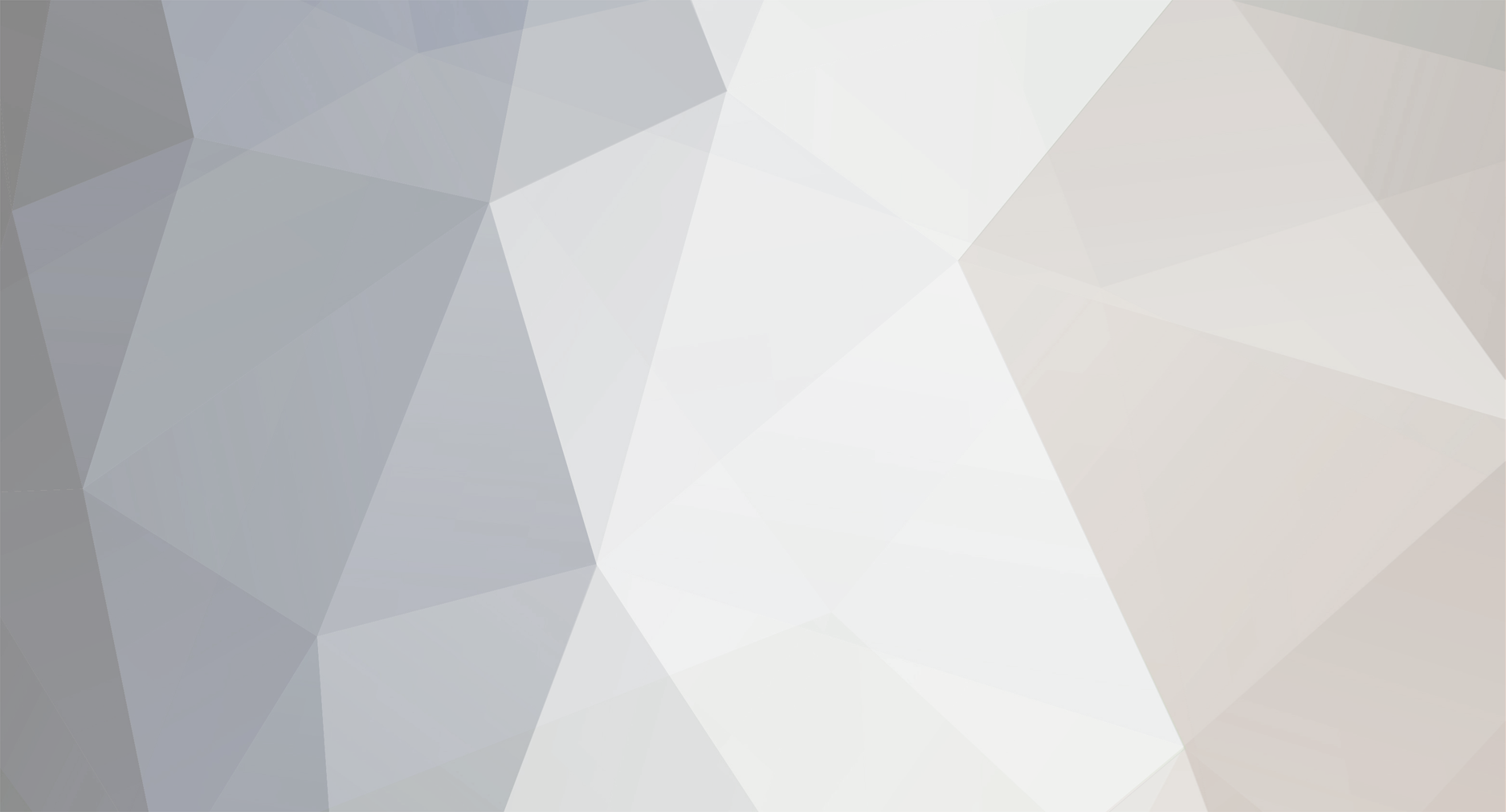
Fadion
-
Posts
1,710 -
Joined
-
Last visited
Posts posted by Fadion
-
-
You have error_reporting set to E_ALL (default is: E_ALL & ~E_NOTICE). While it is a good idea to show them in a development environment, it's not a good one for a production server. Take a look at this section in the PHP Manual.
-
There would be 2 methods to break the output into 4 columns.
