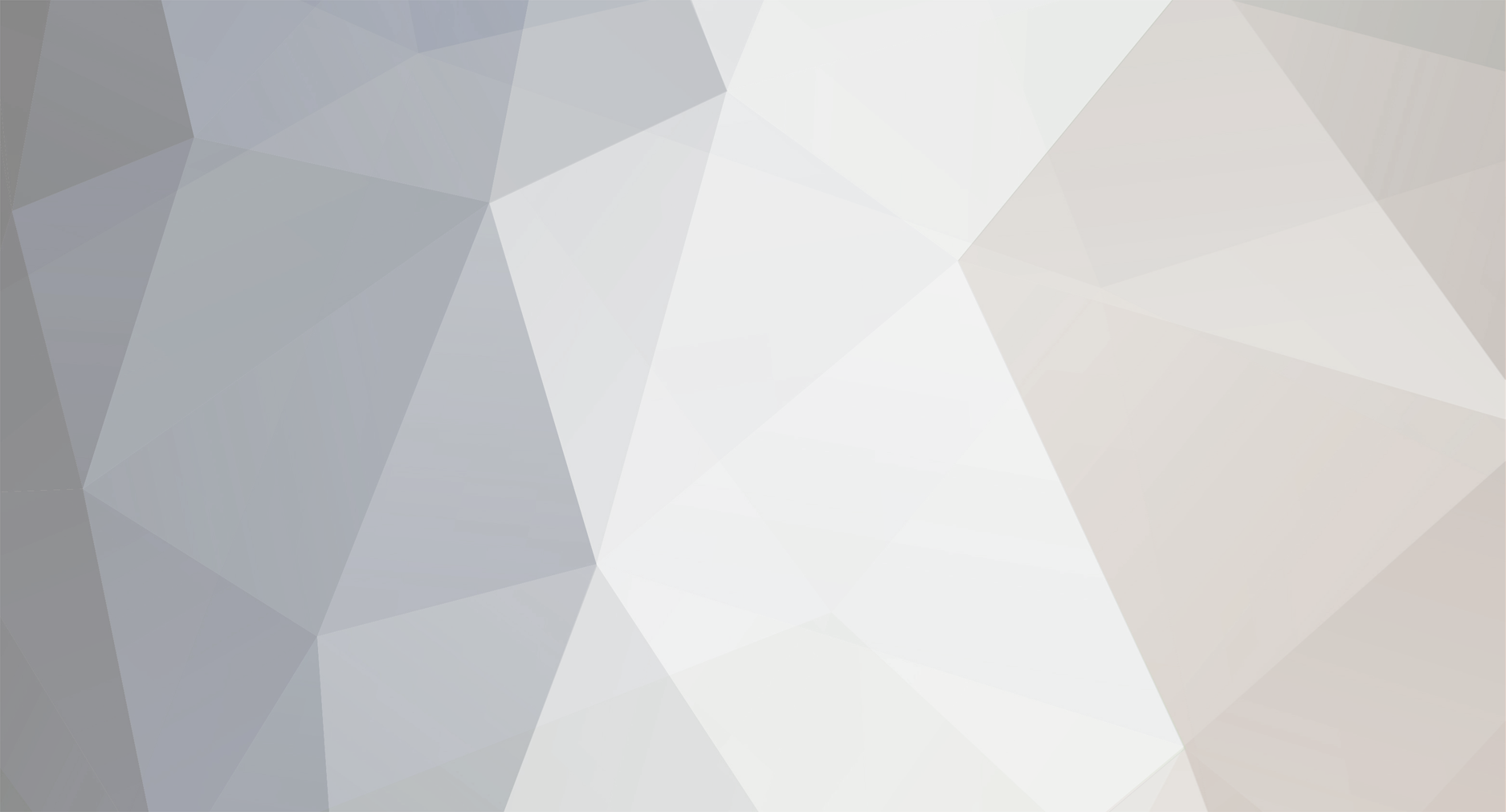
Fadion
-
Posts
1,710 -
Joined
-
Last visited
Posts posted by Fadion
-
-
Although this wont really help you, my personal belief is NEVER pass anything in the URL....never. When i can i pass things to sessions or if i have to POSTS.
