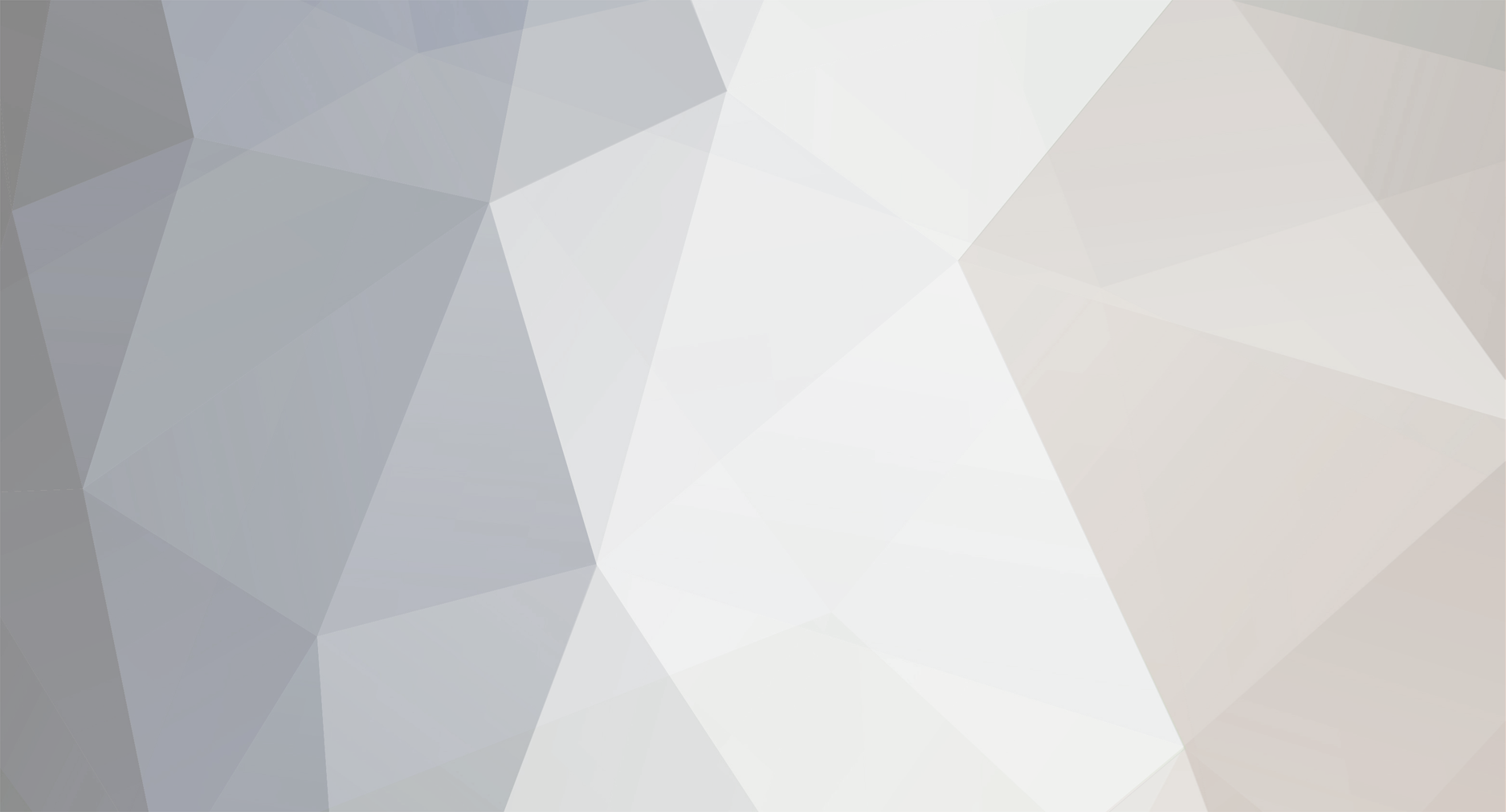
premiso
Members-
Posts
6,951 -
Joined
-
Last visited
-
Days Won
2
Everything posted by premiso
-
To prevent it you can do a header redirect to a "thank you" page. Doing this redirect will clear the POST data.