The first, but not the good one:
<?php $counter = 1; while ($file = readdir($dir_handle) { if ($counter % 4 == 0) { echo '<br />'; } if ($file != '.' or $file != '..' or $file != 'default.php') { echo "<a href=\"images/default/$file\" class=\"thickbox\"><img src=\"images/default/$file\" height=\"150\" width=\"150\" border=\"0\"></a>"; } $counter++; } ?>
Basically it will break the line every 4 images, but there is no way you can control how it looks, how far they're from each other, etc. That brings us method 2.
The second method and recommended one can be achieved just by using CSS. You need to wrap your images in a div, float them and set the width to 1/4 of your container.
The PHP code could look like this (I omitted part of it):
<?php echo '<div id="container">'; while ($file = readdir($dir_handle) { if ($file != '.' or $file != '..' or $file != 'default.php') { echo '<div class="image">'; echo "<a href=\"images/default/$file\" class=\"thickbox\"><img src=\"images/default/$file\" height=\"150\" width=\"150\" border=\"0\"></a>"; echo '</div>'; } } echo '</div>'; ?>
While the CSS code could look like this:
#container { width:800px; overflow:hidden; } div.image { width:190px; margin:0 10px 10px 0; /* top right bottom left */ float:left; }
The width of the container should be your page's width or can be omitted if there is any higher container with a specified width, which will be inherited. While the images div have a width of 190px that means: 800px (container) / 4 columns = 200px - 10px right margin = 190px. Inside that div you can add any other information you want and use once again CSS to style it.
Don't know your level on CSS, but this is pretty basic stuff. Hope you get it.
-
While sprintf() will work perfectly as ProjectFear suggested, there is one problem with it: type specifiers are meaningless to someone not related to programming. If your projects involves more than just you or you want to have variables with meaning inside languages strings, than you could go for another way. Definitely, sprintf() will work faster, so be advised.
The "system" I just wrote involves a function that searches and replaces special variables inside language strings and replaces them with the appropriate value. It looks like this:
<?php function replaceVars ($text, $vars) { if (!is_array($vars)) { $vars = array($vars); } if (preg_match_all('|{(.+?)}|', $text, $matches)) { foreach ($matches[0] as $key=>$match) { $text = str_replace($match, $vars[$key], $text); } return $text; } } ?>
What it does is take 2 parameters: the language string and the variables to be replaced (as string or array). The string is matched against a regular expression, looped through all the matches and replace each one with the supplied variables. The replacement will be incremental, meaning that the first matched occurrence will be replaced with the first element of the variables array.
Usage examples:
<?php //First example: 1 variable to replace $lang['msgs'] = 'you have {num_msg} messages'; echo replaceVars($lang['msgs'], 10); //will output: you have 10 messages //Second example: 2 variables to replace $lang['msgs'] = 'you have {num_msg} messages in your {folder_name} folder'; echo replaceVars($lang['msgs'], array(10, 'Inbox')); //will output: you have 10 messages in your Inbox folder ?>
While it certainly has it's overhead, I guess it's better giving names to your variables for the translators sake than just %d or %s.
-
Strange, but I'll check it out. Thanks.
-
@gizmola. Thnx, glad u like it. Strange it's slow on your site, as the server running the site is quite a machine (2x quad core, 24GB RAM). Even if it's hosted in Europe, latency shouldn't be a problem. Anyway, I'll ask the client to investigate it. As for hiding the controllers, you mean making a url like: /plans/web-hosting/ to just /web-hosting/? That would be difficult as I wouldn't know what the controller is
@ProjectFear. You're right on the social media icons and login button. A bit lazy on my part, but will fix them asap. As for the news page, it's supposed to smooth scroll on a news item (when clicked). A simple anchor system that uses hashes (/news/#news-item). Not sure what happened at your side, but if it didn't scroll to a news item, I'll have to check my Javascript.
Thanks for the input guys.
-
Hi guys.
Just a few days ago I finished a hosting website for a client, which is actually still in a "beta" phase. We're still testing the site for any bugs and problems, but it looks and functions the way it should. It has a custom CMS powering the articles, hosting packets, knowledge base, etc. Also, I did a lot of design work on the WHMCS part to fully integrate it with the new design.
Critiques and suggestions are highly welcomed.
The site is: http://www.thehosting.me/
-
Sorry man, I haven't been here in a while and just now read your posts. Happy you got it fixed; the site looks a lot better now
. Anyway, I would make just a few small changes that won't require more than a few CSS lines.
- Make the background full screen and lighter. I'd go for a #ddd as the bottom point of the gradient and make background-color:#ddd too, because white it looks off.
- Add some horizontal padding to the login block. Something like: padding:0 10px;
- Add some margin-top to the ad block under the menu. Appart from looking better, I'm sure it will help with a higher CTR as it will look more like a sub-menu.