That makes no sense. Never pass a url variable? Great, PHP (and all the other web scripting languages) should abandon GET support then...
As for the problem. Your url should be example.com?smth=3, or maybe you've mod_rewrite your urls. You can solve all this with a session. When someone enters the confirmation page, create a session for them. While they go in the reward page, check if the session is set and destroy it (so they don't re enter again without going to the confirmation page first). It should be easy, but if you need sample code, just ask.
-
Ok as you get the data from a database, i'm going for a full example:
<?php $results = mysql_query("SELECT username FROM users"); while($values = mysql_fetch_array($results)){ $user = preg_replace('/[0-9]/', '', $values['user']); echo $user; } ?>
-
Sure
. It is just replacing every number in the string with nothing. The regular expression is doing that, searching and replacing every number ([0-9]), leaving just the text.
-
I'm a total noob in regular expressions, but this should do it:
<?php $str = 'lynnie20080825122012'; $str = preg_replace('/[0-9]/', '', $str); echo $str; ?>
-
You should check first if the form is submitted, as the query will run every time the page is accessed, not every time the form is submitted. Change the "Writing to the database part" to:
<?php if(isset($_POST['txt_username'])){ $username = mysql_real_escape_string(htmlentities($_POST["txt_username"])); //clean the data $password = $_POST["txt_password"]; $query1 = "INSERT INTO login (username, password) VALUES ('$username', '$password')"; mysql_query($query1, $connection) or die(mysql_error()); } ?>
The password should be hashed so that you don't run the risk of attackers getting your users passwords.
-
What does the layout_text() function do and are you including the language file?
-
It should work with an absolute path, so that's strange. Even though, you should use relative paths, based to the php file that the script runs.
-
If you have written that code by yourself, you should know where to place it. Otherwise, i'd suggest to start writing some code from scratch as it's the only way to learn. I don't know what the GetSQLValueString() custom function does and your code has too many not needed lines imo, so i'll just suggest the normal way of doing it:
<?php if(isset($_POST['submit'])){ $date = $_POST['date']; list($month, $day, $year) = explode('-', $date); if(!strtotime($date)){ echo 'The date format is invalid. It should be: mm-dd-yyyy'; } elseif(!checkdate($month, $day, $year)){ echo 'The date is invalid. Either the month, day or year are of a not normal range.'; } } ?>
-
What is the PHP code you're using? You can post here the part where you make the query and fetch the data.
-
I just realized that i made a stupid mistake in my previous post, used UPDATE instead of INSERT :-\
<?php mysql_query("INSERT table (col1, col2) VALUES ('value1', 'value2')"); ?>
Just insert the row, it will be automatically added. Your query may be something like, instead of the WHERE part:
mysql_query("INSERT INTO Ysers (Comments, email) VALUES ('$CF', '$Friend')");
-
Give this a go:
SELECT cat.cat_id, cat.cat_title, for.forum_id, for.cat_id, for.forum_title FROM categories AS cat INNER JOIN forums AS for ON cat.id=for.cat_id ORDER BY cat.cat_title
-
I can't seem to find the date variable there, but anyway the approach to this can be using:
<?php $date = '09-03-2008'; list($month, $day, $year) = explode('-', $date); if(!checkdate($month, $day, $year)){ echo 'The date is invalid'; } ?>
It will check if the date is a normal one and not something like "45-78-99999". But it won't check for the date format, instead you can add such a thing:
<?php $date = '09-03-2008'; if(!strtotime($date)){ echo 'The date is invalid'; } ?>
-
Try this:
<?php $url = "/kunden/homepages/12/d214897219/htdocs/rubberduckiee/rubberduckiee/www.adamplowman.co.uk/random"; $url = substr($url, 0, strrpos($url, '/') + 1); $foldername = 'somefolder'; echo $url . $foldername; ?>
-
First of all congrats. You've created a really nice design. Overall I like it, being the design and the way you handled content. But critiques are always welcome, right?
