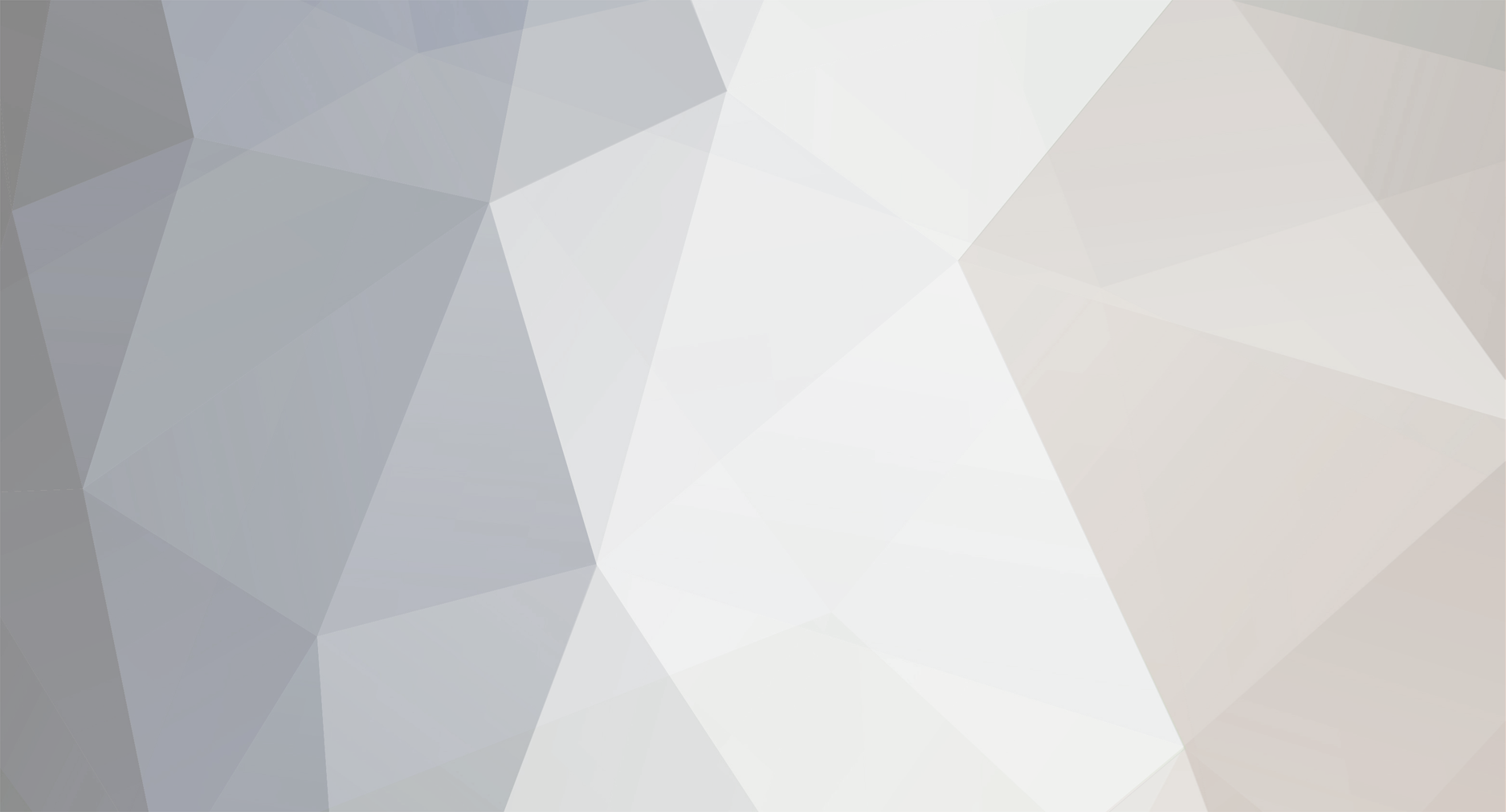
premiso
Members-
Posts
6,951 -
Joined
-
Last visited
-
Days Won
2
Everything posted by premiso
-
<?php $file = isset($_GET['a'])?basename($_GET['a']):index; if (!file_exists($file)) $file = "index"; include($file . ".php"); ?> Should do it. Use caution though, I took steps in there to prevent someone from loading a different file, but I am not guaranteeing that at all, I would prefer to use an array of defined files that are allowed and if $file is in that array then include it. This is an example of that: <?php $file = isset($_GET['a'])?basename($_GET['a']):index; $allowed = array("index", "home", "contact"); if (!file_exists($file)) $file = "index"; elseif (!in_array($file, $allowed)) $file = "index"; include($file . ".php"); ?> That should be the safer version.
