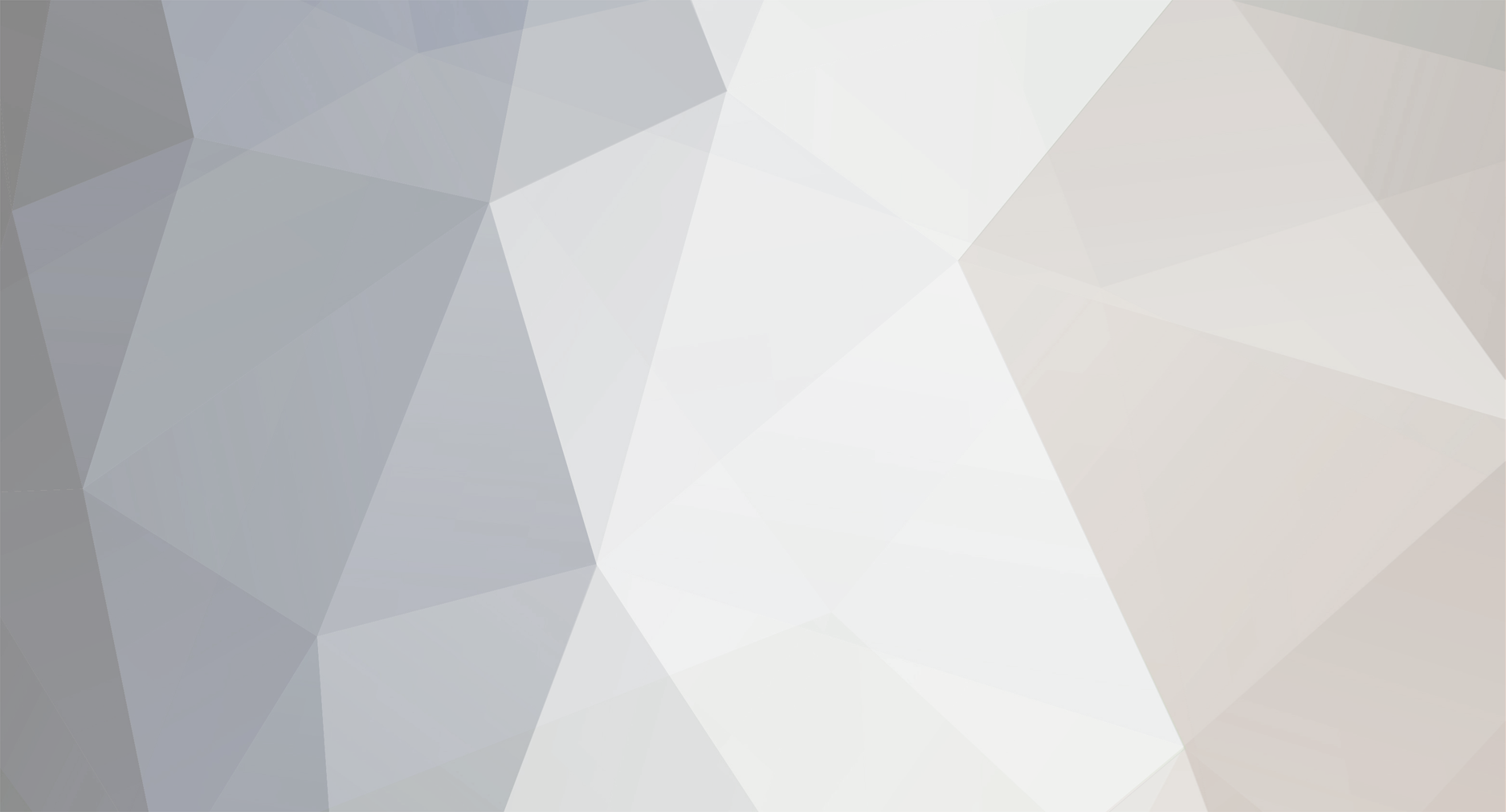
bronzemonkey
Members-
Posts
433 -
Joined
-
Last visited
Never
Everything posted by bronzemonkey
-
In the HTML forum someone asked how to remove /add the default text within form inputs onfocus / onblur. The answers provided used javascript within the html document, and since I'm trying to learn DOM scripting, I wanted to achieve the same results without mixing markup and behaviour. I've got something that works but which seems long-winded. I wanted to post it here to see if anyone could point out the mistakes I've made and how I might be able to do this with an abstract function so that it could be applied to any number of inputs within the document. This is what I've got (just c&p all the code) <!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01//EN" "http://www.w3.org/TR/html4/strict.dtd"> <html> <head> <meta http-equiv="Content-Type" content="text/html; charset=utf-8"> <title>Document</title> <script type="text/javascript"> function addLoadEvent(func) { var oldonload = window.onload; if (typeof window.onload != 'function') { window.onload = func; } else { window.onload = function() { if (oldonload) { oldonload(); } func(); } } } function inputHidePass() { if(!document.getElementById) return false; var elem = document.getElementById("pass"); if (elem.value == ("Password")) { elem.onfocus = function() { elem.value = ""; } } else if (elem.value == ("")); { elem.onblur = function() { elem.value = "Password"; } } } function inputHideUser() { if(!document.getElementById) return false; var elem = document.getElementById("email"); if (elem.value == ("Username")) { elem.onfocus = function() { elem.value = ""; } } else if (elem.value == ("")); { elem.onblur = function() { elem.value = "Username"; } } } addLoadEvent(inputHidePass); addLoadEvent(inputHideUser); </script> </head> <body> <form method="post" action="index.php"> <input type="text" id="pass" name="pass" value="Password" /> <input type="text" id="email" name="email" value="Username" /> <input type="submit" id="send" name="send" value="Login" /> </form> </body> </html> This is the type of thing I was trying to do (but was getting "elem has no properties" errors): function