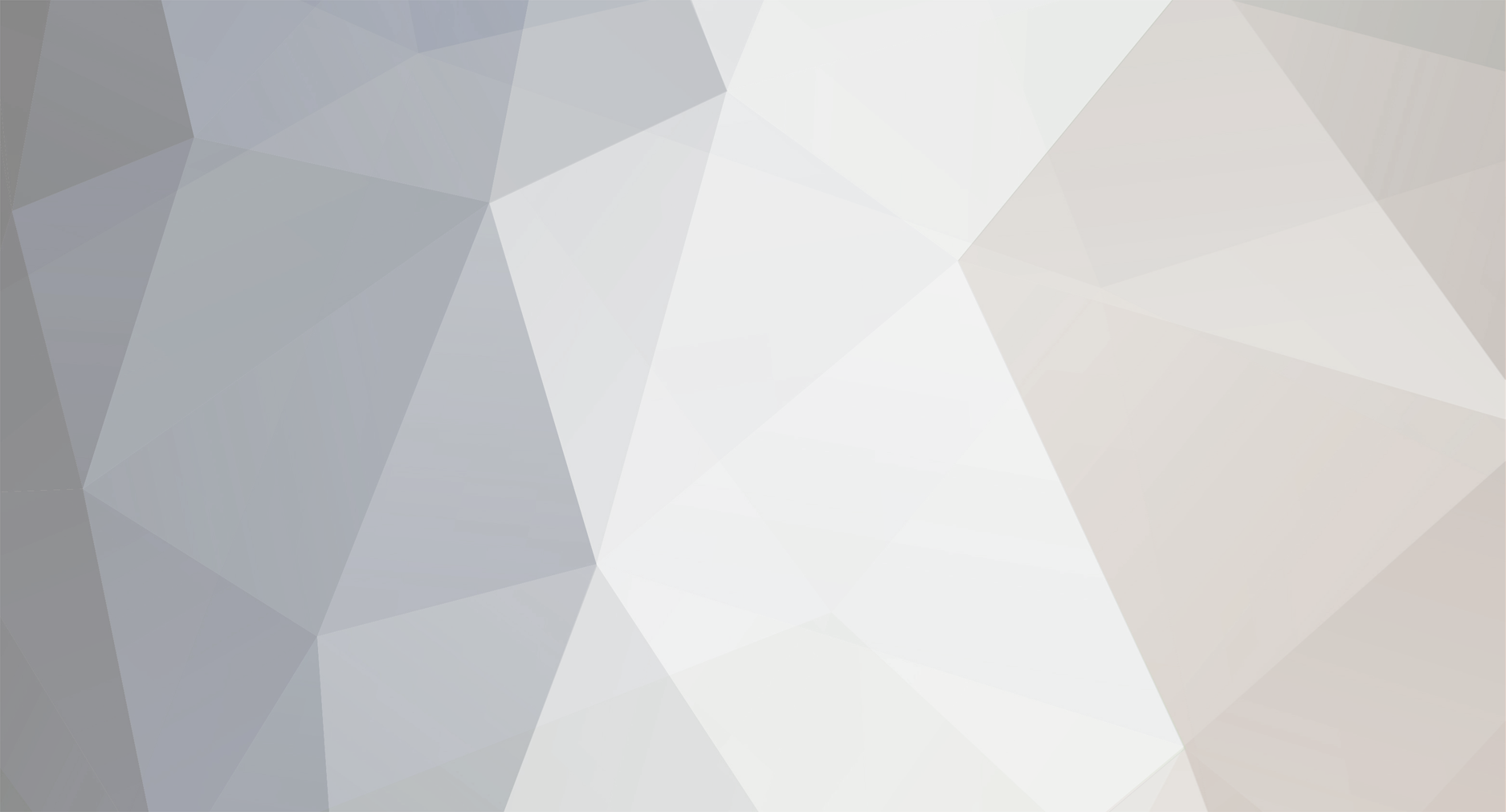
premiso
Members-
Posts
6,951 -
Joined
-
Last visited
-
Days Won
2
Everything posted by premiso
-
Do you have any code started? If not I think you should post in the freelance section, no one on this form wants to write a whole script for a user for free who is not even trying himself.