- Remove the left-padding/margin from the H3 title in the upload boxes as it looks off. Also add some margin-bottom to separate the title from the rest.
Appart from that, everything looks cool. Good job and thank you for the link
- Make the background full screen and lighter. I'd go for a #ddd as the bottom point of the gradient and make background-color:#ddd too, because white it looks off.
-
That would quite more complicated and really slow, but it's easily accomplished. However, without giving us a few event lines, we can't give a proper working solution.
PS: You should really opt for a database approach. It will be 100x easier to handle (inserts, updates, whatever).
-
conan318, you should really read what others have written first. Beginner or not, one should have the patience to read!
The show and reply functionality should work like this:
show.php
<?php session_start(); $myusername = $_SESSION['myusername']; require 'database.php'; $message_id = mysql_real_escape_string($_GET['messageid']); //or (int) $_GET['messageid']; if messageid is supposed to be an integer $results = mysql_query("SELECT message_title, from_user, to_user, message_contents FROM messages WHERE message_id='$message_id'"); $values = mysql_fetch_assoc($results); $message_title = stripslashes($values['message_title']); $message_contents = stripslashes($values['message_contents']); $from_user = $values['from_user']; $to_user = $values['to_user']; //this should be equal to $myusername, or it's ID if it is an ID echo "<h1>Title: $message_title</h1><br><br>"; echo "<h3>From: $from_user<br><br></h3>"; echo "<h3>Message: <br />$message_contents</h3>"; echo '<a href="inbox.php">Back to Inbox</a>'; echo '<a href="reply.php?messageid=' . $message_id . '">Reply</a>; ?>
In the same fashion, you make reply.php
<?php session_start(); $myusername = $_SESSION['myusername']; require 'database.php'; $message_id = mysql_real_escape_string($_GET['messageid']); //or (int) $_GET['messageid']; if messageid is supposed to be an integer $results = mysql_query("SELECT message_title, from_user, to_user, message_contents FROM messages WHERE message_id='$message_id'"); $values = mysql_fetch_assoc($results); $message_title = stripslashes($values['message_title']); $message_contents = stripslashes($values['message_contents']); $to_user = $values['from_user']; //this has become the TO: $from_user = $values['to_user']; //this has become the FROM: $message_contents = "\nOriginal Message:\n" . $message_contents; echo '<form method="post" action="">'; echo '<input type="text" name="title" value="RE: ' . $message_title . '" />'; echo '<textarea name="contents">' . $message_contents . '</textarea>'; echo '</form>'; echo '<a href="inbox.php">Back to Inbox</a>'; if (isset($_POST['title'])) { $title = htmlentities($_POST['title'], ENT_QUOTES); $contents = htmlentities($_POST['contents'], ENT_QUOTES); $results = mysql_query("INSERT INTO messages (message_title, message_contents, from_user, to_user) VALUES ('$title', '$contents', '$from_user', '$to_user')"); echo 'Message reply was sent successfuly!'; } ?>
It may not be a copy/paste solution, but that's the basic idea.
-
When an error occurs at a specific line, you should tell us what that line is. Otherwise it's just guessing.
Anyway, when using POST data in a query, the first thing to do would be sanitizing the input. Appart from that, you are writing array indexes wrong.
//it's NOT $_POST[input1]; //it IS (notice the single slashes) $_POST['input1'];
You can make this modifications to your UPDATE query.
$sno = mysql_real_escape_string($_POST['sno']); $input1 = htmlentities($_POST['input1'], ENT_QUOTES); $input2 = htmlentities($_POST['input2'], ENT_QUOTES); //and so on for every input $u = "UPDATE student SET cname='$input1', sname='$input2' WHERE id = $sno"; //add the other inputs to the update
As we are here, you can't user header() when headers have already been sent. There's a sticky in this forum that explains it.
-
mysql_query() returns a resource identifier, not an actual value. As you've done in the first query, you need to convert that resource into an array with mysql_fetch_assoc().
$getuname = mysql_query("SELECT from_user FROM messages Where message_id='$message_id' AND to_user ='$myusername'"); $uname_values = mysql_fetch_assoc($getuname); $uname = $uname_values['from_user'];
Fixed that problem, your system doesn't seem to look right. If you have a message_id, there's no need to query for the username also. The query with a message ID should return the sender (to_user), receiver (actual user) and message. After that, you just swap places (to_user=>original sender, from_user=>original receiver) and quote the original message.