inputTextShowHide(target,text) { if(!document.getElementById) return false; var elem = document.getElementById(target); if (elem.value == (text)) { elem.onfocus = function() { elem.value = ""; } } else if (elem.value == ("")); { elem.onblur = function() { elem.value = text; } } } inputHideShow("pass","Password"); inputHideShow("email","Username"); addLoadEvent(inputHideShow); Any tips or feedback would be great! Thanks
-
Yes it is. Declare the element widths in terms of ems (a relative unit). Try to avoid using the pt unit (it is for print media) and absolute units in general (pt & px) altogether for font-sizes in screen media stylesheet (to allow text resizing in IE): /* this is just a very simple browser reset it is also declaring the font-size and family to be used */ html, body, div, p, blockquote, ul, ol, li, dl, dt, dd { padding: 0; border: 0; margin: 0; font-size: 0.625em; /*equivalent to ~10px in most browsers*/ font-family: Tahoma; } /* now you can declare the dimensions of the elements in terms of the font-size you'll notice that changing the font-size value above will alter the size of the elements styled below you could use ems for widths/heights/padding/borders/margins of almost every element in the document to get a fully scalable website */ #cartStatusOrange { width: 21.1em; /* 21.1 x 10px = 221px at default font-size */ height: 3.9em; /* 3.9 x 10px = 39px at default font-size */ } #cartStatusGreen { width: 21.1em; height: 3.6em; }
-
How do I have left div, right div, and a bottom div?
bronzemonkey replied to alesgirl's topic in CSS Help
I only come here to try and help people with their problems and learn from the situations they have found themselves in. I simply wanted this guy to know that in css a div and a float are displayed as a block by default. *yawn* I pointed you to Roger's example so that you could see for yourself with your own eyes, in your own copy of IE6, that you were wrong! But instead you refused to look at it and claimed that it was evidence of my stupidity. Konquerer uses the KHTML engine (which Safari is based on) so it's no different in the fact that div is displayed as a block. -
How do I have left div, right div, and a bottom div?