-
$result = mysql_query("SELECT * FROM members WHERE username = '$logged[username]' LIMIT 5"); You have multiple users with the same username? That is a flaw in itself imo...
-
Is there a command to save a pdf file as text in php
premiso replied to samona's topic in PHP Coding Help
If it is an image you would need to use OCR to transfer it, and that is shaky at best. So there probably is, but as to whether it will work is a toss up. Someone else may have done this and know, but as far as I know it is not possible. -
For a user deal that changes a password I would highly recommend using POST instead of GET. Simple because that is saved in the users browser history. Also the </input> is not needed and may be causing issues. Instead do it like the submit one and do /> after the input statement.
-
Using FireFox there is an Error Console log that tells you all javascript errors. I am sure IE has an addon to do the same.
-
Why use 4 echos when 1 would suffice? Just an improvement to that code: while ($row= mysql_fetch_array($result)) { { echo " This user has been located<br /> <b>Userid: </b>" . $row['field_1'] . "<br /> <b>fName: </b>" . $row['field_2']. "<br /> <b>lName: </b>" . $row['field_3'] . "<br />"; } Just felt like pointing that out. Have a good one.
-
One, it does not seem that you are encrypting or hashing the password in the db, not very good practice. Look into md5 and use that for storing password. Two, why not pull out data only if the current password matches the SQL, and since sql is case-insensitive you will have to take that into account also (md5 would make the pass case sensitive). //construct a SQL query that selects users from DB $query="SELECT * FROM clientTable WHERE userName='$userName' AND userPass='$currentPass"; //run the query $result=mysql_query($query); //NOTE: Is this the problem maybe? if(mysql_num_rows($result) == 1) { $row = mysql_fetch_assoc($result); //store into a variables for the current row $myPass=$row['userPass']; //-------------------VALIDATE----------------------// //Now we do the case sensitive check since it is not md5 in the db. if($currentPass != $myPass){ header("Location:changePass.php?error=wrongpassword"); } Now if that does not work, check your form, and make sure it is passing via GET and not POST like most forms do, infact post the form code for us.
-
I, myself, prefer to use ReCaptcha instead of Captchas, The only thing, however, in this code that I can see going wrong, which may just be your preference is you space out everyone from [ [ in the array. I doubt this is the reason why, it is just weird to do imo. Anyhow if you would rather look into ReCaptcha here is an example of how it would work: <?php if (isset($_POST['submit']) && (!isset($_POST['extra']) || !empty($_POST['extra']))) { // execute code }else { ?> <script type="text/javascript"> <!-- function hide(id) { document.getElementById(id).style.display = "none"; } // --> </script> <form method="post"> <input type="text" name="fname" id="fname" /> <input type="text" name="extra" id="extra" /> <input type="submit" name="submit" Value="Submit!" id="submit" /> </form> <script type="text/javascript"> <!-- hide('extra'); // --> </script> <?php } ?> This way the user would never see extra (unless jscript is turned off) and they would not fill it in, since spam bots do not have jscript turned on usually they will almost always fill out that box cause they do not know any better. I know this does not solve the problem, just another idea instead of using captchas =)
-
[SOLVED] Best practices for user authentication?
premiso replied to Boo-urns's topic in PHP Coding Help
Yes...that is the point. But only if "Remember Me" was checked at some point. -
If this does not work, look up an example via jQuery: $.ajax({ url: 'logincheck.php', type: 'POST', data: 'username=' + username + '&password=' + password, success: function(result) { $('#response').remove(); $('#loginresult').html('<p id="response">' + result + '</p>'); setTimeout('$("#loginresult").slideUp("fast")',4000); } }); return false; }); }); That should trash the old stuff and replace it with the new.
