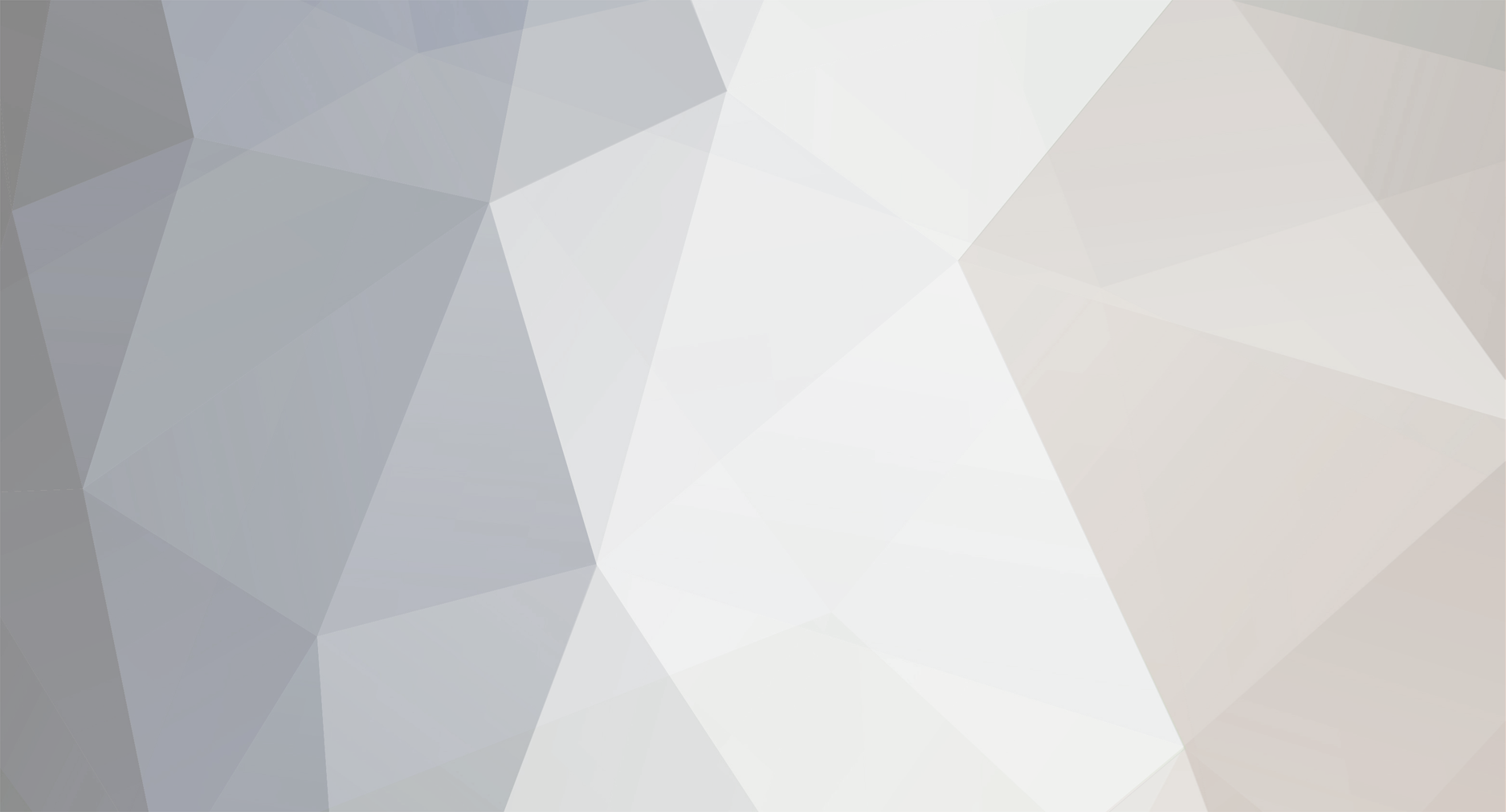
premiso
Members-
Posts
6,951 -
Joined
-
Last visited
-
Days Won
2
Everything posted by premiso
-
<?php $nick = $_SESSION["myusername2"]; echo "Username is: " . $nick; $query="SELECT `access` FROM `user` WHERE `username` = '$nick'"; $result=mysql_query($query)) or die(mysql_error()); // error here fixed $num = mysql_num_rows($result); $result = mysql_fetch_array($result); print_r($result); $isAdmin = ($result['access'] == "admin"); if ($isAdmin) echo "admin test"; else echo "members test"; ?> Try some debugging procedures, make sure what is expected to check against is being checked and that you are getting a result. Also check the case on admin as php is CaSeSenSitIvE.
-
To completely kill a session you have to unset the session cookie. www.php.net/session localhost/test_site/index.php?id1=value&act=title&bob=two&three=four
-
<?php mysql_query("DROP DATABASE $table"); mysql_query("CREATE DATABASE $table"); // need to select database mysql_select_db($table); $fh = file('db_data.sql'); foreach ($fh as $line) { if (trim($line) != "") { mysql_query($line) or echo 'Error: ' . mysql_error() . '<br />'; } } Give that a try.
-
$nick = $_SESSION["myusername2"]; $query="SELECT `access` FROM `user` WHERE `username` = '$nick'"; $result=mysql_query($query)) or die(mysql_error(); $num = mysql_num_rows($result); $result = mysql_fetch_array($result); $isAdmin = ($result['access'] == "admin"); if ($isAdmin) echo "admin test"; else echo "members test"; Forgot to fetch the array of the query result, try that.
