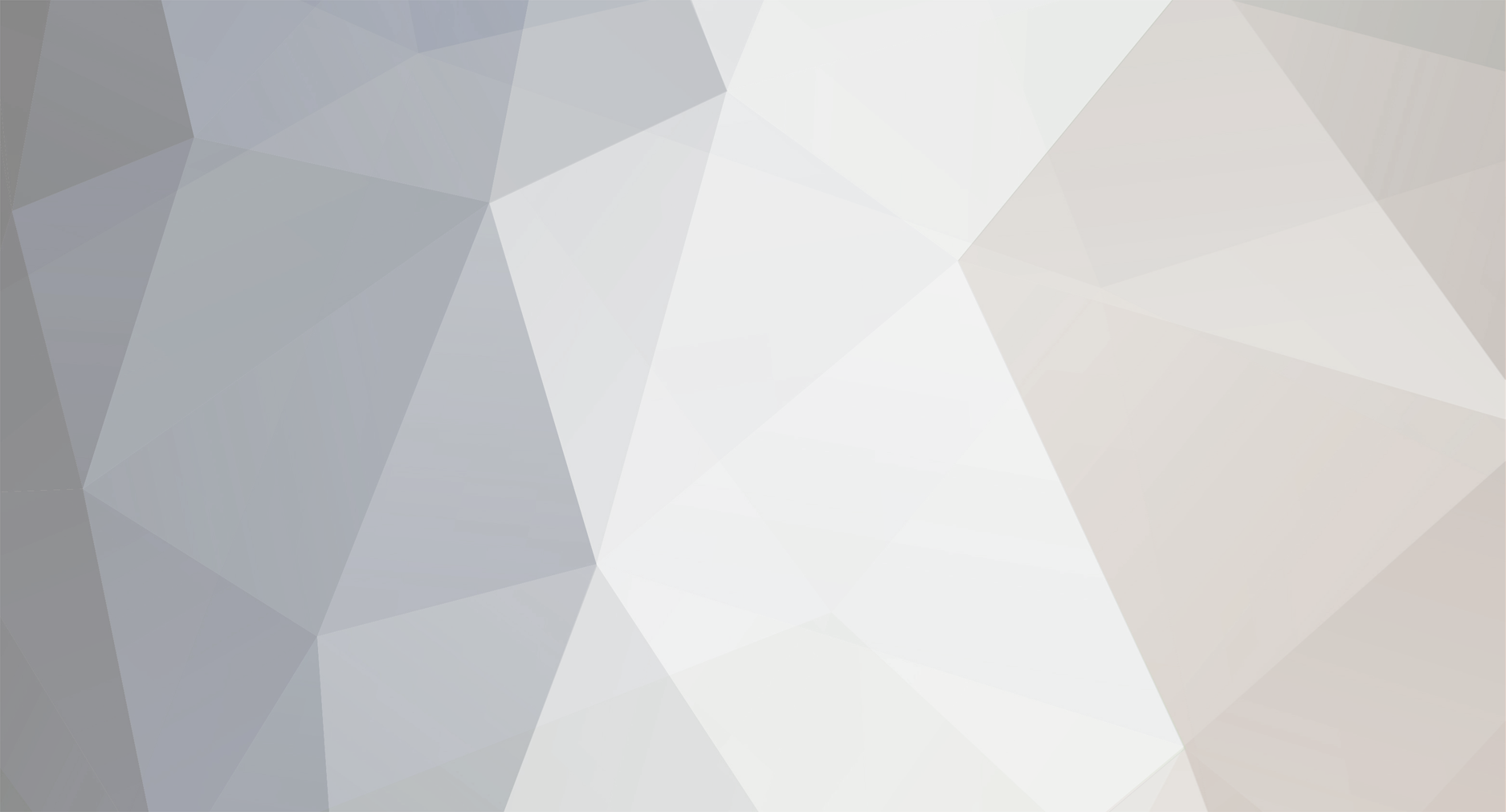
premiso
Members-
Posts
6,951 -
Joined
-
Last visited
-
Days Won
2
Everything posted by premiso
-
You were doing some weird stuff with the single quotes. Here is a corrected version: return str_replace(array('&', '>', '<', '"', "'"), array('&', '>', '<', '"', "'"), str_replace(array('>', '<', '"', "'", '&'), array('>', '<', '"', "'", '&'), $var)); // line 36
-
Reading attributes from single line mySQL query
premiso replied to Nomadic's topic in PHP Coding Help
It is a valid usage. For instance, say I wanted to get one row from a mysql query assigned and wanted to test if it assigned correctly: $query = mysql_query("SELECT * FROM table_name WHERE 0=1"); // expected 0 results, but a valid query. if ($row = mysql_fetch_assoc($query)) { echo 'This should not be true.'; }else { echo 'The result was not returned.'; } Not weird, intentional and has it's uses. -
Sorry, here is a corrected add.php as I got confused and it was doing some weird stuff (1 upload worked but mutiple would not). This version does work, I changed how the $files array is structured / populated. Questions let me know: <?php $dir_upload = $_SERVER['DOCUMENT_ROOT']; $uploadto = $_POST['uploadto']; $upload_path = $dir_upload.$uploadto; // Copy global $_FILES array to new $files array and strip out any empty objects $files = array(); $x = 0; foreach ($_FILES['userfile']['error'] as $key => $value) { if ($value == 0 ) { $files[$x]['tmp_name'] = $_FILES['userfile']['tmp_name'][$key]; $files[$x]['name'] = $_FILES['userfile']['name'][$key]; $x++; } } // Count number of files uploade for ($i = 0; $i < $x; $i++) { // Shorten variable names $tmp_file = $files[$i]['tmp_name']; $filename = $files[$i]['name']; // If the upload was successful... if (is_uploaded_file($tmp_file)) { $move = move_uploaded_file($tmp_file, $dir_upload . $uploadto . $filename); echo $dir_upload . $uploadto . $filename; if($move) { echo "<center>The file name: $filename has been uploaded sucessfully<br><br><b>The File URL for $filename is: <a href=\"nothing here yet\"></a></b><br><hr>"; } // ... otherwise, print an error else { echo "Error uploading file <b>$filename</b>.<br><br>"; } }else { echo "Error: Not an uploaded file"; } } ?>
-
I have never used copy for uploaded files mainly because there is a move_uploaded_file which you would not have to use unlink after. Given that I found a few different issues, the main one was you were not giving the tmp_file location to the copy. Here is a revamped script, encoupled with $_SERVER['DOCUMENT_ROOT'] so it will work on other servers then your own. add.php: <?php $dir_upload = $_SERVER['DOCUMENT_ROOT']; $uploadto = $_POST['uploadto']; $upload_path = $dir_upload.$uploadto; // Copy global $_FILES array to new $files array and strip out any empty objects $files = array(); $x = 0; foreach ($_FILES as $key => $value) { if ((!is_null($value)) && ($value !