Main Page
- I like the handwritten "i was inspired into...", but the next "get your football fix" doesn't look quite good. Also some letters like the "g" and "y" are cut out.
- The "ONYAHEAD.com" text is nice, but a bit more work on it could make it stand out more. Some details on the letters outside (cut them out a bit) should look nice.
- The three columns are a bit stuck to each other and make them difficult to read. In IE7 they look better, but in FF3 not. Also some padding could go well.
- The links that trigger the slide-in animation could be toggle links. Meaning that they open and hide the content, while you can leave the hide link at the end. I was a bit confused in the beginning as the content was long and i couldn't see the [hide] link, but clicking on the links at the beginning triggered the slide-in again.
Sub Pages
- Not much to say here, except that the Quick Links at the bottom should be more visible
-
You mean that script will cheat or hack my point system?
That shouldn't be a surprise, shouldn't it? It's not difficult to make a script which runs through the "browsers" and lower their points. Even if someone fails on an automated approach, people are so patient that they can click and refresh manually.
If I have users paid to keep the site on my site, it turns into no sense. Actually, if users want to keep the site there, they must click on other sites. The more sites they click, the more points they gain. A site has zero point will be out of the list. Earning money? If there are many users, I can put somes little ads to earn.
I was just saying, not making it happen and i stated that it would not increase any earnings. Though, the way the site is now, makes it not really interesting. In my opinion...
-
This thing looks really cool. I'm liking a lot the minimal layout, basically i'm doing what i'm supposed to do...browse. And yep the Element Inspector is a basic version of firebug, but considering this is an early beta, maybe we'll see some some cool stuff in the future. I'm impressed in general, fast and with style.
-
My bad, i did a variable mistake. Try this:
<?php echo "<ol>"; foreach(glob("*.php") as $val){ $file = basename($val, '.php'); echo "<li>$file</li>"; } echo "</ol>"; ?>
-
Try this:
<?php echo "<ol>"; foreach(glob("*.php") as $val){ $file = basename($file, '.php'); echo "<li>$file</li>"; } echo "</ol>"; ?>
-
Hmm. Have the circles a different color then the background? Have you checked that Opacity and Fill Opacity are 100%? If by clicking the shape mask (the black box) you can see the actual shape, it means that the circle is created but its either transparent or has the same color as the background.
-
There are this lines in php.ini
[mail function] SMTP = localhost smtp_port = 25
Add there the smtp of a remote server (ie. gmail). That's for windows only, but after those lines (in php.ini) are the lines for unix.
-
Hello for some reason my post script is not updating the database properly,
it doesn't stack it just overwrites.
I mean that it just overwrites the column instead of keeping previous posts.
All these point in the direction that you should use INSERT instead of UPDATE. INSERT [as the name proposes] adds new records to the database, while UPDATE edits existing ones.
<?php mysql_query("UPDATE table (col1, col2) VALUES ('value1', 'value2')"); ?>
-
valtido you say "lol" to much. there is no need for it, its annoying.
I noticed that too (everybody must have noticed it). You can count 4-5 lols in just one of his post and doing a forum search for "lol" will bring up only valtido's posts
-
I'm not very sure as i'm not a MySQL expert, but from what I know, foreign keys are supported only by the InnoDB storage engine. The syntax should be:
INDEX (user_id, id), FOREIGN KEY (user_id) REFERENCES user_join(id) ON UPDATE CASCADE ON DELETE CASCADE
As you can see, i assigned a user_id field as the foreign key because a foreign key must reference a primary key of the parent table. The "on update cascade" and "on delete cascade" make it so that when a row is deleted in the parent table, every row that references it in the child table is deleted or updated too. Take a look here and here for some detailed information.
-
PS: Hope this becomes a sticky thread as it may help a lot of php developers needing quick references.
That's kind of you, but I doubt there is someone left who hasn't used the manual. Anyway, the manual index is the best way to start learning by topics.
How to extract only first alphabetical characters from a string
in PHP Coding Help
Posted
Glad to hear that. Please mark the topic as solved if you don't have anymore questions.