-
echo timeSince($profile['last_active']); function timeSince($urtime) { $seconds = (((time() - $urtime) / 1) % 60); $minutes = (intval((time() - $urtime) / 60) % 60); $hours = intval((time() - $urtime) / 3600); $days = intval((time() - $urtime) / 86400); $weeks = intval((time() - $urtime) / 604800); $months = intval((time() - $urtime) / 2419200); $years = intval((time() - $urtime) / 29030400); $result = array(); $result['years'] = ($years) ? sprintf('%u year%s,', number_format($years), ($years > 1) ? 's' : '') : FALSE; $result['months'] = ($months) ? sprintf('%u month%s,', number_format($months), ($months > 1) ? 's' : '') : FALSE; $result['weeks'] = ($weeks) ? sprintf('%u week%s,', number_format($weeks), ($weeks > 1) ? 's' : '') : FALSE; $result['days'] = ($days) ? sprintf('%u day%s,', number_format($days), ($days > 1) ? 's' : '') : FALSE; $result['hours'] = ($hours) ? sprintf('%u hour%s,', number_format($hours), ($hours > 1) ? 's' : '') : FALSE; $result[minutes'] = ($minutes) ? sprintf('%u minute%s', $minutes, ($minutes > 1) ? 's' : FALSE) : FALSE; $result['seconds'] = ($seconds) ? sprintf('%s %u second%s',($minutes) ? 'and' : '', $seconds, ($seconds > 1) ? 's' : FALSE) : FALSE; return (count($result) == 0) ? 'Never' : implode(' ', $result).' ago'; }
-
Do install (X|W|L)AMP or everything individually?
premiso replied to Yesideez's topic in Miscellaneous
Under Ubuntu, using Apt-get is very easy to install mysql/apache/php and any extensions you want via the same method. I would go that route over LAMP. -
Not sure where/why that is failing (other than maybe keys is a reserved word). $query = "UPDATE `meta` SET `keys` = '{$_POST['content']}' WHERE `keys` = '{$_POST['keys']}' LIMIT 1 "; Try that and see if it fixes the error. Given this statement: Learn SQL. EDIT: Once you get the basics down you should learn Normalization of SQL (3rd Normal Form). Here is description about auto_incrementing
-
Google SyncBack if you want a software version of it. Great software, I use it at work for a 3rd backup of our network drive.
-
Do install (X|W|L)AMP or everything individually?
premiso replied to Yesideez's topic in Miscellaneous
WAMP for windows i my favorite. On Linux (if using a debian based build) Just use apt-get, it installs everything very well. But LAMP is a linux based version. XAMP I used once and hated it. So if on Windows I would go with WAMP. -
Learn proper SQL first. If you ask me. A primary key should generally be an auto_incrementing integer, this is to prevent confusion with 2 of the same keys and allow you to update the proper records. Because without your update querying will not run, unless you pass the original keys each time, which is sort of ludicrous. Here would be a working example of your script as is. <?php require_once("../includes/connection.php"); // FORM PROCCESS if (isset($_POST['submit'])) { $query = "UPDATE `meta` SET `keys` = '{$_POST['content']}' WHERE keys = '{$_POST['keys']}' LIMIT 1 "; $result = mysql_query($query); if (mysql_affected_rows() == 1) { $message = "Update succesfull"; } else { $message = "There is problem"; $message .= "<br />" .mysql_error(); // needed a .= for appending } } // END OF PROCCESS $sql = "SELECT * FROM meta"; $result = MYSQL_QUERY($sql); $numberOfRows = mysql_num_rows($result); if ($numberOfRows==0) { echo "Sorry. No records found !!"; } else { $row = mysql_fetch_array($result); $keys = $row['keys']; } ?> <FORM action="meta.php" method="post"> <p>Edit:<br /> <input type="hidden" name="keys" value="<?php echo $keys; ?>" /> <textarea name="content" rows="10" cols="40"><?php echo $keys; ?></textarea> </p> <input type="submit" name="submit" value="Update Page" /> <br /> <?php echo $message; ?> </FORM>
-
First you need to know what row you want to update. You need a unique identifier (a Primary Key is preferred) for the table. So what is your table structure? You will need to pass that with the form. Second, your textarea name is "content" not $keys, so you need to use $_POST['content'] to reference the new value of the text area.