== "") ) { $files[$x] = $value; $x++; } } // Count number of files uploaded $num_files = count($files[0]['tmp_name']); for ($i = 0; $i < $num_files; $i++) { // Shorten variable names $tmp_file = $files[0]['tmp_name'][$i]; $filename = $files[0]['name'][$i]; // If the upload was successful... if (is_uploaded_file($tmp_file)) { $move = move_uploaded_file($tmp_file, $dir_upload . $uploadto . $filename); echo $dir_upload . $uploadto . $filename; if($move) { echo "<center>The file name: $filename has been uploaded sucessfully<br><br><b>The File URL for $filename is: <a href=\"nothing here yet\"></a></b><br><hr>"; } // ... otherwise, print an error else { echo "Error uploading file <b>$filename</b>.<br><br>"; } }else { echo "Error: Not an uploaded file"; } } ?> upload.php <script type="text/javascript"> var i = 1; function add_file(file) { var j = i - 1; var box = document.getElementById('file_list'); var num = box.length; var file_exists = 0; for (x = 0; x < num; x++) { if (box.options[x].text == file) { alert('This file has already been added to the list.'); document.getElementById('file_' + j).value = ""; file_exists = 1; break; } } if (file_exists == 0) { // For Internet Explorer try { el = document.createElement('<input type="file" name="userfile[]" id="file_' + i + '" onChange="javascript:add_file(this.value);">'); } // For other browsers catch (e) { el = document.createElement('input'); el.setAttribute('type', 'file'); el.setAttribute('name', 'userfile[]'); el.setAttribute('id', 'file_' + i); el.setAttribute('onChange', 'javascript:add_file(this.value);'); } document.getElementById('file_' + j).style.display = 'none'; if (document.getElementById('list_div').style.display == 'none') { document.getElementById('list_div').style.display = 'block'; } document.getElementById('files_div').appendChild(el); box.options[num] = new Option(file, 'file_' + j); i++; } } function remove_file() { var box = document.getElementById('file_list'); if (box.selectedIndex != -1) { var value = box.options[box.selectedIndex].value; var child = document.getElementById(value); box.options[box.selectedIndex] = null; document.getElementById('files_div').removeChild(child); if (box.length == 0) { document.getElementById('list_div').style.display = 'none'; } } else { alert('You must first select a file from the list.'); } } function do_submit() { // Uncomment this block for the real onSubmit code /* var box = document.getElementById('file_list'); var max_files = 5; if (box.length <= max_files) { var child = document.getElementById('file_' + (i - 1)); div = document.getElementById('files_div'); div.removeChild(child); div.style.display = 'none'; return true; } else { alert('You have more files listed than the maximum allowed.\nPlease limit your upload files to no more than <? echo $upload_max_files; ?