bronzemonkey replied to alesgirl's topic in CSS Help
Yeah, because you've made nearly 5 times as many posts. Unfortunately, your advice is not always correct. In another thread you mistakenly claimed that IE6 supports the tabular display values, suggested that Roger Johansson's clear demonstration example was incorrect, and refused to view his demonstration for yourself in IE6. It's not a competition to see who is best. Rather than invent false information, just accept your errors, there is nothing wrong with that...everyone here has, at some point, posted something that was not correct. -
How do I have left div, right div, and a bottom div?
bronzemonkey replied to alesgirl's topic in CSS Help
obviously you're not thinking -
How do I have left div, right div, and a bottom div?
bronzemonkey replied to alesgirl's topic in CSS Help
Absolute positioning is extremely useful and an essential tool for anyone creating websites. It is of limited use in creating general layouts but it is not complicated...you simply have to understand situations in which it can provide you with the best solution. Definitely read about how to use absolute positioning. A common mistake is not to set {position:relative} to the element that you wish your absolutely positioned element to be positioned relative to. There is no need to display them as blocks because the default display value for divs is block, and the default display value for floated elements is also block. -
How do I have left div, right div, and a bottom div?
bronzemonkey replied to alesgirl's topic in CSS Help
Don't use absolute positioning. What that does is remove the element from the document flow, and so your footer is going to behave as if those absolutely positioned elements aren't even there. Instead use floats and clearing...something like this: /* css code */ #primary-content, #secondary-content { float:left; } #footer { clear:both; } <!-- html code --> <div id="container"> <div id="header"> </div> <div id="content"> <div id="primary-content"> </div> <div id="secondary-content"> </div> </div> <div id="footer"> </div> -
I think that's how almost everyone does things! Certainly is for me. Nothing wrong with that approach as long as it always includes the "later learning how it works and what my mistakes were" part.
-
Why do that when a lot of people use browsing equipment that allows them to access a fuller experience? The point is that your website should gracefully degrade, make sense without style or presentational elements, and be accessible to people who are blind, can't use a mouse, disable images, etc. Perhaps you should read about web accessibility and the W3C Web Accessibility Initiative - http://www.w3.org/WAI/ - rather than making facetious comments.
-
I can't replicate your problems in IE6 so I can't suggest any solutions other than those I already made (like using an image). Whenever you have a problem it is a good habit to check your html/css for errors first. Why would I recommend something if I do it another way? I have yet to find a situation where I needed to use an inline style, and I'm not bothered what you believe because I'm only wasting my time here to help. You're using inline styles in places where simply adding declarations to the stylesheet would be more efficient, give you greater flexibility, and helping you to avoid digging around in html files when all you want to do is alter the presentation. If you want to keep using inline styles, be my guest, I'm not here to beat people over the head. It is the most widely used method of achieving what you are trying to achieve. Surely spending 5 minutes trying it out is worthy of your time? Using an empty div to display a background image, using divs with classes like "sidemenu_header" or "productTitle" to display headings...that is what I'm talking about. A heading should be wrapped in a heading tag, an element should not be empty. It's not going to solve your current problem but it will help you to create cleaner, more semantic, html documents that will prove easier to style and debug. Anyway, I helped you fix your other problems but perhaps someone else will help you with this one. Good luck.
-
Why have styles disabled? Why have images disabled? Why have javascript disabled? Why use a screen reader? It doesn't matter what you think or if you can't understand why these situations would arise, it matters how different people choose (or have) to browse the internet. The internet isn't about catering to the majority and ignoring the numerous other minorities, it is about inclusiveness - both for moral and business reasons. Using purely presentational images in your markup in order to produce rounded corners is not only unsemantic but unnecessary. It can be done better without compromising.
-
It really depends on your requirements: No images? Liquid box? Fixed box? Needs to be rounded even for users with javascript disabled? Every situation requires different solutions and varying amounts of superfluous markup. The worst of all is to use images within the markup. Disable styles on the pages linked in the first reply and you'll see just one reason why.
-
I just refreshed your page 25 times in IE6 and the header images show up every time. Perhaps you have already fixed the problem? But looking at your code there are a few things you should consider: - Put your code through a validator and fix the errors that are getting flagged (some are very simple ampersand errors). Whenever something goes wrong check that it isn't a little coding error first. - Eventually you should get rid of the inline styling and remove the use of print media pt units in a stylesheet for screen media. - It is not semantic or necessary to be using an empty div to contain a background image. Either use an actual image (and store the path, alt text, dimensions in your php array) or apply the background image to an existing "header" div that contains your top level navigation. You can get php to assign different classes to the header div that correspond to different background images. - You don't need to be using {vertical-align:middle;} on a div element and I also never put ' ' around the paths to the background images in my css. It doesn't seem to be invalid but it also might cause problems for some browsers? Things will probably get easier with time as you get used to ditching some of the "table layout" coding concepts (and to be fair everything that has gone wrong for you has been in IE6...no surprise!). Anyway, I had no problems looking at your site headers with IE6!
-
I see the gifs, there just seems to be something wrong with the positioning because the green section of the "live" tab is poking out above the rest of the list. There is also no "live" state on the home, aboutus, projects, or contactus links. Why do you need the png/gif alternatives if you aren't using the alpha channel in the pngs? Don't have the time to wade around in your code but there doesn't appear to be a need for any IE6-specific trickery for the type of menu you are using. I'd also recommend: - Don't use the * reset because it is heavy going for the user agent. Use a reset that targets specific elements. Replace * {margin:0; border:0;} with Eric Meyer's CSS reset (or a suitable adaptation of it). - Don't use xhtml 1.1 served as text/html. There is no reason to do so. The W3C specifically say that xhtml 1.1 should not be served as text/html...even the people who promote the use of xhtml 1.0 won't use xhtml 1.1 for text/html sites. At worst, use xhtml 1.0 strict, but unless you need the xhtml doctype (for a CMS or javascript) try to use the html 4.01 strict doctype.
-
In Firefox, Opera, Safari, IE7, etc., use {min-height: 600px;} In IE5 and IE6 use {height: 600px;}
-
Not really...unless you don't know what you're doing. But then using css will cause more problems that it solves if you don't know how to use it.
-
Sorry, you're totally right. Been coding tables all week, where the abbr attribute can be used in the td or th element, but you can use the abbr element instead: <span><abbr title="Extra Large">XL</abbr></span> This will let people understand what XL is an abbreviation of (if they put the cursor over it) and will help people using screen-readers. It's about improving accessibility - http://www.maxdesign.com.au/presentation/abbreviations/
-
Sounds like default browser styles. Each browser has its own defaults that is applies to element but you can reset them all at the very beginning of a document: html, body, div, p, span, table, ol, ul, li, etc, etc { margin:0; padding:0; border:0; }
-
Don't use a top-margin, it won't even work properly for inline elements. Firebug will show you what they are doing in 5 seconds: - Using align="left" in the image markup - Using {line-height:24px} in the css targeting the anchor Rather than using the depreciated align attribute, just use {float:left;} in the css targeting the image. Two lines of code and you're done. There is no need to be using tables. Use an unordered list instead. If you want to use text then just wrap it in a span element and remember to use the abbr attribute: <span abbr="Extra Large">XL</span>
-
Your link takes me to a page that the validator does not recognise. Your url is wrong. Just go through the validator's error messages one by one and fix the problems. For starters, tfoot should come directly after thead and not after tbody. The transitional doctypes are the most forgiving, so if you're getting hundreds of errors using that, then you are doing something very wrong in your coding.
-
When the contents of an XHTML document are served as XML then it becomes extremely unforgiving. The slightest parse error will result in the whole page not being served. You can serve the contents of an XHTML 1.0 doc as text/html but it should also work when served as xml...but most websites using xhtml doctypes will simply die when served as XML. There is really no point using xhtml when html 4.01 strict will do exactly the same job and not kill your page if you have a small coding error, or some user-generated content that is not 100% valid, or an external link that your CMS didn't fix the ampersands in, etc. But since you are using an xhtml doctype to take advantage of xml you are going to be limited in what browsers will display your pages becauase... IE won't accept an XHTML document being served as "application/xhtml-xml". It's that simple. The W3C says it's ok, with conditions. The whole point is that the contents of an XHTML 1.0 doc can be served as text/html but should also work when served as application/xhtml-xml! The w3.org website has an xml declaration. The w3.org are not infallible either. Some of their definitions in their documentation are simply wrong - famously the distinction between an acronym and an abbreviation. Given the current situation with html5 and xhtml2, I doubt their next site build will be using xhtml, and it certainly wouldn't be done by serving xhtml 1.1 as text/html. The only time I still use xhtml doctypes served as html/text is for professional work, because clients look for agencies that use xhtml, some javascripts that they want to use were built for xhtml doctypes (who knows why), and a lot of CMS now pump out xhtml tags! The reality is that html 5, not any form of xhtml, is going to be the next standard.
-
By the way, your boxes don't resize to fit the content if the text-size is increased...are you using a fixed height for your rounded boxes? Since you're using a fixed width box, there is no point using images for each individual corner, you may as well just use an image on the top and one of the bottom...it will also reduce the amount of unsemantic markup because you can use a background image on the whiteblock itself. You can also get away with using only one image. Imagine this is your top image (the two top corners): _______ / \ And this your bottom image: \_______/ Combine them both into a single image arranged like this: \_______/ _______ / \ And then use background image positioning. You'll notice that the bottom corners are hidden when the image is used for the top corners, and that the top corners are hidden when the image is used for the bottom corners. The same idea can be used for liquid-box rounded corners or complex image borders.
-
Your javascript is probably where the error lies if you're getting problems when using a proper doctype. Perhaps the JS forum is where to look for some help modifying your code?
-
Really, it wasn't a half bad attempt, and it would have worked had it not had a second drop-down level that seemed to trigger some bizarre IE7 behaviour. How are you still stuck? The code I posted should fix the problems. Using Javascript means that people have to have js enabled to use the menu. However, you do realise that the menu won't work in IE6 right?
-
I've found a solution for you but it was pretty heavy going wading through your css file. It's packed with redundant or unnecessary code as well as a couple of parse errors. I'd also recommend that you shorten your list class names to something more practical and meaningful (e.g., nav, sub1, sub2 rather than navigation-1, navigation-2, navigation-3) just to make your life easier. Rather than using {display:none} just position the drop lists offscreen. I also removed some useless padding. ul#navigation-1 li ul.navigation-2 {margin:0; padding:16px 0 0; list-style:none; width:100px; position:absolute; top:21px; left:-9000px; border:0px solid #b9121b; border-top:none;} ul#navigation-1 li:hover ul.navigation-2 {left:0;} ul#navigation-1 li ul.navigation-2 li ul.navigation-3 {margin:0; padding:0; list-style:none; position:absolute; left:-9000px; top:0; z-index:900;} ul#navigation-1 li ul.navigation-2 li:hover ul.navigation-3 {left:100%;} There seems to be an IE7 bug lying in there, even after the whole thing is cleaned up, to do with the display:none/block switch. Once you've revealed the second sub list the backgrounds of the links in the second sub list will show up when the first sub list is revealed again. It can be avoided either by simply positioning the lists offscreen rather than using display:none, or by adding another set of declarations for the links of the second sub list so that they are also {display:none} and then switched to {display:block;} when the second sub list is revealed. There might a reason for this bug but I couldn't see it immediately.