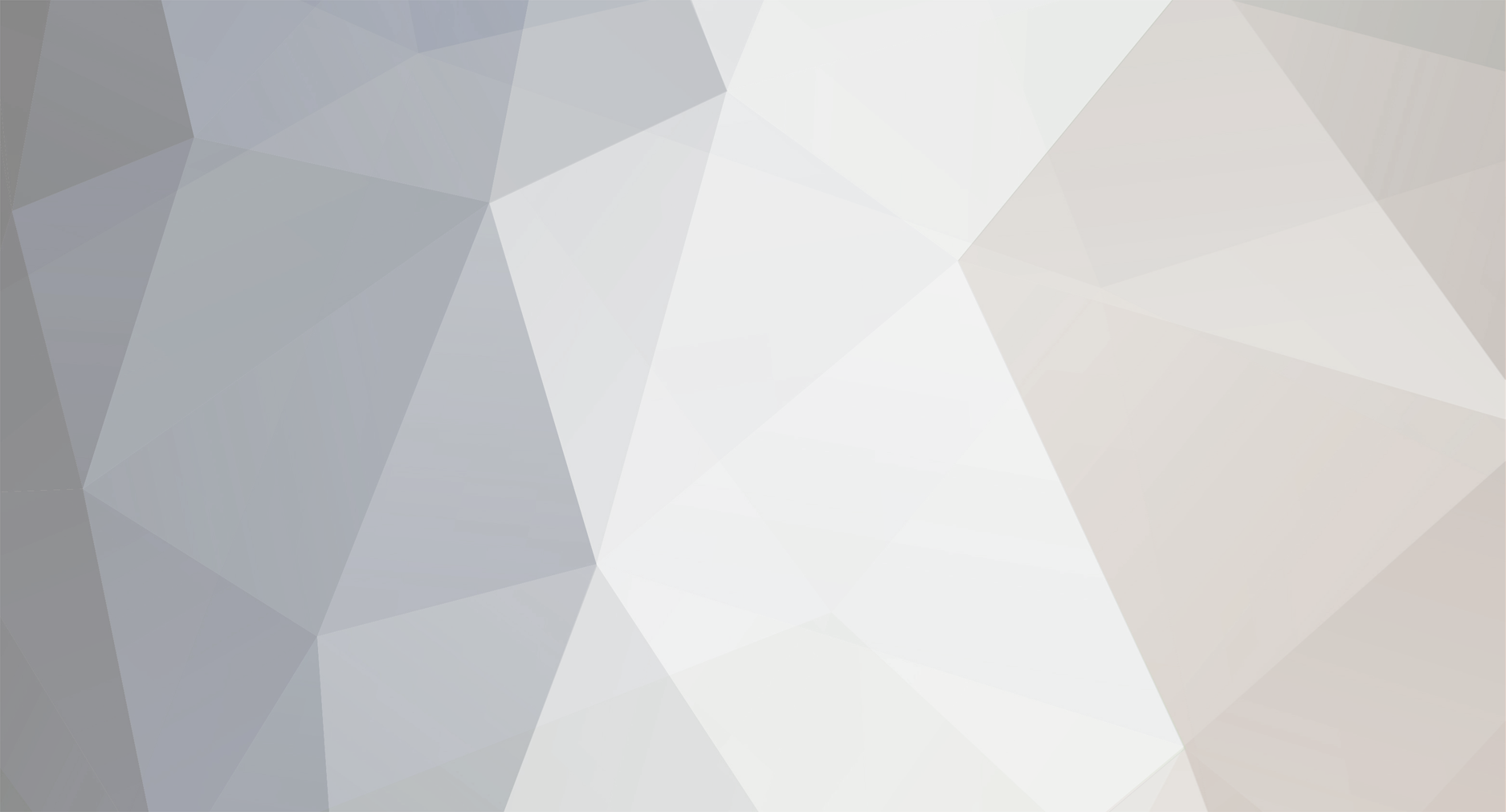
premiso
Members-
Posts
6,951 -
Joined
-
Last visited
-
Days Won
2
Everything posted by premiso
-
It will not work cause you are doing an = to the date. It has to match exactly, meaning you need the hours, minutes and seconds display like they are in the DB with your date value. $date = date ('Y-m-d h:m:s'); // not sure on the hours minutes / seconds look at the date function via php.net for correct usage.
-
My ways are not the best, but they work and since I hate regex this is how I would do it. <?php function grabRSSFeeds($website) { $file = file($website); foreach ($file as $line) { $line = strtolower($line); if (stristr($line, "link") !== false) { if (stristr($line, "application/rss+xml") !== false) { $line = str_replace("\"", "", $line); $line = str_replace("'", "", $line); $splitIt = split("href=", $line); $linked = split(" ", $splitIt[1]); // split at first space $links[] = $linked[0]; } }elseif (stristr($line, "<body")) { break; //kill the foreach if we reached the body tag. } } return $links; } echo '<pre>'; print_r(grabRSSFeeds('http://www.9news.com')); // should return an array of the links echo '</pre>' ?> Untested but should work. EDIT: Added a ' and space check that "should" cover about any type of setup you would find.
-
creating a forum for your website using php and SQL
premiso replied to Jiraiya's topic in PHP Coding Help
If you want to learn, here are a couple of good tutorials for a simple forum using PHP and MySQL. http://www.phpeasystep.com/workshopview.php?id=12 http://www.talk-mania.com/php-perl-java-javascript-cgi-tutorials/27817-creating-simple-php-forum-tutorial.html -
Do you have any code started? The table I would/do use is: create TABLE user_session ( sessionhash varchar50 NOT NULL, userid int(11) NOT NULL, last_activity int(11) NOT NULL, primary key(sessionhash) ) You can do this with or without the sessionhash part, if you remove the session hash I would suggest putting the userid as the primary key. Then each page load you just query and pull out all users where last_activity < 5 minutes ago (or however many minutes you want). Hope that helps, if you are still confused, google "Who's Online PHP" and you should find many pre-made scripts.
-
Depending on how you call the page I would do this: <?php // Retrieve data from database $sql="SELECT * FROM homeblocks"; $result=mysql_query($sql); // Start looping rows in mysql database. while($rows=mysql_fetch_array($result)){ if (isset($_GET['page']) && $_GET['page'] == $rows['id']) { echo "<div class='navlinkactive'><a href='?page=" . $rows['id'] . "'>" . $rows['title'] . "</a></div> "; }else { echo "<div class='navlink'><a href='?page=" . $rows['id'] . "'>" . $rows['title'] . "</a></div> "; } } ?> NOTE:::: You may have to change your script where you used to just use ?= to use page now.
-
Non-SQL version $paid_lookup = mysql_query("SELECT * FROM `check_database` WHERE `bill_code` = '$bill_code'") or die('MySQL Connection Error:'.mysql_error()); $amount_paid = 0; while($paid = mysql_fetch_array($paid_lookup)){ $amount_paid = $amount_paid + $paid[num_amt]; } SQL Version: $paid_lookup = mysql_query("SELECT SUM(num_amt) FROM `check_database` WHERE `bill_code` = '$bill_code'") or die('MySQL Connection Error:'.mysql_error()); $amount_paid = 0; while($paid = mysql_fetch_array($paid_lookup)){ $amount_paid = $paid[num_amt]; } I am unsure if the SQL version will work, I think it will but yea.
-
request help with hyperlinking flat file output...
premiso replied to slashpine's topic in PHP Coding Help
Basic html... <?php $lineData = "<tr><td>" . date("l, F jS Y - H:i:s-"). "</td><td>" . ($ip = $_SERVER['REMOTE_ADDR']) . "</td><td>" . '<a href="' . $_SERVER['HTTP_REFERER'] . '">Referer</a></td></tr>'; fwrite($hFile,); ?> You will need <table> tag somewhere before the first line and a </table> somewhere after the last line. -
Honestly, if thats the way you want to do it I am sure that will prevent against SQL injection. Not sure which way would be better, but if it was me that would not be my choice.
-
The main issue with that is, how are you going to display the username? What if you have another field a user can update, such as their birthdate? What if you have a biography field that you cannot MD5 cause you would not be able to retrieve it/the bio would get cut off... Just not realistic. Better just to use the mysql function.
-
Are you working on a server or localhost? If localhost check out this article... http://www.aeonity.com/frost/php-setcookie-localhost-apache
-
and display them. error_reporting(E_ALL); ini_set('display_errors', 1); I believe that will get the desired results displayed.
-
Below is the code I used. The main downside is if a user is using a free stats counter/tracker that uses javascript/image this may flag it up as being bad. Other than that this will check all content, the javascript page and any page it includes/redirects to for bad stuff. I know it is not the prettiest code in the world, nor the most efficient but it works. You may have errors as this was in a class of mine, so I tried my best to weed out the class variables but hopefully it just works. Questions let me know. <?php /** * Checks the given content for any type of bad javascript. * returns an error message if it is bad content or * returns false if the content is fine. */ function jsSpamCheck($content) { $content = strtolower(stripslashes($content)); if (ereg("script", $content)) { $javaScriptArr = split('<script', $content); foreach ($javaScriptArr as $key => $val) { list($val) = split('</script>', $val); if (ereg("src=", $val)) { list($url) = split(">", $val); if (!ereg("src=", $url)) { continue; } list(,$url) = split("src=", $val); if (ereg("language=", $url)) { }elseif (ereg("type=", $url)) { } $url = str_replace('"', "", $url); $url = str_replace("'", "", $url); $url = str_replace(">", "", $url); $url = trim($url); if (checkURL($url)) { return "You have tried to use disallowed javascript code. We do not allow cookie access or any type of redirects on this site."; } }elseif (ereg("eval", $val)) { list($javaScript, $evalList) = split("eval", $val); $jsTags = split('";', $javaScript); foreach ($jsTags as $jsKey => $jsVal) { list($jsName, $jsValue) = split('="', $jsVal); $jsName = ereg_replace('var ', '', $jsName); $jsName = ereg_replace(" \n", '', $jsName); if (trim($jsName) != "") { $jsEval[trim($jsName)] = $jsValue; } } $evalList = ereg_replace("\(", "", $evalList); $evalList = ereg_replace(");", "", $evalList); $evalKeys = split("\+", $evalList); foreach ($evalKeys as $key => $val) { $jsOutput .= $jsEval[$val]; } if (ereg("dow.locat", $jsOutput)) { return "You have tried to use disallowed javascript code. We do not allow cookie access or any type of redirects on this site."; } } } return false; } } /** * Checks the given url for any type of redirection spam. * returns true if it does redirect and false if it does not. * this is a recursive function. */ function checkURL($url) { $file = @file_get_contents($url); $file = strtolower($file); if (ereg("location.href=", $file) || ereg("location.replace\(", $file)) { return true; }elseif (ereg("src=", $file)) { list(,$newURL) = split('http://', $file); if (ereg("'", $newURL)) { $splitAt = '\''; }else { $splitAt = "\""; } list($newURL) = split($splitAt, $newURL); return checkURL("http://" . $newURL); } return false; } ?>
-
Is there a standard max length for a username field?
premiso replied to limitphp's topic in PHP Coding Help
I personally max all mine out at 25. For my purposes there is usually never a reason for a username to be longer than that other than spamming reasons. -
If you ask me, you should know just about all the data coming into your site, with the exception of a site that uses templates/massive text. But by knowing what is allowed and what is not you should be able to validate everything easily. Age should be an int, a username should only contain certain characters and have a min length, a password should be whatever as long as it is a certain length cause it should be md5 hashed. A text box, if it is not used for html purposes should not contain any html tags, etc. I would also only grab data I expect. I never just loop through the get and post because someone can easily tack on what they want and it could potentially hurt the site. If these steps are taken you should not have any issues with XSS exploits. If you do run a blog site with templates where a user can modify the code, I usually check for document.cookie and other misc. javascript XSS exploits and if it is there (even if included from another website I read in that file and keep following and checking) I tell the user that is not allowed. I actually created a script that will read an off-site js file and if it has the document.cookie spread out between variables, it gathers all those variables and applys them like the script does and if that equals the document.cookie or non-allowed code than I disallow it. If I can find that script I will post it here, but yea. =)
-
Doesnt have categories (those are kind of advanced imo due to recursion). http://www.chipmunk-scripts.com/board/index.php?forumID=43&ID=9268 Check out that script to get you started.