> at a time.'); return false; } */ // Just for test page alert('Files uploaded successfully'); return false; } </script> <form enctype="multipart/form-data" action="add.php" method="post"> <div name="files_div" id="files_div"> Select a File: <input type="file" name="userfile[]" id="file_0" onChange="javascript:add_file(this.value);"> </div> <br><br> <div name="list_div" id="list_div" style="display: none;"> <select multiple name="file_list" id="file_list"></select> <br><br> <input type="button" id="remove_file_btn" value="Remove Selected" onClick="javascript:remove_file();"> <br> <br><br>Destination to Upload (?): <select name="uploadto"> <option>uploads/</option> <?php function getDirectory( $path = '.', $level = 0 ) { // Directories to ignore when listing output. $ignore = array( '.', '..' ); // Open the directory to the handle $dh $dh = @opendir( $path ); // Loop through the directory while( false !== ( $file = readdir( $dh ) ) ) { // Check that this file is not to be ignored if( !in_array( $file, $ignore ) ) { // Show directories only if(is_dir( "$path/$file" ) ) { // Re-call this same function but on a new directory. // this is what makes function recursive. $slash = "/"; echo "<option>$file$slash</option>"; getDirectory( "$path/$file", ($level+1) ); } } } // Close the directory handle closedir( $dh ); } // Your start directory getDirectory($_SERVER['DOCUMENT_ROOT'] . 'uploads/'); ?> </select> <input type="Submit" name="upload" value="Upload"> I tested it and it worked alright on my server. EDIT: Modified the count statement to be correct.
-
You do have a syntax error here : echo "<center>The file name: $filename has been uploaded sucessfully<br><br><b>The File URL for $filename is: <a href=\"nothing here yet"\></a></b><br><hr>"; Change it to: echo "<center>The file name: $filename has been uploaded sucessfully<br><br><b>The File URL for $filename is: <a href=\"nothing here yet\"></a></b><br><hr>"; Not sure if that was the error you were talking about, as you never stated what error you were getting.
-
Not un-heard of, but if you post the code you may get an answer, as it could be an issue with the code.
-
The error you provided does not match your code. As it seems you are trying to include a urlencoded string, but you forgot to exit out of the current string. As for the first statement, you need a space before the WHERE or after the FROM device for that to work properly. But yea, show us the proper code to solve your issue.
-
Nope, you notice your MySQL error now has the tblProducts in it? The issue is you are not selecting any data from the table. If you change it to SELECT * FROM or put the columns you want in place of the star it should work.
-
if ($pages != $startrow) { $next = $startrow + $display; echo '<a href="testing2.php?startrow=' . $next . '">Next</a> '; } Given that $startrow is the current page, that should do it.
