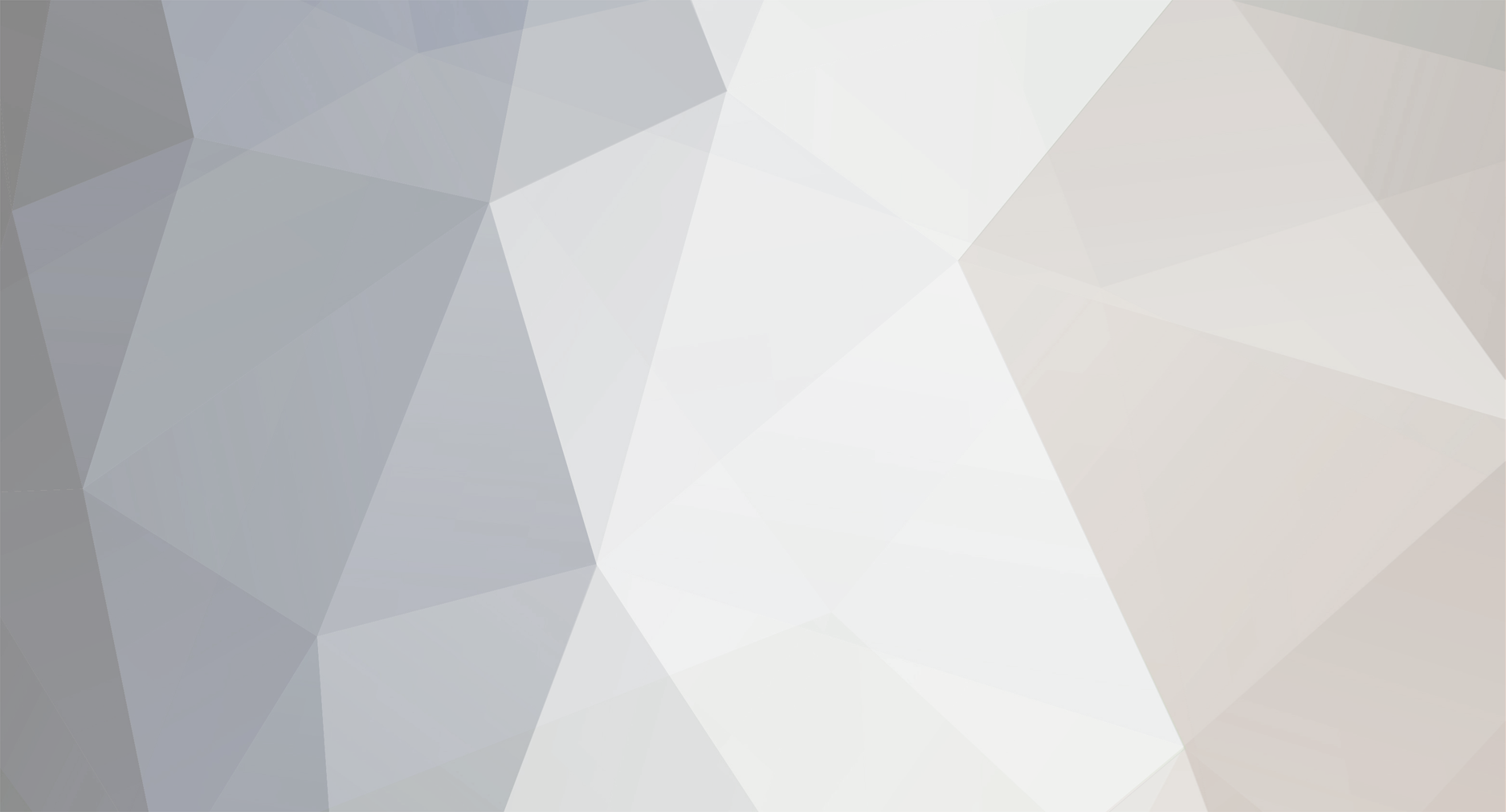
premiso
Members-
Posts
6,951 -
Joined
-
Last visited
-
Days Won
2
Everything posted by premiso
-
Did you try that example? That is done right, the issue you are having is that output is being sent to the screen before the header is called, which is why it is throwing the error. A good practice is to keep all the output in a variable and print it later to avoid such hardships as this and even setcookie functions from raising errors as they have to be done before any output is sent to the page. My suggestion would be to store all output (including the output of included files) into a local variable, such as $display that you can just echo out. This way you have full control over what is sent to the page. EDIT: If you notice all the ones you are trying the header is AFTER output, which is incorrect. I suggest reading up www.php.net/header on the header() function more before you rip all your hair out over a known issue.