-
I just assumed that, but you can easily use the search query in the url. The following form adds a <label> and replaces <input type="submit"> with a <button>. The later is a more flexible form element that doesn't show up in the url (&search=Search) after a get submit.
<form method="get" action="search_results2.php"> <p> <label for="search">Search</label> <input type="text" name="search" id="search" /> </p> <button type="submit">Search</button> </form>
search_results2.php
if (isset($_GET['search'])) { $search = mysql_real_escape_string($_GET['search']; $results = mysql_query("SELECT id, MovieName, Description, Genre, Rating FROM movie WHERE MovieName='%$search% LIMIT 1'"); $values = mysql_fetch_assoc($results); $movie_id = $values['id']; echo '<h1>Title: ' . $values['MovieName'] . '</h1>'; echo '<p>Genre: ' . $values['Genre'] . '</p>'; echo '<p>Description: ' . $values['Description'] . '</p>'; echo '<p>Rating: ' . $values['Rating'] . '</p>'; $results = mysql_query("SELECT FirstName, Review FROM reviews WHERE ReviewID=$movie_id"); while ($values = mysql_fetch_assoc($results)) { echo $values['FirstName'] . '<br />' . $values['Review'] . '<br /><br />'; } } else { echo 'Please make a search first.'; }
The code above should theoretically work copy/paste, but it has 2 problems.
1) It shows only 1 result (hence the LIMIT 1 in the first query). If there will be a partial search with more than 1 result, it doesn't care. That's why it's a good option to make a search result page that just shows matched movie titles and when a user clicks a movie, finally it shows full movie info and reviews.
2) The search isn't really state-of-the-art. As I said before, FULLTEXT searching will provide a way better search functionality and I really suggest it. Just to give you the idea, in your case you would have a query like this:
SELECT id, MATCH(MovieName) AGAINST ('$search' IN BOOLEAN MODE) AS score FROM movie WHERE MATCH(MovieName) AGAINST ('$search' IN BOOLEAN MODE) ORDER BY score DESC
MovieName should be altered to be a FULLTEXT index and you're ready to go
Anyway, as you're a beginner learning his way into PHP and MySQL, I suggest you finish up the script as you've already started it. After that, return to it with a new perspective and try to write it better.
-
The following queries will get only the rows with the date greater than the moment it's executed.
NOW() -> datetime (2011-05-02 12:06:10)
SELECT col1, col2 FROM table WHERE date>=NOW()
CURDATE() -> date (2011-05-02)
SELECT col1, col2 FROM table WHERE date>=CURDATE()
-
You had a missing curly brace before the else. Try the code exactly as I posted and than make further adjustments.
-
You mean that they fill in their name in MainPage.php and it shows up on Answers.php? Whatever that case would be, there's no permanent storage unless you go for a database approach. The options you have, appart a database, are sessions and cookies.
Answers.php
<?php session_start(); //initializes sessions and should be place at the top of the document if (isset($_POST['name'])) { $_SESSION['name'] = htmlentities($_POST['name']); echo $_SESSION['name']; } ?>
Once $_SESSION['name'] has been set, it can be called from any page you like, be it Answers.php, Questions.php or even Ducks.php
-
The code with the error and a few minor problems fixed.
<?php if ($_SESSION['username']){ $query = mysql_query("SELECT id, rating FROM locations WHERE name = '$location'"); while($row = mysql_fetch_array($query)) { $rating = (int) $row['rating']; ?> <div class="floatleft"> <div id="rating_<?php echo $row['id']; ?>"> <span class="star_1"><img src="fivestars/star_blank.png" alt="" <?php if($rating > 0) { echo"class='hover'"; } ?> /></span> <span class="star_2"><img src="fivestars/star_blank.png" alt="" <?php if($rating > 1.5) { echo"class='hover'"; } ?> /></span> <span class="star_3"><img src="fivestars/star_blank.png" alt="" <?php if($rating > 2.5) { echo"class='hover'"; } ?> /></span> <span class="star_4"><img src="fivestars/star_blank.png" alt="" <?php if($rating > 3.5) { echo"class='hover'"; } ?> /></span> <span class="star_5"><img src="fivestars/star_blank.png" alt="" <?php if($rating > 4.5) { echo"class='hover'"; } ?> /></span> </div> </div> <div class="star_rating"> (Rated <strong><?php echo $rating; ?></strong> Stars) </div> <div class="clearleft"> </div> <?php } } else { echo "Log in to review"; } ?>
-
Pages stored in a database? Usually specific data are stored in a database, each one with it's own, unique ID. That "id" url variable can be retrieved with $_GET and be used to fetch the correct row from the database. Simple enough, at least from what your request looks like.