-
Using an initialzed class inside a funtion without using global
premiso replied to djones's topic in PHP Coding Help
One reason he may not want to (which I am not 100% sure happens) is passing it as an argument creates a new object? You may want to try and make the class global global $oClass; $oClass = new class(); function new($id) { global $oClass; } However, like I said, I am not sure if this is what you want or if it is considered good practice, but it is another option for you. -
Ok, read what I write please and look at the link. Variables Scope means that you can only use variables that are: A. Global B. Super Global C. Defined in the Function D. Passed in as a parameter. Given this, you do not have $dbProductsTable defined, passed in or set as a global. Thus it is not set in the function. To fix this error, you either have to define $dbProductsTable as a global or pass it in as a parameter. Here is an example of each: function isValidProduct($input, $dbProductsTable) { $test = mysql_query("SELECT FROM ".$dbProductsTable." WHERE articleNumber=".QuoteSmart(trim($input))) or trigger_error("Query Failed: " . mysql_error()); if (mysql_num_rows($test) > 0) { return TRUE; } return FALSE; } // where the $dbProductsTable variable is defined: global $dbProductsTable; $dbProductsTable = 'products'; function isValidProduct($input) { global $dbProductsTable; $test = mysql_query("SELECT FROM ".$dbProductsTable." WHERE articleNumber=".QuoteSmart(trim($input))) or trigger_error("Query Failed: " . mysql_error()); if (mysql_num_rows($test) > 0) { return TRUE; } return FALSE; } Or a 3rd option, making it a CONSTANT with define // where the $dbProductsTable variable is defined change to: define('DB_PRODUCTS_TABLE', 'products'); function isValidProduct($input) { $test = mysql_query("SELECT FROM ". DB_PRODUCTS_TABLE ." WHERE articleNumber=".QuoteSmart(trim($input))) or trigger_error("Query Failed: " . mysql_error()); if (mysql_num_rows($test) > 0) { return TRUE; } return FALSE; } Please read this thoroughly: http://php.net/manual/en/language.variables.scope.php
-
I never said you were. Yes, it is a function, and I do not doubt you have it defined. But it is not defined in the scope of the function, read my whole line for more information on Variables Scope (note that was a link above).
-
Reading attributes from single line mySQL query
premiso replied to Nomadic's topic in PHP Coding Help
It is always assigning "t" because you are using the assignment operator ( = ). Change this to the comparison operator ( == ) for it to come out correct. if($row['ADMIN'] == "t") { $_SESSION['ADMIN'] = "t"; } -
Fatal error: Cannot redeclare escape_data() (previously declared)
premiso replied to BrentonHale's topic in PHP Coding Help
Usually when you get the connection is reset it means there is or was an infinite loop. Check the other included files and make sure that there are no infinite loops. Also, I would restart your apache server. If this is on a Shared remote host, it could be someone else's script and it may just be your host. -
Change the query line to this: $test = mysql_query("SELECT FROM ".$dbProductsTable." WHERE articleNumber=".QuoteSmart(trim($input))) or trigger_error("Query Failed: " . mysql_error()); The issue is going to be with $dbProductsTable, as it has not been defined within the scope of the function. Check out Variables Scope for more indepth information.
-
Your problem lies within Variable Scopes You define $urlArray outside of the function and it has to be either global, passed as a parameter or defined inside the function to use it there.
-
For this situation isset does not work, cause either way $var is going to be set to a resource or a boolean. mysql_query will tell you what it will return. Returns false on error and Resource on a successful query. Where isset is valid is usually in POST / GET data to test if you received a certain data item and allows you to assign it without causing a notice error.
-
Please do not post the same question in two different places, especially when the first spot was more or less the proper location.
-
$var = mysql_query("SELECT articleNumber FROM tblProducts WHERE articleNumber=".$TestProduct); if (mysql_num_rows($var) > 0) { // field exists } Will tell you if more than 0 rows were returned (IE id number exists).
-
Fatal error: Cannot redeclare escape_data() (previously declared)
premiso replied to BrentonHale's topic in PHP Coding Help
So you removed all of: // Create function for escaping the data. function escape_data ($data) { global $dbc; // Need the connection. if (ini_get('magic_quotes_gpc')) { $data = stripslashes($data); } return mysql_real_escape_string (trim($data), $dbc); } // End of function. ? If not remove that whole section. If so, please point out what line 99 is. -