-
<?php mysql_query("DROP DATABASE $table"); mysql_query("CREATE DATABASE $table"); $fh = file('db_data.sql'); foreach ($fh as $line) { if (trim($line) != "") { mysql_query($line); } } That is the proper way to import. For the database issue, try using double quotes instead of single. Also make sure you have create/drop privileges. Reason being is that mysql_query can only execute one line at a time. It cannot do multiple statements (unless I am incorrect which is possible) that is just my memory talking.
-
if (isset($aFieldValues['SCOMPANY']) && $aFieldValues['SCOMPANY'] != '') { $aShipTo['COMPANY'] = $aFieldValues['SCOMPANY'] }elseif (isset($aFieldValues['COMPANY']) && $aFieldValues['COMPANY'] != '') { $aShipTo['COMPANY'] = $aFieldValues['COMPANY'] }else { $aShipTo['COMPANY'] = $aFieldValues['SFCONTACT'] . ' ' . $aFieldValues['SLCONTACT']; } Like you had it before. For more complex issues you really shouldn't use the ternary operator as you stated it can get confusing to others who look at your code. It was designed to handle small if's that did not need much logic to them.
-
Can you run a PHP script using JS?? (I know it's weird)
premiso replied to markis's topic in PHP Coding Help
You want to look into using AJAX. -
Using the $_GET['src'] where needed: <?php echo '<a href="http://www.someoensdomain.com/?src=' . $_GET['src'] . '">Click Here</a>'; echo '<br /><form method="post" action="site.php?src=' . $_GET['src'] . '"><input type="hidden" src="' . $_GET['src'] . '" name="src" /><input type="submit" name="submit" value="Submit!" /></form>'; ?> I think that is what you are looking for...?
-
Will that still work, even if the images are being displayed on a foum? or on some other website? Should it takes any request and routes it through images.php
-
A suggestion to avoid that happening, is do a check with Javascript for < > or </ > and unlimited spaces. If that is present throw an alert and halt the page from processing. Kinda sucks that the host did that, but it is understandable due to xss reasons. You could even use javascript to convert < to its html counter part < and > which would allow that to be processed without the user knowing what happened.
-
PHP Automated Event Reminder - Is It Possible?
premiso replied to phpQuestioner's topic in PHP Coding Help
Cronjob is what you want, AJAX will not help as it would require client interaction. If you are on a Win host look into Task Scheduler. -
This thread should be stickied as "How NOT to ask Questions".
-
I dunno, I have never seen this error, and I cannot reproduce it on any of my servers. I would look at your php.ini config (phpinfo()) and look for anything that might cause issues. Other than that, contact your hosting company and ask what the heck.
-
Something to try, is see if magic quote are on. I would also contact your webserver and see what they say. It seems like it is a core issue in the php.ini or maybe even in the webserver (apache or IIS).
-
Ah so the problem is that when a user INPUTS </ > it redirects them to a page they should not goto. That is very weird, I am not sure what or why that would be doing that... Try adding die(); at the end of the enquiry file...see if that helps?
-
[SOLVED] A small problem with variables, help please :)
premiso replied to MsRedImp's topic in PHP Coding Help
Your not working within <?php <?php $team1 = "Germany"; ?> <link rel="teams.css" type="text/css" /> <style type="text/css"> input{ text-align:center; } </style> </head> <body> <form action="post2.php" method="post"> <div style="position:absolute; top:195px; left:470px"><input type="submit" value="Enter"/></div> </form> <div style="position:absolute; top:143px; left:371px; text-align:center; width: 256px; height: 31px;"> <table width="250" border="0"> <tr> <td width="80"> <style type="text/css"> <?php echo "$team1"; ?> </style> <style type="text/css"> <?php echo $team1;?> </style> <?php echo "$team1"?> </td> <td width="76"><input name="selection" type="text" size="1" maxlength="1"/><input name="selection1" type="text" size="1" maxlength="1"/></td> <td width="80">England</td> </tr> </table> -
Faster should be determined by where you live. *nix will be cheaper than Windows and more secure. Just about anything works, depends on what you need. You should not pay more than $7/month for PHP and a database depending on your needs.
-
Tested and works on Firefox... As a side note this may be screwing you up: <form action="enquiry_sender.php" method="post"/> should be <form action="enquiry_sender.php" method="post"> The ending slash may be killing the form prematurely as that is not proper HTML and IE may interpret that where as firefox does not.
-
You need the local ip address and or computer name. IE: http://192.168.0.4/file.php or http://YOURCOMPUTERNAME/file.php Should both work.
-
If he wants to add an access level that would work, but it seemed to me he just wanted to say, "Is this user and password correct?". Checking if a user has proper access level, well he would probably want to return the access instead if that is true this would probably be a better way: <?php function auth($user, $pass){ $sql = mysql_fetch_assoc(mysql_query("SELECT username, access FROM `". MEMBERS ."` WHERE `username` = '". $user ."' AND `password` = '". md5($pass) ."'")); return ($sql['username'] == $user)?$sql['access']:false; } if (auth($user, $pass)== 3) { echo "Welcome Admin $user"; }elseif (auth($user, $pass) ==2) { echo "Welcome $user"; }else{ echo "Not logged in."; } ?> Either way, it is always nice to have more than one solution to choose from for what might suit their needs, and make their life easier.
-
Running Scripts to Update DB from 1 Parent Script
premiso replied to ThePilots81's topic in PHP Coding Help
www.php.net/include If x was selected then include('page.php'); That is what you will need, like phreeek said, if/then/else logic and INCLUDE the script. -
You want javascript and/or ajax. As php has no control over the page after it has been processed.