-
You never define $keys, you are trying to update the table where keys = $keys and you are updating keys to $keys, which makes no sense.
-
http://www.tizag.com/phpT/forms.php This is basic HTML with PHP. See the above tutorial for more information about it.
-
As I stated before, no where in the code you posted that checks if it has been 2 minutes do you either run the insert statement or deny it. You need to move the insert statement into that code for this to work. Leaving it outside of it will allow it to run no matter what. Bumping will not help as that is the answer.
-
Sounds like this project is way over your head. Start by learning SQL. The find a file upload in PHP Tutorial. If you do not even know the basics of SQL for File uploading you will not get very far, and we, do not have the time to take to research and explain every little bit to you, which is what you are essentially asking.
-
strcmp would be the easiest to compare 2 strings.
-
[SOLVED] Why won't this work, sort into descending.
premiso replied to jack bro's topic in PHP Coding Help
What is the field type of "rankpoints", it should be a numeric type (int etc) if it is not and it is a varchar or char, that is why. -
Do you have the flash plugin installed on your home computer?
-
Since you did not see that I posted the correct PHP 4 syntax: OOP PHP4 Read up more on it at the PHP Manual.
-
<?php class cal { var $currentMonth = 0; var $currentYear = 0; function cal() { echo 'constructor'; } }
-
Right, and posting that comment really makes me want to help now! If it looks so easy, why can you not do it? For a helping hand since I bothered posting. Where is obj defined? My bet is, that is causing an undefined object error and causing the switch to not run. I would use Firefox for debugging JavaScript as under Tools > Error Console it will show JavaScript errors.
-
To set a variable to a default value, this needs to be done in the constructor. You cannot do it like you are doing. <?php class bigCalendar { public $currentDay, $currentMonth, $currentYear; // etc.. public function __construct() { $this->currentDay = 0; $this->currentMonth = 0; // etc.. }
-
This is a Javascript issue. OnKeyPress is what you want to google/research. http://www.w3schools.com/jsref/jsref_onkeypress.asp
-
$sql = "UPDATE users SET number = '$var' WHERE username = '$username'"; You said the answer yourself....
-
I am stuck in tbody cross-browser help. Someone please help
premiso replied to simpli's topic in Javascript Help
Have you checked in the Javascript Error Console Firefox provides, to see what error that is showing? Tools > Error Console You may want to clear it then try and hiding it again and see what it says. -
Help with variables and combining variables
premiso replied to Darkmatter5's topic in PHP Coding Help
Use arrays <?php $querys=array(); $querys[]="UPDATE games SET title='$_POST[title]', descr='$_POST[descr]' WHERE game_id=$rowcon[game_id]"; $querys[]="UPDATE game_genres SET genre_id='$_POST[genre]' WHERE game_id=$rowcon[game_id]"; foreach ($querys as $sql) { $result = mysql_query($sql) or die(mysql_error()); } ?> The other method would be something like this: $query1="UPDATE games SET title='$_POST[title]', descr='$_POST[descr]' WHERE game_id=$rowcon[game_id]"; $query2="UPDATE game_genres SET genre_id='$_POST[genre]' WHERE game_id=$rowcon[game_id]"; for($i=1;$i<=2;$i++) { $result=mysql_query(${"query" . $i}) or die(mysql_error()); } But I would suggest using arrays. -
Look into jQuery, it is a framework that makes Ajax a bit easier. Alternatively you can generally find some good tutorials using jQuery for how to set it up.
-
.links A:link { text-decoration: underline; color: #FF9900; } .links A:visited { text-decoration: underline; color: #FF9900; } You needed the A:visited there is also an a:active incase that comes up at some time.