Alright, this should be a bit quicker then your old method and perhaps will process more data. I am not sure, as there are still 2 queries that have to be looped due to their nature (I am still trying to think of a way around this). If you have any questions about it let me know. I added the trigger_error for the error reports, used a syntax in MySQL called ON DUPLICATE KEY UPDATE which will allow for 1 less query again and used mysql_insert_id instead of running another query to grab that data. Hopefully it works, unsure as I do not have the system to test it, so pending any syntaxual / SQL issues it should work. <?php $get_data = mysql_query("SELECT id, tweets FROM raw_data WHERE processed = '0' ORDER BY `raw_data`.`id` ASC") or trigger_error('RawDataQueryFailed: ' . mysql_error()); $ids = array(); $users = array(); $insertTweet = array(); $x=0; while($r = mysql_fetch_array($get_data)){ $id = $ids[] = $r['id']; $tweet = json_decode($r['tweets']); if ($tweet->user->screen_name != '' && $tweet->text != ''){ $date = date("U"); $user = $users[] = mysql_real_escape_string($tweet->user->screen_name); $twt = mysql_real_escape_string($tweet->text); $prof_img = $tweet->user->profile_image_url; $link = explode(' ', $tweet->text); foreach ($link as $li){ $li = str_replace(')', '', $li); $li = str_replace('(', '', $li); $pos = substr($li, 0, 4); if( $pos == 'http' ){ $link = $li; if (substr($link, 7, 6) == 'bit.ly'){ // if bit.ly link $long_url = bitly_convert($link); } }else { $link = 'not found'; } } // insert data into db if ($link != 'not found'){ // Record link // $insertTweet[$user] = array('link' => $link, 'long_url' => $long_url, 'user' => $user, 'twt' => $twt, 'prof_img' => $prof_img); $userQuery[] = "('$user', '$prof_img')"; } } } mysql_query("INSERT INTO users (`username`, `profile_img`) VALUES " . implode(', ', $userQuery) . " ON DUPLICATE KEY UPDATE `profile_img` = VALUES(`profile_img`)") or trigger_error("UserQuery Failed: " . mysql_error()); $relations = array(); foreach ($insertTweet as $user => $tweetData) { $link_id = $tweet_id = 0; $sql = mysql_query("INSERT INTO link (url, count, long_url, first_seen, last_seen) VALUES ('{$tweetData['link']}', '1', '{$tweetData['long_url'}', '$date', '$date') ON DUPLICATE KEY UPDATE id=LAST_INSERT_ID(id), count = count+1, last_seen = VALUES(last_seen)") or trigger_error("LinkQuery Failed: " . mysql_error()); if ($sql) { $link_id = mysql_insert_id(); $sql = mysql_query("INSERT INTO tweets (tweet, date, link_id, username) VALUES ('{$tweetData['twt']}', '$date', '$link_id', '$user') ON DUPLICATE KEY UPDATE id=LAST_INSERT_ID(id)") or trigger_error("TweetQuery Failed: " . mysql_error()); if ($sql) { $tweet_id = mysql_insert_id(); $relations[] = "('{$tweetData[$user]}', '$tweet_id', '$link_id')"; } }else { continue; } } mysql_query("INSERT INTO relationships (username, tweet_id, link_id) VALUES " . implode(", ", $relations) . " ON DUPLICATE KEY UPDATE tweet_id = tweet_id") or trigger_error("RelationQuery Failed: " . mysql_error()); mysql_query("DELETE FROM raw_data WHERE id IN(" . implode(',', $ids) . ")") or trigger_error("DeleteQuery Failed: " . mysql_error()); ?>
-
Don't. Not worth the effort for a browser that is 2 versions prior. You are just creating undue heartache for yourself, as IE6 is just horrible. If I were to take a stab at why, it would be the clear:both; command and I bet IE6 does not recognize it.
-
I just tested that, it seems fine on my end, perhaps your browser's font setting is set to a higher setting than not? What browser / version are you using? Tested in FF 3.x and it worked just fine.
-
Fatal error: Cannot redeclare escape_data() (previously declared)
premiso replied to BrentonHale's topic in PHP Coding Help
Try changing your function in the mysql page to this: // Create a function for escaping the data. function escape_data ($data) { // Address Magic Quotes. if (get_magic_quotes_gpc()) { $data = stripslashes($data); } $data = trim($data); // Check for mysql_real_escape_string() support. if (function_exists('mysql_real_escape_string')) { $data = mysql_real_escape_string ($data); }else { $data = mysql_escape_string ($data); } // return the escaped value. return $data; } // End of function. Just re-vamped it a bit and removed the global $dbc, as that is only needed if you are using MySQLi, as the connection is global in itself. -
The query is slowing you down. Store any processed id in an array and do the update separate. $ids = array(); while($r = mysql_fetch_array($get_data)){ $iidd = $ids[] = $r['id']; $tweet = json_decode($r['tweets']); //$update = mysql_query("UPDATE raw_data SET processed = '1' WHERE id = '$iidd'"); // your other code here. } // end while mysql_query("UPDATE raw_data SET processed = '1' WHERE id IN(" . implode(',', $ids) . ")") or trigger_error("Query Failed: " . mysql_error()); Now given that, it seems you omitted a good chunk of code, which I have my suspicions does an insert query. If you would like this tweaked for that as well post the rest of that code. But you optimize the querys / take them out of the loop you will be able to process a ton more than just 1400 a minute.