PS: eval() should be renamed to evil(). As Rasmus Lerdorf [is thought] to say, "If eval() is the answer, you're almost certainly asking the wrong question.".
-
I got your idea and that's what the code I posted in my previous post does. It shows the movie's information and it's reviews. If you search another movie, it will only show it's reviews. As I have no idea how behaviors in Dreamweaver work (I use DW myself, but just for raw editing). The only thing I know is that they're nasty and you should avoid them. Coding things totally yourself isn't hard as long as you get away with the initial learning curve. It will pay a lot, trust me.
Probably if you post the code you have, we can help better.
-
If you're less than 80 years old, there's no excuse to open a thread, forget it and after a few days open an identical one. Anyways, your approach is quite nonsense. If more then two if() run successfully, post the regular if()? Who's the regular if()?
-
We are touching it a bit superficially, but I'll do my best to guess what you mean
As for the search, you probably are using "WHERE movie_title LIKE '%$search%'" or even "WHERE movie_title='$search'". That's why I suggested FULLTEXT, as it will improve a lot your search queries. The first result on google is this article from Zend (back in 2002, but things haven't changed much) that has some good examples on FULLTEXT searching with PHP and MySQL. The boolean mode and order by "score" are things that will give your search results some weight.
As for the logic! I guess you have tables like this:
table "movies"
-----------------
id | title | description | genre
table "comments" (aka reviews, right?)
----------------------
id | movie_id | name | comment
When a movie is searched and found, you redirect the visitor to that movie's page, right? Something like movie.php?id=10. As you have the movie id (10 in that case), appart from the movie's information, you can also show comments made for that movie. So, basically:
<?php $id = (int) $_GET['id']; $results = mysql_query("SELECT title, description, genre FROM movies WHERE id=$id"); $values = mysql_fetch_assoc($results); echo '<h1>Title: ' . $values['title'] . '</h1>'; echo '<p>Genre: ' . $values['genre'] . '</p>'; echo '<p>Description: ' . $values['description'] . '</p>'; $results = mysql_query("SELECT name, comment FROM comments WHERE movie_id=$id"); while ($values = mysql_fetch_assoc($results)) { echo $values['name'] . '<br />' . $values['comment'] . '<br /><br />'; } ?>
Above is just a very simple example of a real world scenario, but with no real world coding (you would need to checks if it exists, apply real formatting, etc). Anyway, that should give the idea if that's what you needed.
-
Exactly! Just that this part:
<?php move_uploaded_file($_FILES["file"]["tmp_name"], "upload/" . $new_filename); echo "Stored in: " . "upload/" . $_FILES["file"]["name"]; ?>
could be put in a conditional, so that you know if the upload works:
<?php if (move_uploaded_file($_FILES["file"]["tmp_name"], "upload/" . $new_filename)) { echo 'Stored in: upload/' . $new_filename; } else { echo 'An error occurred while uploading.'; } ?>
-
I get the idea that people can see and add reviews, but you didn't tell where the movies will be fetched. Are you going to use an external movie database (like IMDB), or are movies going to be in your own database? The first option would be the most obvious choice imo, while reviews are of your own site.
The choice of the database reflects the programming options. If you choose to use IMDB, you'll have their API at your disposal which you can use to make searches and present information. If you make your own database, you'll have to code a simple search engine. MySQL has pretty neat feature called FULLTEXT, which basically indexes columns as "searchable" and has also a few functions to make the search. That would make searching a lot easier.
A search may be exact (generates one result) or partial (many results). If it is partial, you can present a page with the matched movie titles that link to the info and reviews page. Otherwise [if it's exact], you just redirect the user to the movie's reviews page. The process is quite simple and straightforward, even for a beginner.
-
Instead of $_FILES['file']['name'], you can use whatever you want. Take this example:
<?php $file = $_FILES['file']['name']; //get the file extension, we'll need it to construct the new filename $ext = pathinfo($file, PATHINFO_EXTENSION); $new_filename = "any_filename_you_want.$ext"; ?>
-
You mean:
<?php if (!empty($values)) { //insert query } ?>
or
<?php if (count($values)) { //insert query } ?>
PHP variables in MySQL query?
in PHP Coding Help
Posted
You should read a bit more before trying real stuff. Anyway, mysql_query() will return just an unusable [by itself] resource identifier. To convert that resource to real data, you need to fetch it into an array with one of mysql_fetch_*() functions.
If your query would return more than 1 row, you would need to loop through the results: