDetails, INSTR(eventDetails, '|#|EndDate') + 11, 10), UNIX_TIMESTAMP(SUBSTR(eventDetails, INSTR(eventDetails, '|#|EndDate') + 11, 10))) eventEndDate, SUBSTR(eventDetails, INSTR(eventDetails, '|#|State') + 9, INSTR(eventDetails, '|#|Zip') - INSTR(eventDetails, '|#|State') - 9) eventState FROM events WHERE eventDetails NOT LIKE '%Canceled:YES%' AND eventDetails NOT LIKE '%State:|#|%' AND eventDetails NOT LIKE '%Status:DELETED%' AND (eventDetails NOT LIKE '%PromoterPhone:|#|%' OR eventDetails NOT LIKE '%Link1:' OR eventDetails NOT LIKE '%UserNumber:0|#|%' OR eventDetails NOT LIKE '%UserNumber:|#|%') AND SUBSTR(eventDetails, INSTR(eventDetails, '|#|State') + 9, INSTR(eventDetails, '|#|Zip') - INSTR(eventDetails, '|#|State') - 9) = 'RI' HAVING ((eventStartDate BETWEEN '1261980000' AND '1282712400') AND (eventEndDate BETWEEN '1261980000' AND '1282712400')) ORDER BY eventStartDate ASC LIMIT 0, 20;
-
as i said, one way. this is the thing that came up in my mind. but thanks for the another solution.
-
<?php class cache { var $cache_dir = "cache/"; // Directory where the cache files will be stored var $cache_time = 7320; // How much time will keep the cache files in seconds var $caching = false; var $cache_file = ""; // Constructor of the class function cache() { $this->cache_file = $this->cache_dir . md5(urlencode($_SERVER["REQUEST_URI"])); if (file_exists($this->cache_file)) { //Grab the cache: $handle = fopen($this->cache_file, "r"); do { $data = fread($handle, 8192); if (strlen($data) == 0) { break; } echo $data; } while (true); fclose($handle); echo "<span style='font-size:8px;'>cache page loaded</span>"; exit(); } else { //create cache : $this->caching = true; ob_start(); } } // You should have this at the end of each page function close() { if ($this->caching) { // You were caching the contents so display them, and write the cache file $data = ob_get_clean(); echo $data; $fp = fopen(str_replace("/ww.", "/", $this->cache_file), 'w'); fwrite($fp, $data); fclose($fp); } } } ?>
<html> <head> </head> <body> <?php include("cache.class.php"); ?> <?php $ch = new cache(); ?> THIS CONTENT WILL BE CACHED <?php $ch->close(); ?> </body> </html>
-
for security you could use https and mysql_escape_string.
-
why not use <form>?
-
Create two buttons on the page of the form that do separate stuff?
one way is:
function dothis() { document.myform.submit(); } function dothat() { document.myform.submit(); } <input type="button" onclick="dothis()" value="Do This" /> <input type="button" onclick="dothat()" value="Do That" />
it's a start, research further.
-
or you could use image magick
-
use this
<?php header ("Content-type: image/jpeg"); /* Resize an image to 25 x 25 imgsize.php?w=25&h=25&img=path/to/image.jpg Resize an image to 50% the size imgsize.php?percent=50&img=path/to/image.jpg Resize an image to 50 pixels wide and autocompute the height imgsize.php?w=50&img=path/to/image.jpg Resize an image to 100 pixels tall and autocompute the width imgsize.php?h=100&img=path/to/image.jpg Resize to 50 pixels width OR 100 pixels tall, whichever resulting image is smaller imgsize.php?w=50&h=100&constrain=1&img=path/to/image.jpg JPEG / PNG Image Resizer Parameters (passed via URL): img = path / url of jpeg or png image file percent = if this is defined, image is resized by it's value in percent (i.e. 50 to divide by 50 percent) w = image width h = image height constrain = if this is parameter is passed and w and h are set to a size value then the size of the resulting image is constrained by whichever dimension is smaller Requires the PHP GD Extension Outputs the resulting image in JPEG Format By: Michael John G. Lopez - www.sydel.net Filename : imgsize.php */ $img = $_GET['img']; $percent = $_GET['percent']; $constrain = $_GET['constrain']; $w = $_GET['w']; $h = $_GET['h']; // get image size of img $x = @getimagesize($img); // image width $sw = $x[0]; // image height $sh = $x[1]; if ($percent > 0) { // calculate resized height and width if percent is defined $percent = $percent * 0.01; $w = $sw * $percent; $h = $sh * $percent; } else { if (isset ($w) AND !isset ($h)) { // autocompute height if only width is set $h = (100 / ($sw / $w)) * .01; $h = @round ($sh * $h); } elseif (isset ($h) AND !isset ($w)) { // autocompute width if only height is set $w = (100 / ($sh / $h)) * .01; $w = @round ($sw * $w); } elseif (isset ($h) AND isset ($w) AND isset ($constrain)) { // get the smaller resulting image dimension if both height // and width are set and $constrain is also set $hx = (100 / ($sw / $w)) * .01; $hx = @round ($sh * $hx); $wx = (100 / ($sh / $h)) * .01; $wx = @round ($sw * $wx); if ($hx < $h) { $h = (100 / ($sw / $w)) * .01; $h = @round ($sh * $h); } else { $w = (100 / ($sh / $h)) * .01; $w = @round ($sw * $w); } } } $im = @ImageCreateFromJPEG ($img) or // Read JPEG Image $im = @ImageCreateFromPNG ($img) or // or PNG Image $im = @ImageCreateFromGIF ($img) or // or GIF Image $im = false; // If image is not JPEG, PNG, or GIF if (!$im) { // We get errors from PHP's ImageCreate functions... // So let's echo back the contents of the actual image. readfile ($img); } else { // Create the resized image destination $thumb = @ImageCreateTrueColor ($w, $h); // Copy from image source, resize it, and paste to image destination @ImageCopyResampled ($thumb, $im, 0, 0, 0, 0, $w, $h, $sw, $sh); // Output resized image @ImageJPEG ($thumb); } ?>
-
this is what i'm looking for! thanks!
-
how do you compute an equation inside a variable?
$row['equation'] - from database. example content: ORIGINAL_PRICE - (ORIGINAL_PRICE * 0.50)
example
define('ORIGINAL_PRICE', 49.97); $value = $row['equation'];
is it possible?
-
the reason why my i did that code because the computation will be coming from database and i don't know how to use the computation after i get it from the database. got the idea?
-
define('ORIGINAL_PRICE', 49.97); $computation = "ORIGINAL_PRICE - (ORIGINAL_PRICE * 0.50)"; echo (int) $computation;
that code is not working but is that possible or how to code that to work?
-
i tried unzip to extract jar files and it's working. now i could extract it to a new folder.
how to convert this to jquery
in Javascript Help
Posted
how do i write this in jquery?