-
You have to do it with last_time_seen. Basically you cannot really have an up-to-date deal the best way is to have a table with the time the last user is seen, if that time is greater than X minutes they are not longer online. SO you would just check that on page load to see whose time < X minutes if it is they are considered online. Along with that you have to set the last_seen each time the user goes to another page.
-
How is this related to PHP? Should be in the Javascript form....
-
You have html inside the PHP tags. Why are you attempting to connect to a database here? I do not see a spot where you use it on this page? <?php mysql_connect("localhost", "root", "catch2@") or die ("Could not connect to database"); mysql_select_db("guestbook") or die ("Could not select database"); ?> <form action="myscript.php"> <input type="text" name="surname"> <input type="submit" name="submit" value="submit"> </form> <h2>Sign my Guest Book!!!</h2> <form method=post action="create_entry.php"> <b>Name:</b> <input type=text size=40 name=name> <br> <b>Location:</b> <input type=text size=40 name=location> <br> <b>Email:</b> <input type=text size=40 name=email> <br> <b>Home Page URL:</b> <input type=text size=40 name=url> <br> <b>Comments:</b> <textarea name=comments cols=40 rows=4 wrap=virtual></textarea> <br> <input type=submit name=submit value="Sign!"> <input type=reset name=reset value="Start Over"> </form>
-
Sticky form, but already in php tags. Help!
premiso replied to Scorptique's topic in PHP Coding Help
What do you mean by "make it sticky" ? -
One way to check would be using the file_exists. If the file exists, chances are you are not getting XSS attacked. If it does not, you probably are.
-
[SOLVED] Error using AND with multiple OR statements MySQL Query
premiso replied to jaxdevil's topic in MySQL Help
Basic math I guess, order of operations. <?php $result = mysql_query("SELECT count(*) FROM receipt_of_bill WHERE (`status`='$status' AND `bill_code` LIKE '%$searchstring%') OR (`status`='$status' AND `vendor_name` LIKE '%$searchstring%') OR (`status`='$status' AND `date_of_bill` LIKE '%$searchstring%') OR (`status`='$status' AND `ref_number` LIKE '%$searchstring%') OR (`status`='$status' AND `amount_due` LIKE '%$searchstring%') OR (`status`='$status' AND `bill_due` LIKE '%$searchstring%') OR (`status`='$status' AND `memo` LIKE '%$searchstring%')"); ?> You have to surround each pair of AND statements inside parans or else this will ultimately always be true thus return all rows. -
You should always encrypt user passwords with MD5 (a one way hash). This ensures that no one will ever see the clear text password. Although it cannot be reversed, which is a good thing, it protects the user's password. Even if it is echo'd out, it would not do anyone any good. That and I would hope user information is kept in a database such as MySQL.
-
request help with hyperlinking flat file output...
premiso replied to slashpine's topic in PHP Coding Help
umm... <?php $refLink = '<a href="' . $_SERVER['HTTP_REFERER'] . '">Referer</a>'; fwrite($hFile,date("l, F jS Y - H:i:s-").($ip = $_SERVER['REMOTE_ADDR']).($refLink)); ?> If it is displayed as text, it will just be the a href deal, if it is displayed as html should show up as a link. -
Nice job, thanks for the posting the query you used, it may come in hand at a later date.
-
Use an include with variables... is this possible?
premiso replied to Dustin013's topic in PHP Coding Help
Agree with you 100%, I just figured he wanted to define his own $_GET. But you bring up a valid point, if the get is coming from the page that is including the somefile.php the get data will pass thru just fine without doing what I did above. Thanks Mchl for bringing that up. I just figured he would have already known that =)