-
Getting filmID and displaying it in a Form Field
premiso replied to toxictoad's topic in PHP Coding Help
Yep that is correct, if you wanted to you could just put that link on the update.php page in a string and do not worry about having in the form itself. IE: <?php // initiate DB connection mysql_connect ("localhost", "USERNAME", "PASSWORD") or die ('error: ' .mysql_error()); mysql_select_db ("phatjoin_mymovies"); $title = $_POST['title']; $genre = $_POST['genre']; $year = $_POST['year']; $stars = $_POST['stars']; $country = $_POST['country']; //$link = $_POST['link']; $director = $_POST['director']; $plot = $_POST['plot']; $image = $_POST['image']; $trailer = $_POST['trailer']; $rating = $_POST['rating']; mysql_connect ("localhost", "USERNAME", "PASSWORD") or die ('error: ' .mysql_error()); mysql_select_db ("phatjoin_mymovies"); // pull the id for the last film entered into the movies table $lastFilm = mysql_fetch_assoc(mysql_query("SELECT filmID FROM mymovies ORDER BY id DESC LIMIT 1")); $link = "http://www.mydomain.com/mymovies/details.php?id=" . ($lastFilm['id'] + 1); // append the last id plus one to the link before inserting... $query="INSERT INTO mymovies (title, genre, year, stars, country, link, director, plot, image, trailer, rating) VALUES ('".$title."', '".$genre."', '".$year."', '".$stars."', '".$country."', '".$link."', '".$director."', '".$plot."', '".$image."', '".$trailer."', '".$rating."')"; mysql_query($query) or die ('Error updating database'); echo "" ; ?> <html> <head> <title>Updated Database</title> </head> <body> Database Updated<br /> <a href="http://www.mydomain.com/mymovies/form.php">Enter New Movie Information</a></body> </html> -
You are using a while in a for format. <?php $count = 0; // removed the $row = mysql cause that would end the loop prematurely while ($count <= 100) { if ($row = mysql_fetch_array($result)) { // process here } $count++; } ?> That should work for what you want. With a for statement it would look like this: <?php for ($count=0; $count<=100; $count++) { if ($row = mysql_fetch_array($result)) { // processing here } } ?>
-
echo'ing php code ... havin a little trouble here ..
premiso replied to imarockstar's topic in PHP Coding Help
It's fine to do it that way, its even better to build it from the database but to each their own. If it is actual php you are storing in the DB you want to look into www.php.net/eval It can be a huge security flaw to use that, so use it wisely. -
Getting filmID and displaying it in a Form Field
premiso replied to toxictoad's topic in PHP Coding Help
<?php // initiate DB connection mysql_connect ("localhost", "USERNAME", "PASSWORD") or die ('error: ' .mysql_error()); mysql_select_db ("phatjoin_mymovies"); <?php $title = $_POST['title']; $genre = $_POST['genre']; $year = $_POST['year']; $stars = $_POST['stars']; $country = $_POST['country']; $link = $_POST['link']; $director = $_POST['director']; $plot = $_POST['plot']; $image = $_POST['image']; $trailer = $_POST['trailer']; $rating = $_POST['rating']; mysql_connect ("localhost", "USERNAME", "PASSWORD") or die ('error: ' .mysql_error()); mysql_select_db ("phatjoin_mymovies"); // pull the id for the last film entered into the movies table $lastFilm = mysql_fetch_assoc(mysql_query("SELECT filmID FROM mymovies ORDER BY id DESC LIMIT 1")); $link .= ($lastFilm['id'] + 1); // appened the last id plus one to the link before inserting... $query="INSERT INTO mymovies (title, genre, year, stars, country, link, director, plot, image, trailer, rating) VALUES ('".$title."', '".$genre."', '".$year."', '".$stars."', '".$country."', '".$link."', '".$director."', '".$plot."', '".$image."', '".$trailer."', '".$rating."')"; mysql_query($query) or die ('Error updating database'); echo "" ; ?> <html> <head> <title>Updated Database</title> </head> <body> Database Updated<br /> <a href="http://www.mydomain.com/mymovies/form.php">Enter New Movie Information</a></body> </html> ?> I would actually put the linkid portion on the page where the data gets entered into the DB that way you know that the lastID+1 will be a legitimate one and will not be a duplicate. Adding one to the ID would make it a new ID, since the lastID would already have been taken, adding one would ensure that it is a new ID. Hope this helps. -
Getting filmID and displaying it in a Form Field
premiso replied to toxictoad's topic in PHP Coding Help
A side note, you may want to add 1 to the ID pulled from the DB so it is a new ID, I messed up on that part. -
Let's say you have this string: <![CDATA[ Some sample content is included here.<br><a border="0" href="http://www.exampleurl.com/" target="_blank"><img src="http://www.exampleurl.com/rss_image.jpg"/></a> ]]> If you have that string you can easily get the img url by doing the following: <?php $string = ' <![CDATA[ Some sample content is included here.<br><a border="0" href="http://www.exampleurl.com/" target="_blank"><img src="http://www.exampleurl.com/rss_image.jpg"/></a> ]]>'; $parsed = explode("src=\"", $string); $parsed = explode("\"/>", $parsed[1]); $imageUrl = $parsed[0]; echo $imageUrl; ?>
-
http://us.php.net/simplexml A good place to read up on it.