-
[SOLVED] Help extracting certain part of string..
premiso replied to virtuexru's topic in PHP Coding Help
Yep much nicer than my way =P -
header("Location: {$_SERVER['PHP_SELF']}"); Try that and see if that works for ya. Np, I just wanted to make sure I did not miss something =)
-
Hmmm... didn't consider flash. At the moment I'm creating them as pngs, but will reduce them to jpegs. Left the script running and had a gig of images in a few hours. I won't be popular with the techies at work. The info is also being stored in a database and the idea was to only create the movements to the users requirements. So they choose the time frame that they want to view then the images are created, then combined. Worse case scenario is I create all the images then combine them in something like Adobe premiere, but that puts my workload up rather than being dynamic and user driven. I'll have a butchers at flash and ffmpeg, but if thats a new module I might be out of luck getting it installed. ffmpeg is a 3rd party script you would have to install. You would then use exec to run it with the right parameters. But as I said, I have never done this and that was just a shot in the dark of something you can look into and see if it does that.
-
I think going the flash route would be better here. Video creating etc bogs down the server ton. Not sure though, if you must do video look ito ffmpeg, my bet is though Flash would be better, you might even be able to create a .flv dynamically, I do not know.
-
header("Location: {$_SERVER['PHP_SELF']}"); You were using single quotes, $ in single quotes is taken literally. Try the above. How is using php_self bad? It cannot be modified outside since it is a server variable. I fail to see your reasoning, please elaborate for us all, thanks!
-
Thinking about it, do you ever clear the div field of it's old results? I bet that would do the trick. Because there you are just appending it. I do not know exactly how it is called, but yea. If they are two separate errors form 2 different requests, that is your answer, you should clear the result div before appending the new text.
-
[SOLVED] Help extracting certain part of string..
premiso replied to virtuexru's topic in PHP Coding Help
It can be done with regex using preg_match_all. But since I am not good at that here is an alternative way. <?php $string = '<img src="http://somewebsite.com/website/website/pix/20081119/19667358_1.JPG?" width=96 height=72 border=0> <img src="http://somewebsite.com/website/website/pix/20081119/19667358_2.JPG?" width=96 height=72 border=0> <img src="http://somewebsite.com/website/website/pix/20081119/19667358_3.JPG?" width=96 height=72 border=0>'; $strings = explode("border=0>", $string); array_pop($strings); $urlData = array(); foreach ($strings as $line) { list($data) = explode('" width=', $line); list(,$data) = explode('src="', $data); $urlData[] = explode('/', $data); } print_r($urlData); ?> Should work. Untested. Using regex would take out alot of the guessing game. Maybe someone will show you that, which would lower this to a couple lines. -
[SOLVED] Best practices for user authentication?
premiso replied to Boo-urns's topic in PHP Coding Help
Yep. Session files are stored on your server. Just don't store their password in it. But other misc data is fine to prevent having to re-query it each time. -
$arrayList = array(1,2,3,4,5); for($i = 0;$i < count($arrayList); $i++){ //do something here. // then echo completion information echo 'Process: ' . $arrayList[$i] . ' Complete <br />'; } ob_flush(); flush(); It will only work on some browsers. I know it works on FF, unsure about any others.
-
Can you post what the "return_value" function actually does? It sounds like it echos it. Without knowing exactly what that does we are at a loss for helping you...
-
[SOLVED] Best practices for user authentication?
premiso replied to Boo-urns's topic in PHP Coding Help
"The user can then browse around logged in via the session." And then to accomplish this, you check for a session on every page (that uses user info), right? What would you store in the session? sorry for all the questions...I just realized I have to redo my login system and I want to make sure I get it right this time. I generally store basic info in the session, but once a user is logged in/authenticated I set a session variable called "loggedin" to true and I check this each page. Then if I say use their username each page I set that in session also their userid for queries etc. This way it saves some queries from needing to be ran. But that is me. -
Not sure if this will work but try this: $user = (isset($_POST['username']) && !empty($_POST['username'])) ? mysql_real_escape_string( $_POST['username'] ) : false; $pass = (isset($_POST['password']) && !empty($_POST['password'])) ? mysql_real_escape_string( $_POST['password'] ) : false; That way it may return the right code. Unsure, but yea, either way that is a better way of doing it imo.
-
Most likely it is not returning null instead an empty string. Also to check null you need to use is_null Try this: $yearBuilt = $value->return_value("yearBuilt",TRUE); if (is_null($yearBuilt) || empty($yearBuilt)){ echo "no results"; }else{ echo $yearBuilt; } Should work just fine. FYI for future reference please place code in [.code][./code] tags (no period).