-
[SOLVED] Having trouble with challenge key auth system
premiso replied to aosmith's topic in PHP Coding Help
In your sql query, you need to use single quotes not double quotes for starters. -
<?php include('db_connect.php'); $query = 'SELECT * FROM bio'; $r = mysql_query ($query); if ($r = mysql_query ($query)) { while ($row = mysql_fetch_array ($r)) { $data = $row['data']; $id = $row['id']; ?> <p> <FORM action="<?php echo $_SERVER['PHP_SELF']; ?>" method="post" name="submitnews" id="submitnews"> <textarea rows="10" id="news" name="news"><?php echo $data; ?></textarea> </FORM></p> <?php } } else{ print 'Failed'; } ?> You referred to it as $_POST instead of $row.
-
[SOLVED] 'php-include' - call this file every 30 seconds?
premiso replied to mellojoe's topic in PHP Coding Help
<?php header('Content-type: image/png');//we want all images outputted to be png header( 'refresh: 30;' ); include('URLTORANDOMIMAGESCRIPTHERE'); ?> A modification to the above. That should work. -
Can you run a PHP script using JS?? (I know it's weird)
premiso replied to markis's topic in PHP Coding Help
There are a few different ways, but really, the ajax portion should not contain sensitive data in itself. One way to verify it is by using session and storing a hash that is randomly generated each page call and check that the hash is correct. -
Its a pain in the butt. Basically you load all the emails into an array using www.php.net/file From that you loop through writing the emails to the file and making sure you do an if check to omit the email you want removed.
-
www.php.net/date look at that as it has all the masks needed to convert a timestamp to show military time.
-
Any reason NOT to have a mysql_query executed in a while loop?
premiso replied to suttercain's topic in PHP Coding Help
<?php require('../includes/get_connected.php'); // include database //GROUP BY// $sql = "SELECT seriesTitle, series, title, image FROM actionFigures ORDER BY seriesTitle, series, title"; $result = mysql_query($sql) or die(mysql_error()); if ($result) { $prev_title = "" while ($row = mysql_fetch_array($result)) { if ($prev_title != $row['seriesTitle']) { echo $row['seriesTitle']. " " .$row['series']; echo "<br />"; $prev_title = $row['seriesTitle']; } echo "<a href='http://www.supermandatabase.com/images/actionFigures/".$row['image']."'>"; echo $row['title']; echo "</a>"; echo "<br />"; } } ?> Try it without the group by and use order by. Also defining columns in the mysql query is better than using * if you know what columns you need to use. -
Any reason NOT to have a mysql_query executed in a while loop?
premiso replied to suttercain's topic in PHP Coding Help
Understandable. Here is a quick solution to your problem without creating extra load on the mysql server. <?php require('../includes/get_connected.php'); // include database //GROUP BY// $sql = "SELECT * FROM actionFigures GROUP BY seriesTitle, series ORDER BY title"; $result = mysql_query($sql) or die(mysql_error()); if ($result) { $prev_title = "" while ($row = mysql_fetch_array($result)) { if ($prev_title != $row['seriesTitle']) { echo $row['seriesTitle']. " " .$row['series']; echo "<br />"; $prev_title = $row['seriesTitle']; } echo "<a href='http://www.supermandatabase.com/images/actionFigures/".$row['image']."'>"; echo $row['title']; echo "</a>"; echo "<br />"; } } ?> Probably a better way to do it, but that will/should work. -
echo "<li>Time Submitted:" . date("m/d/Y",$timestamp) . "</li>"; Add a $ before timestamp ?
-
Any reason NOT to have a mysql_query executed in a while loop?
premiso replied to suttercain's topic in PHP Coding Help
Probably because it does not make sense to pull the same exact data twice? You are pulling from the same table, same fields. That code is redundant and pointless as this would accomplish what you want: <?php require('../includes/get_connected.php'); // include database //GROUP BY// $sql = "SELECT * FROM actionFigures GROUP BY seriesTitle, series"; $result = mysql_query($sql) or die(mysql_error()); if ($result) { while ($row = mysql_fetch_array($result)) { echo $row['seriesTitle']. " " .$row['series']; echo "<br />"; echo "<a href='http://www.supermandatabase.com/images/actionFigures/".$row['image']."'>"; echo $row['title']; echo "</a>"; echo "<br />"; } } ?> Should pull the same data. If you want it to be organized a certain way, the use order by, but since there is a group by it is already organized. What type of data are you trying to pull out? Why would you need that inner mysql loop? -
www.php.net/readfile That will help you, don't use the fopen stuff. That is what readfile was intended for.
-
As long as you were working with a file in the directory. I would suggest making a constant in a common page define("SERVER_PATH", $_SERVER['DOCUMENT_ROOT']); also remember that $PHP_SELF means register_globals are on which is a security vulnerability. You should use $_SERVER['PHP_SELF'] instead.
-
[SOLVED] 'php-include' - call this file every 30 seconds?
premiso replied to mellojoe's topic in PHP Coding Help
Using AJAX. You set a div with innerhtml tag, when the timer hits 30 seconds ajax makes a call to a script and grabs the next random image url, set that as the new innerhtml and you are good. -
Why is it a bad idea? Many reasons it should be avoided. One it may not be compatible on another server. Two it is not versatile, you cannot take the script and put it in an upper level directory and expect it to work. Finally if you plan on using htaccess for seo and use a "processing" page to include different files etc, you need the absolute path because the relative path is not what you would expect. It is just good practice to use the absolute path over the relative path.
-
Options +FollowSymlinks RewriteEngine on RewriteRule ^(.*)\.(jpg|gif|png)$ images.php?filename=$1 [nc] I am not sure how to send it in the filename...but that will send the gif and png files also to images.php
-
Kind of an oxymoron deal here. As cooldude stated the issue is with strip tags. The first post you are attempting to locate tags, but you ultimately strip them before you even try to process the page. The second post you made you are taking the text between what you want and using that, since there are no tags in the second post it works. Basically if you want to use the table or any html tags to grab data, don't strip tags from the incoming data.
-
<?php $date = "2007-10-02"; $timestamp = strtotime($date); $new_date = date("mdy", $timestamp); ?> www.php.net/date www.php.net/strtotime
-
Implement some debugging standards. What is the filename printing out? Is it printing out the full path or not? You should probably add the full path to it, as the .htaccess rewrite changes the directory to be where the images.php file is. So even though the url is pointing to /images/etc.jpg the relative path would be /image.php so you would then need to add that into the get string. The script will work like it is suppose to, you just have to have the full path to the file.
-
<?php header('Content-type: images/jpeg'); if(!file_exists($_GET['filename'])) readfile("http://tzfiles.com/imageNotFound.jpg"); else readfile($_GET['filename']); ?> In order for it to be interpreted as an image it must be read and written to the browser as an image. The img src is part of html, which is not part of an image file.
-
Remember that you need to use www.php.net/header and www.php.net/readfile The header you need to set the content-type to be jpeg and the readfile is to basically display the image from the file.
-
<?php $table = "tl"; $user = 'root'; $pass = ''; $connect = mysql_connect('localhost', $user, $pass); if(!$connect) { echo 'Can not connect!'; }else{ mysql_query("DROP DATABASE $table"); mysql_query("CREATE DATABASE $table"); $fh = file('db_data.sql'); mysql_select_db($table); $x=0; foreach ($fh as $line) { if (trim($line) != "") { if (stristr($line, "CREATE TABLE")) { $cont = true; $lines = $fh[$x]; $b=$x+1; while ($cont) { $lines .= " " . $fh[$b]; if (stristr($fh[$b], ")")) { $cont = false; }else { $b++; } } mysql_query($lines) or die('Error:' . mysql_error() . '<br />'); }else { mysql_query($line) or die('Error:' . mysql_error() . '<br />'); } } $x++; } } ?> That does not take into account for semicolons, which might also cause problems. Note the above is <b>UN-TESTED</b>.
-
Are you sure that display_errors is turned on via www.php.net/ini_set ?
-
Its not strange, you dont have the errors being handled with: <?php $table = "tl"; $user = 'root'; $pass = ''; $connect = mysql_connect('localhost', $user, $pass); if(!$connect) { echo 'Can not connect!'; }else{ mysql_query("DROP DATABASE $table"); mysql_query("CREATE DATABASE $table"); $fh = file('db_data.sql'); mysql_select_db($table); foreach ($fh as $line) { if (trim($line) != "") { mysql_query($line) or echo 'Error:' . mysql_error() . '<br />'; } } } ?> As suggested earlier. EDIT: As a note, just thinking around. If you are creating tables and the export of sql was a dump or from phpmyadmin chances are the table declarations are spread out on separate lines, which will cause errors. You need to create a routine that checks for a table declaration and then does an internal loop inside checking for the end and combining that into one line. I bet that is the main issue.
-
<?php $get_data = ""; foreach ($_GET as $key => $val) { $get_data .= "&" . $key . "=" . $val; } echo '<a href="index.php?var=1' . $get_data . '">Link Here</a>'; ?> Like that?