-
Getting filmID and displaying it in a Form Field
premiso replied to toxictoad's topic in PHP Coding Help
So what you will need is something like this: <?php // initiate DB connection // pull the id for the last film entered into the movies table $lastFilm = mysql_fetch_assoc(mysql_query("SELECT id FROM movies ORDER BY id DESC LIMIT 1")); $lastFilmID = $lastFilm['id']; ?> <html> <head> <title>update database</title> </head> <body> <form method="post" action="update.php"> Title: <input type="text" name="title" size="70" /><br /> Genre: <select name="genre"> <option value="BLANK"> </option> <option value="Action">Action</option> <option value="Adventure">Adventure</option> <option value="Animation">Animation</option> <option value="Biography">Biography</option> <option value="Comedy">Comedy</option> <option value="Crime">Crime</option> <option value="Documentary">Documentary</option> <option value="Drama">Drama</option> <option value="Fantasy">Fantasy</option> <option value="Horror">Horror</option> <option value="Martial Arts">Martial Arts</option> <option value="Musical">Musical</option> <option value="Mystery">Mystery</option> <option value="Romance">Romance</option> <option value="Sci-Fi">Sci-Fi</option> <option value="Thriller">Thriller</option> <option value="Western">Western</option> <option value="War">War</option> </select><br /> Year: <input type="text" name="year" size="20" /><br /> Stars: <select name="stars"> <option value="0"> </option> <option value="1">1</option> <option value="2">2</option> <option value="3">3</option> <option value="4">4</option> <option value="5">5</option> </select><br /> Country: <input type="text" name="country" size="30" /><br /> Link: <input type="text" name="link" size="60" value="http://www.mydomain.com/mymovies/details.php?id=<?php echo $lastFilmID; ?>" /><br /> Director: <input type="text" name="director" size="40" /><br /> Plot:<br /> <textarea name="plot" cols="50" rows="12"></textarea><br /> Image: <input type="text" name="image" size="30" value="covers/new" /><br /> Trailer: <input type="text" name="trailer" size="30" value="trailers/new" /><br /> Rating: <input type="text" name="rating" size="5" /><br /> <input type="submit" value="Update Database" /><br /> </body> </html> You will have to convert the above page to .php for this to work and have a mysql_connection active. -
Getting filmID and displaying it in a Form Field
premiso replied to toxictoad's topic in PHP Coding Help
Well without any code I am just going on a hunch that this is what you want <?php $newLink = '<a href=" http://www.phatjoints.com/mymovies/details.php?id=' . $_POST['id'] . '">Movie Details</a>'; echo $newLink; ?> If not please post some code or be much more specific. -
I have yet to see any function that actually decrypts an md5 hash. What I have seen is plenty of reverse-lookups against known hash values. Had to reply, just a statement, no where did I state that a function will decrypt an MD5 hash. It will decrypt a hash that has been made to be decrypted using an encrypt/decrypt function someone else created. If you do search the user comments on the php.net md5 page you will find a few functions that will allow encryption/decryption of their own created hash. The kicker is it has to be hashed using that encrypt function and decrypted using their decryption function. This is in no way as secure as MD5 is for the obvious reasons. But there is a way to encrypt/decrypt strings if using the right function. That was simply the point I was making. Thank you.
-
If you can decrypt it, it's not really MD5.... =/ Agreed, but there are functions created on that page that allow encryption/decryption if someone is looking for that type of functionality. =)
-
You can't unless you used a modified version to encrypt it. MD5 is a one-way hash. If you want to be able to decrypt it take a look at the comments under http://www.php.net/md5 I am sure you will find an encrypt/decrypt version there.
-
<?php $time=$_POST["time"]; $person=$_POST["user"]; if (file_exists("register.txt")) { $fileCheck = file("register.txt"); }else { $fileCheck = array(); } $file = fopen("register.txt", "a+"); $newperson = "$time , $person\n"; $infile = false; foreach ($fileCheck as $line) { if (stristr($line, $time) !== false) { echo "The timeslot is taken"; $infile = true; } if (stristr($line, $name) !== false) { echo "The name is taken"; $infile = true; } } if (!$infile) { fwrite($file, $newperson); } echo "Time: $time"; echo "<br> Name: $person"; ?> The above is modified so it will not give out any errors if it is the first entry/has not been created yet. Edit: Just missed a question. The question is if name OR time is found do you want to exit and say that the new stuff cannot be added? If so this version will do just that. <?php $time=$_POST["time"]; $person=$_POST["user"]; if (file_exists("register.txt")) { $fileCheck = file("register.txt"); }else { $fileCheck = array(); } $file = fopen("register.txt", "a+"); $newperson = "$time , $person\n"; $infile = false; foreach ($fileCheck as $line) { if (stristr($line, $time) !== false) { echo "The timeslot is taken"; $infile = true; break; }elseif (stristr($line, $name) !== false) { echo "The name is taken"; $infile = true; break; } } if (!$infile) { fwrite($file, $newperson); } echo "Time: $time"; echo "<br> Name: $person"; ?>
-
I would use www.php.net/file to read the file, this will read the file putting each new line into an array. You can then search the array using the www.php.net/foreach function. <?php <? $time=$_POST["time"]; $person=$_POST["user"]; $fileCheck = file("register.txt"); $file = fopen("register.txt", "a+"); $newperson = "$time , $person\n"; $infile = false; foreach ($fileCheck as $line) { if (stristr($line, $time) !== false) { echo "The timeslot is taken"; $infile = true; } if (stristr($line, $name) !== false) { echo "The name is taken"; $infile = true; } } if (!$infile) { fwrite($file, $newperson); } echo "Time: $time"; echo "<br> Name: $person"; ?> Not sure if that is the best way, but it would work.
-
It would be a combination of substr and strrpos. You would use strpos to locate the last location of the last _ from there you would use substr to pull out 0 to that last position then append the [] to the end of the string. <?php $string = "index_ok_1"; $newString = substr($string, 0, strrpos($string, '_')) . "[]"; echo $newString; ?> Edit: Decided to add the code cause I was bored.
-
[SOLVED] Javascript for id that contains a counter as part of the name
premiso replied to limitphp's topic in Javascript Help
Without more code it is hard to diagnose, that and this is a javascript issue not php. But what you have should work although I would change this: var = "voteCount"+count; to var countName = "voteCount" + count; I would alret the countName and make sure it is what you expect it to be, cause as long as there is a div with that name, it should work flawlessly. -
Nope, I just wanted to make sure there wasn't a difference. I think I am going to be trying to create a new way (at least for me) of verifying the user so what is passed to the php won't exactly work another day. Anyhow thanks for that Corbin.
-
Hello, I am making a script for user authentication to avoid the page reload to authenticate a user. My question is, how secure is it to send the username/password combo to a script to authenticate the user? Should the password be encrypted before passed? Just curious I have always wondered if that would be a potential security flaw with someone sniffing the network. Thanks for any replies!
-
Confirm that you have confirmUserID function somewhere in one of your pages and that it is inclued.
-
No, I was talking with Blade =) Sorry I thought quoting him would show that.
-
Not to be mean or anything, but that is completely wrong =) http://us2.php.net/strpos strpos — Find position of first occurrence of a string Finds the first position, as in my lengthy post I described that a string is an array of Characters, and thus are 0-index based so sony at the very start of the word would return 0, thus rendering the above code as wrong/bad code. That is why they have the !== false Again I am not meaning to be rude, just enlightening you. =)
-
Sorry I thought the question was answered. Here is the answer. The reason the person uses the !== false is because the function may return 0 if sony is at the very beginning of the string as that is the starting position, since a string is really just an array of characters it is 0-index based. So no, it will not return a 1 if the word is in the string, it may return 0. By implementing the !== false you take away from the 0 as being a false instead it is true since that is where the sony position starts at in the string. If you know that sony will never be at the start of the string, that is fine to check it against 0. The point of strpos is to return the first position of the word. I hope that helps clear things up for you. So this $isMobile = strpos($ua, 'sony') or die(); May kill the page even though sony is in $ua, and yea you do not want to do that. $isMobile = strpos($ua, 'sony') !== false; Will return true, if the word is inside $ua and false if the word is not (or a 1 for true/0 for false). The above representation is like a short if statement it could be presented like so: <?php $isMobile = false; if (strpos($ua, 'sony') !== false) { $isMobile = true; } if ($isMobile) { echo 'This came from a mobile!'; }else { echo 'This came from a PC!!! EEEK!'; } ?>